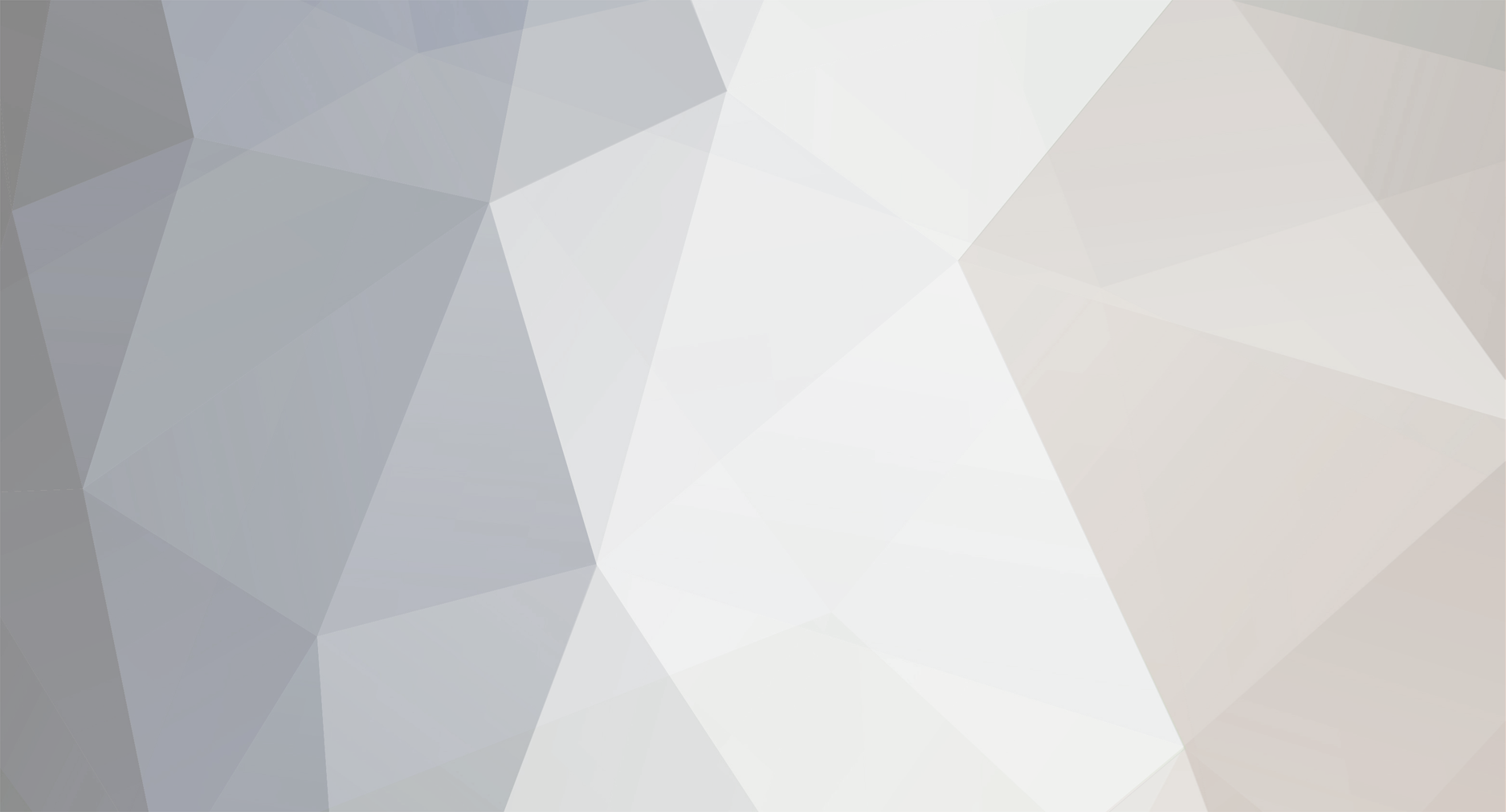
Christian F.
-
Posts
3,072 -
Joined
-
Last visited
-
Days Won
18
Posts posted by Christian F.
-
-
-
You're welcome, glad I could help.
-
First off there's no need to escape that double quote there, as it's not a meta character in either RegExp or single-quoted PHP strings.
Then to your issue: What you want to look for is everything that's not a "<", instead of everything up to the "</a>" tag. In other words
[^<]+
is what you're looking for. -
You're welcome.
-
Ah... Re-reading the code I see I missed out on some details, sorry about that.
Considering what I previously missed, it seems like you want to take the first element from the $files1 array, rename it to the first result from the MySQL query. Then take the next element, rename it to the next number, and so forth.
If that's the case, then you'll want to look at
array_shift ()
instead offoreach ()
andcontinue;
. -
I think you're looking for
continue;
, just add that below the rename and you should be good to go. -
There are a couple of steps that you want to do:
- [*]Create a user-specific random hash value.
[*]Add this to the password.
[*]Hash the password using a strong hashing algorithm (sha256 or better).
[*]Store both the hashed password and the salt in the DB.
Do this every time a user changes the password, and never ever save (or send) the unencrypted password anywhere. Possible exception to this, is if the user generates a random password. Then have it invalidated the first time the user logs on with it, but generally you'll want to avoid this if possible.
Some people advocate running step 3 multiple times, adding the salt to the hash for each time. Whether or not this is actually adding anything is a point where the experts are divided on, so I can't say either way.
I'd also advocate using a second hash value, which is stored in your applications configuration file. A site-specific hash value, if you want. That way even if your DB is leaked, the attackers won't have the full hash without gaining read-access to all of your PHP code. (In which case you're fscked anyway.)
-
Not quite on-topic, but...
I would actually recommend you to scrap DreamWeaver, and instead start to write the PHP code by hand. Start simple, with a tutorial showing you all of the basics, then work your way up. I believe the PHP manual has a good tutorial to start with.
In either case, the PHP manual is a very good resource, and which is highly recommended to read through!
-
If you look at this part here:
if (!isset ($_POST['chosen'])) { $var = "4"; } elseif (isset ($_POST['chosen'])) { array_push ($_SESSION['options_picked'], $var);
You can see that you're trying to push the variable onto the $_SESSION array, without having it defined first. So if none of the predefined categories are selected,
$var
will be undefined and cast to an empty string when used in the query.I assumed this was because you mistakenly believed it being set above, due to the lack of indenting.
PS: I also recommend moving the HTML header, doctype and all that out of the
$output
variable. It doesn't need to be there, after all.PPS: You need to validate input, and escape the output in your SQL queries.
-
For this you'd want to bind () a function to the checkboxes, and have that function check whether or not it's marked (or whatever else you want to do).
-
Your problem is the use of
[^.]
, which translates to "not a character" (or "only newlines", depending upon the modifier used).I've corrected the RegExps, plus removed some unnecessary capturing and make things a bit cleaner.
<?php function insert_rewrite_rules ($rules) { $newrules = array (); $page_name = 'mypage'; //basic rule $newrules['(' . $page_name . ')$'] = 'index.php?pagename=$matches[1]'; //search by id $newrules['(' . $page_name . ')/(\d+)$'] = 'index.php?pagename=$matches[1]&id=$matches[2]&search=1'; //url with page included $newrules['(' . $page_name . ')(?:/(\\w+)-bedrooms)?(?:/(\\w+)-bathrooms)?/([^/]+)/(\\d+).html$'] = 'index.php?pagename=$matches[1]&bedrooms=$matches[2]&bathrooms=$matches[3]&query=$matches[4]&pagem=$matches[5]'; //url with no page $newrules['(' . $page_name . ')(?:/(\\w+)-bedrooms)?(?:/(\\w+)-bathrooms)?(?:/([^/]+))*$'] = 'index.php?pagename=$matches[1]&bedrooms=$matches[2]&bathrooms=$matches[3]&query=$matches[4]'; return $newrules + $rules; } add_filter ('rewrite_rules_array', 'insert_rewrite_rules');
-
You'll first want to read this:
http://php.net/manual/en/function.printer-open.php
If that doesn't help, you'll need to look at the documentation for the printer driver, and see if you can find the syntax of the protocol it uses. Also, the PHP manual should be able to help you figure out how to connect to the printer, depending upon which type of connection it uses.
-
Have you read in the PHP manual for the basic syntax of the language, as well as
substr ()
?We're not here to write the code for you, you know, but to help people who've tried, got stuck, and generally wants to learn.
-
I'd use templates. Either in the form of a template engine, or via
sprintf ()
.Something like this:
// At the top of the page, or perhaps in a different file. $postTemplate = <<<PostTemp <span class="text">%1\$s</span> <div class="info"> <a class="author" href="#"><img src="%2\$s"> <span class="name">%3\$s</span></a> <span>%4\$d curtidas</span> <span>%5\$d comentarios</span> </div> PostTemp; // Inside the function that writes the post. $formatted_bloomp .= sprintf ($postTemplate, htmlspecialchars ($bloomp['text']), rawurlencode (base_url ('upload/picture/' . $bloomp['author_picture'])), htmlspecialchars ($bloomp['author_name']), $bloomp['likes_number'], $bloomp['comments_number']);
-
Please use [code][/code] or [php][/php] tags when posting code, as it makes it a whole lot easier to actually read both the post and the code.
That said: You have a syntax error on line 12, which you should fix.
Line 12: $newrules[(mypage)(/(\.....
-
/^((?: |\s)+.*?) /
That's a slightly better RegExp, as it'll match one or more " " or space, as many as it can, before finding content which isn't. Then it adds that content, until it hit the first " " again (which is not included).
-
They are looking for a PDF file that contains all the information in it so there copy write team can put it on the site.
*Headdesk*
Set up an API do export it as JSON or XML, or even CSV. It's trivial to have a web site import any of those formats, and a LOT more sane than exporting to PDF for then to manually type it into the DB.
That whole PDF issue just reeks of wooden tables.
-
I recommend properly indenting your code, then it should be apparent what the problem is.
-
Can you post the code where you're using this RegExp?
-
I strongly recommend reading up on LEFT JOIN, and how to use it.
From what I gather of your code, you're trying to list up all of the options for all of the cart items of the current user. This is exactly what JOINs are made for, and which will allow you to pull all the relevant data from the database in a single query1.
If you look at a previous post I made, you'll find a nice example on how you can do what you're looking to do here.
PS: Remember that the less code you use to achieve what you want, generally the better it is. You want to keep things simple, and avoid repeating patterns of code. Your if-tests and echos are prime examples of repeated patterns which could have been merged, using variables for the tiny bits that differ between them.
1Database queries are quite expensive time-wise, so most of the time you really want to avoid using them in a loop.
-
What you need is an UPDATE statement, with a WHERE clause to limit the rows updated to what you want.
Something like this should work, though it might require you to join table B so try it on some test data first.
UPDATE `table_a` AS a SET a.`boolean` = $Val WHERE `table_b`.`name` = a.`name`
Another way of doing it is by using WHERE IN() and a subquery. A quick google search will give you lots of examples on how this is done.
-
What you're looking for is to use a HTML form to POST back data to the script.
If the script detects that data has been POSTed back, then take that data, validate it and then use it to constrain the MySQL query.
The PHP manual has a little howto on this subject, which I recommend reading.
-
Try Google: Lots and lots of examples on using AJAX and PHP to communicate with databases.
-
Compiler != decompiler. The former is to make things machine-readable (and/or quicker to execute), while the latter is for making obfuscated/compiled code human readable again.
variable not passing second time
in PHP Coding Help
Posted
As far as I can see
$var
is still not being set, unless one of the IF-tests checks out true. Which means that it's highly likely that they don't.I'd run a
var_dump ()
on the contents of the$_POST
array, and see what it contains. Also, run a test of invalid categories, before running the loop. That way you control what should be done, and not the database driver.PS: I'd use the value from the database as the value for the categories, and not base it upon the name. Or, if that's not viable, use an array of key->value bindings and check against it for validation.
Example of the latter: