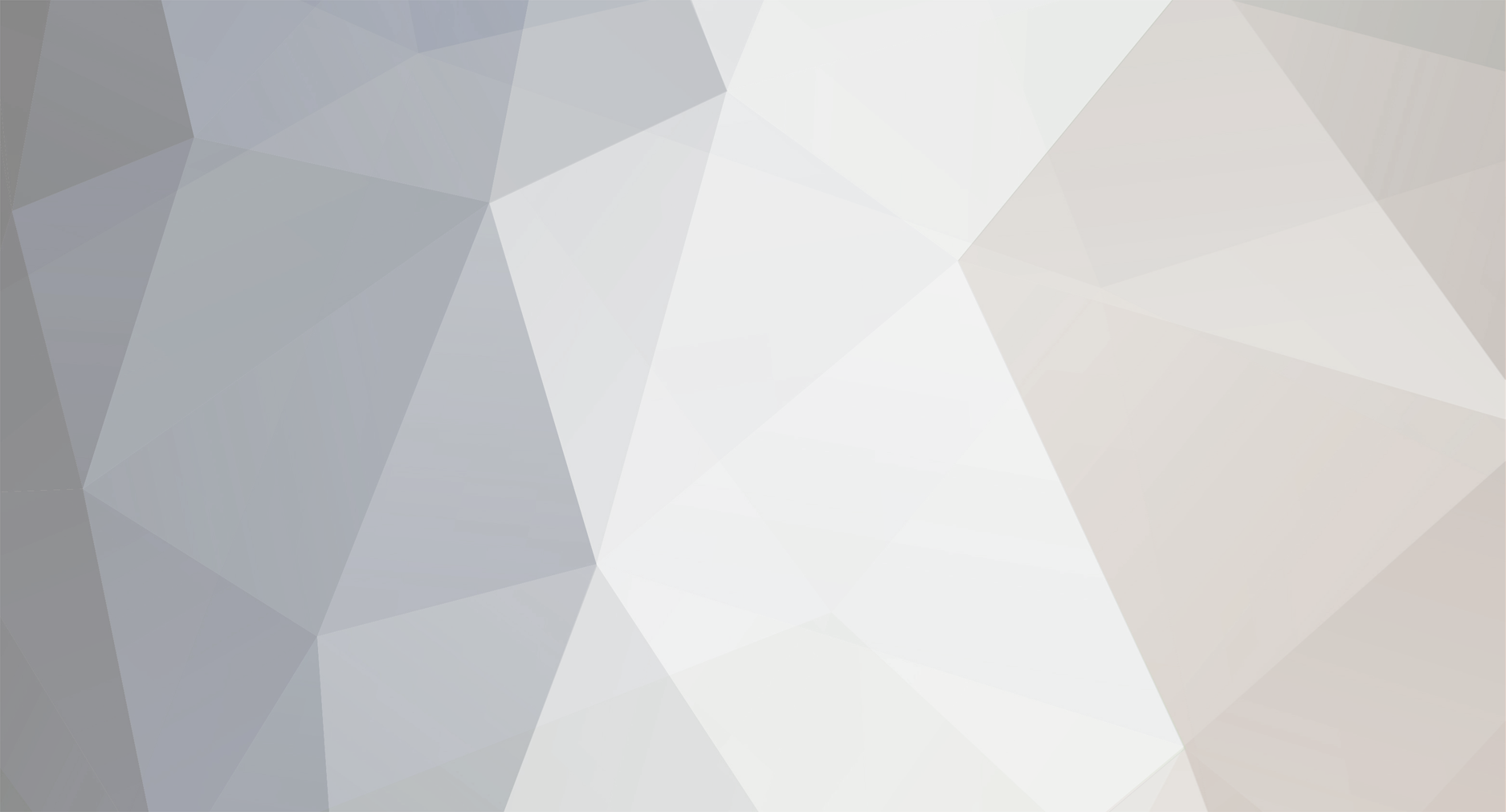
ToonMariner
Members-
Posts
3,342 -
Joined
-
Last visited
Everything posted by ToonMariner
-
<?php $sql = sprintf("SELECT * FROM test ORDER BY RAND() LIMIT 0,10"); $rs = mysql_query($sql); $i=1; while ($row = mysql_fetch_array ($rs)) { echo ("Question " . $i++ .": " . $row["question"] ."<br><br>"); echo ("A: " . $row["answer1"] ."<br>"); echo ("B: " . $row["answer2"] ."<br>"); echo ("C: " . $row["answer3"] ."<br>"); echo ("D: " . $row["answer4"] ."<br>"); } ?> querying in a loop is inefficient and you have to check that the random generated has not already been used - let the database do all that for you.
-
you are not populating your arrays as you think you are... simply pushing an array onto an array creates an element containing an array of the indices you are interested in - making it harder for you to access those elements. use print_r to output the two arrays you build and you should see what I mean. In the mean time try this... <?php // these affiliations need to populate the attr_list table NOT the breed table. $dbc = mysqli_connect(DB_HOST, DB_USER, DB_PASSWORD, DB_NAME) or die('ERROR connecting to database'); $affiliationsQuery = "SELECT * FROM affiliations"; $affiliationsData = mysqli_query($dbc, $affiliationsQuery) or die (mysqli_error($dbc)); $affiliation = array(); while($row10 = mysqli_fetch_array($affiliationsData)){ foreach($row10 as $key => $val){ $affiliation[$key][] = $val; } } $affQuery = "SELECT affiliation_id FROM aff_list WHERE breed_id = '$breedId'"; $data2 = mysqli_query($dbc, $affQuery); $aff_list = array(); while($row2 = mysqli_fetch_array($data2)){ $aff_list[] = $row2['affiliation_id']; } echo '<fieldset><table><tr>'; foreach($affiliation['affiliation_id'] as $key => $val){ $checked = (array_search($val, $aff_list) ? ' checked="checked"' : ''); printf('<td><input type="checkbox" value="%1$d" name="affiliations[]"%3$s/>%2$d</td>',$val,$affiliation['affiliation_name'][$key],$checked); } echo '</tr></table></fieldset>'; mysqli_close($dbc); ?>
-
it DOES make a difference - check your operator precedence. The concatenation operator takes precedence over mathematical ones so if you want any computation within a concatenated string it must be contained within parentheses.
-
You can get around that issue but it involves tricking other machines than just you and the service in question - and this is much skull-duggery and, I'm sure, illegal at some juncture.
-
You have an array of affiliate IDs and an array of affiliate IDs associated with a breed. Loop through the affiliate IDs and for each one check if that ID is present in the array of IDs associated with a breed. <?php foreach($affiliation as $aff) { $checked = array_search($aff['affiliation_id'], $aff_list) ? ' checked="checked"' : NULL; echo '<td><input type="checkbox" value="' . $aff['affiliation_id'] . '" name="affiliations[]"' . $checked . ' />' . $aff['affiliation'] . '</td>'; } ?>
-
sc00tz - go read those articles - try some code and come back when you need some help. Making mistakes is one of the best ways of learning...
-
its called spoofing - if you google for 'spoof my ip address' I am certain you will get some info. note some ISPs don't like you doing this. You could look at cycling through a few proxies but if what you are trying to do is monitored by some web service and will be blocked on detection there a reason for it; along the lines of 'don't do that - we don't like it'...
-
http://www.sitepoint.com/article/php-amp-mysql-1-installation/ there's a place to start (you can ignore the 1st part in the series if you have everything installed.
-
what if javascript is off??? just before your check for the value of affiliate echo out its value foreach($affiliation as $aff){ echo $row2['affiliation_id']; if($row2['affiliation_id'] == 2){ echo '<td><input type="checkbox" value="' . $aff['affiliation_id'] . '" name="affiliations[]" checked="checked" />' . $aff['affiliation'] . '</td>'; } else{ echo '<td><input type="checkbox" value="' . $aff['affiliation_id'] . '" name="affiliations[]" />' . $aff['affiliation'] . '</td>'; } } obviously stick it inside some tags so it appears in the expected place...
-
@danny - the method does not require 2 queries to complete the task. The database can report back on any error all by itself if you have the correct structure. set the filed in the database so it must be unique. ALTER TABLE `mymembers` ADD UNIQUE (`email`) in your code... $query_users = "INSERT INTO `mymembers` (`email`, `password`) VALUES ('email', SHA1('password')"; $users = mysql_query($query_users) or die(mysql_error()); if you attempt to insert somet value already there it will kill the script. If you want to catch that error then have a look at this page which tells you what that function returns and what you can do with it. (you could just echo out the error and remove the die so that the user could enter a different email address)
-
2 methods. 1 query the database for the username - if its not taken do the insert. 2 (preferred method) set the username field to be unique try the insert if the query fails check the error - it should say filed must be unique. the benefit of the latter is only one query is run.
-
have a look at the native functionality for doing such things http://uk2.php.net/manual/en/refs.xml.php
-
after writing to the file close it as mark suggests but also clear the status cache (clearstatcache())
-
OOP is one of those things you learn more about by doing. I would suggest you have a read of some texts on OOP and design patterns to see where real world comes in to play. http://www.amazon.com/Design-Patterns-Elements-Reusable-Object-Oriented/dp/0201633612 is apparently a ubiquitous text. I would also recommend http://www.amazon.com/PHP-Architects-Guide-Design-Patterns/dp/0973589825 - old book but I found it useful. As you progress you will find more resources and I'm sure others will contribute valuable resources if asked. Good luck with it fella - once you get into OOP you won't look back.
-
OOP Question -> Object to contain a collection of objects
ToonMariner replied to enoch's topic in PHP Coding Help
-
OOP Question -> Object to contain a collection of objects
ToonMariner replied to enoch's topic in PHP Coding Help
not quite sure why you need the bookshelf class - perhaps the book object itself would benefit from having a shelfmark member and status (whether its in lib or not). Just appears to me that would provide with a simpler solution... -
[SOLVED] What's the best way to achieve this?
ToonMariner replied to Bricktop's topic in PHP Coding Help
don't pass the name through the url - pass the index key - numeric much easier to deal with. -
eval is a very expensive way of going about things - I can't really think of a situation where I would use it on purpose. I'd always look to avoid it if possible...
-
[SOLVED] What's the best way to achieve this?
ToonMariner replied to Bricktop's topic in PHP Coding Help
The structure of your array makes it quite difficult to work with... You see that each index of your array contains further indices of name, username and password. This makes searching that array much more difficult... Create the array so its structure looks like this. (is there any other way of doing this instead of putting the username and password details in?) <?php $clients = array('names' => array('john', frank, 'bob'), 'username' => 'john1', 'frank2', 'bob3'), 'password' => 'pass1', 'pass2', 'pass3'); ?> Now all you need do is loop through the child arrays. <select name="clientid" name="clientid"> <?php foreach($clients['names'] as $key => $val) { ?> <option value="<?php echo $key; ?>"><?php echo $val; ?>/option> <?php } ?> </select> Now in the processing script you can easily retrieve which user by the key that has been passed. <?php function backUp($id) { global $clients; $name = $clients['name'][$id]; $username = $clients['username'][$id]; $password = $clients['password '][$id]; // .. do your stuff.... } $clientid = $_POST['clientid']; // do some sanitization just incase! - like check its a number. backUp($clientid); ?> There are several other (perhaps preferable) ways of achieving this and perhaps you should have a think about your application and see if there is scope improve implementation. -
you could decorate the classes as there is a variable number of players in a game - subs etc. could also include match officials in that too!
-
thank you - could not see woods for trees!!!
-
Need regex that allows digits '-' and ' ' but must have at least 1 digit... (?!^[0-9]*$)^([0-9\ -]+)$ is where I'm at now but its just not working... [0-9]+ and [\ \-]? but NOTHING else cheers peeps
-
somewhere in your code you are using 'test' - make sure you have updated all your files on your server/local dev so they are using what you think they should be using. if you get an error it should tell you which file and line number where it exists...