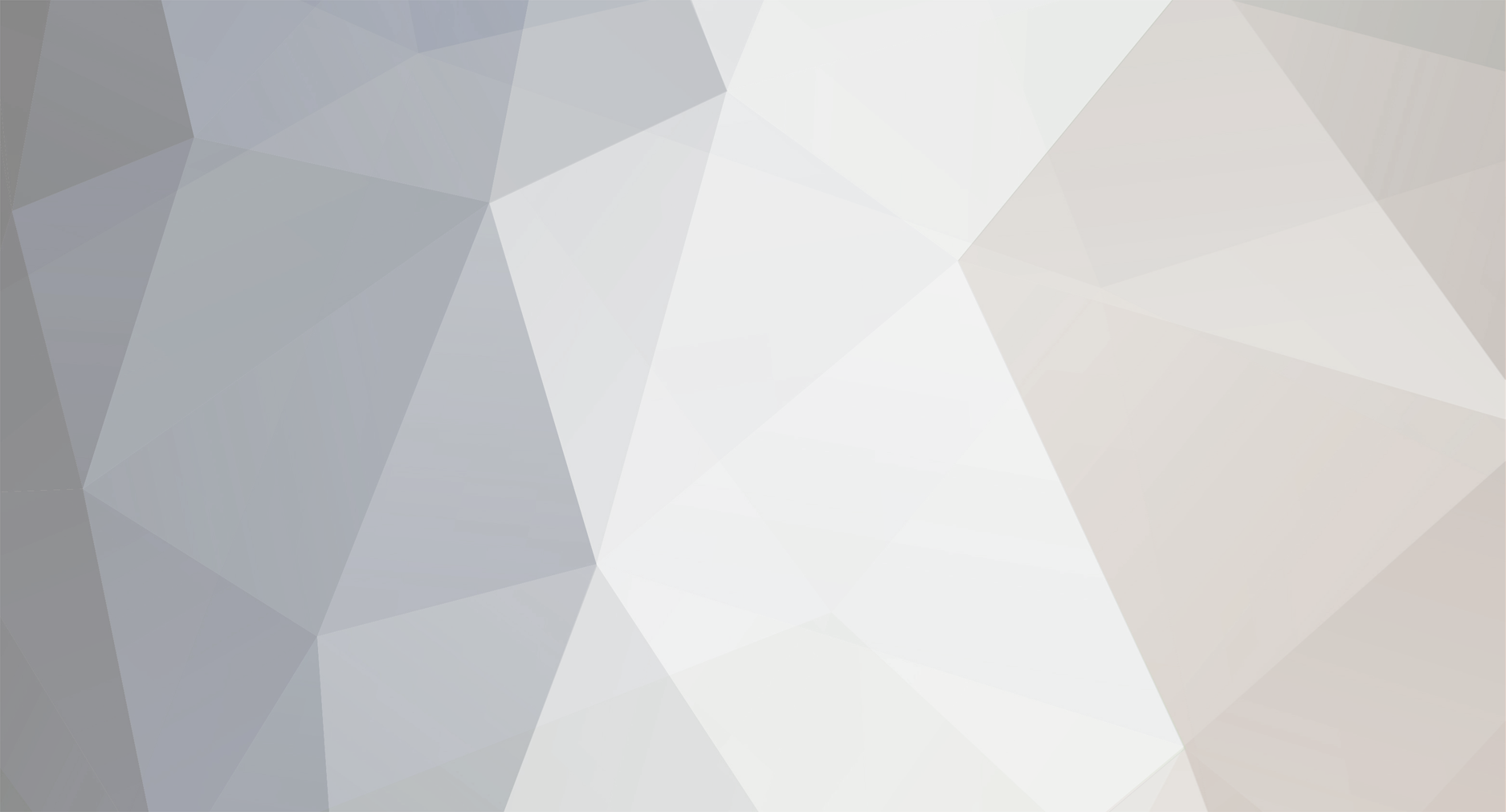
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
A little. Sure. $num = $_POST['one'] . $_POST['two'] . $_POST['three']; EDIT: Although I will tell you that having three fields for a phone number is very irritating as an end user. It's not really needed, and makes things like copy/paste difficult.
-
No. You'd need something like this: <?php $server = $_POST["server"]; $username = $_POST["username"]; $password = $_POST["password"]; $data = "<?php\n \n \$server = '$server';\n \$username = '$username';\n \$password = '$password';\n"; file_put_contents("/test/databasedata.php", $data);
-
I'm sure there's an easier way to do this but this is my quick attempt: $num = '(555) 123-4567'; // remove non-numerical characters $num = preg_replace('/[^\d]/', '', $num); // count the remaining characters if (strlen($num) == 10) { // separate the area code $areacode = substr($num, 0, 3); $num = substr($num, 3, strlen($num)); // split the rest into two parts $num1 = substr($num, 0, 3); $num2 = substr($num, 3, strlen($num)); // stick everything back together $num = '(' . $areacode . ') ' . $num1 . '-' . $num2; } You can do something similar with Javascript just for the user's convenience, but you should still validate server-side because Javascript can't be trusted.
-
Can you style the context that appears from the php code, if so how?
scootstah replied to usman07's topic in PHP Coding Help
Try changing "span" to "div". -
That's why you check the length after stripping that stuff out.
-
You need a float datatype. http://dev.mysql.com/doc/refman/5.0/en/floating-point-types.html
-
Why not accept it in any format they want to give it and then just construct it into your own format? For example they can input it as: (555) 555-5555 (555)5555555 555-555-5555 5555555555 It really shouldn't matter how they input it. Strip out all non-numerical characters, make sure it is the appropriate length, and then convert it to your preferred format.
-
Can you style the context that appears from the php code, if so how?
scootstah replied to usman07's topic in PHP Coding Help
<span style="font-family:helvetica; font-size:50px; padding-left:10px;"> -
Can you style the context that appears from the php code, if so how?
scootstah replied to usman07's topic in PHP Coding Help
<span style="font-family:helvetica; font-size:50px;"> You should take a look at some CSS tutorials. http://www.tizag.com/cssT/ http://www.html.net/tutorials/css/ http://www.csstutorial.net/ -
Can you style the context that appears from the php code, if so how?
scootstah replied to usman07's topic in PHP Coding Help
So you can either style everything in the while loop, like this echo '<span style="color:red;">'; while($row = mysql_fetch_array($result)) { // Loop through results $i++; echo "Displaying record $i<br>\n"; echo "<b>" . $row['id'] . "</b><br>\n"; // Where 'id' is the column/field title in the database echo $row['Location'] . "<br>\n"; // Where 'location' is the column/field title in the database echo $row['Property_type'] . "<br>\n"; // as above echo $row['Number_of_bedrooms'] . "<br>\n"; // .. echo $row['Purchase_type'] . "<br>\n"; // .. echo $row['Price_range'] . "<br>\n"; // .. } echo '</span>'; Or color everything in the while loop for each loop while($row = mysql_fetch_array($result)) { // Loop through results $i++; echo '<span style="color:red;">'; echo "Displaying record $i<br>\n"; echo "<b>" . $row['id'] . "</b><br>\n"; // Where 'id' is the column/field title in the database echo $row['Location'] . "<br>\n"; // Where 'location' is the column/field title in the database echo $row['Property_type'] . "<br>\n"; // as above echo $row['Number_of_bedrooms'] . "<br>\n"; // .. echo $row['Purchase_type'] . "<br>\n"; // .. echo $row['Price_range'] . "<br>\n"; // .. echo '</span>'; } Or color things in the while loop individually while($row = mysql_fetch_array($result)) { // Loop through results $i++; echo "<span style=\"color:red;\">Displaying record $i</span><br>\n"; echo "<b>" . $row['id'] . "</b><br>\n"; // Where 'id' is the column/field title in the database echo "<span style=\"color:green;\">" . $row['Location'] . "</span><br>\n"; // Where 'location' is the column/field title in the database echo $row['Property_type'] . "<br>\n"; // as above echo "<span style=\"color:blue;\">" . $row['Number_of_bedrooms'] . "</span><br>\n"; // .. echo $row['Purchase_type'] . "<br>\n"; // .. echo $row['Price_range'] . "<br>\n"; // .. } -
Can you style the context that appears from the php code, if so how?
scootstah replied to usman07's topic in PHP Coding Help
The same way you would with just HTML. <?php echo '<span style="color:red;">some red text</span>'; ?> -
I think you are opening a connection and you just don't realize it. You can't run queries without a connection.
-
Update Table ONLY if the value has changed
scootstah replied to blackdogupya's topic in PHP Coding Help
Yeah, this. Otherwise you're going to have to do something which is inefficient and nonsensical. -
Can you style the context that appears from the php code, if so how?
scootstah replied to usman07's topic in PHP Coding Help
<?php echo '<b>this is some html inside php!</b>'; ?> -
Why?
-
Can you style the context that appears from the php code, if so how?
scootstah replied to usman07's topic in PHP Coding Help
Use HTML. -
Try it instead with an actual HTML tag, and you will see that it does indeed change. $str = '<b>bold</b>'; echo htmlspecialchars($str); On screen this will be: <b>bold</b> In the source, it will be: <b>>bold<</b>
-
So what is the value of $MyCode? extract($row); var_dump($MyCode);
-
Typically you will have an "install" script that must be run before the application can be used. Usually, among other things, that install script will ask for the MySQL credentials. Then they will be saved to a file (using file_put_contents()) which your main application includes.
-
This is one of those times where reusing code is of massive benefit. Otherwise, you're going to have repeated logic all over the place, which is not a good way to work. So, in lieu of that I whipped up this class. I did this in only a few minutes so, I'm sure there's some catastrophic failure to be had. However, from my few tests it appears to work alright. If anything, it's a place to start. class FormValidation { private $errors = array(); private $rules = array(); private $error_msgs; public function __construct() { // set some error messages $this->error_msgs = array( 'required' => '%s is a required field' ); } public function setRules($rules) { // add some rules $this->rules = array_merge($this->rules, $rules); } public function getErrors($field = null) { // if no field is specified, get all errors if ($field == null) { return $this->errors; } // otherwise try to return an error for the specific field return isset($this->errors[$field]) ? $this->errors[$field] : null; } public function run() { // if POST is empty, do nothing if (empty($_POST)) return true; // loop through the rules foreach($this->rules as $rules) { $field = $rules['field']; $label = $rules['label']; $rules = explode('|', $rules['rules']); // loop through and test each rule foreach($rules as $rule) { // do we have a method to handle this rule? if (method_exists($this, $rule)) { $result = $this->$rule($field); // nope. how about a function? } else if (function_exists($rule)) { if (isset($_POST[$field])) { $result = $rule($_POST[$field]); } } // no problems, NEXT! if (!isset($result)) continue; // if $result is a bool and FALSE, set an error if ($result === false) { $this->setError($field, $label, $rule); // otherwise, set the $_POST value to the one returned } else { $_POST[$field] = $result; } } } // return a bool return empty($this->errors); } private function setError($field, $label, $rule) { // set an error $this->errors[$field] = sprintf($this->error_msgs[$rule], $label); } private function required($field) { return isset($_POST[$field]) && !empty($_POST[$field]); } } And to use it // instantiate the class $val = new FormValidation; // set some rules // each array represents a single field // the "field" is the name of the form element // the "label" is a friendly name (what appears in the error) // the "rules" is the criteria it must meet to pass // you can separate rules with a vertical pipe ( | ), // and use any function that accepts a single parameter // or any methods for the FormValidation class $val->setRules(array( array('field' => 'firstname', 'label' => 'First Name', 'rules' => 'required'), array('field' => 'lastname', 'label' => 'Last Name', 'rules' => 'required') )); if ($val->run()) { echo 'PASSED'; } else { echo 'DIDNT PASS'; print_r($val->getErrors()); }
-
Then something like this should do that (modifying Psycho's function): function removeHTML($inputStr) { $outputStr = preg_replace('#<br[\s\/]*>#i', "\n", $inputStr); $outputStr = strip_tags($outputStr); $outputStr = nl2br($outputStr); return $outputStr; } This will: 1. Convert all variations of <br> to newline characters, 2. Remove all HTML tags, and 3. Convert newline characters back to <br>. So you will remove all HTML while preserving line breaks.