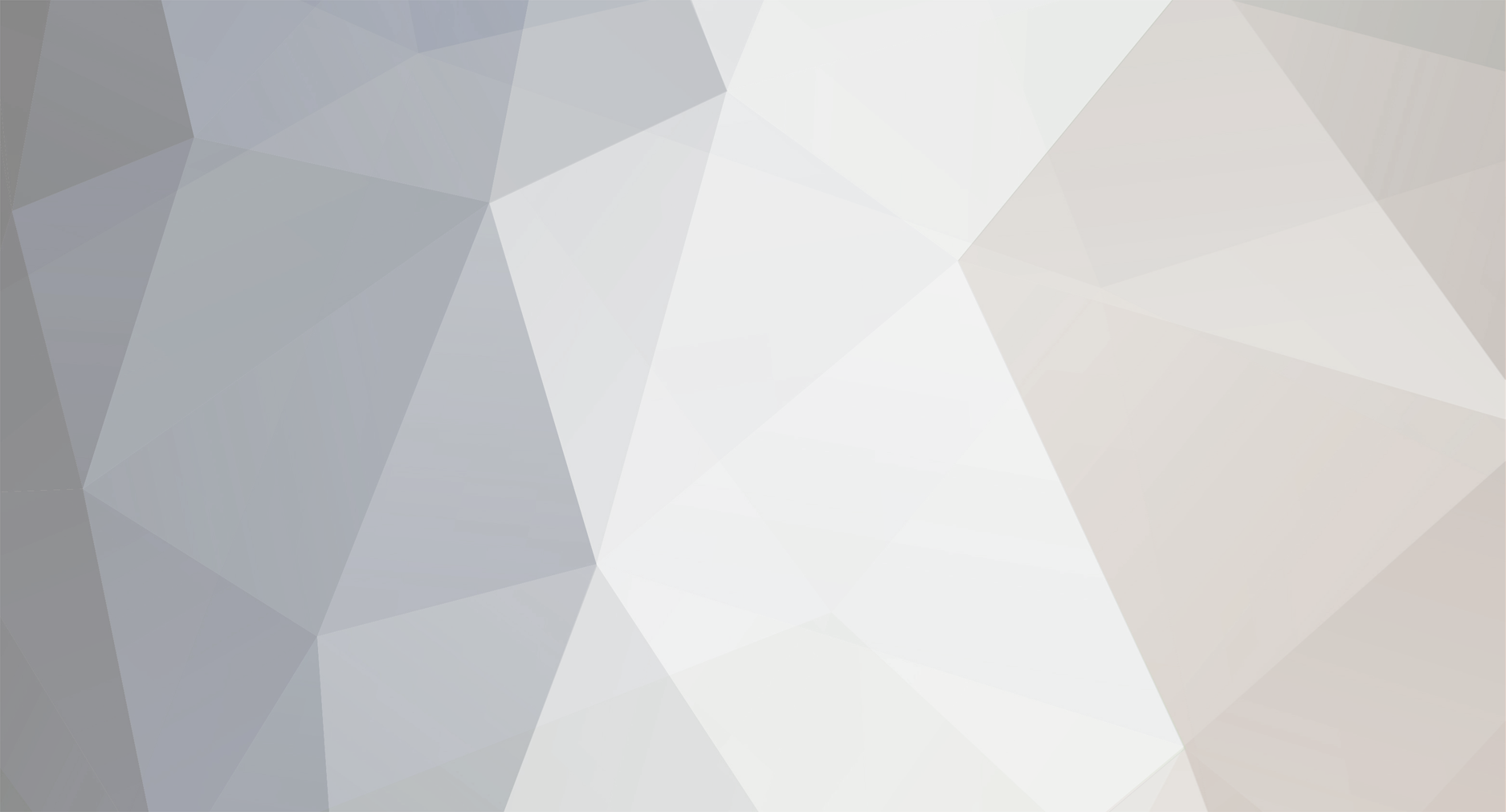
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Yes. But you can convert a JSON object to a PHP variable with json_decode().
-
json_encode and json_decode
-
The problem is that you are not outputting anything in the loop, you are just setting a variable to output later. But, that variable overwrites itself on every iteration, so the value will only be equal to the last row. Here's an example, in case that didn't make any sense. If this is your data set: ID | Year | Make | Model 5 | 2004 | Ford | Explorer 5 | 2002 | Dodge | Durango 5 | 2005 | Chrysler | Town & Country And this is your loop: $sql = mysql_query("SELECT * FROM car_data WHERE id='$id'"); while($row = mysql_fetch_array($sql)){ $year = $row["year"]; $make = $row["make"]; $model = $row["model"]; } This is how it will work out: Iteration 1 $year = 2004 $make = Ford $model = Explorer Iteration 2 $year = 2002 $make = Dodge $model = Durango Iteration 3 $year = 2005 $make = Chrysler $model = Town & Country So, as you can see, on each iteration you are over writing the previously-set variable with the current row's data. So in the end, you only have one row's worth of data. You can do a couple of things here: 1. Output directly from the while loop. This doesn't seem like a good idea in this case, since you have all your markup afterwards 2. Make a new array and push to it on each iteration, and then loop through it later. This doesn't sound like a good idea either, since you aren't doing anything else in the while loop. 3. Move the while loop to where it needs to be output. 4. Construct an output string inside the while loop, to be output later. I would go with either #3 or #4.
-
This topic has been moved to PHP Regex. http://forums.phpfreaks.com/index.php?topic=363666.0
-
That's an argument for selecting only the fields that he needs, which I agree that he should be doing instead of using "*". Though, that's not only for performance reasons, but also for readability. Well, you said that the difference would only be ~500bytes. I'm saying that you cannot possibly know that, because you don't know what data the table holds. If he is selecting all of the rows, and then you double that, that could be a pretty big difference. I agree that micro-optimization is not really a big concern. However, this is not micro-optimization, it has (can have) a pretty significant impact on memory usage. And this has absolutely nothing to do with readability. This is how the three mysql_fetch functions (fetch_assoc, fetch_array, fetch_row) work: data set: id | title | content 1 | some title | some content mysql_fetch_assoc(): array( 'id' => 1, 'title' => 'some title', 'content' => 'some content' ) mysql_fetch_row(): array( 0 => 1, 1 => 'some title', 2 => 'some content' ) mysql_fetch_array(): array( 0 => 1, 'id' => 1, 1 => 'some title', 'title' => 'some title', 2 => 'some content', 'content' => 'some content' ) So, as you can see, mysql_fetch_array() is simply combining mysql_fetch_row() and mysql_fetch_assoc(). Why would you ever want to do that, especially when you are only using string keys? In some cases, I could see the need for numerical indexes. Say, something like this: $result = mysql_query("SELECT COUNT(*) FROM table"); list($num) = mysql_fetch_row($result); In which case, you would simply use mysql_fetch_row(). But, I don't know why you would ever need both forms of data at the same time. It is inefficient, and should be avoided.
-
By looping through the results. I can't really help more than that without seeing some code.
-
Oops, I overlooked that before. It looks like you tried to copy the ternary statement that I used, but didn't copy the whole thing. So change to: $module = isset($_GET['module']) ? $_GET['module'] : ''; You can put a default module instead of an empty string if you want, but if you use an empty string you can just run the default: block in the switch statement.
-
What do you get when you echo $module, just above the switch statement?
-
Which part are you stuck on exactly? Here is a good way to iterate through $_FILES when you have multiple uploads: for($i = 0; $i < count($_FILES['file']['name']); $i++) { echo "Name: {$_FILES['file']['name'][$i]}<br /> Type: {$_FILES['file']['type'][$i]}<br /> Temp name: {$_FILES['file']['tmp_name'][$i]}<br /> Error: {$_FILES['file']['error'][$i]}<br /> Size: {$_FILES['file']['size'][$i]}<br />"; } This way, you can access all of the properties on each iteration without having to have loops for each one, if that makes sense.
-
1. You have improper array and string syntax. 2. You can't put quotes around MySQL field names. They require either back ticks (`), or nothing at all (unless they have a space in the name) 3. post_id does not need quotes around the value, because it is an integer 4. You are not calling mysql_query(). 5. You are not sanitizing the input data, which could lead to SQL injection $title = mysql_real_escape_string($_POST['title']); $content = mysql_real_escape_stirng($_POST['content']); $sql = "UPDATE posts SET title='$title', content='$content' WHERE post_id = $post_id"; mysql_query($sql);
-
He is selecting all columns from the table, though. Who knows how much data that is. Also, a varchar can hold 65,535 bytes, which is the maximum row size. And just because this is a case where the difference might be negligible, it is still bad practice. What is the point to holding the extra data if you're not even going to use it?
-
How do you figure that? You are fetching the same data twice. array( 0 => 'some title', 'title' => 'some title', 1 => 'some content', 'content' => 'some content' )
-
You could use a CSRF token in the query string, and that would be OK, but you should still stick to POST for that stuff. I sometimes run a bit of AJAX on the link to avoid the extra button click.
-
This topic has been moved to PHP Regex. http://forums.phpfreaks.com/index.php?topic=363625.0
-
The switch statement isn't the issue. What you should be doing to stay secure is validating the input. In the example I just gave you, I didn't validate input, and so this is a huge security risk: if (file_exists($file = 'apps/' . $app . '.php')) { include $file; } The reason it is a security risk is because an attacker can traverse the file system in any way that he wants and include any file. So, files that are outside of the docroot that should be inaccessible, are, in fact accessible. So, to combat this, take advantage of the code I posted in my first reply. In this code, I am checking that the input matches an array of valid modules. So, only the array of valid modules will ever get loaded, and no LFI attacks will work. If the input is not one of the valid modules, it will terminate the script and output "Page Not Found". // if there is no module, use "news" as default $module = isset($_GET['module']) ? $_GET['module'] : 'news'; // define an array of acceptable modules $valid_modules = array( 'news' => 'news.php', 'members' => 'members.php', 'forums' => 'forums.php', 'contact' => 'contact.php', 'about' => 'about.php' ); // make sure the module is in the list // and that the file exists if (!in_array($module, $valid_modules) || !file_exists($valid_modules[$module])) { // send 404 header header('HTTP/1.1 404 Not Found'); die('<h1>404 - Page Not Found</h1>'); }
-
I'm running a Intel i5 2500k, and with all 4 cores at 100% load I'm at ~55*C (give or take a degree on each core), using a CoolerMaster Hyper212 cooler. Not great, but not too bad either considering how damn hot the ambient temperature is right now. @tendouser, download Prime95 (64bit 32 bit) to stress test your CPU, which will put you at 100% load. That way, you can get an accurate load temperature. Also, download CoreTemp, because it has more accurate readings. Once you have Prime95 downloaded, follow these steps: 1. Start up "prime95.exe" 2. Click "Just stress testing" on the dialogue box 3. When the box titled "Run A Torture Test" pops up (if it doesn't pop-up, go to Options > Torture Test) click the middle option, "In-place large FFTs" and then press OK (also, make sure the "Number of torture test threads to run" is equal to the number of cores in your CPU). 4. At this point, all cores of your CPU will be at 100% load. After 10 seconds or so, check the temperature. 5. To stop the test, go to Test > Stop, and then exit Prime95.
-
1. Do not use PHP_SELF, because it makes your page vulnerable to XSS attacks. Instead, use an absolute URL for the action attribute, leave it blank, or omit it from the form tag. 2. What are you doing when the form is submitted? Currently all that would happen is a bunch of undefined variable ($row) errors. 3. Don't use mysql_fetch_array() when you're not using numerical indexes, it is a waste of memory. Use mysql_fetch_assoc() instead.
-
No, you would use an UPDATE for that, with an empty value. UPDATE posts SET post_title='' WHERE post_id=$post_id With that aside, you are doing something very wrong. <a href=\"delete_post.php?post_id=" . $row['post_id'] . "\">Delete</a> <-- DO NOT DO THIS! GET should only be used for GETting data, not manipulating it in any way. Use a POST request to delete things, so that you can properly secure it from CSRF attacks.
-
70*C on a desktop even under full load is still pretty damn hot. I definitely wouldn't want to run my machine at that temperature for prolonged periods of time. However, he is using a laptop, which is a different story. 70*C at full load on a laptop is perfectly acceptable. If that is 70*C at idle, though, you might have some problems.
-
It is important to understand that the URL does not have to directly map to a directory structure. You don't need an entire directory for every URI segment. I think your terminology is a little weird (the way you are using "app" and "modules"), but I think I know what you mean. I assume that "app" refers to a page, and "module" refers to sections within that page. If that is the case, here is a basic example. For simplicity's sake, the routing part of this example is going to be much less code, and does not take any security into consideration, as it is not the focus of my example. However, feel free to tie it into the examples that ChristianF and I wrote above. directory structure: / apps/ member.php index.php index.php <?php // get the app from the query string $app = $_GET['app']; // include the app file // NEVER DO THIS IN PRODUCTION // IT IS AN EXAMPLE ONLY // AND IS OPEN TO SECURITY VULNERABILITIES if (file_exists($file = 'apps/' . $app . '.php')) { include $file; } apps/member.php <?php $module = $_GET['module']; switch($module) { case "login": // login form here break; case "process": // process the login break; case "profile"; // member profile here break; default: // if no module was given break; } URLs: http://example.com/index.php?app=member http://example.com/index.php?app=member&module=login http://example.com/index.php?app=member&module=process http://example.com/index.php?app=member&module=profile This is a very basic way to do what I think you are trying to do. There are more complicated ways to do it, which will better organize things and make things less redundant. But, this illustrates the basic principle. Again, this code is by no means secure. You can use .htaccess to disable access to a directory. Order Deny,Allow Deny from all What are the contents of your .htaccess, and what is the error message? Check Apache's error.log file to find the error.
-
Yes. If you don't have a persistent socket, it will just be closed when the script terminates. So, AJAX or not, you will still be re-opening the connection on every request.
-
There's two things to think about here: dynamically loading a page, and dynamically adding content to a page. Dynamically loading a page is pretty easy. At a basic level, this is all you need: <?php // if there is no module, use "news" as default $module = isset($_GET['module']) ? $_GET['module'] : 'news'; // define an array of acceptable modules $valid_modules = array( 'news' => 'news.php', 'members' => 'members.php', 'forums' => 'forums.php', 'contact' => 'contact.php', 'about' => 'about.php' ); // make sure the module is in the list // and that the file exists if (!in_array($module, $valid_modules) || !file_exists($valid_modules[$module])) { // send 404 header header('HTTP/1.1 404 Not Found'); die('<h1>404 - Page Not Found</h1>'); } // get the page content ob_start(); include $valid_modules[$module]; $content = ob_get_clean(); // output the page echo '<!DOCTYPE html> <html> <head></head> <body> <div id="header">My logo</div> <div id="content">' . $content . '</div> <div id="footer">Stuff that goes in a footer</div> </body> </html>'; So basically, your website would funnel through index.php. If no module is defined from GET, then "news" is used as a default. If a module is defined, the script will make sure that the module exists in the "valid" list (to prevent a local/remote file inclusion attack). If the module does not exist in the valid list, or the module file does not exist, a "404 Not Found" header is sent and the script is terminated. If this check is passed then the module file is included, loaded into a buffer and then into the $content variable, which is later added to the page markup. Then, all you have to do is create the module files (like "news.php", "members.php" and so forth) and add some content.
-
Worst case scenario is it's NOT the motherboard, and you just get a refund. I don't know where you live, but I'm from USA and always use http://newegg.com for stuff. I'm pretty certain you can return for a refund within a small window of time.
-
Have you tried resetting the BIOS? To do that... 1. Unplug the powersupply, or switch it off 2. Move the jumper over 1 pin and wait 10-15 seconds, then move it back to the original position (or) Remove the CMOS battery and wait 10-15 seconds, then replace it This image shows both the CMOS battery and the jumper: Also, try removing all non-essential devices connected to the PC. This means all USB devices (except for a keyboard), the floppy drive (if you have one), any optical drives, any hard drives, sound cards, ethernet cards, etc. Remove anything that isn't required to boot the PC and get a visual display. I would recommend using the onboard video out for trouble shooting, just because it removes a variable. If none of the above yields any results, unfortunately you'll have to find some spare parts to test things. It is unlikely to be the RAM, because usually bad RAM will at least power on, though not always. It is even more unlikely that all sticks of RAM are bad, and unable to boot from any slots. So, therefore it is most likely either a faulty CPU or a faulty motherboard, and I'm going to lean towards the motherboard. Usually if the CPU is bad, it will power off again. One of the best things you can do is go buy a motherboard speaker. They're very cheap, and should be available at Best Buy or Radio shack or something like that. It is important to know if there are any beep codes.