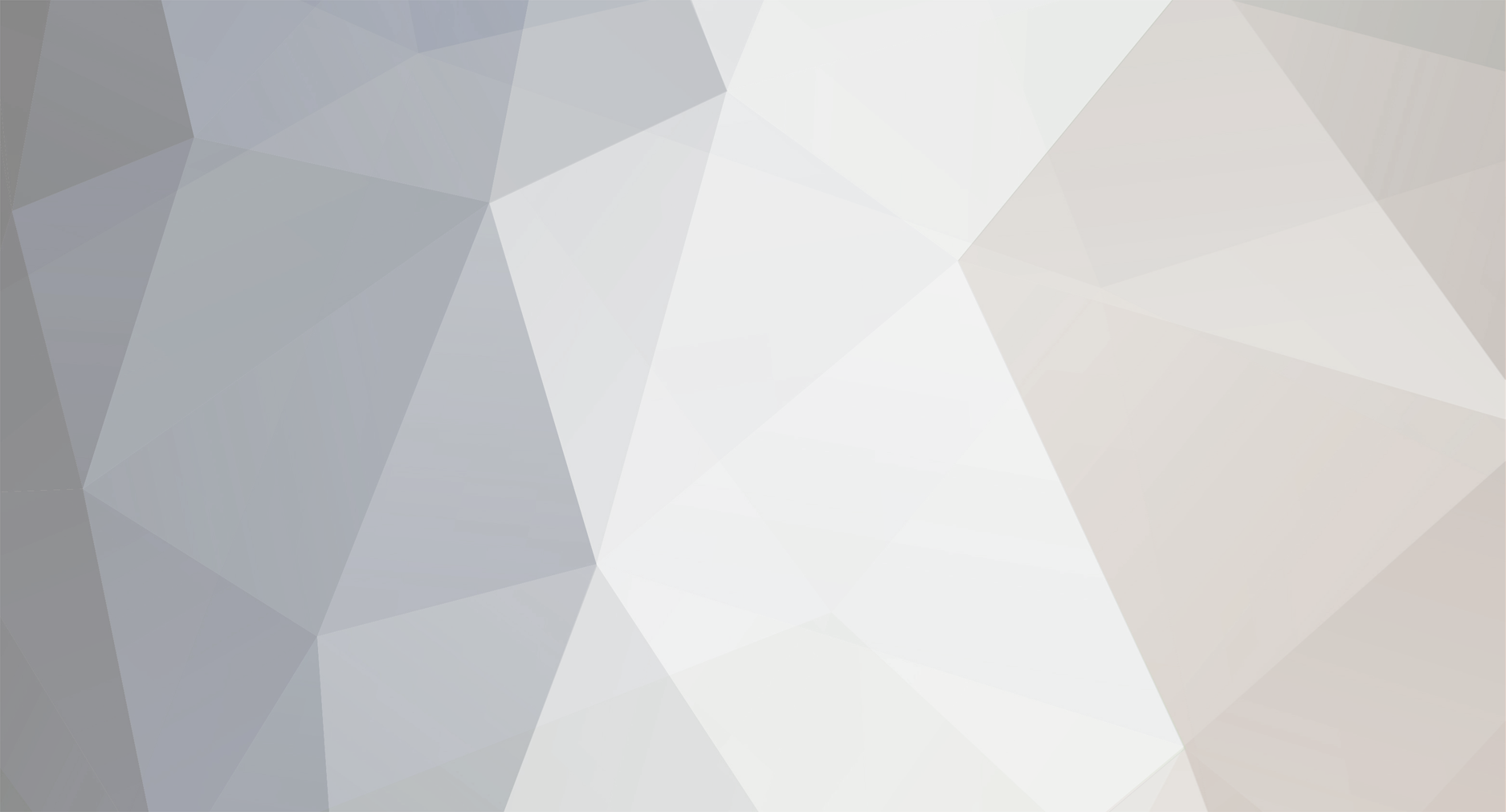
nethnet
Members-
Posts
284 -
Joined
-
Last visited
Everything posted by nethnet
-
As far as I know, there's no support for anything like this in PHP.
-
Yes, the problem is that when PHP comes across those single quotes, it thinks it is supposed to stop echoing and move on to the next command, but you don't want it to do that, so you need to escape them by placing a \ before them. This will essentially tell the PHP parser to skip over them in terms of syntax. So yeah, put a backslash (\) before all of the single quotes (') except for the two that I pointed out earlier, since you DO what PHP to parse those
-
I don't know what exactly Plesk has to offer, but it may have an SSH client built in to the control panel. If not, download PuTTy and use that. It's really easy to use, and if you are going to be editing config files, I would recommend learning how to use SSH
-
Also, this section of the code should look like: greensite='.$domain.' ^ These should be the only single quotes in that line that you do not escape.
-
You need to escape every single quote in the line you added by placing a backslash in front of it.
-
Try this: <?php // Some variables to keep track of the bgcolor $ticker = 0; $lastcustomer = ""; // Begin the table echo "<table>"; $query = ("SELECT * FROM `table` ORDER BY `customer`, `id`"); while ($row = mysql_fetch_assoc($query)) { if ($row['customer'] != $lastcustomer) { $ticker++; $counter = 1; } $bgcolor = ($ticker % 2 == 1) ? "#FFFFFF" : "#CCCCCC"; echo "<tr>"; foreach ($row as $key => $value) if ($key == "id") $value = $counter; echo "<td style=\"background-color: $bgcolor; \">$value</td>"; echo "</tr>"; $lastcustomer = $row['customer']; $counter++; } // End the table echo "</table>"; ?> Change `table` to your table name, obviously
-
Well, it would be the same thing that is used to query your database for login. Do you already have that part written? Include any code you already have for the login script, and I'll try to modify it to allow what you want.
-
Use sessions. Include this at the top of every page: <?php session_start(); ?> Then, when a user logs in, set a session variable. <?php $_SESSION['name'] = $name // Or whatever you have it named ?> That variable will "carry over" onto every page as long as you have session_start() at the top. <?php echo "Hello, {$_SESSION['name']}!"; ?>
-
You could add a `replyto` field in your first table, and get rid of the second table altogether. Just include the `id` of the first message in the `replyto` field if it's a direct reply, otherwise have it set to 0. When you select your messages from the database, do a double ORDER BY.. first on the field `replyto` and second on the field `date`. Any rows with a `replyto` value of 0 will be standalone messages (or the first message of a thread), and all others will be replies. Then if a user is viewing a message with `id` of 45, any messages with `replyto` of value 45 should be printed as well in a thread style.
-
I see, I read that wrong. I thought you were searching based on today's date. But regardless, TIMESTAMP is essentially the same as DATETIME, so just build a string around the search date, and use that in place of NOW() in the example I posted. $searchdate = $_POST['year'] . "-" . $POST_['month'] . "-" . $_POST['day'] . " 12:00:00";
-
Put all of your functions that will be used across multiple scripts in an include file, something like 'functions.inc.php' and then include them at the start of every page. include "functions.inc.php"; That way you won't have to duplicate code.
-
Thanks for pointing that out. Reading back, I'm not even sure why I brought that up in the first place. I guess that's a sign that it's bed time!
-
Are your fields for startdate and enddate the mysql DATE type? If so, you could just do this: SELECT * FROM `table` WHERE `startdate` < NOW() AND `enddate` > NOW()
-
They are similar, but have different outputs and default split lengths. str_split() returns an array and defaults to a split being made every character. chunk_split() returns a string with \r\n placed every 76 characters. For both functions, the split length can be changed with an optional 2nd parameter. In essence, str_split is useful for exploding strings based on a chunk length as opposed to a needle with explode(). chunk_split() is primarily useful for formatting certain long strings to meet RFC semantics. This is mostly for hash values and encryption methods that potentially produce strings longer than 76 characters.
-
I always recommend storing dates in MySQL as DATETIME, or just DATE. Lots of people just end up doing it as VARCHAR, but DATETIME is the best way to go. This gives way to all of MySQL's built in features for handling them when extracting data, as well as (albeit not as useful) inputting data. Then, when you go to do a SELECT statement, you can just ORDER BY your DATETIME field. ASC would put oldest first, while DESC would put newest first. SELECT * FROM `table` ORDER BY `date` DESC LIMIT 5 That would display the 5 latest events. As far as hiding past events, you could use the handy UNIX_TIMESTAMP() function built into MySQL and just add a bit more to your SELECT statement. SELECT *, UNIX_TIMESTAMP(`date`) AS `unixdate` FROM `table` WHERE `unixdate` > NOW() ORDER BY `unixdate` DESC LIMIT 5
-
If I understand correctly, your data might look something like the following: +----+----------+------+--------+ | id | customer | case | status | +----+----------+------+--------+ | 1 | Mollie | c1 | Active | | 2 | Mollie | c2 | Closed | | 3 | Drew | c3 | Active | | 4 | Drew | c1 | Active | | 5 | Drew | c4 | Pend | | 6 | Ed | c2 | Active | | 7 | Sara | c1 | Closed | +----+----------+------+--------+ I have no idea what `case` and `status` are, so I just gave them values for how I might picture them. Correct me if I'm wrong but what you want to do is print out each record, and alternate the bgcolor of the rows for each NEW customer, and NOT for each new row.. right? If so... <?php // Some variables to keep track of the bgcolor $ticker = 0; $lastcustomer = ""; // Begin the table echo "<table>"; $query = ("SELECT * FROM `table` ORDER BY `customer`"); while ($row = mysql_fetch_assoc($query)) { if ($row['customer'] != $lastcustomer) $ticker++; $bgcolor = ($ticker % 2 == 1) ? "#FFFFFF" : "#CCCCCC"; echo "<tr>"; foreach ($row as $value) echo "<td style=\"background-color: $bgcolor; \">$value</td>"; echo "</tr>"; $lastcustomer = $row['customer']; } // End the table echo "</table>"; ?> This is untested, so I may have forgotten a semicolon or something. Edit: Looks like I was beaten to the punch.
-
And if you want to get really savvy, you could rent a 5-digit phone number made specifically for handling this sort of thing and then build a nice C application around it for handling not only outgoing texts, but incoming ones as well.
-
Well, any scripting language that supports SMTP will inherently allow SMS messaging as well, since nowadays phone numbers are (essentially) email addresses, but whether or not they have extensive support regarding this is questionable. I'd look into Coldfusion or a .NET framework. I would imagine they would be most likely to serve extended compatibility with SMS. I'd be surprised to find anything with Ruby, but I suppose there is a chance that there is a Rails library set up for doing this, since it has become a more common feature in recent years. Rails is just so... new... with regards to most other scripting frameworks, so it's a tossup really. Now that I think about it, I'm pretty sure I remember running across a full featured Coldfusion script for doing this, so that's where I'd put my money.
-
Welcome jj-dr You'll probably soon come to realize that these forums are just about as addictive as PHP itself
-
I must have missed this line when I read your message :/ I just did some research into it, and apparently this method is only viable if the number is exactly 10 digits, and if you are operating in a US gateway. Sorry for overlooking that. PHP isn't your friend in this case.
-
First of all, PHP *probably* isn't your best option. Sure, it can be done, but PHP lacks any inherent capabilities of handling this specifically, at least in it's current versions. Regardless, if you must use PHP to do it, realize the following: 1. You will need to know the users mobile carrier. 2. Some carriers will append ugly text to your SMS. 3. This may or may not work internationally. Essentially, you will be using the users phone number in conjunction with PHP's mail() function. You will need to use a switch statement to decide which extension to use in your "to" field, such as "@vmobl.com" for Virgin Mobile or "@vtext.com" for Verizon Wireless. Each carrier has a unique extension, so you will need to find a list of all applicable ones for your purposes. A user with a phone number of 215-555-8993 for T-Mobile would have an address of "[email protected]"
-
I did indeed read that and tried it as well. Turns out the official Ruby version when doing a yum install on Fedora is 1.8.6. I was attempting to use RubyGems to get Rails 3.0.*, which require Ruby 1.8.7 -or- 1.9.2. I ended up downloading RVM and using that to install and default Ruby 1.9.2 on my server (which automatically included the latest release of RubyGems.. problem solved!). After that it was just a matter of installing rails. Thanks for the input Maq. I had noticed references to RVM on several sites that I found, so I finally decided to look into it. Turns out it was the solution all along.
-
First of all, I'm new to Ruby. Second of all, don't castrate me for running Fedora Core 7. I'm at the mercy of GoDaddy's virtual dedicated servers Okay, so basically, I've been trying to get Rails installed on my server for the past day, and I'm not entirely sure what the problem is. Ruby and RubyGems are already installed, and a quick check shows their paths: [root@ip-*** /]# which ruby /usr/bin/ruby [root@ip-*** /]# which gem /usr/bin/gem So, according to like.. 20 different sources I've found online, all I would need to do is... gem install rails This is where I encounter my problem. [root@ip-*** /]# gem install rails ERROR: While executing gem ... (Gem::RemoteSourceException) HTTP Response 302 Other sources have said I need to update my RubyGems, but this error duplicates itself when I try. [root@ip-*** /]# gem update --system Updating RubyGems... ERROR: While executing gem ... (Gem::RemoteSourceException) HTTP Response 302 Env data for RubyGems shows: [root@ip-*** /]# gem env RubyGems Environment: - VERSION: 0.9.4 (0.9.4) - INSTALLATION DIRECTORY: /usr/lib/ruby/gems/1.8 - GEM PATH: - /usr/lib/ruby/gems/1.8 - REMOTE SOURCES: - http://gems.rubyforge.org Like I said, I'm a Ruby n00b, so any help would be appreciated. I would really like to get Rails up and running on my system. Thanks! If you need more info, just let me know what I'm leaving out.
-
I didn't read through your code completely since it was a bit long (and it's a bit late) but essentially what it sounds like you want to do is a simple setup like this: <?php if ($_SESSION['UserID'] > 0) { // Everything that should happen if the user is already logged in } else { // Log the user in and set the $_SESSION['UserID'] variable } ?> I put "> 0" in the if statement, because I noticed you set the variable equal to 0 in the htmlheader.php file.
-
Replace the three instances of $stringtotest with the variable that contains your *possible* base64 hash. I'm guessing that's your text file that you have stored in $data? If you wanted to continue to refer to it as $data after the test, replace $decoded with $data as well. Either way, the $decoded/$data variable will contain the decoded version of the base64.