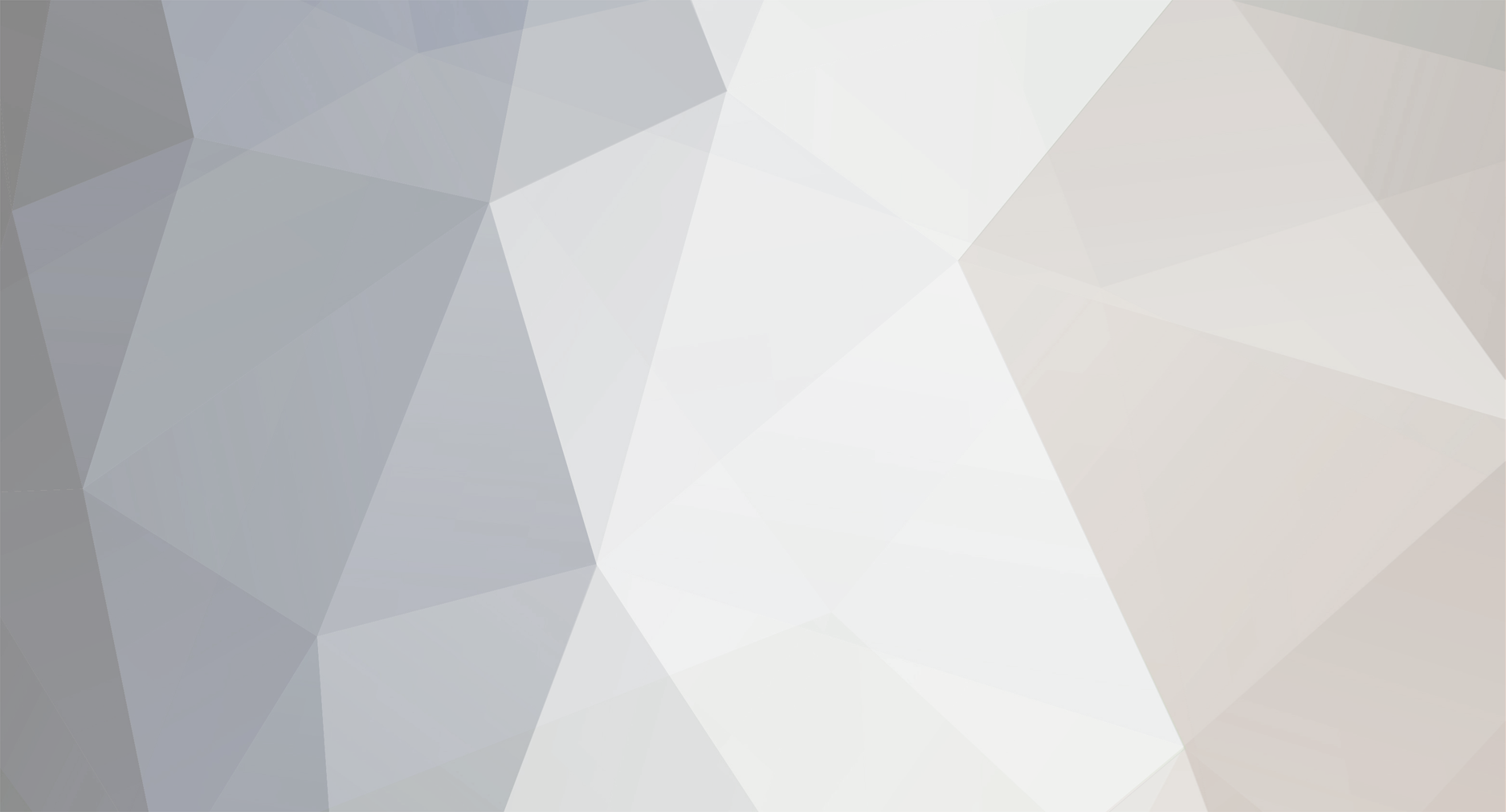
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Well for starters, it will always say "submitted successfully", because there is no else statement. But, your problem is that your submit button is of type button when it should be of type submit.
-
Create a Database connection class and delete function
scootstah replied to iseriouslyneedhelp's topic in PHP Coding Help
Once again, that class is doing nothing. You need to be returning the mysqli object, and you are not even calling the openConnection() method. At the very least, the class should look something like this: <?php class DbConn { private $dbhost; private $dbusername; private $dbpword; private $dbname; private $conn; public function __construct($dbhost, $dbuname, $dbpword, $dbname) { $this->dbhost = $dbhost; $this->dbusername = $dbuname; $this->dbpword = $dbpword; $this->dbname = $dbname; } public function openConnection() { $this->conn = @new mysqli($this->dbhost, $this->dbusername, $this->dbpword, $this->dbname); if ($this->conn->connect_error) { // ... handle the connection error } return $this->conn; } } Also, remove the new mysqli(...) from underneath the class definition, it isn't needed. Here's the thing, though. Even with the updated class above, the class still doesn't do anything. It has no methods, and it can't interact with the mysqli class. All you are doing is instantiating a class to return a mysqli object. A wrapper class offering no additional functionality, is pointless. mysqli already has an OOP interface, you don't need to wrap it in a class. You could create a Singleton wrapper if you wanted, which would return a mysqli object, but the object can only ever exist once. This is a common thing, but I have to warn you not to let Singletons get to your head - they might seem useful and the best thing since sliced bread, but they really aren't. They open up a whole bunch of problems for unit testing and such, so use them wisely. On to your delete function. It is a bit of a mess. It is not really reusable and is pretty limited in its use. To make it a little more dynamic, you ought to pass the ID as a second parameter. That way, the ID doesn't always have to come from a querystring. Something like this: function delete_contact($db, $id) { // make sure $id is an int $id = (int) $id; // run the query $result = $db->query("DELETE FROM contacts WHERE id=$id"); return $result; } You can call it like this: delete_contact($db, $_GET['ID']); Or like this: delete_contact($db, 17); Or like this: delete_contact($db, someFunctionToGetAnID()); So you see, now it is more flexible. You might also note that I didn't put the redirect in there. The redirect shouldn't really be part of the function, that makes it too rigid. Put the redirect underneath the function call if/when you want a redirect. It now also returns a mysqli result, in case you need to work with it. I hope some of my rambling helped out. -
php upload size.. HOW to increase the max upload file size??
scootstah replied to vinsux's topic in PHP Coding Help
So... what did you change upload_max_size and post_max_size to? Did you restart Apache afterwards? What makes you think you are limited to 600KB? -
Thanks for that. The fact that a lawyer was also speaking made it that much better, in my opinion.
-
Then you'll probably want to attach an ID to the button, or a hidden form element or something to use in a WHERE clause.
-
Help in building a simple Shoping cart system
scootstah replied to Alucine's topic in PHP Coding Help
That sounds like a good place to start. -
You are using mysqli syntax with the mysql driver. It should be: while ($result = mysql_fetch_assoc($myData)){
-
Delete Stmt not deleting or going to header location
scootstah replied to iseriouslyneedhelp's topic in PHP Coding Help
Well, where/how are you calling it? You will need to pass in the mysqli function. -
Delete Stmt not deleting or going to header location
scootstah replied to iseriouslyneedhelp's topic in PHP Coding Help
It still doesn't add any useful functionality, and it is still using old code (namely ereg_* and mysql_*). -
Delete Stmt not deleting or going to header location
scootstah replied to iseriouslyneedhelp's topic in PHP Coding Help
Sorry, but that code looks like it was written 10 years ago. Please, do not use that. Everything in that wrapper is already natively supported by the mysqli driver. -
I'm not sure I fully understand your post, but you can't read remote files with PHP without using something like FTP.
-
Delete Stmt not deleting or going to header location
scootstah replied to iseriouslyneedhelp's topic in PHP Coding Help
Honestly, I think you are in need of a good book or two. You seem to be missing some of the basic fundamentals of (PHP) programming. -
Delete Stmt not deleting or going to header location
scootstah replied to iseriouslyneedhelp's topic in PHP Coding Help
That class is doing absolutely nothing, and you're not even instantiating it. -
Delete Stmt not deleting or going to header location
scootstah replied to iseriouslyneedhelp's topic in PHP Coding Help
No, now you are attempting to use $_GET['ID'] before the if conditional which checks to see if it is available. You need to put the assignment inside the if conditional. if(isset($_GET['ID']) && $_GET['ID'] == $ID) { $ID = $_GET['ID']; No, it needs to be $conn - because that is what you used for the function parameter. Can we see that? -
Delete Stmt not deleting or going to header location
scootstah replied to iseriouslyneedhelp's topic in PHP Coding Help
For starters, use brackets for conditionals. if(isset($_GET['ID']) && $_GET['ID'] == "$ID") { $stmt->query("DELETE FROM contacts WHERE ID = '$ID'"); header('Location: ' . $_SERVER['HTTP_REFERER']); } Where are you defining $ID? The function parameter is $conn, but you are using $stmt. -
You can use PDO::FETCH_ORI_ABS, but unfortunately it's not supported by MySQL. $result = $stmt->fetch(PDO::FETCH_ASSOC, PDO::FETCH_ORI_ABS, [row number]);
-
rofl I like your style.
-
Looks neat. Maybe I'll give it a try sometime.
-
Meh, I don't find Eclipse to be that slow. Sure it takes a second or two to startup, but that only happens once a day. What do you use?
-
increase count, but limited to one click?
scootstah replied to thetoolman's topic in PHP Coding Help
Some people's IP changes frequently, like daily/weekly. But, it is pretty easy to tunnel through a proxy which would give you a different IP. -
For the most part I use the default Eclipse theme, though I have modified a few of the colors for syntax highlighting. I also use the DejaVu monospace font.
-
increase count, but limited to one click?
scootstah replied to thetoolman's topic in PHP Coding Help
Not sessions or cookies - the user could clear them and recount. The "best" way would be to limit it to one per user, but that requires user registration. If you don't have user registration, the best you can do is by IP. But, there is no 100% reliable way to do it by IP since they are easily changed. -
I would change it to the following: $('img#accept').click(function(){ $('td#title-'+ id +' a').eq(0).css('color', 'white'); }); If that doesn't work, please include the markup in your next post.
-
No, that would get all the anchors within the element. By the way, if you already know the ID, you can use a more specific selector. $('td#title-' + id) However, if you use the .eq() method you can target a specific match. So, say we have this: <div id="test"> <a href="#">one</a> <a href="#">two</a> <a href="#">three</a> </div> Let's say you want to change the color of the second anchor to yellow. If you just did $('div#test a').css('color', 'yellow'); it would change all of them. But if you add .eq(), we can target the second one. $('div#test a').eq(1).css('color', 'yellow');