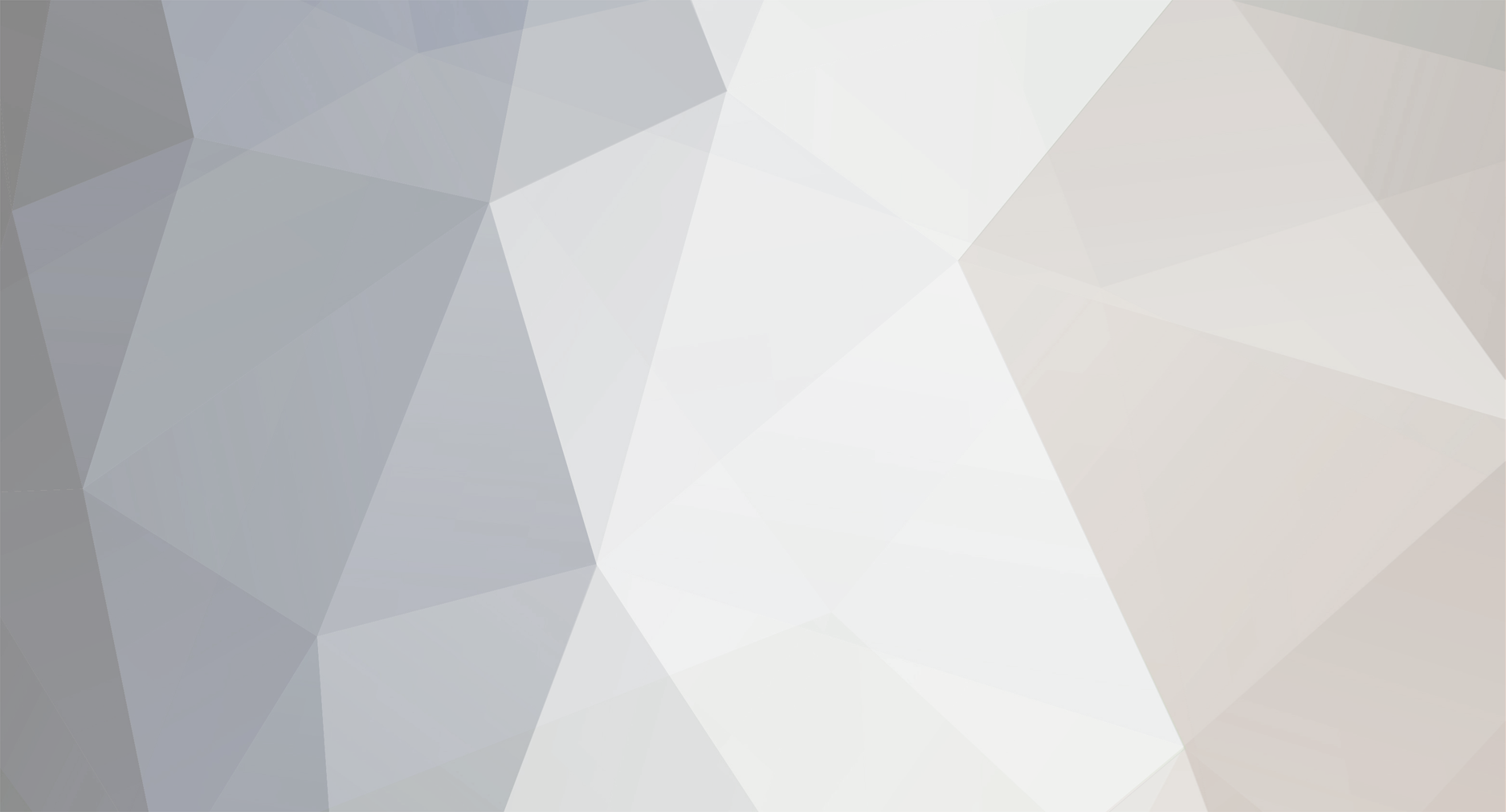
White_Lily
-
Posts
531 -
Joined
-
Last visited
-
Days Won
1
Posts posted by White_Lily
-
-
Its because the boxes are returning a false (or empty or 0) value.
Its the same as an undefined variable, it's because that variable is not storing any data.
-
<form action="http://www.institute-of-photography.com/moodle/login/index.php" id="voucherForm" method="POST" class="voucherForm">
Try that instead.
-
Error 404 only appears when the page in question does not exist, try making sure the link is spelt right and the page you want them to go to exists.
-
Done also. Used htmlspecialchars().
-
Done - I have escaped all the inputs with mysql_real_escape_string().
-
Okay lol. (Sorry for the code being long - I think they can be tidied up a bit but that will have to come later when there is some kind of function to the forum).
Registration Form:
<?php if($_POST["submitReg"]){ $regName = $_POST["name"]; $regUsername = $_POST["username"]; $regPassword = $_POST["password"]; $regREpassword = $_POST["rePassword"]; $email = $_POST["email"]; if(empty($regName) && empty($regUsername) && empty($regPassword) && empty($regREpassword)){ $msg3 = "The form was submitted empty."; }else{ if(empty($regName)){ $msg3 .= "The name field was empty."; }else{ if(empty($email)){ $msg3 .= "The email field was empty."; }else{ if(preg_match("^[a-zA-Z0-9_.-]+@[a-zA-Z0-9-]+.[a-zA-Z0-9-.]+$", $_POST["email"]) === 0){ $msg3 .= "Please enter a valid email."; }else{ if(empty($regUsername)){ $msg3 .= "The username field was empty."; }else{ if(empty($regPassword)){ $msg3 .= "The password field was empty."; }else{ if(empty($regREpassword)){ $msg3 .= "The repeat password field was empty."; }else{ if($regPassword != $regREpassword OR $regREpassword != $regPassword){ $msg3 .= "The password fields didn't match."; }else{ $check = select("*", "members", "username = '$regUsername'"); $assoc = mysql_fetch_assoc($check); if($regUsername == $assoc["username"]){ $msg3 .= "That username has been taken, pick another."; }else{ $regPassword = sha1($regPassword); $id = uniqid(); $register = insert("members", "name, email, username, password, user_level, id, ban", "'$regName', '$email', '$regUsername', '$regPassword', 1, '$id', 0"); if($register){ $newsTitle = "New member registered!"; $cont = $regUsername." has just joined Fusion Forums!<br>"; $cont.= "Check out his/her profile:<br><br>"; $cont.= "View Profile"; $newsCont = $cont; $newMem = insert("news", "news_title, news_content, username", "'$newsTitle', '$newsCont', '$regUsername'"); if($newMem){ $to = $email; $subject = "Fusion Forums - Account Confirmation"; $message = "Hello! You have recently registered to Fusion Forum's.<br><br>"; $message.= "This is a confirmation email, below you will find your account details along with a Unique ID.<br><br>"; $message.= "In order to activate your account you must first enter the ID into the text field that follows the link at the end of this email. Your details are as follows:<br><br>"; $message.= "<table>"; $message.= "<tr>"; $message.= "<td><strong>Name:</strong></td>"; $message.= "<td></td>"; $message.= "<td>".$regName."</td>"; $message.= "<tr>"; $message.= "<tr>"; $message.= "<td><strong>Email:</strong></td>"; $message.= "<td></td>"; $message.= "<td>".$email."</td>"; $message.= "<tr>"; $message.= "<tr>"; $message.= "<td><strong>Username:</strong></td>"; $message.= "<td></td>"; $message.= "<td>".$regUsername."</td>"; $message.= "<tr>"; $message.= "<tr>"; $message.= "<td><strong>Unique ID:</strong></td>"; $message.= "<td></td>"; $message.= "<td>".$id."</td>"; $message.= "<tr>"; $message.= "</table><br><br>"; $message.= "Please follow this link in order to activate your account (opens in your default browser):<br>"; $message.= "<a href='http://www.janedealsart.co.uk/activate.php?id=".$id."'>Activate Account</a>"; $from = "noreply@janedealsart.co.uk"; $headers = 'MIME-Version: 1.0' . "\r\n"; $headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n"; $headers.= "From: ".$from; mail($to, $subject, $message, $headers); $done = "You have successfully registered to Fusion Fourm's.<br>"; $done.= "We have sent you an email with a confirmation code on it,"; $done.= " when you go to confirm your account you will need this code in order to be able to access the forum's."; $msg2 .= $done; }else{ $msg3 .= "Sorry, we could not register your account details, if this persists contact the webmaster. "; } }else{ $msg3 .= "Sorry, we could not register your account details, if this persists contact the webmaster. "; } } } } } } } } } } } if($msg2){ echo '<div class="success">Success: '.$msg2.'</div>'; }else{ if($msg3){ echo '<div class="error">Error: '.$msg3.'</div>'; } echo '<form action="" method="POST">'; echo '<label>Full Name:</label>'; echo '<input type="text" class="field" name="name" />'; echo '<div class="clear"></div>'; echo '<label>Email:</label>'; echo '<input type="text" class="field" name="email" />'; echo '<div class="clear"></div>'; echo '<label>Username:</label>'; echo '<input type="text" class="field" name="username" />'; echo '<div class="clear"></div>'; echo '<label>Password:</label>'; echo '<input type="password" class="field" name="password" />'; echo '<div class="clear"></div>'; echo '<label>Again:</label>'; echo '<input type="password" class="field" name="rePassword" />'; echo '<div class="clear"></div>'; echo '<input type="submit" class="button" name="submitReg" value="Register" />'; echo '<div class="clear"></div>'; echo '</form>'; } ?>
The Login Form:
<?php //Variables holding the post values. $submit = $_POST["submit"]; $username = mysql_real_escape_string($_POST["username"]); $password = mysql_real_escape_string($_POST["password"]); //Runs this if the form was submitted. if($submit){ //Checks if the form was empty or not. if(empty($username) && empty($password)){ $msg = "The form was submitted empty."; }else{ //Checks to make sure a username was entered. if(empty($username)){ $msg .= "Please enter a username."; }else{ //Checks to make sure a password was entered. if(empty($password)){ $msg .= "Please enter a password."; }else{ $query = mysql_query("SELECT * FROM members WHERE username = '$username'");//Queries the database. $num = mysql_num_rows($query);//Collects the rows (if any). $row = mysql_fetch_assoc($query);//A variable to grab rows with. //If there are members this checks the entered username against those in the database. if($row["username"] != $username){ $msg .= "The username does not match any registered members."; }else{ //Turn the normal password into a sha1() encrypted password. $password = sha1($password); //If there are members this checks the entered password against those in the database. if($row["password"] != $password){ $msg .= "The password does not match any registered members."; }else{ if($row["id"] != "Confirmed"){ $msg .= "You cannot log in yet, please confirm your account.<br>"; $msg .= "Upon registration you were sent an email with a confirmation code, if you didnt recieve it, click below:<br><br>"; $msg .= "<a href='/resend.php'>Re-send Confirmation Email</a>"; }else{ if($row["ban"] == 1){ $msg .= "Your account has been banned for 24 hours, come back later."; }else{ if($row["ban"] == 2){ $msg .= "Your account has been banned for 14 days, come back later."; }else{ if($row["ban"] == 3){ $msg .= "Your account has been banned permanantly.<br>"; $msg .= "If you think this is wrong, you can submit an appeal for the admins and webmaster to decide whether to unban you or not.<br><br>"; $msg .= "<a href='appeal.php'>Submit an Appeal</a>"; }else{ if($row["ban"] == 0){ //If everything succeeds then the sessions will start. session_start(); $_SESSION["username"] = $username; $_SESSION["password"] = $password; //Re-directs the user to the home page. header("Location: profile.php"); } } } } } } } } } } //Echos errors, should there be any. echo '<div class="error">Error: '.$msg.'</div>'; } ?>
-
This may need to be a different query. But...
WHERE id >=100 AND id < 200 AND quantity > 0 ORDER BY id DESC');
-
You answered the question yourself as Maq so correctly stated lol.
-
What I'm not quite understanding is if the system worked using locations, why scrap it?
Also, you should probably plan the page before coding it so that you wouldn't have the problem of posting on a forum to help sort your code out.
-
Okay I have implemented the changes you had all suggested, and it works great! Thank you, any more suggestions on security (for either the registration form or the login form, forum template is in my signature) is also appreciated here
-
Your code would be a start.
-
That does actually lol
I will try to find a way to implement it into my script later, however I may not now be on for a few days. So I may be a while before posting results.
-
I don't even know what Objective-C is lol
-
Sorry John but that doesn't appear to help much.
I did however search google and found on php.net a function could "rand();" however I didn't see any way in which i could make it unique. Any ideas?
-
Okay - I can't seem to be able to get the hang on the md5 hash as a code for the confirmation. So, I thought - how difficult would it be to have some code that generates a random (preferrably unique) number that is then inserted into the database and used as that person's personal confirmation code?
-
With the excel spreadsheet try this set of search results:
Whether this helps I don't know - but it's a start.
-
Right! Okay, I will give it a go and post the results
-
that seems easy enough - however why would the email be put into md5?
-
Hi, I have a registration and i wish to improve the security somewhat.
I want to be able to have an email sent to the user that has registered which will consist of his/her;
Name
Username
Password???
and some form of Security Code
I was wondering how I should go about doing the security code, since the code will be stored in the database.
When the user clicks on a link in the email they would be directed to a page where they enter their username and security code, and the processing script would then compare them, and either allow access or deny it.
I was thinking something like sha1, md5, etc... Any help with this would be greatly appreciated
-
Solution:
<div class="news"> <div class="newsHeading">News</div> <?php $news = select("*", "news", NULL, "news_id DESC", "1"); $display = mysql_fetch_assoc($news); echo '<a href="news.php?articleID='.$display["news_id"].'"><h2>'.$display["news_title"].'</h2></a>'; echo '<p>'.$display["news_content"].'</p>'; ?> </div>
"id DESC" was supposed to be "news_id DESC" sorry for this >_<
-
Hi, I have a news box that whenever a person registers to the website, will update and you should see something like "New member has registered!" However, you do not see this.
Warning: mysql_fetch_assoc(): supplied argument is not a valid MySQL result resource in/home/sites/janedealsart.co.uk/public_html/index.phpon line 61.
Here id the code for the news box:
<div class="news"> <div class="newsHeading">News</div> <?php $news = select("*", "news", NULL, "id DESC", "1"); $display = mysql_fetch_assoc($news); echo '<a href="news.php?articleID='.$display["news_id"].'"><h2>'.$display["news_title"].'</h2></a>'; echo '<p>'.$display["news_content"].'</p>'; ?> </div>
Here is the custom select() function:
//Select function select($row = "*", $table, $where = NULL, $order = NULL, $limit = NULL) { $query = "SELECT ".$row." FROM ".$table; if($where != NULL){ $query .= " WHERE ".$where; }if($order != NULL){ $query .= " ORDER BY ".$order; }if($limit != NULL){ $query .= " LIMIT ".$limit; } $result = mysql_query($query); return $result; }
mysql_fetch_assoc() has a valid variable in it, and the select function is fine since the rest of the site is able to get data without problems. I can't see why this is happening! :/
Any help would be appreciated.
-
You don't always have to use the primary key column (but you can if you want to).
-
Just a little to add, the errors say "on line 23." This does not actually mean that the error is on that line. The error could of been caused anywhere above or below that line, however sometimes it is the specified line - maybe in future try posting the pages code, or the block associated with the errored line.
-
Ah that! Yeah I know about that sorry. If there are any id's (or classes for that matter) then it's because we are using TinyMCE to display text fields etc.
Add Data To Specific Database Table Column
in PHP Coding Help
Posted · Edited by White_Lily
If I'm reading it correctly, try using update rather than insert.
Update, updates (obviously) existing entries, whereas insert creates a new row for each entry.
Also, since functions such as select, insert, update, delete, etc are very common functions to use in php - can I suggest that you write custom functions for them?
Selecting:
Calling the function: