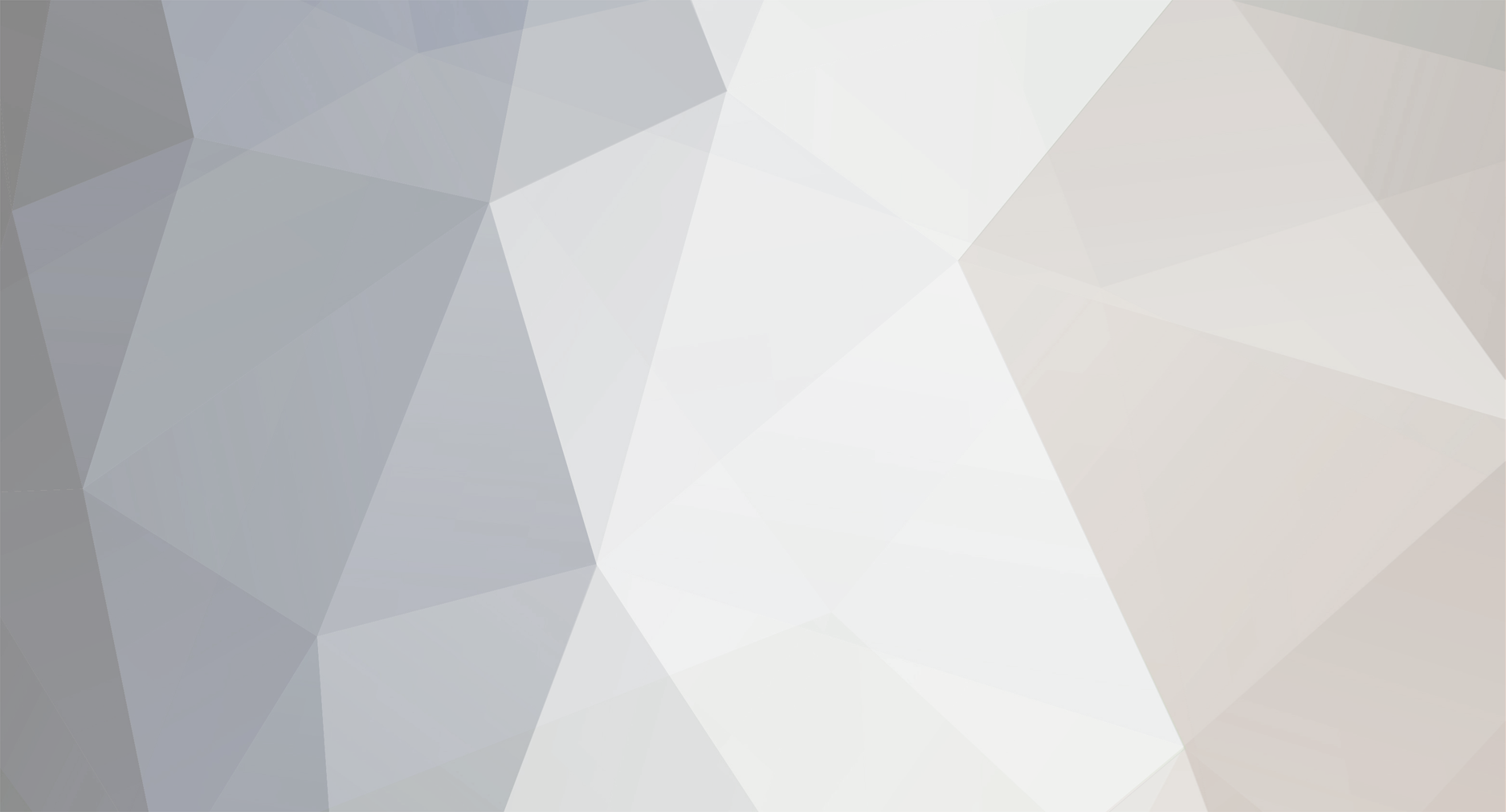
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
getting a query field to display as a hyperlink
scootstah replied to Plamph's topic in PHP Coding Help
Except you use periods not commas. -
Replace all the AND's with commas.
-
Some people find it more convenient to write if ($i == 1) doSomething(); than if ($i == 1) { doSomething(); } Both ways do the exact same thing so it's just a preference thing. You can also use the syntax Psycho posted which is usually favorable for templates when you are going in and out of PHP tags. For example, <?php if ($i == 1): ?> some html here <?php endif; ?> is a lot cleaner and more readable than <?php if ($i == 1) { ?> some html here <?php } ?> You can also use this syntax with foreach and while loops which is again more readable. You can use else and elseif while omitting brackets. if ($i == 1) doSomething(); else if($i == 2) doSomethingElse(); else dontDoAnything(); This works just fine.
-
Some Easy questions regarding PHPMYADMIN and PHP?
scootstah replied to radiations3's topic in Applications
I just want to point out that you don't need to have Xampp installed to use mysqldump. You just need the mysqldump client. Also, you can use the -P (port) and -h (host) flags to get a dump from a remote server. -
Oh, I see. You would need to put your checkboxes inside the foreach loop. Like.. foreach ($chkbxs as $value) { $check=""; if ($cmp[$value] == 1) { $check = "checked"; echo ''.$value.' <br/>'; } echo '<input type="checkbox" name="hardware[]" value="' . $value . '" '.$check.'>';
-
Well there is your problem.... And there's your other problem.
-
It should be: $check = 'checked="checked"';
-
You can't hurt a database with spaces. Furthermore, simply removing spaces is not going to prevent SQL injection. To do that, you need to use mysql_real_escape_string() which will escape harmful characters (like quotes) before they interact with the database.
-
That's not how you use salts. A salt needs to be a randomly generated string with as much entropy as possible. And it needs to be unique to each user. If your salt is the same for every user then it is the same as not having a salt to begin with. A salt has only one purpose: to make the hash of users with like passwords different. If you MD5 "qwerty" the hash would be "d8578edf8458ce06fbc5bb76a58c5ca4". So if someone got a dump of your users table, and they see two or more people with the hash "d8578edf8458ce06fbc5bb76a58c5ca4", all they have to do is crack it once and they have multiple logins. If you were to use a random salt then each hash would be different, even if the password is the same. So if the salt was "kfdgjk34639" then the hash is now "8d72631f8a2230116b735c286d8a59ba". If the salt is unique to each user, no two users will ever have the same hash. Additionally, since the salt will be unique to each user you need to store the salt in the database when they create an account. Since the hash will be computed based on the salt, the salt is required to re-hash the password and get a match. So when a user tries to login you have to first retrieve the salt from the database and then hash the password with it. Aside from that, another big no-no here is that you are vulnerable to SQL injection. You need to use mysql_real_escape_string() to prevent that. Here is a quick concept factoring in my above points: register.php if (!empty($_POST)) { $username = mysql_real_escape_string($_POST['username']); $password = mysql_real_escape_string($_POST['password']); if (!empty($username) && !empty($password)) { // create salt // the salt will be a random 10 character alpha-numeric string $salt = substr(sha1(uniqid(mt_rand(), true)), 0, 10); // hash the password $hash = md5($password . $salt); $query = mysql_query("INSERT INTO users (username, password, salt) VALUES ('" . $username . "', '" . $hash . "', '" . $salt . "'"); } } else { echo '<form action="" method="post"> Username: <input type="text" name="username" /><br /> Password: <input type="password" name="password" /><br /> <input type="submit" name="submit" value="Register" /> </form>'; } login.php if (!empty($_POST)) { $username = mysql_real_escape_string($_POST['username']); $password = mysql_real_escape_string($_POST['password']); if (!empty($username) && !empty($password)) { $query = mysql_query("SELECT username, password, salt FROM users WHERE username='" . $username . "'"); if (mysql_num_rows($query) == 1) { $row = mysql_fetch_assoc($query); // re-hash the password $hash = md5($password . $row['salt']); // match them if ($hash == $row['password']) { // matched, so the user is logged in } } } } else { echo '<form action="" method="post"> Username: <input type="text" name="username" /><br /> Password: <input type="password" name="password" /><br /> <input type="submit" name="submit" value="Register" /> </form>'; }
-
In the future, when queries appear to do nothing, use mysql_error() to print any query errors out.
-
So add the WHERE clause that I posted to your UPDATE query.
-
What is line 177 in login.php?
-
Remove the comma between the last set and the where clause. ExpiryDate='".mysql_real_escape_string($_POST['ExpiryDate'])."', where BuyerCareerID=".$_POST['BuyerCareerID']
-
the simplest thing in the world fails / LOL
scootstah replied to cybernet's topic in PHP Coding Help
The error is not because of $id, it is because of $_GET['id']. The error says that $_GET['id'] is undefined. Try commenting out the include and see if it works then. -
This should work... In an SQL query: ... WHERE date <= DATE_SUB(NOW(), INTERVAL 10 MINUTE)
-
They can be named anything you want. They are simply methods in a class. Yes and no. With the code you provided they won't do anything without being called manually. However, you could call them from magic methods (like __get and __set) if you wanted to.
-
the simplest thing in the world fails / LOL
scootstah replied to cybernet's topic in PHP Coding Help
Unless you are unsetting $_GET variables in main.php, the problem is most likely nginx. -
There shouldn't be any problem passing query strings to a header. What is the code for CurPageUrl()?
-
So you think function( $parm1, $parm2, round(($upper+$lower)/2+0.5) ); is more readable than function($parm1, $parm2, round(($upper+$lower)/2+0.5)); It takes extra brain power to manually process that there is a space there and that should be the end of the statement. But when I see a big red bracket, I instantly know it has to be the end. Furthermore, If I have a big complex if statement that has several bracketed conditions in it, I can easily use the arrow keys to go through the brackets and instantly know what goes to what. Try doing that by just looking at it. even with spaces it gets confusing.
-
the simplest thing in the world fails / LOL
scootstah replied to cybernet's topic in PHP Coding Help
Automatically converted to what? -
I don't know, other than it's a very ass backwards way of getting database results.
-
They don't have numerical indexes so [1] isn't going to work. If you do a print_r($keyws) you should see all the indexes you can use.
-
Try this while($latest = mysql_fetch_assoc($news)) { $news_date = date("Y-m-d",$latest['date']); echo"<div class='rightLinks'>".$news_date."</div>"; echo $latest['article_title']."</h2><p>".$latest['article_text']; }
-
What is the PHP error?