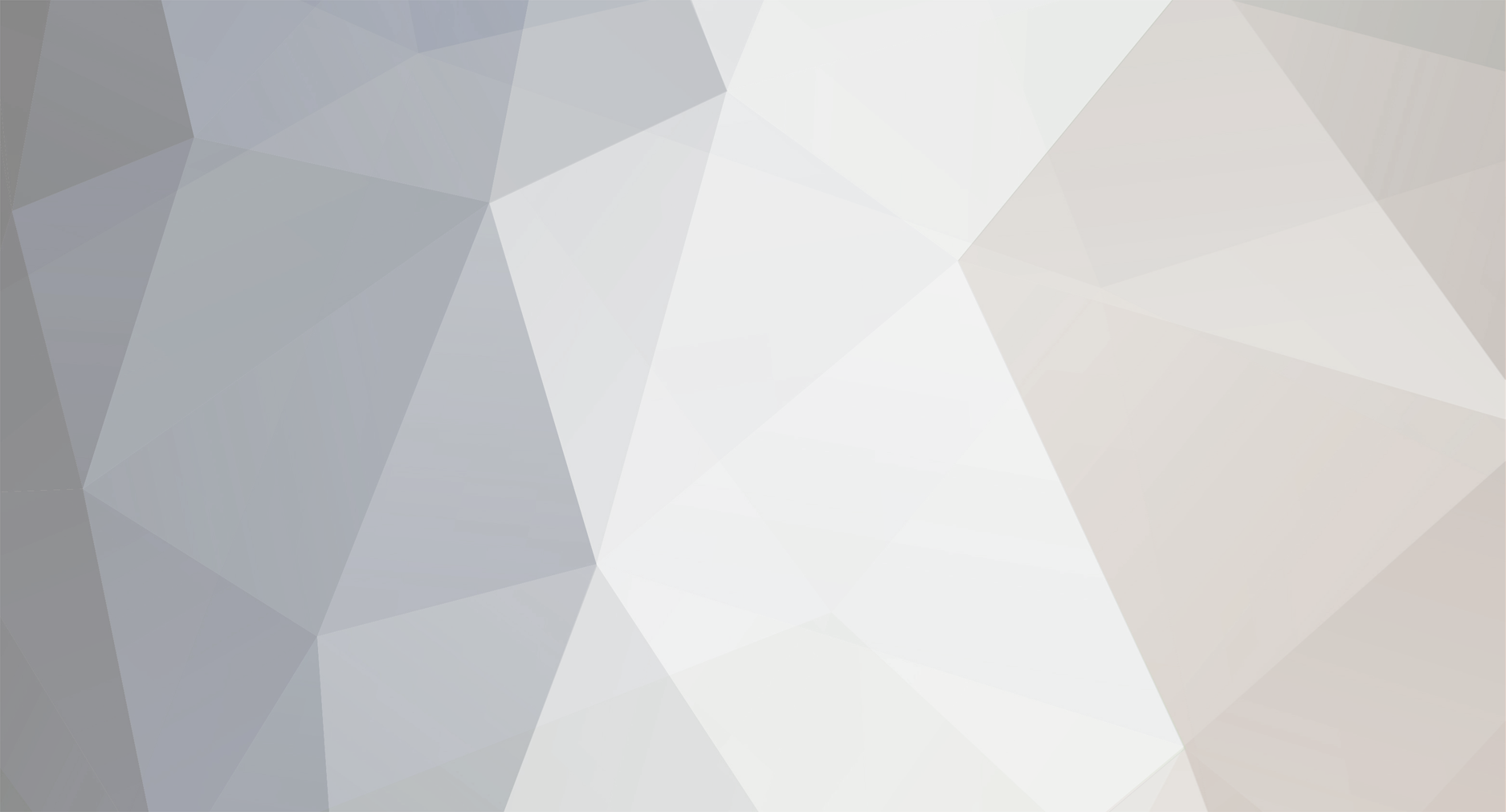
phdphd
Members-
Posts
248 -
Joined
-
Last visited
Everything posted by phdphd
-
Thanks to all!
-
Thanks.
-
Hi All, Among other data, my database stores passwords. In the case of individuals, the "forgotten password"/"secret question" approach is OK to recover the password. However, I am not sure for companies, where staff changes from day to day and not everyone would give the same answer to "what is the maiden name of your mother?". So suppose that for whatever reason a company loses the password - what is the best approach to recover it? Thanks for your opinion.
-
I guess this is because the JS is not dependent on an event being trigged.
-
Hi all, I managed to display multiple Google maps on same page. Here is my sample code (mix of html/js): <body> <script src="https://maps.googleapis.com/maps/api/js"></script> <script> function initialize(param1,param2,param3) { var mapCanvas = document.getElementById(param1); var mapOptions = { center: new google.maps.LatLng(param2, param3), zoom: 17, mapTypeId: google.maps.MapTypeId.ROADMAP } var map = new google.maps.Map(mapCanvas, mapOptions) }; </script> <div id="map_canvas"><script>initialize('map_canvas',51.5403,-2.5463);</script></div> <div id="map_canvas2"><script>initialize('map_canvas2',41.5403,-2.5463);</script></div> </body> This works. However it does not if I follow the best pratice of putting the js code at the end, right before the closing body tag. (On the actual page, the number of maps is not fixed, and varies upon user's choices.) Thanks for your help!
-
The use of possessive quantifiers is indeed very efficient! Thanks for this great tip.
-
Hi All, I have a preg_match that checks if a string is made up of a number, or of a comma-separated list of numbers. The regex is : ^[0-9]+(,[0-9]+)*$ When the form is processed, the browser crashes if the string is very long. (It does not if I just do not run the preg_match). I guess the solution might be adjusting the pcre.backtrack_limit setting. However I would like to know if there is a better solution than the above preg_match to check the string. Thanks.
-
thanks!
-
Hi all. I have some jquery that adds a class to siblings on hover and removes it when hovering ends. So this works [selector_syntax].hover( function(){$(this).parent().parent().siblings().addClass('fade');}, function(){$(this).parent().parent().siblings().removeClass('fade');} ) But this does not. [selector_syntax].hover( function(){$(this).parent().parent().siblings().addClass('fade');}, setTimeout(function(){$(this).parent().parent().siblings().removeClass('fade');}, 3000); ) Thanks for you help!
-
Mysqli : "There is no next result set" error message
phdphd replied to phdphd's topic in PHP Coding Help
Prepending mysqli_next_result with an @ would offer a visual solution, but would it be a good technical one ? -
Hi All, I get the following message when running a multiline query through mysqli. Here is my simplified code : $sql='SELECT CURRENT_USER();'; $sql.='SELECT CURRENT_time();'; $rs = @mysqli_multi_query($link,$sql); if (!$rs) { } else { do { if ($rs = mysqli_store_result($link)) { while ($row = mysqli_fetch_row($rs)) { echo "<br/> ". $row[0]; } mysqli_free_result($rs); } if (mysqli_more_results($link)) { echo "<br/>-----------------<br/>"; } } while (mysqli_next_result($link)); } If I use "while (mysqli_next_result($link) && mysqli_more_results($link));" instead of "while (mysqli_next_result($link))", I get no error message, but the current time (returned by the second query) won't display. Thanks for your help!
-
Selecting All Rows With Multiple Conditions In 2 Other Tables
phdphd replied to phdphd's topic in MySQL Help
Thank you! -
Selecting All Rows With Multiple Conditions In 2 Other Tables
phdphd replied to phdphd's topic in MySQL Help
Based on the fiddle provided, let's say the tables have following structure and contents: -- ----------------------------------------------------- -- Table `products` -- ----------------------------------------------------- CREATE TABLE IF NOT EXISTS `products` ( `id` INT NULL AUTO_INCREMENT, `name` VARCHAR(45) NULL, PRIMARY KEY (`id`)) ENGINE = InnoDB; -- ----------------------------------------------------- -- Table `properties` -- ----------------------------------------------------- CREATE TABLE IF NOT EXISTS `properties` ( `id` INT NOT NULL AUTO_INCREMENT, `product_id` INT NULL, `key` VARCHAR(45) NULL, `value` VARCHAR(45) NULL, PRIMARY KEY (`id`)) ENGINE = InnoDB; -- ----------------------------------------------------- -- Table `properties2` -- ----------------------------------------------------- CREATE TABLE IF NOT EXISTS `properties2` ( `id` INT NOT NULL AUTO_INCREMENT, `product_id` INT NULL, `continent` VARCHAR(45) NULL, `country` VARCHAR(45) NULL, PRIMARY KEY (`id`)) ENGINE = InnoDB; -- ----------------------------------------------------- -- Data for table `products` -- ----------------------------------------------------- INSERT INTO `products` (`id`, `name`) VALUES (1, 'English Book with AudioCD'); INSERT INTO `products` (`id`, `name`) VALUES (2, 'Polish Book'); -- ----------------------------------------------------- -- Data for table `properties` -- ----------------------------------------------------- INSERT INTO `properties` (`id`, `product_id`, `key`, `value`) VALUES (1, 1, 'Format', 'Book'); INSERT INTO `properties` (`id`, `product_id`, `key`, `value`) VALUES (2, 1, 'Format', 'Audio'); INSERT INTO `properties` (`id`, `product_id`, `key`, `value`) VALUES (3, 2, 'Format', 'Book'); INSERT INTO `properties` (`id`, `product_id`, `key`, `value`) VALUES (4, 1, 'Language', 'English'); INSERT INTO `properties` (`id`, `product_id`, `key`, `value`) VALUES (5, 2, 'Language', 'Polish'); -- ----------------------------------------------------- -- Data for table `properties2` -- ----------------------------------------------------- INSERT INTO `properties2` (`id`, `product_id`, `continent`, `country`) VALUES (1, 1, 'Asia', 'China'); INSERT INTO `properties2` (`id`, `product_id`, `continent`, `country`) VALUES (2, 1, 'Asia', 'India'); INSERT INTO `properties2` (`id`, `product_id`, `continent`, `country`) VALUES (3, 2, 'Asia', 'China'); INSERT INTO `properties2` (`id`, `product_id`, `continent`, `country`) VALUES (4, 1, 'Europe', 'France'); INSERT INTO `properties2` (`id`, `product_id`, `continent`, `country`) VALUES (5, 2, 'Europe', 'UK'); Let's say I want to find products in table "products" that have Format/Book and Format/Audio as key/value (table "properties"), and Asia/China and Asia/India as continent/country (table "properties2"). The following does not work : select p.id, p.name from products p inner join properties t on p.id = t.product_id inner join properties2 t2 on p.id = t2.product_id where t.value in ('Book', 'Audio') group by p.id, p.name having count(distinct t.value) = 2 and t2.country in ('China', 'India') group by p.id, p.name having count(distinct t2.country) = 2 I get the message 'check the manual that corresponds to your MySQL server version for the right syntax to use near 'group by p.id, p.name having count(distinct t2.country) = 2" Thanks. -
Hi All, I am trying to find a way to select all rows in one table that meet multiple conditions in 2 other tables, using a combination of inner join, group by, having count syntax. I know how to do it with just one other table, but not with 2. There is a good fiddle example with one table here : http://sqlfiddle.com/#!2/58b4b1/8 I tried to create and fill another table, then edit the existing query accordingly, but I always get a syntax error message. I do not even know if it is technically feasible. Thanks for your help !
-
Me again. I reopen the topic despite the main point was solved. Is it possible to prepend the div with a string? I tried to add the following at the beginning of the JS function, but it does not fire. var printElement = 'text to add before the div to print<br>'; printElement+=document.getElementById(el); Thanks.
-
Hi All, My page contains a JS function that enables to print the content of a DIV, and contains also a jQuery Autocomplete textbox that does not belong to that DIV. Here is the JS for printing the div function printContent(el){ var restorepage = document.body.innerHTML; var printcontent=document.getElementById(el).innerHTML; document.body.innerHTML = printcontent; window.print(); document.body.innerHTML = restorepage; } The HTML attached to it is <div id="div1">DIV 1 content... </div> <button onclick="printContent('div1')">Print Content</button> If the user clicks the button to print the content of the DIV, they cannot afterwards make use of the Autocomplete textbox. It seems the issue occurs with any kind of jQuery UI, not just autocomplete. In this case, the HTML of the Autocomplete is <div class="ui-widget"> <label for="tags">Tags: </label> <input id="tags" /> </div> and the script $(function () { var availableTags = [ "ActionScript", "AppleScript", "Asp", "BASIC", "C", "C++", "Clojure", "COBOL", "ColdFusion", "Erlang", "Fortran", "Groovy", "Haskell", "Java", "JavaScript", "Lisp", "Perl" ]; $("#tags").autocomplete({ source: availableTags, minLength: 0, delay: 0 }); }); jquery-ui-1.10.3.custom.css, jquery-1.9.1.js and jquery-ui-1.10.3.custom.js are used. Any idea of how to keep the jQuery UI functional after the div-printing JS function has been used ? Thanks for your help.
-
Hi all. I want to print a specific div when a button linked to it is clicked. Here is the code that works, even in Opera : function printContent(el){ var restorepage = document.body.innerHTML; printcontent=document.getElementById(el).innerHTML; document.body.innerHTML = printcontent; window.print(); document.body.innerHTML = restorepage; } However I want to add an image and some text before the content of the div. Here is the code that does not work in Opera : function printContent(el){ var restorepage = document.body.innerHTML; var printcontent = '<center><img alt="logo" src="image.jpg" height="160" width="240" /> BLABLABLA<br></center>'; printcontent+=document.getElementById(el).innerHTML; document.body.innerHTML = printcontent; window.print(); document.body.innerHTML = restorepage; } If I add just text instead of an image, the script runs normally. Thanks for your help.
-
I got it $input_table=array('1'=>'toto','2'=>'tota','3'=>'hello','4'=>'TOTO','5'=>'toto'); $tosearch='Tot'; $output_table = array_filter($input_table, function($v) use ($tosearch){return stripos($v, $tosearch)!==false;}); var_dump($output_table); Result : array(4) { [1]=> string(4) "toto" [2]=> string(4) "tota" [4]=> string(4) "TOTO" [5]=> string(4) "toto" }
-
Thank you. It works great. However, it does not work when using a variable as the string to look for (here "$to_search"): $input_table=array('1'=>'toto','2'=>'tota','3'=>'hello','4'=>'TOTO','5'=>'toto'); $to_search='Tot'; $output_table = array_filter($input_table, function($v){return stripos($v, $to_search)!==false;}); var_dump($output_table); I get array(0) { }
-
Hi All, I want to copy into a table values from another table that partially match a given value, case-insensitively. So far I do as follows but I wonder whether there is a quicker way. $input_table=array('1'=>'toto','2'=>'tota','3'=>'hello','4'=>'TOTO','5'=>'toto'); $input_table_2 = array_map('strtolower', $input_table); $value_to_look_for='Tot'; $value_to_look_for_2=strtolower($value_to_look_for); $output_table=array(); foreach ($input_table_2 as $k=>$v) { if(false !== strpos($v, $value_to_look_for_2)) { $output_table[]=$input_table[$k]; } } One drawback is that $input_table_2 is 'foreached' whereas there might be no occurrences, which would lead to a loss of time/resources for big arrays. Thanks.
-
Thanks a lot, CroNiX, it is working. Now the beginning of the JS looks like this (the only change is the insertion of "$('#some_other_input_id').val(ui.item.id);": jQuery(document).ready(function(){ $('#input_id').autocomplete({source:'my_jquery_suggest.php', select: function(event, ui) { $(event.target).val(ui.item.value); $('#some_other_input_id').val(ui.item.id); $('#form_id').submit(); return false; }, minLength:2,delay: 1000}).focus(function () { window.pageIndex = 0; $(this).autocomplete("search"); });