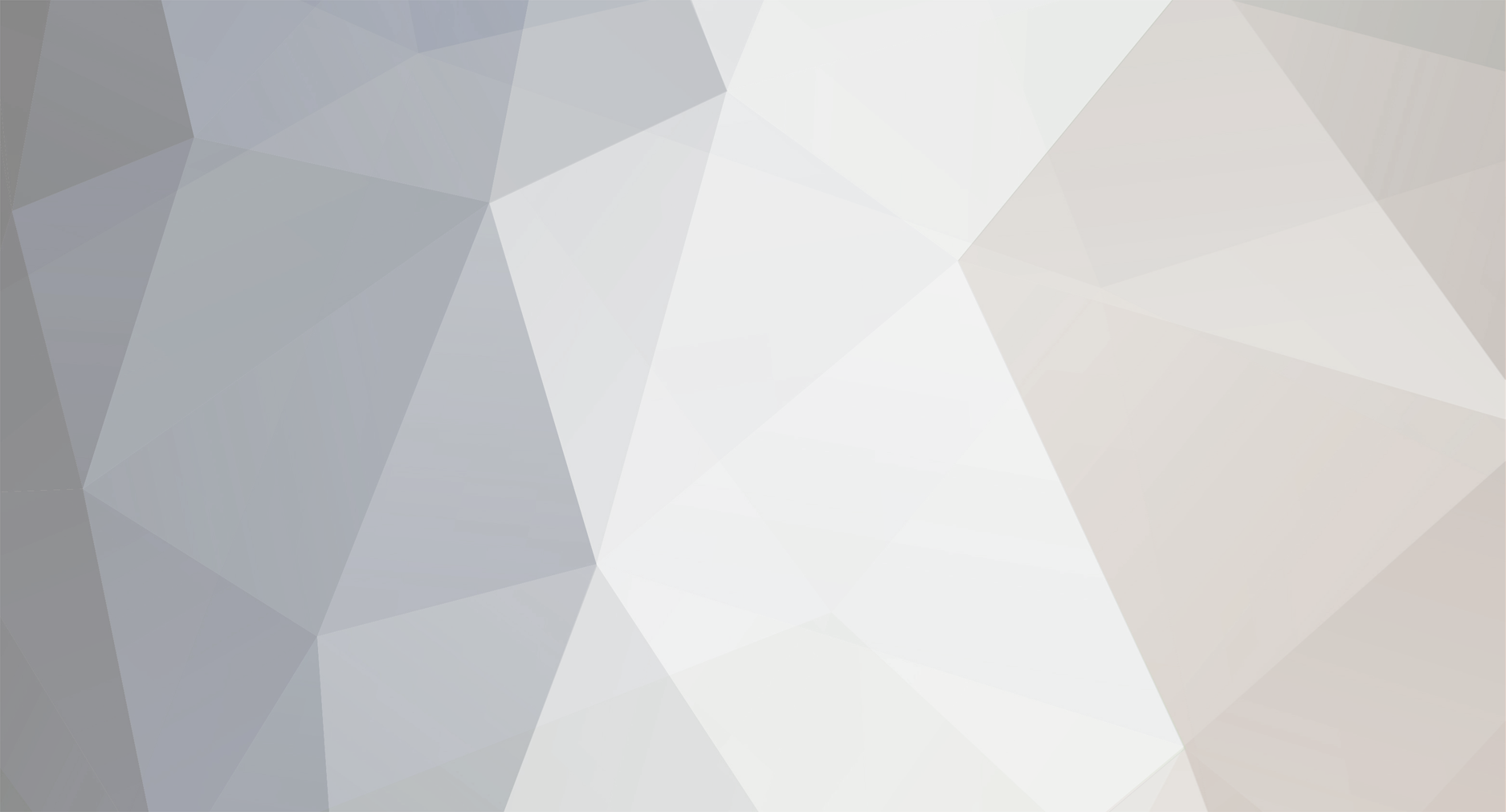
jazzman1
Staff Alumni-
Posts
2,713 -
Joined
-
Last visited
-
Days Won
12
Everything posted by jazzman1
-
In this case, I could use regEx, take a look at suggestions: // match any characters in the string $tshirt = "Tes-t, ... Testzaz ^5 &&, Name-of-athe-tshirt-A123456759 Test after"; $pattern = '/[A-Za-z-\S]+(?=\-A\d)/'; if(preg_match($pattern, $tshirt, $matches)){ echo '<pre>'.print_r($matches, true).'</pre>'; } // match any characters in the string $tshirt = "Test, ... Testzaz ^5 &%, Name-of-*&%athe-Atshirt-A123456789 Test after"; $pattern = '/[.\S]*(?=\-A\d+)/'; if(preg_match($pattern, $tshirt, $matches)){ echo '<pre>'.print_r($matches, true).'</pre>'; } // match string which contains at least 9 digits in the end $tshirt = "Test, ... Testzaz ^5 &%, New-Code-Name-of-athe-tshirt-A123456889 Test after"; $pattern = '/[A-Za-z-\S]+(?=\-A\d{9})/'; if(preg_match($pattern, $tshirt, $matches)){ echo '<pre>'.print_r($matches, true).'</pre>'; }
-
Take a note: <?php if (!$mysqli->query("INSERT INTO test(id) VALUES (1), (2), (3), (4)")) { echo "Multi-INSERT failed: (" . $mysqli->errno . ") " . $mysqli->error; } ?>
-
Check out this guide -> http://php.net/manual/en/mysqli.quickstart.prepared-statements.php
-
Start from very beginning: <?php $mysqli = new mysqli("localhost", "user", "pass", "database"); /* check connection */ if (mysqli_connect_errno()) { printf("Connect failed: %s\n", mysqli_connect_error()); exit(); } // continue with prepare statement
-
mysql_num_rows(): supplied argument is not a valid MySQL result resource
jazzman1 replied to pes96's topic in MySQL Help
Go back to post #13. -
mysql_num_rows(): supplied argument is not a valid MySQL result resource
jazzman1 replied to pes96's topic in MySQL Help
I think, you need to start learning php from beginning - step by step . -
mysql_num_rows(): supplied argument is not a valid MySQL result resource
jazzman1 replied to pes96's topic in MySQL Help
My apologies, your sql syntax is correct :-\ First, I thought that the line 28 is just before the query I gave you above. Secondly, you can make a simple debugging test to check, what actually values you will send to database. <?php $username = 'jazzman'; $password = 'password'; $id = 1; $sql1 = "SELECT * FROM user_accounts WHERE username='".$username."' AND password='".$password."' AND user_id='".$id."'"; $sql2 = 'SELECT * FROM user_accounts WHERE username ='.$username.' AND password='.$password.' AND user_id='.$id; // integer id $sql3 = "SELECT * FROM user_accounts WHERE username='$username' AND password='$password' AND user_id='$id'"; $sql4 = 'SELECT * FROM user_accounts WHERE username=$username AND password=$password'; // invalid query printf('%s<br />%s<br />%s <br />%s', $sql1, $sql2, $sql3, $sql4); -
mysql_num_rows(): supplied argument is not a valid MySQL result resource
jazzman1 replied to pes96's topic in MySQL Help
You have to learn escaping single quotes in "php varibles". Take a look at the example: $str1 = 'string'; $str2 = '$str1'; printf('%s and %s', $str1, $str2); -
mysql_num_rows(): supplied argument is not a valid MySQL result resource
jazzman1 replied to pes96's topic in MySQL Help
Your query is wrong I think. Copy/paste this: $query = mysql_query("SELECT * FROM user_accounts WHERE username='".$username."' AND password='".$password."'"); -
Does this query come from any php framework or not ?
-
What do you mean ? Did you loop it ? For example: $newItems = array('computer','RAM', 'CD Rom'); $pattern = '/(?<=Replaced )(?=(\w+))/'; $str = 'Replaced disk, Replaced floppy, Replaced memory'; if(preg_match_all($pattern, $str,$matches)){ for($i=0;$i<count($newItems);$i++){ $matches[1][$i] = $newItems[$i]; } echo '<pre>'.print_r($matches[1], true).'</pre>'; }
-
What kind if result you got it : echo basename('quotes/'.htmlentities($row["Quote_ID"]).'-'.htmlentities($row["Client"]).'-'.htmlentities($row["QuoteRevision"]).'.pdf');
-
Where is deployed the project, on local machine or to server too ?
-
OR: if(trim(strip_tags($line['action'])) == "wow_displayed")
-
do you have a public link to this?
-
No, this is link to css nivo slider file, you need to include js script files to your header. Take a look at my project and check out the html source code -> http://kaneffbrookside.ca/stdimitar/
-
Did you link the query.nivo.slider.pack script file ? I can not see nothing of that
-
I've modified a little bit your code I think now is gonna be fine. Good luck index.php: // include jquery library <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js"></script> // include jquery confirm method <script src="jquery.confirm.js"></script> <script type="text/javascript"> $(document).ready(function(){ $('form.delete').submit(function(e){ e.preventDefault(); var elem = $(this).closest('.delete'); var lid = $(this).serialize(); $.confirm({ 'title' : 'Delete Confirmation', 'message' : 'You are about to delete this item. <br />It cannot be restored at a later time! Continue?', 'buttons' : { 'Yes' : { 'class' : 'blue', 'action': function(){ //elem.slideUp(); $.ajax({ url: 'deletelocation.php', type: 'POST', data: lid, success: function(response) { console.log('success', response); }, error: function() { console.log('error') } }); } }, 'No' : { 'class' : 'gray', 'action': function(){} // Nothing to do in this case. You can as well omit the action property. } } }); }); }) </script> <form name="delete" id="delete" class="delete"> <input type="hidden" name="lid" id="lid" value="<?php echo $theID ?>" /> <input type="submit" value="Delete Record"/> </form> deletelocation.php $lid = intval($_POST['lid']); $query = mysql_query("DELETE FROM table WHERE locationid='".$lid."'"); jquery.confirm.js (function($){ $.confirm = function(params){ if($('#confirmOverlay').length){ // A confirm is already shown on the page: return false; } var buttonHTML = ''; $.each(params.buttons,function(name,obj){ // Generating the markup for the buttons: buttonHTML += '<a href="#" class="button '+obj['class']+'">'+name+'<span></span></a>'; if(!obj.action){ obj.action = function(){}; } }); var markup = [ '<div id="confirmOverlay">', '<div id="confirmBox">', '<h1>',params.title,'</h1>', '<p>',params.message,'</p>', '<div id="confirmButtons">', buttonHTML, '</div></div></div>' ].join(''); $(markup).hide().appendTo('body').fadeIn(); var buttons = $('#confirmBox .button'), i = 0; $.each(params.buttons,function(name,obj){ buttons.eq(i++).click(function(){ // Calling the action attribute when a // click occurs, and hiding the confirm. obj.action(); $.confirm.hide(); return false; }); }); } $.confirm.hide = function(){ $('#confirmOverlay').fadeOut(function(){ $(this).remove(); }); } })(jQuery);
-
MySql connection collation on all database to be : utf8_general_ci and collation of the tables too. Did you fix the problem with "SET NAMES UTF8" ?
-
In future use only utf 8 character encoding in your database. Before send your php sql statement to database, add this piece of code: mysql_query("SET NAMES utf8", $connection);
-
If you want an event to work on your page, you should call it inside the $(document).ready() function: <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js"></script> <script type="text/javascript"> $(document).ready(function(){ $('form.delete').submit(function(e){ console.log('submit'); return false; }) }) </script> <form name="delete" id="delete" class="delete"> <input type="hidden" name="lid" id="lid" value="1" /> <input type="submit" value="Delete Record"/> </form>
-
This statement is wrong : $('.delete').submit(function(e) { }
-
Need help with first time mysqli access from PHP
jazzman1 replied to YigalB's topic in PHP Coding Help
@ jcbones, the console is very hard to understand for people comming from Microsoft's world @YigalB, open up your phpmyadmin application, go to "SQL" tab (on the top), and copy/paste this code: GRANT ALL PRIVILEGES ON `db_base` . * TO 'php24sql'@'localhost';