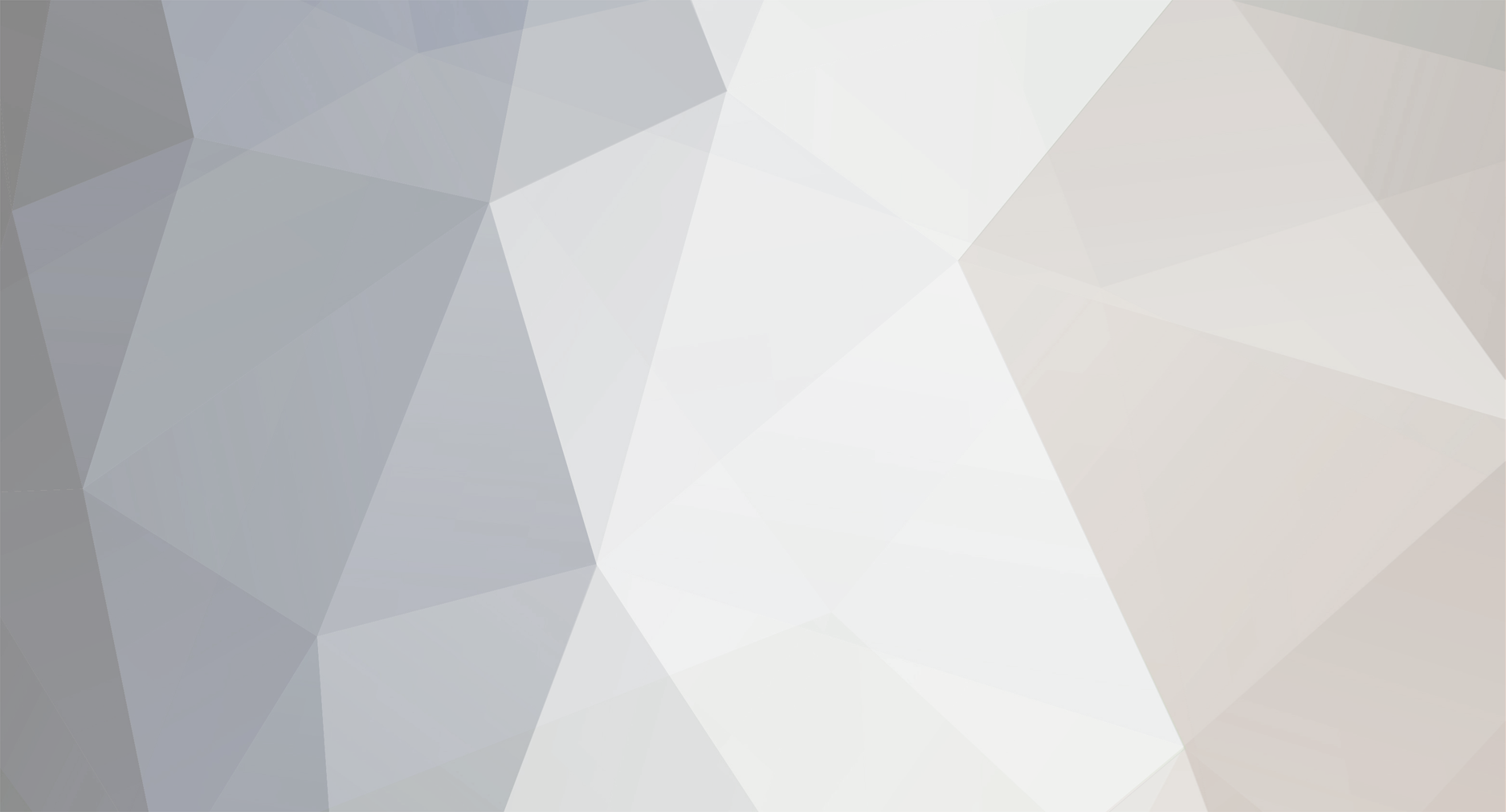
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Sidebar on site (Controlled by PHP sessions?
scootstah replied to KingBeau's topic in PHP Coding Help
First of all, session_is_registered() is deprecated as of PHP5.3 and removed as of PHP5.4. You should be using the $_SESSION superglobal now. So, it should be: if (isset($_SESSION['username'])). On to your problem. Ideally, you should have some reusable code to verify an active login - maybe a class method, or just a simple function. You could then use that to figure out if you should display the sidebar or not. if (is_admin()) { require 'sidebar.php'; } -
You use MySQL's functions. $query = "select id from table1 where some_date = NOW()"
-
http://us.php.net/manual/en/language.types.array.php
-
You are never retrieving the pagenum from the querystring. if(!isset($pagenum)) { $pagenum = 1; } I'm assuming this snippet is relying on register_globals to be enabled. It should be changed to: if(isset($_GET['pagenum'])) { $pagenum = (int) $_GET['pagenum']; } else { $pagenum = 1; }
-
You can use the index, or loop through it.
-
What about, $features[$x][1], $features[$x][2], $features[$x][3]
-
Is it ok to put an insert statement inside a foreach?
scootstah replied to bugzy's topic in PHP Coding Help
Yeah, that should work. Although if you find a webserver running a version of PHP that doesn't have mysql_real_escape_string(), something is very, very wrong. -
You would need variable variables here. Something along the lines of: if ($i > 00 && $i <= $ii){$var_name = "$features_{$x}_1"; $$var_name .= "<input type='checkbox' name='features($r[id])' value='1' />$r[name] </br>";} But, that looks hideous. Can't you just use arrays? $features[$x] = "<input type='checkbox' name='features($r[id])' value='1' />$r[name] </br>";
-
Is it ok to put an insert statement inside a foreach?
scootstah replied to bugzy's topic in PHP Coding Help
No, and that's not how you use is_numeric(). is_numeric() returns true if the input is a number, or false if it is not. Note that any number will return true, even if it is a decimal. If you only want integers, you should be using is_int(). On the other hand, (int) is for type casting. It is used when you want to explicitly convert one data type to another. In this case, you would end up with an integer no matter what the data type started out as. Does your function actually use mysql_real_escape_string()? If not, it probably isn't secure. -
Is it ok to put an insert statement inside a foreach?
scootstah replied to bugzy's topic in PHP Coding Help
No, that really isn't okay. What also is not okay is that you are using untrusted user input directly in the query. I don't believe there are any security problems with mysql_insert_id(). First and foremost you need to be escaping user input with mysql_real_escape_string to prevent SQL injection. If the column type is an integer, you can safely typecast the data to integer - removing any non-int characters. Where do these variables come from: '{$item_code}','{$item_description}','{$item_price}','{$item_stock}', '{$item_sale}'? Are they escaped? Anyways, MySQL supports multiple INSERT values in the query, so that is what you should be using. This way you have one query, but many inserted rows. Something like this ought to work: $query = "Insert INTO item(item_code, item_description, item_price, item_stock, item_sale, item_reg) VALUES('{$item_code}','{$item_description}','{$item_price}','{$item_stock}', '{$item_sale}', NOW())"; mysql_query($query); $last_id = mysql_insert_id(); $insert_data = array(); foreach($_POST['item_cat'] as $item_cat) { $item_cat = (int) $item_cat; $insert_data[] = "($item_cat, $last_id)"; } $query = "INSERT INTO item_category (category_id, item_id) VALUES " . implode(',', $insert_data); mysql_query($query); Basically, this just loops through the $_POST array and adds the set of values to an array. Later, the array is imploded to create the SQL syntax. Which, in case you don't know, the multiple insert value syntax looks like this: INSERT INTO item_category (category_id, item_id) VALUES (1, 1), (2, 1), (3, 1) ... -
Can you explain the question more clearly please?
-
So what's wrong with Googling it? This took about 30 seconds: DROP TABLE IF EXISTS ENGINE=MyISAM AUTO_INCREMENT LOCK & UNLOCK
-
I don't know what you are asking. The query above creates a table and then inserts data to it.
-
ok, what do you need the book for? seems more of a pita to get a book, when the info is at your googletips. Some people trust a book more than the ramblings of random blogs.
-
Can you echo the problem query out and post it here? echo $q4; (or whatever query is the problem).
-
I think by "html encodes functions" you are talking about htmlentities or htmlspecialchars, neither of which will prevent SQL injection. To prevent SQL injection, you need to either: escape the data, or use prepared statements. There are a lot of aspects to secure code. You can go a long ways by following a few best practices (like what I mentioned above regarding SQL injection, always sanitizing user input, etc) and by leaving things that are controversial to people that are qualified to make the hard decisions (like user authentication, password storage, encryption, XSS filtering).
-
Which query isn't working? This one? $q4 = "INSERT INTO institutes ( login_id, address_id, contact_id, institute_code, institute_name, institute_slogan, introduction_note, institute_history, institute_present, active, registration_date ) VALUES ( '$loginId', '$addressId', '$contactId', '$instituteCode', '$insName', '$Slogan', '$instroduction', '$history', '$present', '$active', NOW() )"; Have you checked if there is a MySQL error? $r4 = mysqli_query ($dbc, $q4) or die ('MySQL error!<br />Query: ' . $q4 . '<br />Error: ' . mysqli_error($dbc));
-
Redirect to static web page from user input box
scootstah replied to Pointmewheretogo's topic in PHP Coding Help
This is just a simple matter of capturing the input and then using it in a string (for link) or header call (for redirect). if (!empty($_POST)) { $page = $_POST['page']; // link echo '<a href="http://example.com/pages/' . $page . '.html">' . $page . '</a>'; // redirect header('location: http://example.com/pages/' . $page . '.html'); exit; } else { echo '<form method="post"> Page: <input type="text" name="page" /><button type="submit">Go</button> </form>'; } This will get you started. You may want to validate and/or sanitize the input. -
Check out these two articles: one two for examples on how to store hierarchical data.
-
Surely you're not typing out thousands of categories by hand, are you? I would hope you are storing them in a database, and looping through them.
-
Preserving values in input field after page gets refreshed
scootstah replied to eldan88's topic in PHP Coding Help
Eh, I don't find it to be a big deal. I've trained my hands to go for F5 and ctrl+F5 (reload the cache, thus clearing the form fields) instead of the refresh icon. -
Preserving values in input field after page gets refreshed
scootstah replied to eldan88's topic in PHP Coding Help
I thought browsers did this already? At least, Firefox 13 does. Your example code would work if they submit the form (and if you assign $name to the POST variable), but not on a page refresh. I'm not sure if there is an elegant solution for doing it on page refresh if the browser doesn't do it for you. The only thing that springs to mind is using Javascript to put the form data into a cookie or a PHP session - but neither of those sound like good ideas. -
Well, I don't know what $begin and $finish hold, so I'm only guessing here.
-
Post code please.