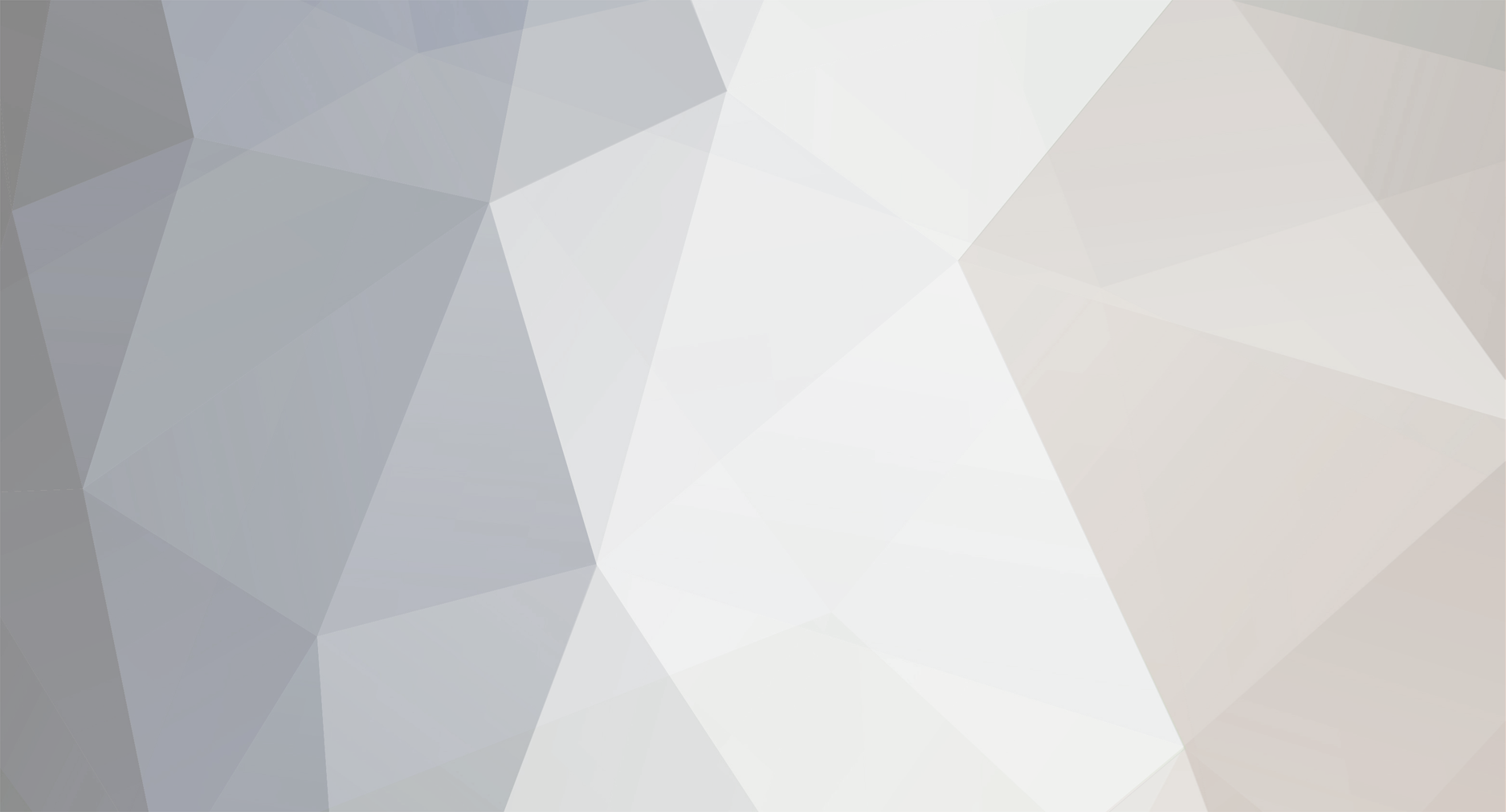
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
If I fudge some data in, your script works for me. Did you try looking at the page source to see if you have some weird HTML?
-
Your solution is not secure. Anyone that stumbles across that directory would be able to download the file. You can use PHP to send a Content-Disposition header (and some other headers) which will "force" a file download. Since it's through PHP, you can validate the request and make sure the user is allowed to have the file. Google "PHP force download". EDIT: You should store the files on the server, but you can keep a file pointer in the database.
-
The full template code, for the list view.
-
The easiest way with Javascript would be to return both templates with PHP, in a containing element, and then show/hide them on a click event. <div id="grid"> grid template here </div> <div id="list"> list template here </div>But, that's pretty inefficient, since you're doubling the amount of data you're returning to the browser. Another way is to return JSON or something, and format it into your templates with Javascript. If you don't want to use Javascript, your PHP logic is basically as such: $view = 'grid'; if (isset($_GET['view'])) { $view = $_GET['view']; } if ($view == 'grid') { // return grid template } else { // return list template }
-
To do it without a page reload will require Javascript. Otherwise, your PHP logic would basically just check which view was clicked and display the corresponding template. You could differentiate with a querystring variable.
-
Do you mean create a PDF with PHP? Yes, that is possible. See the PECL PDF extension here: http://php.net/manual/en/book.pdf.php
-
Displaying BLOB images horizontally from mysql
scootstah replied to projecttool8's topic in PHP Coding Help
You could actually just use CSS styling to achieve this. Give the images display: inline-block; and they will tile horizontally until they get to the edge of the parent container, then drop down and continue on. Example: http://jsfiddle.net/fzbe4jpm/ To achieve it with a for loop, you can use the modulus operator to decide when to insert a line break. $i = 1; foreach ($images as $image) { if ($i % 4 === 0) { echo '<br />'; } echo '<img src="' . $image . '" />'; $i++; }Something like that. -
You can process multiple file uploads by making the file input an array. <input type="file" name="files[]" /> <input type="file" name="files[]" />See the manual here: http://php.net/manual/en/features.file-upload.multiple.php
-
We're not here to write stuff for you, unless you want to provide compensation. You need to give it your best effort. If you're stuck on something specific, then you can post your code here and we'd be happy to help you through it.
-
Simply acquiring the refresh token does nothing on its own. The refresh token is used to "refresh" the access token. Normally in OAuth2, the access token is short-lived and expires quickly, usually in a few hours. You must send the access token in an Authorization header for each request so that the endpoint can authenticate you. When the access token expires, you would then send a different request to refresh it and get a new access token, without having to enter credentials again.
-
Seems like credential problems in both cases. Do you have a mail server on your localhost?
- 1 reply
-
- send email
- post to 127.0.0.1
- (and 2 more)
-
No you need to assign it to a variable. $refreshToken = $client->getRefreshToken();
-
$client->getRefreshToken() returns the refresh token. You're not doing anything with the return value. From the docs: /** * Get the OAuth 2.0 refresh token. * @return string $refreshToken refresh token or null if not available */ public function getRefreshToken() { return $this->getAuth()->getRefreshToken(); }
-
Best way to approach subboards on custom coded forums?
scootstah replied to idkwhy's topic in PHP Coding Help
Something like this? SELECT b.id, b.name FROM boards b LEFT JOIN boards sub_b ON sub_b.parent_id = b.idWhich will give results like:$results = array( array('id' => 1, 'name' => 'Parent Board', 'parent_id' => null), array('id' => 2, 'name' => 'Child Board 1', 'parent_id' => 1), array('id' => 3, 'name' => 'Child Board 2', 'parent_id' => 1), );Which you can then do something like:$boards = array(); foreach ($results as $result) { if ($result['parent_id'] !== null) { $parentId = $result['parent_id']; } else { $parentId = $result['id']; } if (!isset($boards[$parentId])) { $boards[$parentId] = array('children' => array()); } if ($result['parent_id'] !== null) { $boards[$result['parent_id']]['children'][] = $result; } else { $boards[$result['id']] += $result; } } foreach ($boards as $board) { echo '<h1>' . $board['name'] . '</h1>'; if (!empty($board['children'])) { foreach ($board['children'] as $child) { echo '<h2>' . $child['name'] . '</h2>'; } } }I just scribbled this out quickly. It works, but could be more optimized I'm sure. -
Everything is working. The problem with the styling is because your CURL request is only downloading the HTML, but not the associated CSS, JS, and image files.
-
What happens if you call $client->getRefreshToken()?
-
To session_regenerate_id() or to session_regenerate_id(true)
scootstah replied to ajoo's topic in PHP Coding Help
If you have a bunch of abandoned sessions laying around then it increases the chances that someone could guess a session ID. Again, you don't need to or want to be regenerating a session ID on every page load. What happens if a user opens your site in another tab? Now the session in the first tab will be expired. Also think about async requests from AJAX or such. -
There is no built in jQuery method to fetch query parameters. You could use something like this though: function getParam(paramSearch) { var queryString = document.URL.split('?')[1]; if (queryString) { var pairs = queryString.split('&'); for (var i = 0; i < pairs.length; i++) { var param = pairs[i].split('='); if (param[0] == paramSearch) { return param[1]; } } } return null; } var ref = getParam('ref'); if (ref) { document.write('<a href="http://www.newwebsite.com?ref=' + ref + '"><img src="image.png" /></a>'); }
-
Did you bother looking at the manual? I mean, one could probably guess with a reasonable amount of accuracy what this code does just by the name of the functions.
-
I would guess that you're either overwriting the session somewhere, not using session_start() appropriately, or some other math error resulting in 0. But without any code to look at, I'm just speculating here.
-
To session_regenerate_id() or to session_regenerate_id(true)
scootstah replied to ajoo's topic in PHP Coding Help
Yes, just a session_start(). It doesn't really have any bearing on session security. What I mentioned above only really helps when a session is actually compromised. It's an additional measure to try to make sure that a compromised session can't actually do anything special. For example, if you're an authenticated user going from a normal page to a restricted page, and want to do some restricted action, then the user should be re-authenticated (just have to enter their password) first. Then, regenerate the session. This elevated session should expire after a little bit of inactivity (10-15 minutes). In this way, if a user's session was compromised, the attacker may not actually be able to do anything without knowing the user's password. The best way to deal with session hijacking is to take every step to prevent it from happening in the first place. Here's some common things: - If on shared hosting, do not use the default session storage. Save it to your local web root, or to a database or something. Make sure if you do file-based sessions that the permissions are such that other system users can't access it. - Make sure you do not have any XSS vulnerabilities. - Make sure that the session cookie is using the "httponly" flag. This prevents it from being accessed client-side. - Optionally, you could choose to track the user IP and browser that created a session. By comparing these past and present values you might be able to detect a hijacked session. This isn't definitive because the user might be able to manipulate the values. Still though, it can be effective at times. - There are some additional entropy options that you can use to generate stronger session ID's. Have a look at these: http://php.net/manual/en/session.configuration.php#ini.session.entropy-file http://php.net/manual/en/session.configuration.php#ini.session.entropy-length http://us2.php.net/manual/en/session.configuration.php#ini.session.hash-bits-per-character http://us2.php.net/manual/en/session.configuration.php#ini.session.hash-function Also, check out this phpsec article. It helps you to understand how session hijacking and fixation occurs and ways to prevent it.[/url] -
Why would you use an Android IDE for PHP?
-
To session_regenerate_id() or to session_regenerate_id(true)
scootstah replied to ajoo's topic in PHP Coding Help
You do not need to regenerate the session ID on every page load. You'd normally only do that in cases where privileges change, or you enter a heightened area, etc. An example would be after a user logs in, you would want to regenerate the session ID after successful login. -
The ^ character inside of a character class ([ ]) inverts the pattern. This is a way of creating a whitelist of allowed characters. If $fullURL contains characters that are outside of the allowed whitelist, then the pattern will match and run the code inside the conditional. Hopefully that makes sense.