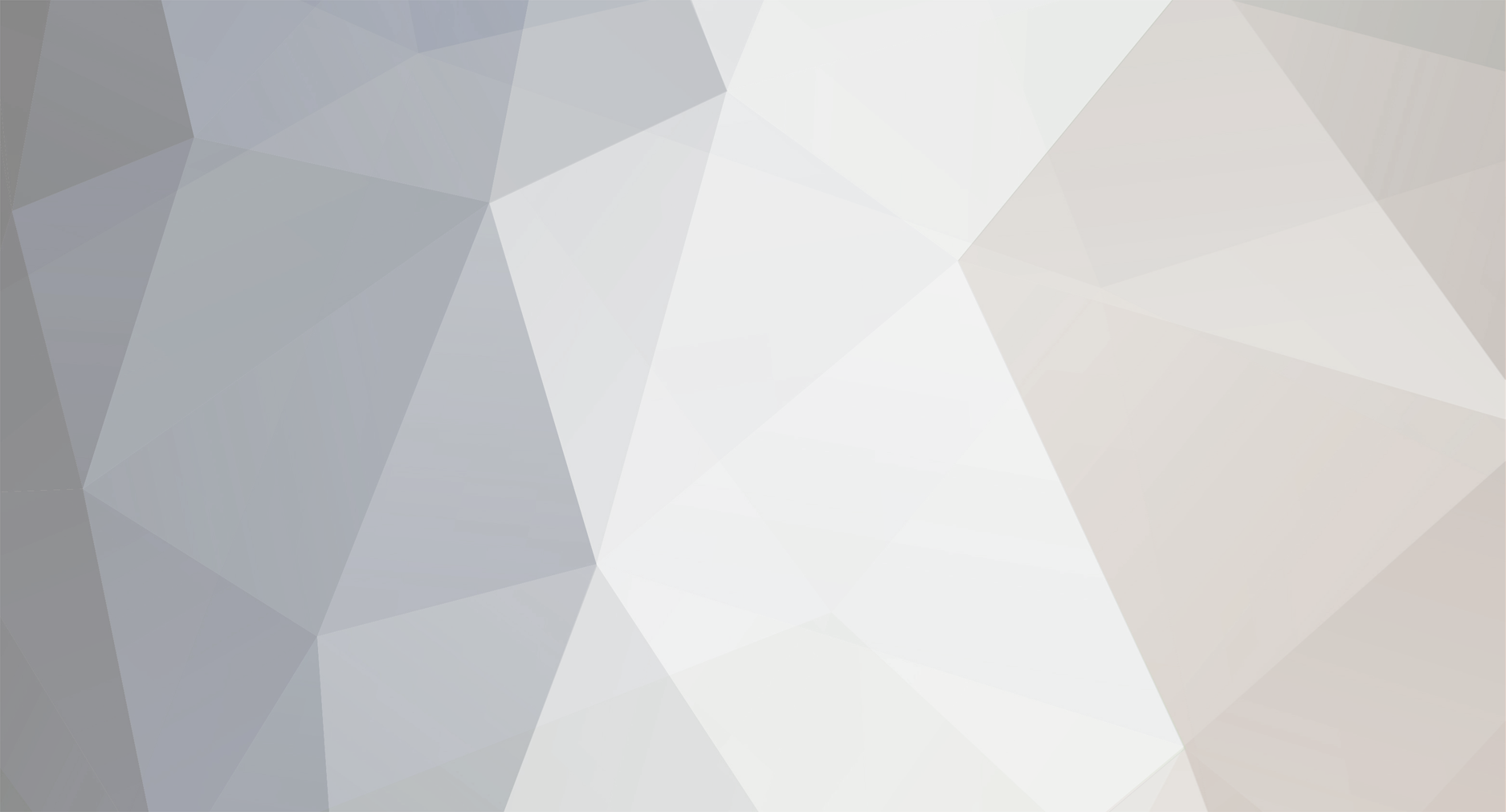
White_Lily
-
Posts
531 -
Joined
-
Last visited
-
Days Won
1
Posts posted by White_Lily
-
-
Here is some simple form and validation:
<html> <head> </head> <body> <?php //Variables holding the post values $submit = $_POST["submit"]; $username = mysql_real_escape_string($_POST["username"]); $password = mysql_real_escape_string($_POST["password"]); //Runs this if the form was submitted if($submit){ //checks if the form was empty or not if(!$username && !$password){ $msg = "The form was submitted empty."; }else{ //checks to make sure a username was entered if(!$username){ $msg .= "Please enter a username."; }else{ //checks to make sure a password was entered if(!$password){ $msg .= "Please enter a password."; }else{ $query = mysql_query("SELECT * FROM members");//Queries the database $num = mysql_num_rows($query);//Collects the rows (if any) $row = mysql_fetch_assoc($query);//a variable to grab rows with //checks if there are any registered members if($num == 0){ $msg .= "There are no members in the database, register first."; }else{ //if there are members this checks the entered username against those in the database if($row["username"] != $username){ $msg .= "The username does not match any registered members."; }else{ //if there are members this checks the entered password against those in the database if($row["password"] != $password){ $msg .= "The password does not match any registered members."; }else{ //if everything succeeds then the sessions will start session_start(); $_SESSION["username"] = $username; $_SESSION["password"] = $password; //re-directs the user to the home page. header("Location: index.php"); } } } } } } echo $msg; } ?> <form action="" method="POST"> <label>Username:</label> <input type="text" name="username" /> <label>Password:</label> <input type="password" name="password" /> <input type="submit" name="submit" value="Login" /> </form> </body> </html>
Remember: if the user has logged in you need to carry over the sessions to every single page on the website,
this is done at the very top of the source code (before the doctype):
<?php session_start(); $username = $_SESSION["username"]; $password = $_SESSION["password"]; ?>
-
Its actually quite simple with just a few if and else statements with the odd comparison to entries in a database.
-
Ive already seen the biggest problem... Your posting values without a form lol
-
Thats scary - knowing mods can just randomly edit our original posts lol
-
As for the directory being specified, you have done it slightly wrong - you are using xampp, this means that htdocs (to a browser) doesnt technically exist and is replaced with "http://localhost/siteName" to prove this, echo this out:
$filename = $_SERVER["DOCUMENT_ROOT"]; echo $filename;
This means this line:
$directory = 'C:\Users\test\Desktop\Main\xampp\htdocs\mp3';
Should be:
$directory = $_SERVER["DOCUMENT_ROOT"]."/mp3/";
-
Re-post the code with the coding tags please.
-
Please re-post your code in the [ code][/ code] tags, makes things so much easier.
-
If the table is being found in the database try echoing the rows:
$num = mysql_num_rows($issues); echo $num;
If it echos nothing (not even a 0) then your query is probably the problem, if it echos '0' then its saying you have no entries, anything higher and the problem is elsewhere.
-
you could just do str_replace();?
eg:
$string = $_POST["somethingRandom"]; $string = str_replace("<?php", "", $string); $string = str_replace("<?", "", $string); $string = str_replace("?>", "", $string); echo $string;
-
If they are all the same then why not delete two of them, then the default one would be whatevers left.
-
use these:
[ CODE]
CODE HERE
[ /CODE]
PS: Take out the spaces in the code tags
-
Sorted - PFMaBiSmAd & cyberRobot were correct, the first entry was being ignored didn't even relize i had called the same query twice lol
-
I echoed everything that should return any form of number(s) or word(s) and everything is correct... i don't quite understand why its not doing what ive asked it to do...
-
Like with the select function - the rest of the site has NO problem with the function.
-
I ehcoed $filename, the path(s) were correct,
$num echos "1" which is correct due to there only being 1 row in the database, if i use a while and echo $get["file_path"] and $get["page_name], they are also correct... no errors show on the screen.
-
Your not out of line - once ive finished developing it and its fully pre-live checked and post-live checked i will post a link
A CMS should be capable of development throughout its life-time, this means that you should be about to take away features and add features depending on your sites needs. This is the one reason why i hate pre-built CMS's such as WordPress, and Joomla. Very difficult to customise those. (sets my teeth on edge just mentioning them >_<)
-
i have a php.ini file that has display errors turned on and error reporting to E_ALL,
Yes select() is a custom funtion and is well with that as the rest of the site doesnt have a problem with it.
-
Hi,
I tried writing some code for my navigation menu to make it easier on my part (with creating the site etc).
I have written this so far:
<?php $nav = select("*", "navigation", NULL, NULL, NULL); $num = mysql_num_rows($nav); $get = mysql_fetch_assoc($nav); $filename = file_exists($_SERVER["DOCUMENT_ROOT"]."forum/".$get["file_path"]); if($num > 0){ while($get = mysql_fetch_assoc($nav)){ if($filename){ $path = $GLOBALS["siteUrl"].$get["file_path"]; echo '<li><a href="'.$path.'">'.$get["page_name"].'</a></li>'; } } }else{ echo "<li>Could not find a navigation menu.</li>"; } ?>
Problem is, even though there is an entry in the database (to a page that DOES exist) nothing shows in the navigation area, can't think why.
Any help would be appreciated.
-
If i remember rightly you can have different names for each row.
-
Either way, people clearly do it differently, I just find more easier to do it how I do it now.
-
Most still take backups whether they make changes directly or not.
Im told that if i make a change to a live preview or live site then i take a backup of the site and database before making the changes
-
Just quickly again, would this be a similar process for deleting multiple albums to? (the images associated with this album should also be deleted along with the album).
-
You dont need to be experienced, create a simple one with simple error checking and then just develop it over time.
-
could check to see if the field is empty?
The HTML:
<html> <form action="" method="POST" enctype="multipart/form-data"> <label>File Upload</label><input type="file" name="file" /> <input type="submit" name="submit" value="Upload" /> </form> </html>
The PHP:
<?php if(!$_POST["file"]){ echo "No file selected."; }else{ //processing code } ?>
Calling Php For Authentication
in PHP Coding Help
Posted
If its so bad jessica, post a better one.