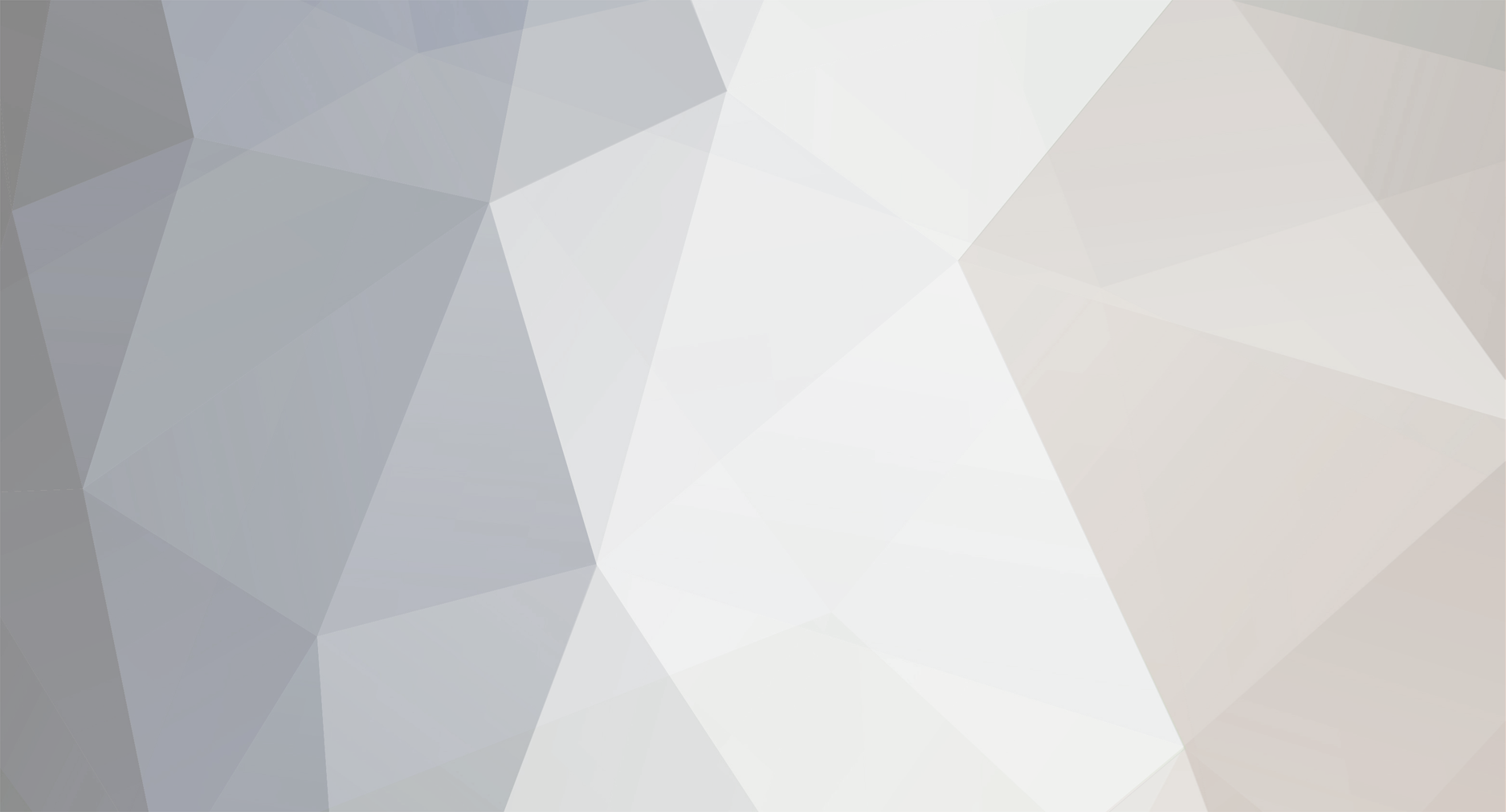
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Like this.
-
In the middleish I put a comment, // do database stuff here. That is basically the place where the upload has succeeded.
-
Yes but you could have other errors, and you're not checking those. I've restructured your code to have a logical flow. Hopefully this makes sense to you: <?php // this should be in its own file, and then include() it $mysqli = new mysqli(DB_HOST, DB_USER, DB_PASS, DB_NAME); # check connection if ($mysqli->connect_errno) { echo "<p>MySQL error no {$mysqli->connect_errno} : {$mysqli->connect_error}</p>"; exit(); } // check if a form was submitted if (!empty($_POST)) { // check if there are any upload errors if ($_FILES['cvfile']['error'] === UPLOAD_ERR_OK) { // make sure the file is not too large if ($_FILES["cvfile"]["size"] <= 500000) { $target = "/home/sites/broadwaymediadesigns.co.uk/public_html/sites/recruitment-site/candidatecvs/"; $target = $target . basename($_FILES['cvfile']['name']); // make sure the file doesn't already exist if (!file_exists($target)) { $allowedMimes = array('application/pdf', 'application/msword'); $finfo = new finfo(FILEINFO_MIME); $mimetype = $finfo->file($_FILES['cvfile']['tmp_name']); // make sure we have an allowed MIME type if (in_array($mimetype, $allowedMimes)) { // make sure the file was moved to the destination if (move_uploaded_file($_FILES['cvfile']['tmp_name'], $target) !== false) { // do database stuff here // finally, show success message echo "<p class='success'>Form has been submitted successfully.</p>"; } else { // file could not be moved to destination echo "Sorry, there was an error uploading your file."; } } else { // disallowed MIME type echo "Sorry, only PDF, DOC & DOCX files are allowed."; } } else { // file already exists echo "Sorry, file already exists."; } } else { // file is too large echo "Sorry, your file is too large."; } } else { // upload error echo "Sorry, there was an error uploading your file."; } }
-
You didn't send a password through the form, so you cannot access it with $_POST['password']. I'm guessing you want this instead: $user['password']. With that said, that means you are storing your password in plaintext in the database, and then emailing it to someone in plaintext. Both of those ideas are very bad. Don't do that. Passwords needed to be irreversibly hashed before they are stored in a database. PHP5.5 has built in functions for hashing a password. Also, you don't want to be emailing sensitive things like passwords. A traditional forgot password system works by creating a temporary token, and emailing it to the given email. The user would then click a link containing the token (something like http://example.com/reset_password.php?token=abc123), which would then prompt them to create a new password.
-
If PHPMailer is not returning errors then it leads me to believe it has something to do with the SMTP service. Are you sending from the correct domain for the SMTP? Are you sure the encryption settings are correct? Without being able to see some logs it would be difficult to troubleshoot. Perhaps you can contact your host and they can look for you?
-
That's where you are setting it, but you are not using it. You need to give the parameters to the execute() method. $result = $stmt->execute($query_params);
-
Okay, so according to PHPMailer everything has worked. Is there any sort of logging on your SMTP service, which might indicate why it was not delivered?
-
Good. Now then... are you getting any mail errors? Specifically, what is $mail->send() returning here? if(!$mail->send()) { echo 'Message could not be sent.'; echo 'Mailer Error: ' . $mail->ErrorInfo; } else { echo 'Message has been sent'; }
-
First of all, remove your login credentials from your post and then go change them on your server.
-
You're sending "Form has been submitted successfully." at the top before you even do any processing. And you're still only checking the file extension. Did you read my post above? I showed you how to check the mime type. Also, you're still not exiting the script when there are form errors, so your query is still going to happen at the bottom.
-
You didn't tell your form to use POST. <form method="POST" name="sentMessage" id="contactForm" novalidate>Unless your form HTML is on the same script (Contact_Me.PHP), you also need to put an action. <form method="POST" action="Contact_Me.php" name="sentMessage" id="contactForm" novalidate>Also, saying return false; in the middle of a script does nothing. Everything underneath that is still going to execute.
-
You're still only checking the file extension (so file name). You need to check the MIME type, not the file extension. And why are you checking for an image? $check = getimagesize($_FILES["cvfile"]["tmp_name"]); I thought you wanted to only upload pdf, doc, and docx? You're still inserting into the database because you never exit the script after your $uploadOk is 0 (and please, don't use 1 and 0, use true and false).
-
You don't even need arrays, you just need to do some action * number of tickets. That is what a for loop is used for. $numAdults = $_POST['adults']; $numKids = $_POST['kids']; if ($numAdults != 0) { for ($i = 0; $i < $numAdults; $i++) { // create an adult ticket } } if ($numKids != 0) { for ($i = 0; $i < $numKids; $i++) { // create a kid ticket } }Rather than running an INSERT query on each loop iteration, though, I'd recommend that you compile a list of values to insert and do it all at once with a batch insert. Manual here.
-
Eh... I have to respectfully disagree.
-
The value in the middle of what? What is the desired output from your example input?
-
Couple things: 1. Do not use $_SERVER['PHP_SELF'] as the form action, because it can be manipulated and is not safe. If you want to POST to the same page then just leave the action attribute out completely. <form method="post">2. You need to escape user input when you put it in a query, otherwise you are vulnerable to SQL injection. The easiest way is this, although I'd recommend you learn how to do prepared statements since you're already using MySQLI.$username = $mysqli->real_escape_string($_POST['username']); $password = $mysqli->real_escape_string($_POST['password']);3. Do not store passwords in plaintext in the database. Bad. You need to hash them with a secure hashing algorithm. PHP>=5.5 has this built in, with password_hash() and password_verify(). 4. You don't use LIKE for this purpose. Your WHERE clause should look like: WHERE pemail = '{$username}' AND password = '{$password}'Other than that, I don't see anything that would make your email not work. Logging in with email as the username is exactly the same as logging in with a username as a username.
-
Based on your other thread, latitude and longitude are not arrays. So you just need: position: new google.maps.LatLng(coords[i].latitude, coords[i].longitude),
-
If it works until you run swapContent(), then the problem is with whatever swapContent() is doing. You are boogering the HTML up that accordion wants, or something like this. Or, your script is encountering an error. Again, are you seeing errors in the developer console?
-
You want an "onKeyUp" event on the input field, which will get whatever you typed in and place it in the table using .innerHTML.
-
You can use strtotime() to do math on a date. Or, you can also use DateTime to do the same.
-
So are you running everything on one server? Are you using file_get_contents() to call a script that exists on the same server over HTTP? Sockets could work too, and is probably faster.
-
The function you posted isn't really doing anything with the database, that seems to happen inside $__db->query(), so we'll need to see that.
-
Please post the relevant portions of code here in code tags.
-
Well, that's going to be your bottleneck right there. The way you're doing it, you have to wait for the HTTP response before your script finishes. That could be anywhere from 100ms to several seconds. What you want to be doing is asynchronous requests, so that your script can end and start again before the HTTP call is finished. This should speed up your script considerably. There's many ways to do it, and many ways to scale it. You could start off with your one server, but if that is taking too long you can look into something like a Gearman cluster.
-
The mimetype cannot be manipulated. Trusting anything from $_FILES is pointless because it can just be forged - it is just client input. The browser can lie. The mimetype cannot lie, it is the very signature of the file. The best way to store files is to have a properly set up server. Even in the event that a script gets uploaded, it should not have any privileges to do anything.