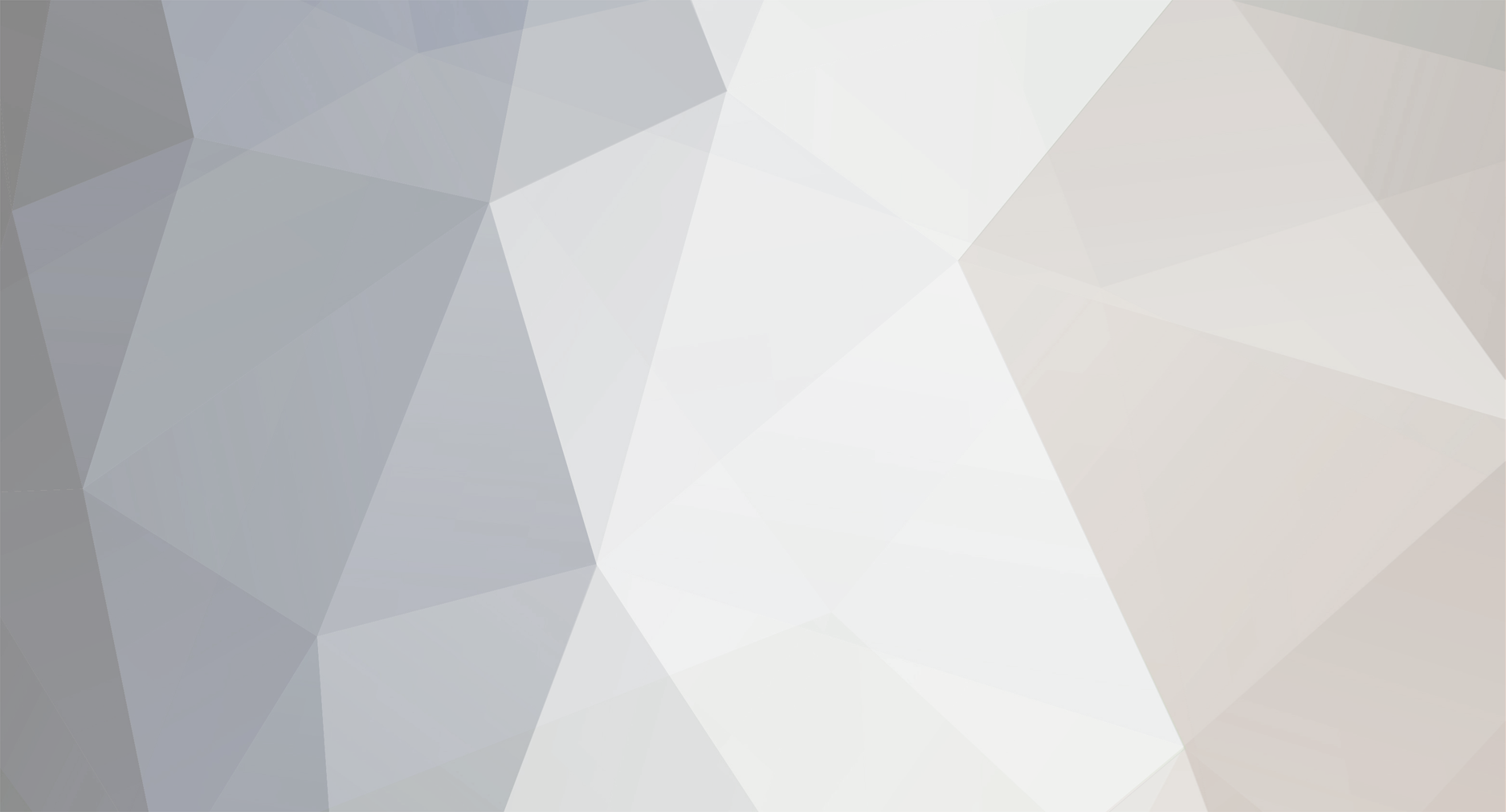
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
php URL field commands, Like .php?productID=X
scootstah replied to lobfredd's topic in PHP Coding Help
You'll have to construct your links to contain the querystring. When you receive items from the database, you'll have to loop through and dynamically create the links so they have the proper ID. To use the querystrings from the URL you'll need to use the $_GET superglobal array. The key will be whatever you put in the URL. For example if your link is http://example.com/products.php?id=7 then you would access it with $_GET['id']. Make sure, though, that you NEVER allow user input in your database queries. For example this is bad: SELECT * FROM products WHERE id=$_GET['id']; You always need to escape your user input to prevent SQL injection. In the case of numerical ID's, though, we can cheat a little by simply casting the value to an integer. Any bad characters will simply be removed, and you don't have to worry about it. So this would be okay: $id = (int) $_GET['id']; $result = mysql_query("SELECT * FROM products WHERE id='$id'"); -
That sounds good in theory, but prices fluctuate constantly (at least around here). I bounce between 3 different stores for groceries. Sometimes stuff is cheaper in one, sometimes it's cheaper in another. If the stores had some sort of API you could use that would work out well. Otherwise you could try to scrape for it, or just do a lot of manual work heh.
-
Ah, I see what you're getting at. My bad then, I should have been more specific.
-
Ah. Well that's not really related to the database at all.
-
Huh? I was implying the use of prepared statements. I figured if he was still using magic quotes there was a good chance he's also using the mysql extension, which of course does not support prepared statements. He could switch to MySQLi and use prepared statements too if he wanted, either way. I'm pretty sure you can use placeholders for LIMIT and such as well.
-
Well, you could add quotes automatically to $_POST, $_GET, and $_COOKIE. Those aren't necessarily your entire input, though, so I wouldn't feel comfortable doing that. You'll need to change stuff to remove them again though. I think you're going to have to bite the bullet on this one. While you're at it, swap to PDO so you don't have to worry about escaping.
-
Help converting an array into comma separated values
scootstah replied to TheBrandon's topic in PHP Coding Help
It's probably not the most efficient way, but this works: $input = array('3' => 3, '9' => 1, '15' => 9); $str = ''; foreach($input as $key => $val) { for($i = 0; $i < $val; $i++) { $str .= $key . ','; } } $str = rtrim($str, ','); EDIT: This is better: $input = array('3' => 3, '9' => 1, '15' => 9); $str = ''; foreach($input as $key => $val) { $str .= str_repeat($key . ',', $val); } $str = rtrim($str, ','); -
Oops, it was a typo ... I put a "-" instead of a "_". Fixed: $values[] = "('{$dm->sender_id}', '{$dm->sender_screen_name}')";
-
Firstly the JSON will be an object, not an array. You will need to use json_decode to turn the JSON string into a PHP object, which you can then loop through. Aside from that your insert syntax is incorrect. Try this: $values = array(); $values2 = array(); foreach(json_decode($direct_message) as $dm) { $values[] = "('{$dm->sender_id}', '{$dm->sender_screen-name}')"; $values2[] = "('{$dm->id}', '{$dm->text}')"; } $query = "INSERT INTO followers (user_id, user_alias) VALUES " . implode(',', $values); $query2 = "INSERT INTO d_messages (message_id, message) VALUES " . implode(',', $values2); mysql_query($query); mysql_query($query2); Also, there's really no need for a function here unless you are reusing it somewhere else too. It's just adding unnecessary overhead.
-
The difference in performance is negligible, though you are correct. If changes like these actually affect the way your script runs, you might want to look at moving away from PHP to a language designed for speed Or buy another stick of RAM and forget about it. Shaving nanoseconds off script execution is a waste of effort.
-
That's because $mysqli does not exist in that scope. You can't use variables globally in functions or classes. There is a number of ways to deal with this problem. Here is a couple of options: - Make a database wrapper which uses the Singleton pattern. This allows you to call the class globally. Singletons are a bit controversial however; they are considered an anti-pattern. It makes mocking difficult/impossible and violates a few OOP principles. - Use Dependency Injection to pass the mysqli object into your classes. This is probably considered the best option, but you may need to redesign parts of your application to make it work properly. - Use a Service Locator pattern to manage your database object (and others). This is on the same idea as the Singleton pattern and has the same downfall. - If all else fails, you can use the procedural API for the mysqli extension instead of the OOP one. This will allow you to globally call the functions anywhere in your application just like you did with the old mysql extension. If the concepts above are beyond your skill level at the time being, this may be an acceptable option. However, I encourage you to investigate the above options and try to extend your knowledge of how OOP works.
-
I do need properly placed <p> tags in order to style things like I want... Debbie How do you want to style it? I'm confident you can achieve it using CSS which is a lot less effort.
-
You can, in most cases. The procedural API for the mysqli extension is very similar to that of the mysql extension. Where exactly are you having trouble?
-
You don't actually need all of those paragraph tags for proper markup.
-
I *think* if you change the fadeOut part to this it should work: img.parent().fadeOut('slow');
-
can i Assign normal variables the Value of my SESSIOn variable
scootstah replied to rakyy's topic in PHP Coding Help
You need to have session_start() on both pages - the one where you set the session, and the one where you are trying to receive the session. It's easy enough to test, just make these two files: setsession.php <?php session_start(); $_SESSION['foo'] = 'bar'; getsession.php <?php session_start(); echo $_SESSION['foo']; -
This is probably unrelated, but one of your Javascript libraries (jquery-ui-1.8.19.custom.min) you are requesting is a 404 - you didn't put the ".js" at the end.
-
can i Assign normal variables the Value of my SESSIOn variable
scootstah replied to rakyy's topic in PHP Coding Help
Have you placed session_start() at the top of your script? -
Whoops, sorry about that. Make sure you only change it on the right side and not the left... IE: data : { image : img.attr('src') }, because otherwise you will need to change it in the PHP script as well. Speaking of which, are you using the PHP script I provided?
-
can i Assign normal variables the Value of my SESSIOn variable
scootstah replied to rakyy's topic in PHP Coding Help
Just like that. Yes, though make sure to use quotes between the square brackets otherwise you will get an error. $_SESSION['USER_NAME'] not $_SESSION[uSER_NAME] Also, make sure you have session_start at the top of your script. In order to work with sessions, this function MUST have been called. -
Okay, the problem is that you removed all of your Javascript and replaced it with mine. Your gallery appears to be fueled by Javascript so therefore it requires your code to function. Instead, you need to add the AJAX portion of my code to yours. This might work: Galleria.ready(function() { this.$('thumblink').click(); $(".galleria-image").append( "<span class='btn-delete ui-icon ui-icon-trash'></span>"); $(".btn-delete").live("click", function(){ var img = $(this).closest(".galleria-image").find("img"); // send the AJAX request $.ajax({ url : 'delete.php', type : 'post', data : { image : image.attr('src') }, success : function(){ alert('Deleting image... '); $(this).closest(".galleria-image"). fadeOut('slow'); } }); return false; }); });
-
Nothing in my script should do any of that.
-
If you're not sure what the proper forum for a topic is just place it in the one you think it should be in. If it's in the wrong spot a mod will move it.
-
You can't have two else's with the same if. You can, however, have multiple elseif's and then a else. For example: if (1 == 3) { // one equals three } else if (1 == 2) { // one equals two } else { // one doesnt equal either three or two }
-
Your code should work, but you need to place the AJAX portion in there.