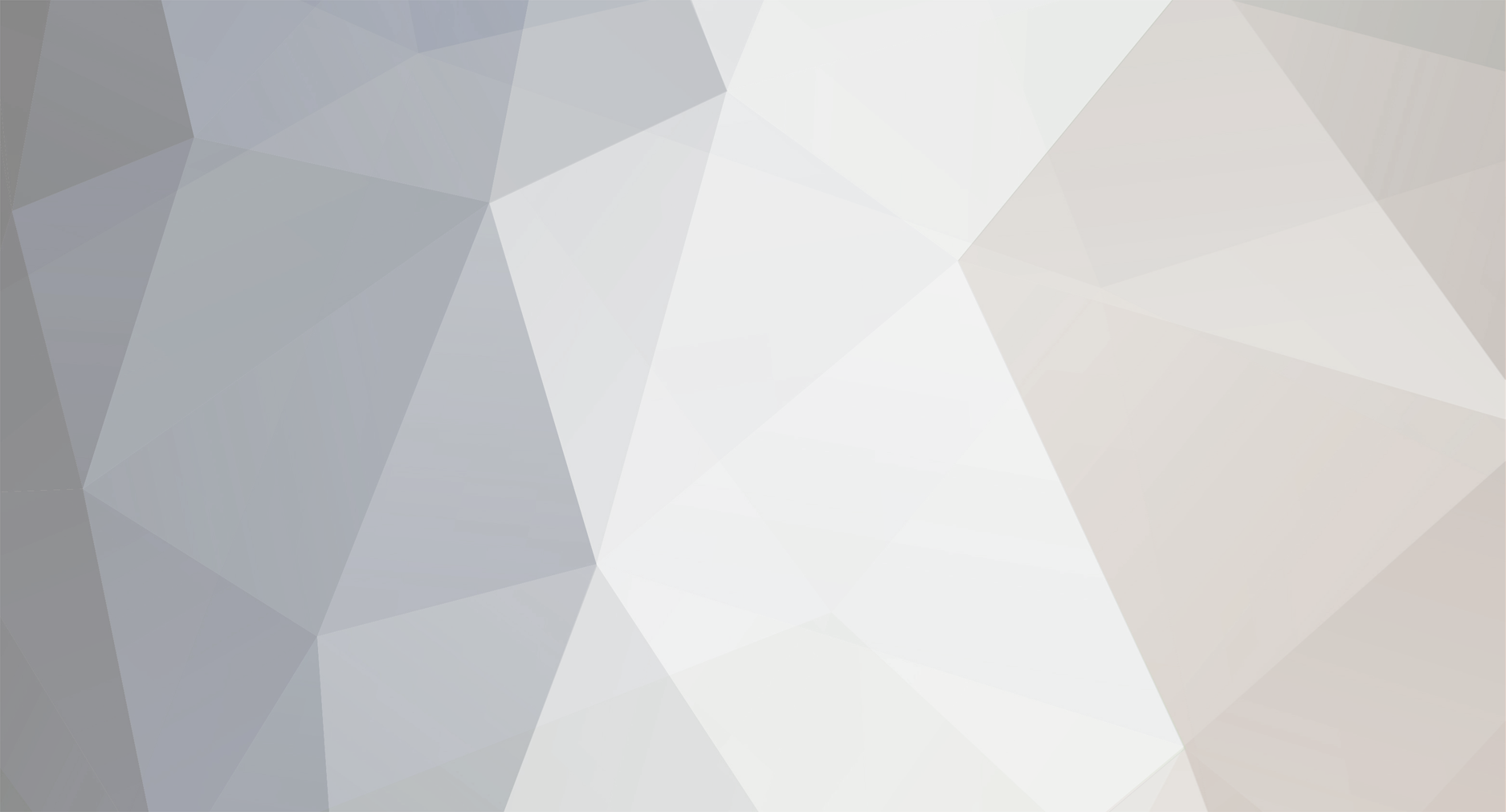
nethnet
Members-
Posts
284 -
Joined
-
Last visited
Everything posted by nethnet
-
You set $image1 to equal the string "image1" and then you tried echoing that into your <img> tag, so essentially what you printed to the browser was: <img src="image1" width="200"> What kadeous was saying is to store the location of the image in your database, not the image itself. Say you wanted to use the image located at "http://www.yoursite.com/images/test.gif". In your database, you could store this as just a filename and location, and then echo that to your <img> tag. Your database would look something like: ------ -------------------- | id | location | ------ -------------------- | 0 | 'images/test.gif' | | 1 | 'images/test2.gif' | ------ -------------------- And then you would select it from the DB in the same way as you do the rest of your data, and print it like this: <img src="<?php echo $result['location']; ?>" width="200">
-
Try this instead: WHERE `question` LIKE '%$question%' AND `notes` LIKE '%$notes%' LIMIT 0, 10 Edit: I'm assuming you want your `notes` field in your database to contain the $notes variable you defined before the SQL.
-
Start a session name from a result from a login
nethnet replied to Russia's topic in PHP Coding Help
Before this line, $_SESSION['uID'] = $result['user_id']; You need to give the $result array some actual values. Right now that variable is just a resource identifier for your mysql_query. You can use any of the mysql_fetch_array(), mysql_fetch_assoc(), or mysql_fetch_object() functions to do this. I'm more of an OOP guy so I prefer mysql_fetch_object, but for your purposes, any of them would work equally well. $assoc = mysql_fetch_assoc($result); $_SESSION['uID'] = $assoc['user_id']; Hope this helps. More about these functions: mysql_fetch_array() | mysql_fetch_assoc() | mysql_fetch_object() -
Well, that depends what you are trying to do with 'searchterm'. What section of the database are you trying to search through? In general, a search query would look something like this: $query = mysql_query("SELECT * FROM `table_name` WHERE `column` LIKE '%{$_POST['searchterm']}%'"); `column` is whatever field you want to search for 'searchterm' in. You have a query similar to this already in your script using the $question variable as your search term, so are you trying to combine these two queries into one (a.k.a. find records that match BOTH of these requirements)? A little bit more detail about what exactly you are trying to search for and how you want to implement that with your pre-existing code would help.
-
If you're sure it's a timeout issue, you can either change the value of max_execution_time in your php.ini file, or probably more appropriately just use set_time_limit() at the top of your script so as not to change your timeout server-wide.
-
Don't mention it, that's what I'm on these forums to do As long as you understand what the code is doing, I don't feel too guilty writing it myself, haha.
-
Well, if they clear their sessions or close their browser, you would have no way of knowing through PHP unless you used a more robust AJAX ping system, which for most practical purposes, is unnecessary (unless, of course, you are building some chat interface or game schematic where showing users online in realtime is important). So yeah, in those cases it would stay TRUE, but after 5 minutes of inactivity, they would still show up as offline (the timer acts as a bit of a fallback for users who don't click logout or close their browsers).
-
It wouldn't be very difficult to do that, try something like this (I added the `loggedin` field to the script that I mentioned in my last post.. just remove that part from the SQL syntax if you don't want to do that): <?php $fifteenminsago = time() - (5 * 60); $sql = "SELECT * FROM `users` WHERE `lastactive` >= '$fifteenminsago' AND `loggedin` = TRUE ORDER BY `lastactive` DESC"; $query = mysql_query($sql) or die (mysql_error()); $rows = mysql_num_rows($query); if ($rows > 0) { $i = 1; while($online = mysql_fetch_assoc($query)) { echo '<a style="color:#F0CD87;" href="profile?id='.$online['user_id'].'">'; echo ucFirst($online['username']); echo '</a>'; if ($i < $rows) { echo ', '; } $i++; } } else { // No records found } ?> I also made the timer set to 5 minutes. This is the most common 'timeout' that most websites use to determine which users are online. But again, just change it to whatever if you don't want to make it 5 minutes.
-
A better way to make users who log off not show up anymore would be to create a boolean `loggedin` field in your users database. Whenever a user logs in, set this value to TRUE. When a user logs out, change it to FALSE. Then, in your online users list, change the SQL to exclude any user who has a FALSE `loggedin` flag. SELECT * FROM `users` WHERE `lastactive` >= '$fifteenminsago' AND `loggedin` = TRUE ORDER BY `lastactive` DESC
-
Just change the $fifteenminsago variable to: $fifteenminsago = time() - (1 * 60); All you have to do is change the integer '1' to however many minutes you want (it was at 15 before).
-
<?php $fifteenminsago = time() - (15 * 60); $sql = "SELECT * FROM `users` WHERE `lastactive` >= '$fifteenminsago' ORDER BY `lastactive` DESC"; $query = mysql_query($sql) or die (mysql_error()); if (mysql_num_rows($query) > 0) { while($online = mysql_fetch_assoc($query)) { echo '<a style="color:#F0CD87;" href="profile?id='.$online['user_id'].'">'; echo ucFirst($online['username']); echo '</a>, '; } } else { // No records found } ?>
-
It works AND gives you a MySQL error? Usually when I run into that error, it is because I have prematurely called mysql_fetch_assoc before confirming that there are indeed rows returned by the query (if the query returns 0 rows, it will throw that error). Usually I would confirm that there is at least 1 record returned using mysql_num_rows before I call mysql_fetch_assoc, but if your script is working and returning records from the database, I'm not sure why/how there would be a MySQL error.
-
Try something like this in your query: SELECT * FROM `users` WHERE `lastactive` >= '$fifteenminsago' ORDER BY `lastactive` DESC You would define your timer variables as such: $fifteenminsago = time() - (15 * 60); Hope that helps.
-
Just as I said, use the OR operator. SELECT count(*) AS total FROM `users` WHERE `user_name` = '$name' OR `user_name` = '$name2' OR `user_name` = '$name3' That way it will match any record where `user_name` is equal to any of the three $name variables.
-
The strtotime() function is notoriously strict on the input you can give it. I'm not particularly well versed in its acceptable syntax, but I would guess that you cannot have any whitespace between the plus sign and the integer when marking it 4 days from now.
-
You say if you enter a number, it will show properly. But where are you entering a value? Where is your $user array declared? If we see that, we may be able to better pinpoint the issue.
-
Hi mnewberry, To check multiple fields in an SQL SELECT statement, use the AND and/or OR operators. For example: SELECT * FROM `people` WHERE `firstName` = "Sam" AND `lastName` = "Jones" SELECT * FROM `people` WHERE `location` = "USA" OR `age` > 18
-
At the top of the script where you have this... echo "Welcome, ".$user['username'] etc.. does that properly show 'Tom' on the webpage, or does it also show 'Array'? If it shows 'Tom' then it's a simple matter of a typo in your script.
-
in_array not working--trouble with needle array
nethnet replied to RopeADope's topic in PHP Coding Help
The problem is that you are using an array as your needle in the array_search() in your else statement. It is using only the value at key 0 for this (a.k.a your '.' directory). Here's a quick fix: <?php $needles=array('.','..','css','php','input_forms'); $t1=scandir($_SERVER['DOCUMENT_ROOT']); foreach($t1 as $t1_value){ if (!is_dir($t1_value)){ unset($t1[array_search($t1_value,$t1)]); }else if (array_search($t1_value,$needles) !== false){ unset($t1[array_search($t1_value,$t1)]); } } ?> This time, if it's a directory, it is checked against the $needles array, and if it does in fact match, then it is unset from the scandir array. -nethnet -
Include the entire file here so we can see why there's a problem.
-
It would only read that if there was an error in the username or password. If that 'else' statement isn't executed, then $error will never be declared. You can put $error = false in the 'if' part of that statement, so that no matter which way it goes, $error is being declared, and the notice will never be generated.
-
Put the error reporting lines back at the top of the script (and for future reference, it is helpful to always have these lines at the top of your scripts during development, and then remove them when you make your scripts public, or set their values to 0). Error reporting is a programmers best method of debugging. This will show you your original problem, an undeclared variable. Something like this is a rather unimportant matter when compared to things like parse errors and stuff like that, but nevertheless, if PHP is pointing it out, it's a good idea to fix it. If you put this at the top of your script, after your error reporting lines, the problem should be fixed. $error = false; Basically, the problem was that you were attempting to use your $error variable before you declared it and gave it a value. By adding that line, you declare it, so it will no longer produce the undeclared variable notice when you try to use it later. Additionally, it will still return false when you do use it in your if statement, which is what you want. Later, you will be able to add your own error checking code to the script, which should give $error a value such as "Invalid username" or whatever errors you need for your form (I'm assuming it is a registration script?) That way, $error will initially have a value of false, but this value will be changed to whatever error your script finds (if there is one).
-
Well, for sake of clarification, the scripts do not "work" anymore now than they did before. All you did by removing the error reporting was to tell PHP to not notify you of any notices. It is still happening in the background, you just can't see it.
-
You aren't listening to what we are saying. The error is happening because the $error variable is not registered as having any value. Therefore, when you attempt to use it, PHP throws a notice to the browser (the lowest level of error). This notice is NOT displayed when you only run the second set of code by itself because, since it is only a notice, it is not displayed by default in PHP's settings. When you put the two sets of code together, it is displayed, because you have the lines: ini_set('display_errors', 1); error_reporting(-1); Which is essentially saying "If there are any errors at all, including miniscule notices, print them to the browser for the user to see." The first set of code isn't preventing the second set from working, it is merely telling the second set to print this notice of an undeclared variable to the browser. The important part of this is that the error is happening whether or not you include the first set of code. Like I said earlier, you are trying to use the $error variable without previously defining it in your script. Try adding this to your code after your error reporting statements: $error = FALSE; This will declare the variable for your script's sake, but it will still prevent your if clause from returning any error present. Edit: Place that boolean declaration as high in your script as possible, so that any code that may give $error a value later in the script will still do so without it being reverted back to FALSE.
-
Look into the ZipArchive class. I believe this extension is preloaded on most hosts, but if it's not on yours, you should contact your webhost to have it added. A quick phpinfo() will show you if it's there. And of course, if you manage your own server, compile PHP using --enable-zip (on Linux) or enable php_zip.dll in your php.ini (on Windows).