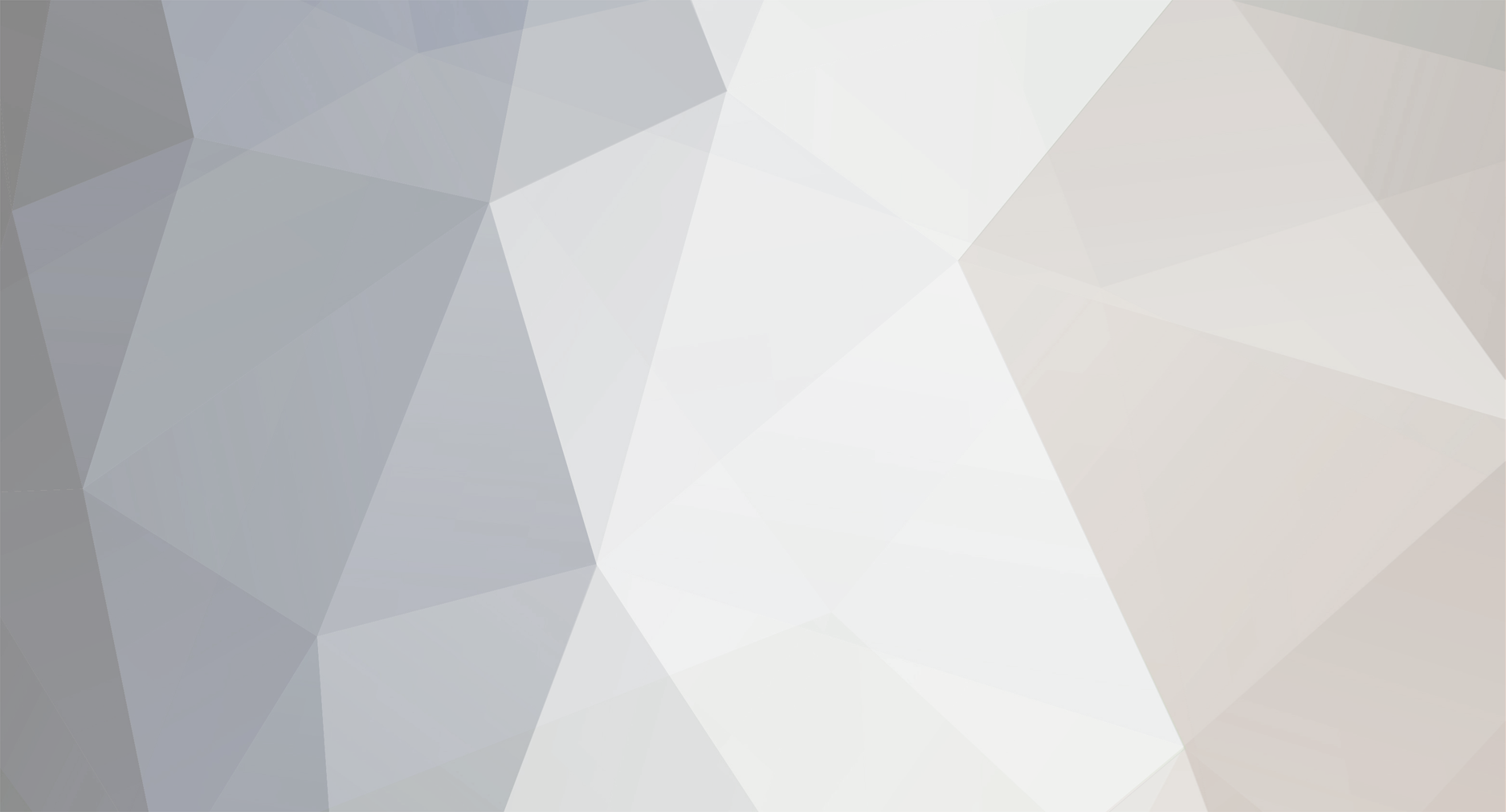
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Logic for my php project---need urgent assistance
scootstah replied to EdwinMurgoh's topic in PHP Coding Help
I figured he'd be doing that with application logic. -
I think my classes have to much responsibility
scootstah replied to fastsol's topic in PHP Coding Help
The problem is where do you start? If you change one thing, you break five others. Then you fix those, and break more. It's a tough situation. It is difficult to explain in a couple of paragraphs. My best advice is to dig through some other codebases and see how other people have solved these problems. Symfony2 is a good one. They've done a really great job at decoupling their components. Most object oriented web apps these days share at least a few commonalities. Typically they will have some way of routing requests to a controller. The controller is a class, with some methods inside. The controller is like a module, and the methods are the actions of that module. For example you might have a "NewsController", with methods like "create", "edit", and "delete". A controller should be very lightweight, and just act as the middle man to tie things together. The controller should usually return a response of some kind, and this response is then usually sent to the browser and the application request is then considered complete. If it were me refactoring your app, I would start with this lifecycle process. The code that you edited in for your admin page is in dire need of refactoring. You need to have separation of concerns. Right now you have HTML all mixed in with application logic... this is a very terrible way to work. Your view logic should be entirely separate. I like Twig. Twig is a template engine which gives you a very easy way to separate your view logic. There are other ways to do it, but that is my go-to. Once you can break your view logic out, and have more reasonable controllers, then you can get to work on your original two classes that you posted. Unfortunately you're going to be making a lot of dramatic, breaking changes. But, it's all for the better! And while you're doing all that refactoring, now is as good a time as any to get going with unit testing. -
Accessing global in constructor or parent class
scootstah replied to jbonnett's topic in PHP Coding Help
TDD basically implies that you're coding to a design. You design what the app should do, write tests, then write code. Yes, refactoring is important and a regular part of development. But you should still have a pretty good general idea of what you're building. -
Tons of ideas here: http://www.dreamincode.net/forums/topic/78802-martyr2s-mega-project-ideas-list/
-
Is your site on the internet so we can see it? Are you using Javascript to do anything on the inputs?
-
Loading messages from Twilio Account to Page
scootstah replied to Mckenzo101's topic in Third Party Scripts
I'm not sure what the difference between Message and SMSMessage are, but I found a delete method here: https://github.com/twilio/twilio-php/blob/master/Services/Twilio/Rest/Message.php#L63 -
Accessing global in constructor or parent class
scootstah replied to jbonnett's topic in PHP Coding Help
Writing code should be the last part of the process. You need a good idea of what your application is going to do before you start writing it. You can avoid lots of refactoring if you know where you're going to start with. -
I think my classes have to much responsibility
scootstah replied to fastsol's topic in PHP Coding Help
That's a pretty douchey thing to say. Especially for somebody with 3 posts. Just an FYI....object oriented is not the end-all, be-all. If you think otherwise, it is you who is the amateur. -
Then you need a for loop. http://php.net/manual/en/control-structures.for.php
-
Loading messages from Twilio Account to Page
scootstah replied to Mckenzo101's topic in Third Party Scripts
Did you try their documentation? https://www.twilio.com/docs/api/rest/message -
There's several ways that you could structure your frontend to obtain the desired information. An easy one is to just put a quantity beside Adult and Kid.
-
I mean that you cannot send output, wait for the API calls, and then send some more output. Output buffering can be used to order the output, but it still has to wait for the script to finish.
-
Your code works for me. Try dumping your session to see if it is working properly. Right underneath session_start();, add: var_dump($_SESSION);It should be empty the first time, and then contain the current page after a refresh.
-
Please use proper code indentation, so that your code is readable. <?php error_reporting(E_ALL); if (isset($_POST['submit'])) { $fielderror = ""; if ($_POST["percent"] == "" || $_POST["spots"] == "" || $_POST["cancel"] == "" || $_POST["mini"] == "" || $_POST["start"] == "" || $_POST["finish"] == "") { $fielderror = "Please fill the fields annotated with an asterisk<br>"; $doNotSubmit = true; } if (!is_numeric($_POST["percent"]) /*!(preg_match('#^\d+(\.(\d{2}))?$#',($_POST["amount"])))*/) { $fielderror = "Only Numbers In The Deposit Field"; $doNotSubmit = true; } if ($_POST['spots'] != "") { if (!is_numeric($_POST['spots'])) { $fielderror .= "Only Numbers In The Spots Field"; $doNotSubmit = true; } } if ($_POST['cancel'] != "") { if (!is_numeric($_POST['cancel'])) { $fielderror .= "Only Numbers In The Cancelation Field"; $doNotSubmit = true; } } if ($_POST['mini'] != "") { if (!is_numeric($_POST['mini'])) { $fielderror .= "Only Numbers In The Minimum Stay Field"; $doNotSubmit = true; } } if ($doNotSubmit == false) { $dbhost = 'localhost'; $dbuser = '####'; $dbpass = '####'; $dbname = '####'; // Database name $tbl_name = 'bookings'; // Table name $conn = new mysqli($dbhost, $dbuser, $dbpass, $dbname); $username = $_SESSION["class"]["username"]; // check connection if ($conn->connect_error) { trigger_error('Database connection failed: ' . $conn->connect_error, E_USER_ERROR); } $title = mysqli_real_escape_string($conn, $_POST['title']); $percent = mysqli_real_escape_string($conn, $_POST['percent']); $spots = mysqli_real_escape_string($conn, $_POST['spots']); $mini = mysqli_real_escape_string($conn, $_POST['mini']); $cancel = mysqli_real_escape_string($conn, $_POST['cancel']); $start = mysqli_real_escape_string($conn, $_POST['start']); $finish = mysqli_real_escape_string($conn, $_POST['finish']); $insert_row = $conn->query("INSERT INTO bookings (username, title, percent, spots, mini, cancel, start, finish, date_created) VALUES ('" . $username . "','" . $title . "','" . $percent . "','" . $spots . "','" . $mini . "','" . $cancel . "','" . $start . "','" . $finish . "', NOW(), ')"); if ($insert_row) { print 'Success! <br />'; } else { die('Error This happen : (' . $conn->errno . ') ' . $conn->error); } } }Your first problem is that you have only defined $doNotSubmit if one of the conditionals matches. So, you need to define a default for it. if (isset($_POST['submit'])) { $doNotSubmit = false;Your other problem is that you have a syntax error at the end of your query. NOW(), ')");
-
I think my classes have to much responsibility
scootstah replied to fastsol's topic in PHP Coding Help
Can you show me where processQuote() is being used? What is calling that, and when? -
What is your installer actually doing? Is it a typical installer where the user would enter in the database credentials and such? If so, the easiest way IMO is to just have a config value in that file saying that the site is installed. The database should return error codes if a connection could not be made. You can use these error codes to determine what is wrong. You will be able to differentiate the server being offline vs the database not existing.
-
I have to agree. It seems like you're in a bit over your head. You need to actually understand the things you are typing into your editor, and not just copy/pasting things that people told you should work.
-
Ha, yeah, there's your problem. Since your query is failing, you're running die() and thus your redirect never happens.
-
I would guess that the script is never reaching the bottom part, where you perform the query and redirect. Unrelated but this caught my eye: // Prepared statements are better, but at least escape things before tossing them into a query $id = mysqli_real_escape_string($db, $id);If you know you have an integer ID, you can just typecast it instead of escaping it. $id = (int) $id;
-
Accessing global in constructor or parent class
scootstah replied to jbonnett's topic in PHP Coding Help
If you're running out of memory, you probably have an infinite loop or circular reference or something somewhere. That can be difficult to track down without proper tooling. -
Logic for my php project---need urgent assistance
scootstah replied to EdwinMurgoh's topic in PHP Coding Help
You need to use SQL JOIN's. Your database schema needs some tweaking as well. You should not be storing multiple username fields across multiple tables. Instead, use a column to store the primary key of the related table. Also, contact_info should have a primary key. register has (id,firstname,lastname,email,username and password) health_profile has (id,user_id,blood_group,age and weight) contact_info has (id, user_id, phone, address, town).Now you can make user_id a foreign key associated with register.id and perform efficient queries. -
The term you're looking for is "asynchronous". You can't delay output with PHP alone. Output is sent once the script is finished. You would need to use Javascript to accomplish this. There are a number of ways to solve this. You could use AJAX with JSONP. Or if the API's allow CORS, you can just use straight up AJAX. Or, you could have a PHP script run in the background and then use AJAX to call that script.
-
I think my classes have to much responsibility
scootstah replied to fastsol's topic in PHP Coding Help
In processQuote() you're doing a whole bunch of validation. The way you are doing it is going to be very repetitive with different components, because now you have to implement the same repeated validation logic into every component. So, you can move all of that logic to some kind of validation or form component. Also, it looks like a lot of the code in processQuote() belongs in a controller of some sort. Overall your classes definitely have too much responsibility. When you create a class, you have to ask yourself what the class is going to do. It should have a very specific function, and only do that thing. If your class ends up doing more than one thing, you should break the extra thing into its own class and then tie them together with dependency injection. The same goes for methods within a class. Each method should only do one specific thing. It's very hard to write tests if your method is performing validation, updating database rows, and sending emails all at the same time. EDIT: From the class names, I'm not really sure what their specific purpose was supposed to be. "QuoteSystem" sounds very broad, and "QuoteResponse" could mean several things. Is it supposed to be an object that is a response to a quote, or is it supposed to be an output response for a quote? If you can clarify the specific purpose of these classes we might be able to help more. -
Look at your query. You are not selecting any columns with the names "insurance", "tax", or "mot".
-
Usually the installer would create a config file. You can simply check that some values exist in this config file to determine if it is installed.