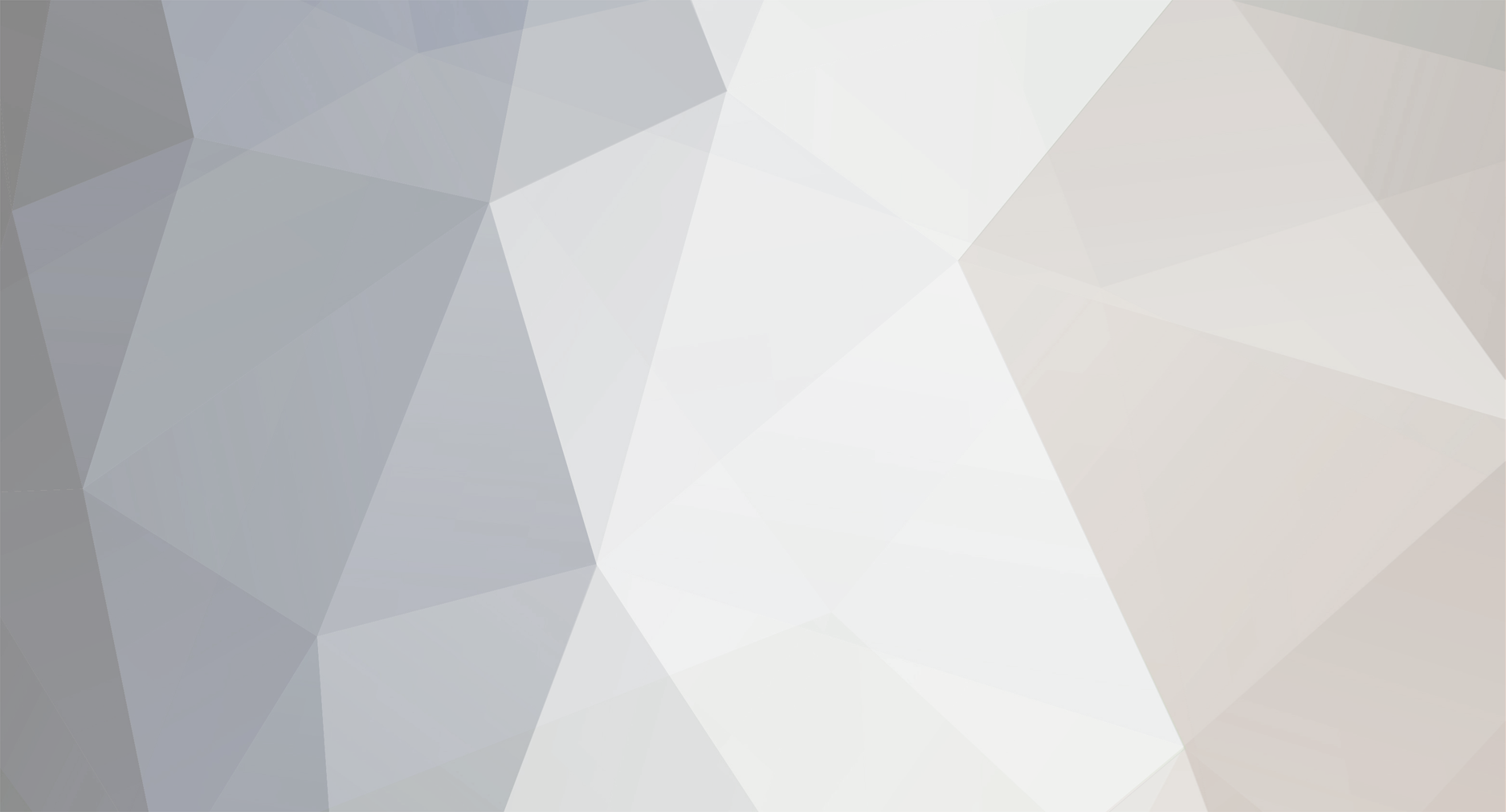
cobusbo
-
Posts
224 -
Joined
-
Last visited
Posts posted by cobusbo
-
-
Hi I'm currently struggling with a problem with my string
Lets say for example I got a sentence like
"This is my shoes"
and I want to check for the word shoes in that string and if it is within the string I want it to replace the whole string with another string
"That was your shoes"
is there a way to do this?
I tried preg_replace but it only replace the word shoes not the whole string.
-
Yes, I tried that but it didn't work, so I tried $batches and forgot to change it when I posted it here. The problem is still there.
I've been looking at an example script that was made to work with MongoDB, but its difficult to change it into php and mysql version. I'm going to post it below and maybe you guys can tell me what I missed in my attempt to create the Myqsl version.
<?php /* Require the PHP wrapper for the Mxit API */ require_once ('MxitAPI.php'); /* Function to count the number of users in MongoDB */ function count_users() { $mongo = new Mongo('127.0.0.1'); $collection = $mongo->sampleapp->users; $collection->ensureIndex(array('uid' => 1)); return $collection->count(); } /* Function to get batches of users from MongoDB */ function get_users($skip=0, $limit=50) { $mongo = new Mongo('127.0.0.1'); $collection = $mongo->sampleapp->users; $collection->ensureIndex(array('mxitid' => 1, 'created_at' => 1)); $users = $collection->find()->sort(array('created_at' => 1))->skip($skip)->limit($limit); return iterator_to_array($users); } /* Instantiate the Mxit API */ $api = new MxitAPI($key, $secret); /* Set up the message */ $message = "(\*) Congratulations to our winners (\*)\n\n"; $message .= "1st place - Michael with 100 points\n"; $message .= "2nd place - Sipho with 50 points\n"; $message .= "3nd place - Carla with 25 points\n\n"; $message .= 'Good Luck! $Click here$'; /* Mxit Markup is included in the message, so set ContainsMarkup to true */ $contains_markup = 'true'; /* Count the number of users in the database */ $count = count_users(); /* Initialise the variable that counts how many messages have been sent */ $sent = 0; /* Keep looping through the user list, until the number of messages sent equals the number of users */ while ($sent < $count) { /* Get users in batches of 50 */ $users = get_users($sent, 50); /* The list where the user MxitIDs will be stored */ $list = array(); foreach ($users as $user) { $list[] = $user['mxitid']; $sent++; } /* If there is a problem getting an access token, retry */ $access_token = NULL; while (is_null($access_token)) { /* We are sending a message so request access to the message/send scope */ $api->get_app_token('message/send'); $token = $api->get_token(); $access_token = $token['access_token']; // Only attempt to send a message if we have a valid auth token if (!is_null($access_token)) { $users = implode(',', $list); echo "\n$sent: $users\n"; $api->send_message($app, $users, $message, $contains_markup); } } } echo "\n\nBroadcast to $sent users\n\n";
Well I found the solution
foreach ($batches as $batch) { $list = join(',', $batch); // process the batch list here $api->send_message($app, $list, $message1, 'true'); }
Should be implode
foreach ($batches as $batch) { $list = implode(',', $batch); // process the batch list here $api->send_message($app, $list, $message1, 'true'); }
-
jcbones,
check out array_chunk
cobusbo,
Shouldn't you be sending $list in the send__message and not $batches
Yes, I tried that but it didn't work, so I tried $batches and forgot to change it when I posted it here. The problem is still there.
I've been looking at an example script that was made to work with MongoDB, but its difficult to change it into php and mysql version. I'm going to post it below and maybe you guys can tell me what I missed in my attempt to create the Myqsl version.
<?php /* Require the PHP wrapper for the Mxit API */ require_once ('MxitAPI.php'); /* Function to count the number of users in MongoDB */ function count_users() { $mongo = new Mongo('127.0.0.1'); $collection = $mongo->sampleapp->users; $collection->ensureIndex(array('uid' => 1)); return $collection->count(); } /* Function to get batches of users from MongoDB */ function get_users($skip=0, $limit=50) { $mongo = new Mongo('127.0.0.1'); $collection = $mongo->sampleapp->users; $collection->ensureIndex(array('mxitid' => 1, 'created_at' => 1)); $users = $collection->find()->sort(array('created_at' => 1))->skip($skip)->limit($limit); return iterator_to_array($users); } /* Instantiate the Mxit API */ $api = new MxitAPI($key, $secret); /* Set up the message */ $message = "(\*) Congratulations to our winners (\*)\n\n"; $message .= "1st place - Michael with 100 points\n"; $message .= "2nd place - Sipho with 50 points\n"; $message .= "3nd place - Carla with 25 points\n\n"; $message .= 'Good Luck! $Click here$'; /* Mxit Markup is included in the message, so set ContainsMarkup to true */ $contains_markup = 'true'; /* Count the number of users in the database */ $count = count_users(); /* Initialise the variable that counts how many messages have been sent */ $sent = 0; /* Keep looping through the user list, until the number of messages sent equals the number of users */ while ($sent < $count) { /* Get users in batches of 50 */ $users = get_users($sent, 50); /* The list where the user MxitIDs will be stored */ $list = array(); foreach ($users as $user) { $list[] = $user['mxitid']; $sent++; } /* If there is a problem getting an access token, retry */ $access_token = NULL; while (is_null($access_token)) { /* We are sending a message so request access to the message/send scope */ $api->get_app_token('message/send'); $token = $api->get_token(); $access_token = $token['access_token']; // Only attempt to send a message if we have a valid auth token if (!is_null($access_token)) { $users = implode(',', $list); echo "\n$sent: $users\n"; $api->send_message($app, $users, $message, $contains_markup); } } } echo "\n\nBroadcast to $sent users\n\n";
-
Any help please?
-
The first thing that crosses my mind when you say that you want to put a database column into an array is, "You are storing your data wrong".
That being said, $batch would never be an array, since it would be sent as a string from the database, so you cannot join it.
Well here is my DB layout
-- phpMyAdmin SQL Dump -- version 3.5.2.2 -- http://www.phpmyadmin.net -- -- Host: localhost -- Generation Time: Feb 28, 2015 at 09:13 PM -- Server version: 10.0.11-MariaDB -- PHP Version: 5.2.17 SET SQL_MODE="NO_AUTO_VALUE_ON_ZERO"; SET time_zone = "+00:00"; /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8 */; -- -- Database: `u342037492_chat` -- -- -------------------------------------------------------- -- -- Table structure for table `broadcast` -- CREATE TABLE IF NOT EXISTS `broadcast` ( `ID` int(11) NOT NULL AUTO_INCREMENT, `mxitid` varchar(30) COLLATE utf8_unicode_ci NOT NULL, `onorof` varchar(100) COLLATE utf8_unicode_ci NOT NULL, PRIMARY KEY (`ID`) ) ENGINE=MyISAM DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci AUTO_INCREMENT=2 ; -- -- Dumping data for table `broadcast` -- INSERT INTO `broadcast` (`ID`, `mxitid`, `onorof`) VALUES (1, '27765238453', '27765238453'); /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
I basically want to make batches before making an array just like this code below but from my mysql database not text file
$visitor = 'http://guniverse.hol.es/unique.csv'; $f_pointer=fopen($visitor,"r"); // file pointer $users = array(); while ($ar=fgetcsv($f_pointer)) { if ($ar[0] != '') $users[] = $ar[0]; //only store line in new array if it contains something } fclose ($f_pointer); $batchSize = 50; // set size of your batches $batches = array_chunk($users, $batchSize); require_once ('MxitAPI.php'); /* Instantiate the Mxit API */ $key = '50709335604c4feeaf9009b6e5f024a1'; $secret = '45a7b65b216d4f638a3cf4fc4f4e802f'; $app = 'guniverse'; $nick = urldecode($_SERVER['HTTP_X_MXIT_NICK']); if(!isset($nick)) { $nick = "Debater"; } $message = $_POST["message"]; $message1 = "*" . $nick . "*" . ": " . $message; $api = new MxitAPI($key, $secret); $api->get_app_token('message/send'); foreach ($batches as $batch) { $list = join(',', $batch); // process the batch list here $api->send_message($app, $list, $message1, 'true'); }
-
Hi I'm trying to recall a column from my table and insert it into an array. and then use the array and devide it into batches. Here is the code I got so far.
$query = "SELECT * FROM broadcast"; $result1 = mysql_query($query) or die(mysql_error()); $users = array(); while($row = mysql_fetch_array($result1)){ $users[] = $row['onorof']; } $batchSize = 50; // set size of your batches $batches = array_chunk($users, $batchSize); require_once ('MxitAPI.php'); /* Instantiate the Mxit API */ $key = '50709335604c4feeaf9009b6e5f024a1'; $secret = '45a7b65b216d4f638a3cf4fc4f4e802f'; $app = 'guniverse'; $nick = urldecode($_SERVER['HTTP_X_MXIT_NICK']); if(!isset($nick)) { $nick = "Debater"; } $message = $_POST["message"]; $message1 = "*" . $nick . "*" . ": " . $message; $api = new MxitAPI($key, $secret); $api->get_app_token('message/send'); foreach ($batches as $batch) { $list = join(',', $batch); // process the batch list here $api->send_message($app, $batches, $message1, 'true'); }
-
I assume HTTP_X_MXIT_USERID_R contains the users username? If so then try doing a simple str_replace
$raw = file_get_contents($source) or die("Cannot read file"); $raw = str_replace("$mxituid\n", "", $raw); file_put_contents($source, $raw);
I see no need for regex for removing a user from the file.
Yes it recall the username - and still the username is found inside the unique.csv file. Selecting the option to remove it have no effect. What I'm basicly trying to achieve is to give users the opportunity to add and remove their usernames from my unique.csv Because part 4 of my script will be using the unique.csv file to keep sending pop up messages to the people on the unique.csv file. So I'm not sure what I'm doing wrong in my script because the broadcasting option aint working neither.
-
Hi I've created a scricpt thats devided into 4 parts
Part 1
Inserting info into a text file
Part 2
Removing info from the text file
Part 3
Lookup the value stored within the text file if it match show form if not don't show form
Part 4
Broadcasting the message
I'm currently experiencing a problem with Part 2. Every time I click the button it doesn't remove the info needed after a few clicks the whole unique.csv file gets removed
<html> <head> </head> <body> Welcome To The Chat! To enter please Click <a href=\?func=yes>HERE</a> To exit please Click <a href=\?func=no>HERE</a> <? //Part 1 if($_GET['func']=='yes') { $myfile = fopen("users.csv", "a+") or die("Unable to open file!"); $mxituid = $_SERVER["HTTP_X_MXIT_USERID_R"]; if(!isset($mxituid)) { $mxituid = "Debater"; } $txt = "$mxituid\n"; fwrite($myfile, $txt); fclose($myfile); $list = file('users.csv'); $list = array_unique($list); file_put_contents('unique.csv', implode('', $list)); header("Location: index.php"); exit; } //Part 2 if($_GET['func']=='no') { $mxituid = $_SERVER["HTTP_X_MXIT_USERID_R"]; $source = "unique.csv"; $raw = file_get_contents($source) or die("Cannot read file"); $wordlist = $mxituid; $raw = preg_replace($wordlist, "", $raw); file_put_contents($source, $raw); header("Location: index.php"); exit; } //Part 3 $mxituid = $_SERVER["HTTP_X_MXIT_USERID_R"]; $file = 'unique.csv'; $searchfor = $mxituid; // get the file contents, assuming the file to be readable (and exist) $contents = file_get_contents($file); // escape special characters in the query $pattern = preg_quote($searchfor, '/'); // finalise the regular expression, matching the whole line $pattern = "/^.*$pattern.*\$/m"; // search, and store all matching occurences in $matches if(preg_match_all($pattern, $contents, $matches)){ print "Type in your message<br>"; print <<<HERE <form method="post" action="index.php"> <input type = "text" name = "message" value = ""> <input type="submit" value="Guess"> </form> HERE; } else{ echo "You should Enter Chat first!"; } //Part 4 $visitor = 'unique.csv'; $f_pointer=fopen($visitor,"r"); // file pointer $users = array(); while ($ar=fgetcsv($f_pointer)) { if ($ar[0] != '') $users[] = $ar[0]; //only store line in new array if it contains something } fclose ($f_pointer); $batchSize = 50; // set size of your batches $batches = array_chunk($users, $batchSize); require_once ('MxitAPI.php'); /* Instantiate the Mxit API */ $key = '50709335604c4feeaf9009b6e5f0424a1'; $secret = '45a7b65b216d4f638a3cf4fc84f4e802f'; $app = 'guniver3se'; $message = $_GET["message"]; $api = new MxitAPI($key, $secret); $api->get_app_token('message/send'); foreach ($batches as $batch) { $list = join(',', $batch); // process the batch list here $api->send_message($app, $list, $message, 'true'); } echo 'Success<br>'; ?> </body> </html>
-
Hi I'm currently having a problem with concatenation. My fields is as follow
Leerder ID - Van - Eerste Voornaam - Datum
120 - Botha - Peter - 15/02/2015
120 - Botha - Peter - 16/02/2015
121 - Jacobs - John - 17/02/2015
I want it to show as
Leerder ID - Van - Eerste Voornaam - Datum
120 - Botha - Peter - 15/02/2015 & 16/02/2015
121 - Jacobs - John - 17/02/2015
My current SQL code is as follow
SELECT [Leerder Afwesigheid].[Leerder ID], Students.Van, Students.[Eerste Voornaam], Count([Leerder Afwesigheid].Datum) AS CountOfDatum FROM Students RIGHT JOIN [Leerder Afwesigheid] ON Students.ID = [Leerder Afwesigheid].[Leerder ID] GROUP BY [Leerder Afwesigheid].[Leerder ID], Students.Van, Students.[Eerste Voornaam] HAVING (((Count([Leerder Afwesigheid].Datum))>=5));
How can I change it to add another field Datums Afwesig with the concatenated info?
-
Most chat applications that I've seen don't sent HTML back. They send JSON (which is a lot more compact), and then the javascript builds the HTML based on the values from the JSON data and inserts it into the DOM. The timestamp can easily be a field in the JSON data.
the problem is I must do it without javascript the since its not supported on the platform im using
-
Hi I'm currently having a problem with my form. Users submit an empty field into the database and the next time another user tries to enter it just says username has been taken. I need some help on how to confirm that the username and email field isnt empty when inserted and that the email address is in the correct format.
<?php include "base.php"; ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>User Management System (Tom Cameron for NetTuts)</title> <link rel="stylesheet" href="style.css" type="text/css" /> </head> <body> <div id="main"> <?php $username = mysql_real_escape_string($_POST["username"]); $email = mysql_real_escape_string($_POST["email"]); $mxitid = mysql_real_escape_string($_SERVER["HTTP_X_MXIT_USERID_R"]); if(!isset($mxitid)) { $mxitid = "DEFAULT"; } $checkusername = mysql_query("SELECT * FROM Users WHERE mxitid = '".$mxitid."'"); $checkemail = mysql_query("SELECT * FROM Users WHERE email = '".$email."'"); if(mysql_num_rows($checkusername) == 1) { echo "<h1>Error</h1>"; echo "<p>Sorry, that username is taken. Please go <a href=\"register.php\">back</a>and try again.</p>"; } elseif(mysql_num_rows($checkemail) == 1) { echo "<h1>Error</h1>"; echo "<p>Sorry, that email is taken. Please go <a href=\"register.php\">back</a>and try again.</p>"; } elseif ($_POST["register"]) { $username = mysql_real_escape_string($_POST["username"]); if(!isset($mxitid)) $mxitid = mysql_real_escape_string($_SERVER["HTTP_X_MXIT_USERID_R"]); if(!isset($mxitid)) { $mxitid = "DEFAULT"; } $registerquery = mysql_query("INSERT INTO Users (Username,mxitid,email) VALUES('".$username."','".$mxitid."','".$email."')"); if($registerquery) { echo "<h1>Success</h1>"; echo "<p>Your account was successfully created. Please <a href=\"index9.php\">click here to start chatting</a>.</p>"; } } else { ?> <h1>Register</h1> <p>Please enter your details below to register.</p> <p>Keep it clean! or you might get banned!</p> <form method="post" action="register.php" name="registerform" id="registerform"> <fieldset> <label for="username">Username:<br> Using emoticons in your name won't work!</label><input type="text" name="username" id="username" /><br /> <label for="email">Email:<br>(We need your real one to be able to contact you!)<br> If you don't have one make use of your mxit email service.</label><input type="email" name="email" id="email" /><br /> <input type="hidden" name="mxitid" id="mxitid" value="<? $_SERVER['HTTP_X_MXIT_USERID_R']; ?>"/><br /> <input type="submit" name="register" id="register" value="Register" /> </fieldset> </form> <?php } ?> </div> </body> </html>
I tried using
function check_input($data, $problem='') { $data = trim($data); $data = stripslashes($data); $data = htmlspecialchars($data); if ($problem && strlen($data) == 0) { die($problem); } return $data; }
but it didnt seems to work
-
You will need to output your results using some html and css to do your highlighting. From what you wrote I'm guessing you are just echo'ing some query results. Try putting them into an html table or in an html list and use css to produce the highlighting.
echo "<table>"; while($row = $pdo->fetch()) { if ($row['datefld'] > $highlight_date) $classname = 'hilight'; else $classname = 'normal'; echo "<tr class='$classname'><td>{$row['field1']}</td><td>{$row['field2']}</td><td>{$row['field3']}</td></tr>"; } echo "</table>";
You would need to set the value of the $highlight_date field based on something and write a couple of css classes to achieve your highlight or normal effects.the problem is the timestamp since last reload how would I be able to get that timestamp to compare it against the new post when I press reload since I only got timestamps of when the message was inserted into the db
-
It works anyway you want it to. How is the refresh done? By the user clicking something that triggers a POST? You can put something on the page to mark the last shown record and then highlight ones that succeed that on your next page build. You can use a datetime value for this, or a record id value.
It's really not an exact science - it's just some algorithm that you yourself make up and follow. The highlight is the easy part using css. It's the recognizing that you will have to work out for yourself.
I'm currently printing the values from the database and when I click reload it just a hyperlink to the same page to reload it
-
Hi I'm currently wondering how people check for latest records each time they refresh only the latest records display in another color. For example a chat. When people submit post and another user refresh their chat page the newest post always get highlighted. Does it work with sessions or how does it work?
-
echo urldecode("Galaxy+Universe+Admin"); #--> Galaxy Universe Admin
Thank you it helped removing the + signs and by adding
<html> <head> <meta charset="utf-8"><!-- Your HTML file can still use UTF-8--> </head> <body> <?php header('Content-Type: text/html; charset=ISO-8859-1');
helped with the special characters.
-
Hi I'm currently experiencing problems with my super global outputs.
I'm using
$_SERVER['HTTP_X_MXIT_NICK'];to retrieve info but in the place of a space it gets replaced with a +
Galaxy+Universe+Admin
I've also tried using special characters like this in my name
ᄃӨBЦƧBӨ {~KΛMΛKΛZI~}
But the output is
%E1%84%83%D3%A8B%D0%A6%C6%A7B%D3%A8+%7B~K%CE%9BM%CE%9BK%CE%9BZI~%7D
Is there maybe a way to clean it up to show as normal text in Sentence case?
-
Make the $_SERVER["HTTP_X_MXIT_USERID_R"] value part of a unique key in your table then it becomes impossible to write a duplicate.
EG> UNIQUE KEY (poll_id, user_id)
The reason why I'm asking is the script posted in my first message works with plain text documents and doesnt require a database. I'm not familiar how to do that without using a database. I want to keep it in .txt files
-
Do you realize that many people can (and will) be using the same IP when behind a NAT? Any home (and many businesses) use a router with, NAT, that connects through the ISP and has one external IP address. Then, internally, all the connected devices have local IP addresses. All requests go through the router using the external IP address. When the responses come back, the router determines which internal IP to direct the response to.
So, a web application will only see the external IP address of all the machines behind that router. There is really no perfect way to prevent people from voting multiple times. You could use a cookie as a first-line of defense (of course it can be deleted by the user). Then, if you really want to make it difficult, require that users are registered and authenticate before they can vote. Then only allowed users to vote once. Of course, they could create multiple accounts, but you would verify the email address and it would be a major PITA for users to vote multiple times at that point.
As said, there really isn't a perfect answer. In general though, IP alone is not an ideal solution. Entire companies, schools, libraries, etc.. may share the same external address.
In most cases i've just used cookies for small scripts. Unless the user had to be logged in to vote and from there you can use other methods.
Like I said in the place of
$_SERVER['REMOTE_ADDR']
I want to use
$_SERVER["HTTP_X_MXIT_USERID_R"];
as the so called "IP" in this scenario. I run my website via a mobi. portal on an instant mesaging platform called Mxit. And the above code will recall their unique login id into the Instant messanger. Thats why I need to change my code to make sure that a user with the same ID wont be able to vote again.
-
Hi, I'm currently using a voting script, but have a problem with people voting more then once, and want to add a way to keep the voting unique and 1 per person via IP check can anybody assist me how to implement it in the following script please?
<?php // the questions and the answers $pool_question="Do you think I should keep Galaxy Universe open?"; $pool_option[1]="Yes"; $pool_option[2]="No"; // If counter files are not available,they will be created // You may remove next lines after the first use of the script if (!file_exists("pool_5483543_1.txt")){ // next two lines will not work if writing permissions are not available // you may create the files bellow manualy with "0" as their unique content file_put_contents ("pool_5483543_1.txt",0); file_put_contents ("pool_5483543_2.txt",0); } // retrieve data saved in files $pool_responses[1]=file_get_contents("pool_5483543_1.txt"); $pool_responses[2]=file_get_contents("pool_5483543_2.txt"); // if user votes, increase corresponding value if ($_POST["5483543"] and $_POST["5483543b"]==""){ if ($_POST["5483543"]==1) {$pool_responses[1]++;file_put_contents("pool_5483543_1.txt",$pool_responses[1]);} if ($_POST["5483543"]==2) {$pool_responses[2]++;file_put_contents("pool_5483543_2.txt",$pool_responses[2]);} } // get percentajes for each answer in the pool // get total number of answers $total_responses=$pool_responses[1]+$pool_responses[2]; if ($total_responses==0){$total_responses=1;} // to avoid errors at start // compute percentajes (with one decimal number) $pool_percentaje[1] = round((100*$pool_responses[1])/$total_responses,1); $pool_percentaje[2] = round((100*$pool_responses[2])/$total_responses,1); // print the form, which includes de answers and the percentajes print "<center>\n"; print "<form method=post action=".$_SERVER["PHP_SELF"].">\n"; print "<b>".$pool_question."</b>\n"; print "<table cellpadding=4>\n<br>"; // answer 1 print "<tr>\n"; print "<td><input type=radio name=5483543 value=1> ".$pool_option[1]."</td>\n"; print "<td bgcolor=DDDDFF>" .$pool_responses[1]." (".$pool_percentaje[1]."%)</td>\n"; print "</tr>\n"; // answer 2 print "<tr>\n"; print "<td><input type=radio name=5483543 value=2> ".$pool_option[2]."</td>\n"; print "<td bgcolor=DDDDFF>" .$pool_responses[2]." (".$pool_percentaje[2]."%)</td>\n"; print "</tr>\n"; print "</table>\n"; // a simple control to avoid one user to vote several times if ($_POST["5483543"]){ print "<input type=hidden name=5483543b value=1>\n"; } print "<input TYPE=submit value=Add my answer>\n"; print "</form>\n"; print "</center>\n"; ?>
The reason why I ask for IP check is I wan't to use
$_SERVER["HTTP_X_MXIT_USERID_R"];
in the place of the Ip since it give a unique name via the platform I want to implement it.
-
You do realize this has been done many times before you attempted it? Have you tried googling for "php swear filter" or anything? There are lots of libraries out there that already are working, and probably a lot better than you can do trying from scratch because the problem is a bit more complex than I think you are considering.
You don't want the filter to work on substrings for the reason that Jacques1 stated. "I assume you're talking about me" is a legitimate, clean piece of text. "ass" in assume should not be filtered. So you probably want it to work on individual words. That gets a bit complex for the regex because "you're an ass." (with a period after ass) is harder to match than "I think you're an ass dude." where ass is by itself. I'm sure there are regex modifiers that will do all of that, but trying to think of all main scenarios and then coding for it will be a bit of a challenge unless you are really good at regex. I'd suggest looking for something off-the-shelf and ready to go, all tried and tested.
Thank you I totally see your point. Will search for a good one but in the meantime I found a solution for the problem.
function filterBadWords($str) { $result1 = mysql_query("SELECT word FROM StringyChat_WordBan") or die(mysql_error()); $replacements = "#~"; while($row = mysql_fetch_assoc($result1)) { $str = preg_replace('/\b' . $row['word'].'\b/ie', str_repeat('#~', strlen($row['word'])), $str); } return $str; }
-
A ban? You mean a kid who likes Charles Dickens would be thrown out of your chat? C'mon, you can't be serious.
Even the best filter has false positives, meaning a legitimate user making legitimate statements will be erroneously flagged. If you automatically ban them, sorry, then your application sucks.
Well the filter will only be in messages not Names so if a username is Charles Dickens it would be accepted but not if someone mention it in a message. My Hosting rules recommend I must use some kind of method to keep chats clean. Can someone just point me in the right direction?
-
I've always found those to be next to useless. You can always alter how you spell things and still get the intention of the word to come across. Are you going to be able to filter all possibilities, including misspellings and phonetics? No. I don't know what would be less offensive..to be called a dick, dik, diq, etc. All have the same intention.
It's true but it will help with the basic words. I'm going to add like a time out ban if such a word would be detected.
-
PCRE regexes need delimiters. Like so: '/.../' (note the slashes).
Besides that, the whole approach looks rather problematic. If you do a substring search, what happens with “Charles Dickens”? Is this name politically incorrect and will be censored?
Of course censorship itself is crap, but that's a different discussion ...
So how would you approach this scenario where I want to use it as a profanity filter?
-
Hi I'm trying to get rid of the deprecated functions like the eregi replace, but is currently experiancing a few problems.
I have the following function
function filterBadWords($str) { $result1 = mysql_query("SELECT word FROM StringyChat_WordBan") or die(mysql_error()); $replacements = "#~"; while($row = mysql_fetch_assoc($result1)) { $str = eregi_replace($row['word'], str_repeat('#~', strlen($row['word'])), $str); } return $str; }
and tried changing it into
function filterBadWords($str) { $result1 = mysql_query("SELECT word FROM StringyChat_WordBan") or die(mysql_error()); $replacements = "(G)"; while($row = mysql_fetch_assoc($result1)) { $str = preg_replace($row['word'], str_repeat('(G)', strlen($row['word'])), $str); } return $str; }
but now I'm getting errors like
preg_replace(): Delimiter must not be alphanumeric or backslash
Any help regarding this?
String if a specific word is found in the string replace the whole string
in PHP Coding Help
Posted