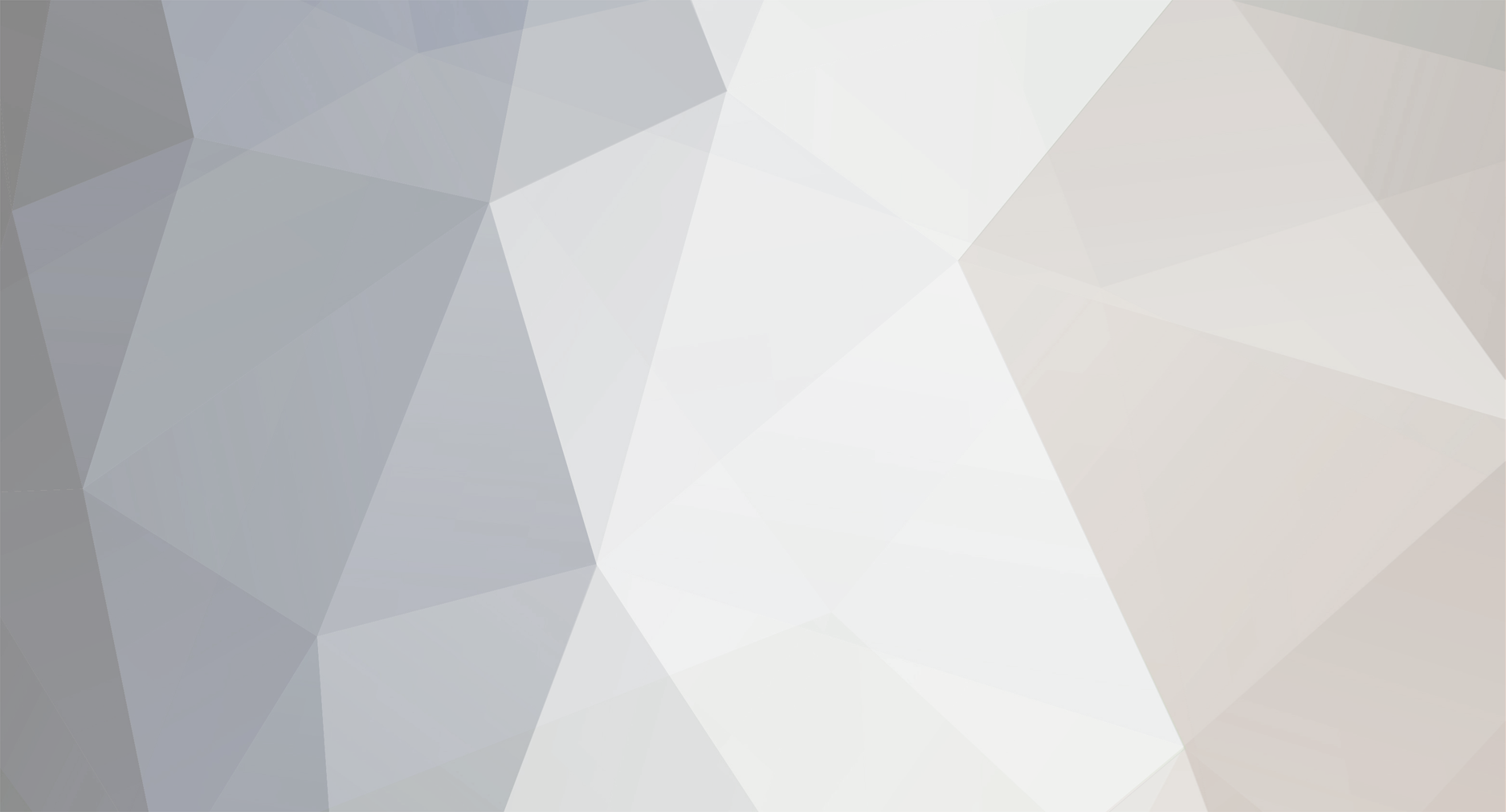
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
This will work: ^\d+(,{1}\d+)*$ Quantifiers need to be placed to the right of the subject. You are modifying the quantifier for the comma, not the digit.
-
How is the PHP accepting it? What are you doing with it?
-
Yes, the \d is a character class which means "digit". It is the same as [0-9]. The + means "match 1 or more of this pattern". The $ marks the end of the string. If that wasn't there, it would ignore anything after the pattern is matched as long as the pattern was matched once. So 1,2asdfsafaf would be valid. I find this cheat sheet to be a good reference: http://www.addedbytes.com/cheat-sheets/download/regular-expressions-cheat-sheet-v2.png Also, this site: http://www.regular-expressions.info/tutorial.html
-
Do you want to just strip characters out that aren't integers or commas, or give the user feedback? Strip out any characters that are not integers or commas: $input = '1,:25,,l2@'; $input = preg_replace('/([^\d,])+/', '', $input); // we might end up with two or more commas next to eachother, so filter that out $input = preg_replace('/(,{2,})+/', '', $input); Only accept the format: 1,2,3,4,5 $input = '1,2,3,4,5'; if (!preg_match('/^\d+(,{1}\d)*$/', $input)) { echo 'invalid input'; }
-
Since you're not using an AJAX form, it is kind of pointless to do this with Javascript. You need another page load anyway so you might as well just use PHP. You're going to have to use PHP anyway, because Javascript is easily circumvented - and since you need another page load anyway the Javascript won't matter. Save the selection to a session each time they submit, and then just omit the ones in the session from the dropdown box when they go to vote again.
-
Presumably in the character class, between the [ and ].
-
accessing methods of outer class from methods in inner class...
scootstah replied to Hall of Famer's topic in PHP Coding Help
You can extend the User class with the UserProfile and then access all of User's methods/objects. -
All you need to do is call date with just the day. echo date('d'); If you have a date string like 2008-12-30, you can first convert it to a UNIX timestamp and then you're free to use the date function. $date = '2008-12-30'; // convert to UNIX timestamp $timestamp = strtotime($date); echo date('d', $timestamp); Also, if you are getting this date from a MySQL database, you can optionally format it in the query instead.
-
Some people have Javascript disabled, so your banners would never show. That, and Javascript just doesn't seem to be the best solution here. Javascript should be used for manipulating client-side behavior. This doesn't really fall into that category.
-
No, you don't need jQuery for this. Simple Javascript will do.
-
In most cases you can simply add an "i" to the function to use the mysqli extension. However, there are a few small differences. Also, the mysqli extension supports both a procedural and an object-oriented API. You have to pick one though, you can't use them interchangeably. In my example I was using the object-oriented API, but in that last snippet you posted you are using the procedural API - which is fine, you just need to update the other things to procedural code. One more thing - the mysqli extension doesn't need the mysqli_select_db() call, because the database is usually selected during the connection. Keep in mind also that you need to carry around the mysqli connection for use with the procedural functions. The connection link identifier must be the first parameter for some of the functions (like mysqli_query()).
-
I just gave you another (working) way to do it, with PHP.
-
It is because in your example, you are using the mysql extension but in my example I am using the mysqli (notice the i) extension. The mysqli extension is the "updated" version of the mysql extension, and they are not backwards-compatible. The mysql extension was made for older versions of PHP, but has stuck around. It no longer has active development, and should really be avoided.
-
Well, it is called SimpleXML....
-
I don't know what things like "not displaying correctly" or "banner is wrong" mean. I'm not looking at your monitor. You need to include detail in your questions. Why are you even doing this in Javascript? From what I can see there is no reason to do so. $banners = array( array( 'url' => 'http://www.weareapackage.com', 'img' => 'http://www.weareapackage.com/images/WePackageBanner.gif', 'des' => 'Dating for Single Parents', 'wid' => 468, 'hei' => 60 ), array( 'url' => 'http://www.friendsordating.com', 'img' => 'http://www.friendsordating.com/images/bannersketch (2).png', 'des' => 'Free Dating for Singles', 'wid' => 468, 'hei' => 60 ), array( 'url' => 'http://www.lovelooker.com', 'img' => 'http://parentmeets.com/images/LoveLooker.gif', 'des' => 'Adult Dating', 'wid' => 468, 'hei' => 60 ) ); $banner = $banners[array_rand($banners)]; echo '<a href="' . $banner['url'] . '" target="_blank"><img src="' . $banner['img'] . '" width="' . $banner['wid'] . '" height="' . $banner['hei'] . '" border="0" alt="' . $banner['des'] . '" /></a>';
-
When you loop through $xml->children(), you will get an object for each child. So you would have a separate object for auto_play, loop, volume, and artist. These elements then become the root. So to get the song titles, you would do: foreach($xml->children() as $child) { echo $child->song_title[0] . '<br />'; }
-
Click a table, then click Structure, then click Relation View.
-
How would I go about writing a referral script?
scootstah replied to ffxpwns's topic in Applications
You use an UPDATE query with a WHERE clause. http://dev.mysql.com/doc/refman/5.5/en/update.html -
Do you agree with this statement or not?
scootstah replied to Hall of Famer's topic in Miscellaneous
That statement cannot be true because not everything in PHP is an object. -
echo <<<HEREDOC <script type="text/javascript"> banners = new Array(); banners[0] = "www.weareapackage.com,weareapackage.com/images/WePackageBanner.gif,Dating for Single Parents,468,60"; banners[1] = "www.friendsordating.com,www.friendsordating.com/images/bannersketch (2).png,Free Dating for Singles,468,60"; banners[2] = "www.lovelooker.com,parentmeets.com/images/LoveLooker.gif,Adult Dating,468,60"; rand = Math.floor(Math.random() * banners.length); b = banners[rand].split(","); url = b[0]; img = b[1]; des = b[2]; wid = b[3]; hei = b[4]; document.write('<a href="' + url + '" target="_blank"><img src="' + img + '" width="'+ wid +'" height="'+ hei +'" border="0" alt="' + des + '" /></a>'); </script> HEREDOC;
-
What do these give you? var_dump(file_exists('world.xml'), is_readable('world.xml'));
-
Because you have unclosed tags. It was just a snippet, you need to put the rest of the Javascript in there.
-
Does "world.xml" exist in the same directory as that script?
-
Or use HEREDOC. echo <<<HEREDOC <script type="text/javascript"> banners = new Array(); banners[0] = "www.weareapackage.com,weareapackage.com/images/WePackageBanner.gif,Dating for Single Parents,468,60"; banners[1] = "www.friendsordating.com,www.friendsordating.com/images/bannersketch (2).png,Free Dating for Singles,468,60"; banners[2] = "www.lovelooker.com,parentmeets.com/images/LoveLooker.gif,Adult Dating,468,60"; rand = Math.floor(Math.random() * banners.length); b = banners[rand].split(","); HEREDOC;
-
Yes.