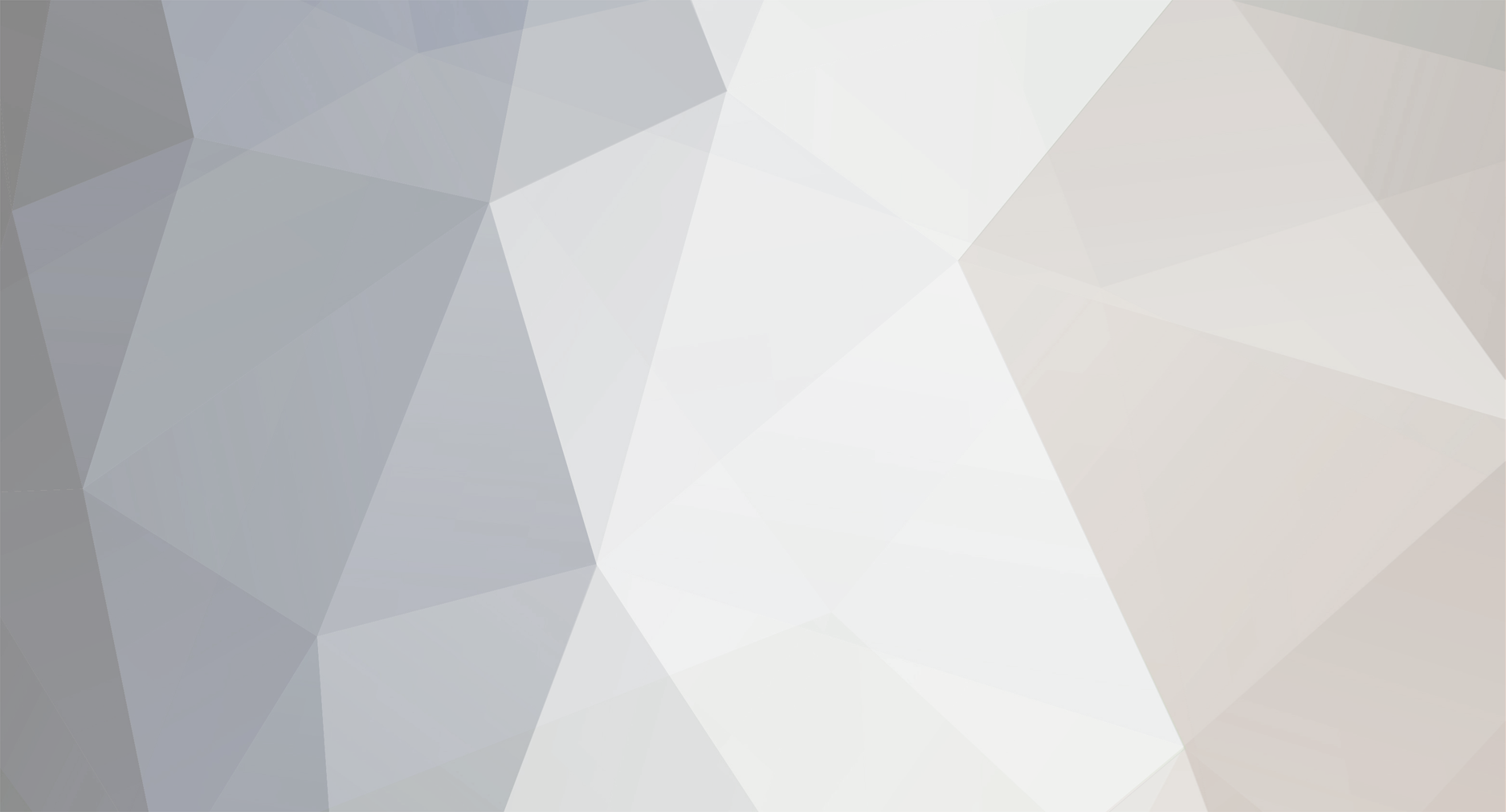
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Under most circumstances, you're absolutely correct. However, in the case of getimagesize(), you have to use the @ sign unless you have a known existing file and know that the file is a valid image. Unfortunately it does not throw an exception, and there's no other way to capture the error. OP: Since you're already using getimagesize(), you checking the file extension the way you are is pointless. getimagesize() returns the MIME type of the file, which is what you should be using to check the file type anyway - the file name provided in $_FILES is considered user input, and as such you cannot trust it. getimagesize() will return boolean false if the provided file either does not exist, or is not a valid image file. You can use this to your advantage. @$info = getimagesize($destination . $value); if ($info !== false) { // the image file is valid // allowed MIME types $allowed = array('image/jpeg', 'image/png', 'image/gif'); if (in_array($info['mime'], $allowed)) { // the file is a valid MIME type } }
-
You can use the width and height attributes on an <img/> tag to scale the image down. But it still loads from your server at full size, so that's not the most efficient way.
-
What mail client are you viewing it with? Most of the time HTML will be disabled by default, and you have to explicitly allow HTML in the email.
-
What is line 16?
- 16 replies
-
- php
- sort array
-
(and 1 more)
Tagged with:
-
Probably because you have a syntax error. Turn error reporting on! foreach($result['rows'] as $distance) {; <---
- 16 replies
-
- php
- sort array
-
(and 1 more)
Tagged with:
-
This is most likely a problem with your IIS configuration. Make sure that the POST verb is allowed, and make sure you don't have any extensions that will interfere with POST.
- 2 replies
-
- codeigniter
- post
-
(and 1 more)
Tagged with:
-
Here's how I'd do it. Change your form to this: echo"<input type='checkbox' name='comp[" . $row['id'] . "' value= '1' /> ".$row['competency']." <br /> </td>"; echo"<td> <select name='level[" . $row['id'] . "]'> <option></option> <option value='1'>level 1</option> <option value='2'>level 2</option> <option value='3'>level 3</option> <option value='4'>level 4</option> <option value='5'>level 5</option> </select> </td> ";And then process as such: <?php session_start(); $id = $_SESSION['user_id']; include ('mysql_connect.php'); $hobbies = $_POST['comp']; if (count($hobbies) !== 0) { echo("<p>You selected " . count($hobbies) . " Hobbies(s): "); $insert = array(); foreach (array_keys($hobbies) as $hobbyId) { $level = $_POST['level'][$hobbyId]; $insert[] = "(" . intval($id) . ", " . intval($hobbyId) . ", '" . mysql_real_escape_string($level) . "')"; } if (!empty($insert)) { $query = "INSERT INTO competency_result (user_id, competency_id, level) VALUES " . implode(',', $insert); mysql_query($query) or die (mysql_error()); $insertedId = mysql_insert_id(); } }
-
Had you actually bothered to read the code that Barand posted, you would probably realize that there is no header() in the middle of the HTML. You are simply using a PHP script as an image, with a simple <img/> tag. Perhaps you should read your own post - considering it does not say anywhere that you can not use this approach. Nor does it say you can't use CSS - in fact you even pointed out that you could use CSS. You're either a massive idiot, or just another worthless troll.
-
Live Search using Jquery (json) with Codeigniter
scootstah replied to skemi90's topic in PHP Coding Help
$.getJSON expects the first argument to be a URL. You're giving it a database result. -
1. The "rank" that you're using does not have any "members". 2. You're looping over a scalar in foreach(), not an array. Your foreach() should look like this (notice I changed the "ranks" index to 0, as that is the only one that has members in the data URL you provided): foreach ($data->data->hierarchy->Data->Ranks[0]->Members as $member) { echo $member->Name; echo '<br />'; }3. There is only one "member" for the 0th "rank" index. But, there are many ranks, and it looks like each one may have a member, so you might want to adjust your logic to get those as well.
-
Extract .zip file from other server to current server
scootstah replied to JenniferLawrence's topic in PHP Coding Help
How big is the file you're trying to download? You can try adding these two CURL options. It seems these have worked for others with the same problem. curl_setopt($ch, CURLOPT_HEADER, 0); curl_setopt($ch, CURLOPT_BINARYTRANSFER, 1); -
I'm not 100% sure what you're asking for here, but it sounds like you want a text field to show search results as you type using AJAX. If so, you just need an onkeyup event to trigger when typing in the text field. In the event handler, you'd perform an AJAX call to your server-side script, get the results, and then do whatever logic to add them to the search results. Here's a quick JSFiddle to illustrate. http://jsfiddle.net/2zrd10av/
-
You just call the properties like any other PHP object. echo $data->Total; echo $data->CrewId;If you pass true as the second argument to json_decode(), you will get an associative array instead. $data = json_decode($json, true); echo $data['Total']; echo $data['CrewId'];
-
Help improve login, session getting lost on android device
scootstah replied to lovephp's topic in PHP Coding Help
Absolutely do not use anything from that article. That's like 12 year old code and is very unsafe. You should never store passwords in a cookie (or any other sensitive information), and you should never use MD5() to store passwords. If I have to implement autologin myself (which, really, you shouldn't have to - use libraries), I will generate a random token and store it in the database, and a cookie. When a user visits the site and does not have an active session, the token from the cookie will look up the user it belongs to and they will be logged in. You can optionally add some additional checks like comparing browsers and IP to attempt to mitigate cookie theft. Make sure to also use HTTP only cookies, and always use HTTPS. You can also add a TTL to the tokens, and/or limit how many tokens can be active for a single user at one time. There's lots of variations here. -
Extract .zip file from other server to current server
scootstah replied to JenniferLawrence's topic in PHP Coding Help
Working code: <?php $ch = curl_init('http://localhost/phpfreaks/test.zip'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); $data = curl_exec($ch); curl_close($ch); file_put_contents(($zipPath = sys_get_temp_dir() . '/test.zip'), $data); $zip = new ZipArchive(); if ($zip->open($zipPath) !== false) { $extractPath = __DIR__ . '/extracted'; if (!file_exists($extractPath)) { mkdir($extractPath, null, true); } $zip->extractTo($extractPath); $zip->close(); } -
You need to set a default timezone in your php.ini http://php.net/manual/en/datetime.configuration.php#ini.date.timezone
- 16 replies
-
- php
- sort array
-
(and 1 more)
Tagged with:
-
$items is not defined in the code you gave at the time of calling ksort(). You should be developing with E_NOTICE errors turned on. You would have seen the undefined variable error. ini_set('display_errors', 1); error_reporting(-1);
- 16 replies
-
- php
- sort array
-
(and 1 more)
Tagged with:
-
Accessing global in constructor or parent class
scootstah replied to jbonnett's topic in PHP Coding Help
If you can't find anything obvious in your code, you should try using a profiler like Xdebug. Most decent IDE's will support Xdebug. You can use Xdebug to profile your application and determine exactly which functions are using lots of memory. This should help you pin down your memory leak. -
PHP Email Sends to Recipient Multiple Times
scootstah replied to dachshund's topic in PHP Coding Help
There's nothing there that would cause mail to be sent twice. You're either executing the page twice, or you have some weird mail server issue that I've never heard of before. -
OpenSSL certificate errors while connecting online
scootstah replied to mjahkoh's topic in PHP Coding Help
Re-install OpenSSL, and figure out where the cert.pem goes on Windows. On Linux it should be /usr/local/etc/openssl/cert.pem. Update openssl.cafile ini setting with the path. -
I think my classes have to much responsibility
scootstah replied to fastsol's topic in PHP Coding Help
DI is much better, in fact. It makes testing possible, and decouples your components. What if you wanted to take some of your classes and reuse them in another project? With hard coded dependencies it's basically not feasible to do so. Your other problem is that you're using static classes. You sort of lose most of the benefits of classes if you do that, you might as well just have a bunch of functions. If you instantiated Config, you could pass it as a constructor argument to GoogleAuth, or wherever it's needed. You've now decoupled your dependencies. If you want to reuse GoogleAuth in the future, you know that it depends on a Config class, but it is not implementation specific. -
If you don't know if the file is an image, you'll have to check getimagesize() for errors. If you don't give it an image, it will complain, so you have to suppress it. $imageSize = @getimagesize($file); if ($imageSize !== 0) { // good to go }
-
PHP Email Sends to Recipient Multiple Times
scootstah replied to dachshund's topic in PHP Coding Help
The email client that you're viewing the email with is probably adding that. If Zane is correct (which is what I suspect as well), you can solve the multiple emails by using a nonce token. The basic principle is that when the form is loaded, a token is generated and stored in a session, as well as in a hidden form field. When the form is submitted, your processing code will compare the hidden token with the session token, and then delete the token from the session once the form is finished processing. Therefore, the first time the form is submitted the token will be correct, but subsequent requests will fail since the token has been deleted from the session. -
No, you'd just make a "projects" table and then add a "project_id" to RFI's and submittals. A valid concern, but there are ways to prevent or mitigate that, such as mirroring and routine backups. These should be in place regardless. Well, your contractors wouldn't have access to the database, right? Therefore you can implement these restrictions in the code. I often see new comers worried about large databases. A modern database engine can scale to millions of records with ease. Although multiple databases can be a sound architectural decision in certain circumstances, I don't think this is one of them, based on the reasons you have given. I think you are just setting yourself up for a management nightmare.
-
I have the same problem... I was just messing with you mac It's definitely about 20 minutes behind.