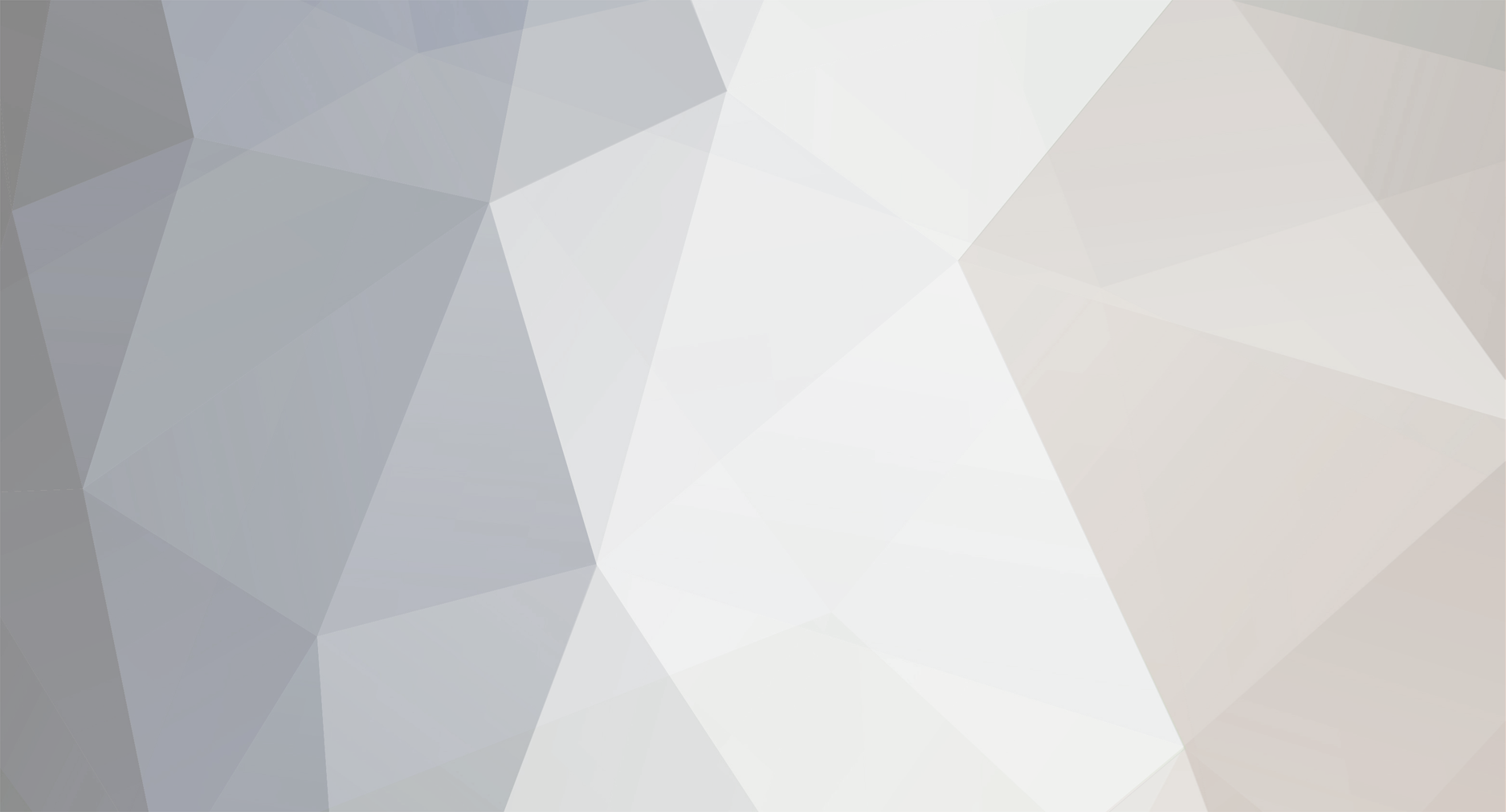
melloorr
Members-
Posts
142 -
Joined
-
Last visited
Never
Everything posted by melloorr
-
I have not tested it, but does this work? <?php error_reporting(E_ALL); if(isset($submit)) { extract ($_POST); $Recipient = "$email"; $MsgSubject = "message subject"; $MsgBody = "message body."; mail($Recipient, $MsgSubject, $MsgBody); echo " thank you for your order. It is being processed. Thank you for your business."; } else { echo " Error sending email"; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> </head> <body> <form action=" <?php echo $_SERVER['PHP_SELF']?>" method="post" name="test"> Name: <input name="name " type="text" id="name " size="8" /><br /> email <input name="email" type="text" size="9" maxlength="30" /><br /> <input name="submit" type="submit" value="submit" /> </form> </body> </html>
-
Just have: mail($Recipient, $MsgSubject, $MsgBody) And if you need their email address, just put it inside the email
-
I just took out the 'from' function completely, now it just says that it is being sent by my godaddy email address
-
sorry for double post
-
Are you getting any errors? Also is it a local host or are you using a service? Because I had this problem today, and it was because godaddy does not send emails the the 'from' in the mail() function if it is from hotmail.com, yahoo.com, gmail.com, etc.
-
Well, when you store the post in the database, store the username at the same time. For example: ("INSERT INTO posts (id, post, username) VALUES ('$id, $post, $username' "); Something along the lines of that. So when you store the username in the cookie and use the other code I posted, it checks that username against the database, and returns any post that was created by that user
-
This code will get rid of the errors, and I also noticed that your form action was "post.php" (which I am assuming is the very same file) so I have changed that too: <?php session_start(); include('db_connect.php'); ?> Welcome to the fear blog <a href="logout.php">log out</a><hr/> <?php if(isset($_COOKIE["username"]; { $username = $_COOKIE["username"]; $sql = mysql_query("SELECT * FROM posts WHERE username = '$username' ORDER BY id "); while($row = mysql_fetch_array($sql)){ $title = $row['title']; $content = $row['content']; $category = $row['category']; ?> <div id="post"> <div id="wrapper"> <div id="title"> <label>Title</label> <?php echo $title; ?> </div> <div id="category"><label>category</label> <?php echo $category; ?> </div> <div id="content"> <label>Content</label><?php echo $content; ?> </div> </div> <?php } } ?> <div id="contents"> <form action="<?php echo $_SERVER['PHP_SELF']?>" method="post"> <label> Title:</label><input type="text" name="title" /><br/> <label> Category:</label><input type="text" name="category" /><br /> <label> Content:</label><textarea name="content"></textarea><br/> <input type="submit" name="submit" value="Post"/> </form> </div> </div> </div> </div>
-
When they log in,set a cookie setcookie(username, $username, time() 3600); If you are storing their username in the database, next to their post, this this SHOULD work: <?php session_start(); include('db_connect.php'); ?> Welcome to the fear blog <a href="logout.php">log out</a><hr/> <?php $username = $_COOKIE["username"]; $sql = mysql_query("SELECT * FROM posts WHERE username = '$username' ORDER BY id "); while($row = mysql_fetch_array($sql)){ $title = $row['title']; $content = $row['content']; $category = $row['category']; ?> <div id="post"> <div id="wrapper"> <div id="title"> <label>Title</label> <?php echo $title; ?> </div> <div id="category"><label>category</label> <?php echo $category; ?> </div> <div id="content"> <label>Content</label><?php echo $content; ?> </div> </div> <?php } ?> <div id="contents"> <form action="post.php" method="post"> <label> Title:</label><input type="text" name="title" /><br/> <label> Category:</label><input type="text" name="category" /><br /> <label> Content:</label><textarea name="content"></textarea><br/> <input type="submit" name="submit" value="Post"/> </form> </div> </div> </div> </div> But this is just very basic, you should probably have more security, and you will probs get errors if they are not logged in
-
Store the username in the database along with the post, (and also in a cookie or a session or something) then: ("SELECT * FROM posts WHERE username = '$username' ORDER BY id "); Something like that?
-
I don't understand what you mean. Could you give an example?
-
How about storing the images in a database, along with the right captcha then use RAND() to call a random one each time?
-
See if this works: [code]<?php session_start(); $dbuser = "root"; $dbpass = ""; $dbhost = "localhost"; $dbname = "escola_musica"; $connect = mysql_connect($dbhost, $dbuser, $dbpass) or die("Erro na ligação à Base de Dados."); $username = $_POST['username']; $pass = $_POST['pass']; mysql_select_db($dbname, $connect); $sql="SELECT username, password FROM alunos WHERE username='". $username. "'"; $resultado = mysql_query($sql, $connect) or die(mysql_error()); $num = mysql_fetch_assoc($resultado); if ($username == $num['username'] AND $pass == $num['password']) { header("Location: index.php"); } else { header("Location: login_form.php"); } mysql_close($con); ?> [/code]
-
It doesn't make sense. At the top of you code you have the variable $username as "root" which is to log into you database, and at the bottom you have this: if ($num > 0 AND ($username == true AND $pass == true)) { header("Location: index.php"); } else { header("Location: login_form.php"); } Which, i THINK says, if you have the variable $username, then you can log in (same goes for password) Also, do you have a login form? If yes post the code please
-
confused, Im gettinbg double entries in text file
melloorr replied to bakhtn's topic in PHP Coding Help
Try closing the While statement before the if statement -
Never mind I have solved it now
-
Hey everyone. I am trying to use a database for a simple search script, which searches tags. But I want to make is so, if the user searches "cars" then the database returns any value that has "cars" in it, even if the tags column has other words in it. If you know what I mean. Basically, if the column has 'bike car boat' in it, and I used the WHERE function to search for cars, then that value would not appear, even though I would like it to. How can I get around this?? Thanks EDIT Its Server version: 5.5.16
-
Now I feel pretty stupid because it seems so obvious :-\ Thanks you for all the help
-
Hey everyone. I have been trying to assign dates to image so I could sort them, and also display the date they were uploaded. But I need some help. I have a script that uploads an image to a file directory, and also stores the file directory and the upload date in a database. And I also have a script that displays the images in the file directory but I cannot find a way to assign the right date to the right image. Here is the script that displays the images: if (isset($_POST['submit'])) { // fill an array with all items from a directory $dir = "thumbs/"; $handle = opendir($dir); while ($file = readdir($handle)) { $files[] = $file; // This removes the full stops from the array unset($files[0]); unset($files[1]); } foreach($files as $fileimg) { echo "<img src='". $dir . $fileimg ."'/>\n"; echo "$date"; } } and here is the script for calling the date from the database: $query = mysql_query("SELECT imgaddress, uploaddate FROM thumbs WHERE imgaddress='" . $dir . $file . "'"); if (mysql_num_rows($query) == 1) { $row = mysql_fetch_assoc($query); $date = $row['uploaddate']; If I use the database statement before the foreach, then it does not get the right file directory, but if I call it inside the foreach, then I cannot use krsort() to sort the array. Any help would be greatly appreciated, thanks
-
I have just been doing exactly what you are doing (with the same code too). And I have finally got it to work! <?php // fill an array with all items from a directory $dir = "thumbs/"; $handle = opendir($dir); while ($file = readdir($handle)) { $files[] = $file; // This removes the full stops from the array unset($files[0]); unset($files[1]); } foreach($files as $file) { echo "<img src='" . $dir . $file . "' />\n"; } ?>
-
I'm not sure I understand. You want to log into the site the login script is on, but also log into a different site at the same time, with the same login script?
-
Here is the link to the text file: http://testwebsitetesting.hostzi.com/phpfreaks.dat (it is .dat because 000webhost does not allow .txt) Hey everyone, I am trying to test out the security of my login script and I could use your help. So I need to you to try to hack into it and log into the user called: melloorr (I am not using passwords that I also use for anything else) http://testwebsitetesting.hostzi.com If you found it easy to hack into, or you want to offer advice on how I could improve it then just let me know Thanks
-
I know but the variable is set in a different file, and the whole point of this is to check if the if in the cookie is the same as the one in the database, and if it is not, then the cookie is deleted and they are logged out due to the cookie being deleted. All the code works as it is, they are logged out if the cookie is not correct, and they will have to log in again. It is all secure (i think). I may upload it to be beta tested But thanks for all your help
-
For this, you must do this if(!isset($_COOKIE['name'])) set_cookie('name', 'value'); else // Do something with existing COOKIE I do not understand this. That code is saying, if there is no cookie, then set one?
-
Yeah I was thinking that too. But the error_reporting(E_ALL) should have been at the bottom, not the top. And even without the error_reporting(E_ALL) at the botton, error would still only be turned off for that if statement only
-
I know. I have only disabled errors for the following code if($_COOKIE) { error_reporting(0); if (isset($_COOKIE['Key_my_site']) == $cookiedbid) { echo "Your cookie is okay."; } elseif (isset($_COOKIE['Key_my_site']) !== $cookiedbid) { header( "refresh:5;url=index.php" ); echo "You have been logged out because your cookie has been compromised"; setcookie(Key_my_site, 0, $past); } else { echo "Your cookie is no longer there."; } error_reporting(1); }