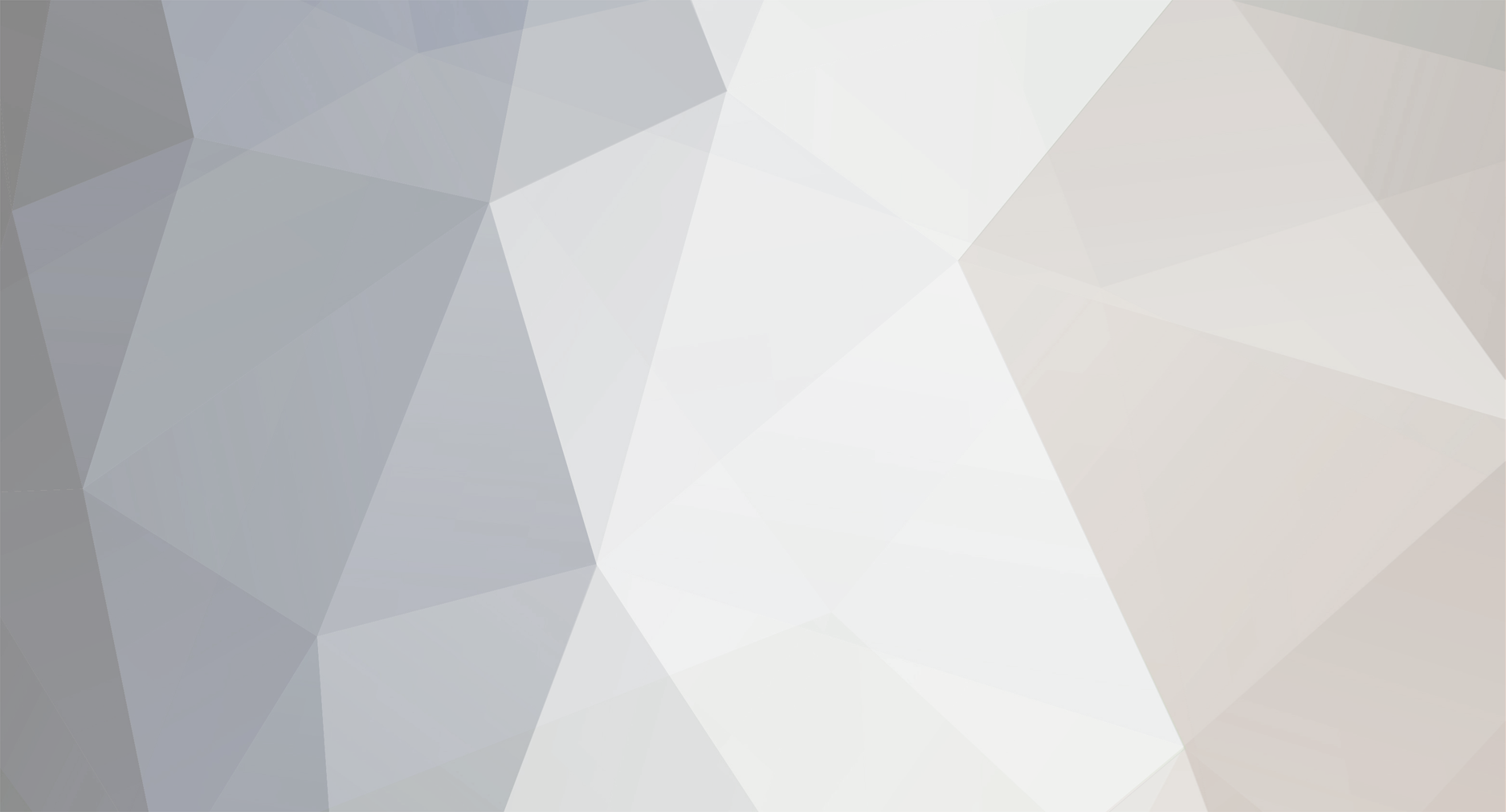
cobusbo
-
Posts
224 -
Joined
-
Last visited
Posts posted by cobusbo
-
-
Anything outside of the <?php ?> tags is considered output too.
The error will be triggered because of the <html> before the <?php
Modify your code so HTML is output after any business logic in your code.
Ok the error message is gone now but messages still get submitted with every refresh..
<?php /*** begin the session ***/ session_start(); /*** create the form token ***/ $form_token = uniqid(); /*** add the form token to the session ***/ $_SESSION['form_token'] = $form_token; define('TIMEZONE', 'Africa/Harare'); date_default_timezone_set(TIMEZONE); // database connection info $conn = mysql_connect('********','***********','***********') or trigger_error("SQL", E_USER_ERROR); $db = mysql_select_db('u506124311_cobus',$conn) or trigger_error("SQL", E_USER_ERROR); // find out how many rows are in the table $sql = "SELECT COUNT(*) FROM StringyChat"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); $r = mysql_fetch_row($result); $numrows = $r[0]; // number of rows to show per page $rowsperpage = 20; // find out total pages $totalpages = ceil($numrows / $rowsperpage); // get the current page or set a default if (isset($_GET['currentpage']) && is_numeric($_GET['currentpage'])) { // cast var as int $currentpage = (int) $_GET['currentpage']; } else { // default page num $currentpage = 1; } // end if // if current page is greater than total pages... if ($currentpage > $totalpages) { // set current page to last page $currentpage = $totalpages; } // end if // if current page is less than first page... if ($currentpage < 1) { // set current page to first page $currentpage = 1; } // end if // the offset of the list, based on current page $offset = ($currentpage - 1) * $rowsperpage; $ip = $_SERVER["REMOTE_ADDR"]; $name = $_SERVER["HTTP_X_MXIT_USERID_R"]; $msg = $_POST['message']; $time = date("U"); $mxitid = $_SERVER["HTTP_X_MXIT_USERID_R"]; if(!isset($mxitid, $name )) { $mxitid = "DEFAULT"; $name = "SYSOP"; } $sqli = "INSERT INTO StringyChat (StringyChat_ip, StringyChat_name, StringyChat_message, StringyChat_time, mxit_id) VALUES ('$ip', '$name', '$msg', '$time', '$mxitid')"; $result = mysql_query($sqli, $conn) or trigger_error("SQL", E_USER_ERROR); // get the info from the db $sql = "SELECT StringyChat_time, StringyChat_name, StringyChat_message FROM StringyChat ORDER BY id DESC LIMIT $offset, $rowsperpage"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); function filterBadWords($str) { $result1 = mysql_query("SELECT word FROM StringyChat_WordBan") or die(mysql_error()); $replacements = ":-x"; while($row = mysql_fetch_assoc($result1)) { $str = eregi_replace($row['word'], str_repeat(':-x', strlen($row['word'])), $str); } return $str; } // while there are rows to be fetched... while ($list = mysql_fetch_assoc($result)) //while (($pmsg = $list['StringyChat_message'] == $bwords) ? ":-x" : $list['StringyChat_message']) { // echo data //echo ($pmsg = ($list['StringyChat_message'] == $bwords) ? ":-x" : $list['StringyChat_message']) print '<span style="color:#828282">' . '(' . date( 'D H:i:s', $list['StringyChat_time'] ) . ') ' . '</span>' . '<b>' . $list['StringyChat_name'] . '</b>' . ' : ' . filterBadWords($list['StringyChat_message']) . '<br />'; } // end while /****** build the pagination links ******/ // range of num links to show $range = 3; // if not on page 1, don't show back links if ($currentpage > 1) { // show << link to go back to page 1 echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=1'><<</a> "; // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$prevpage'><</a> "; } // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($x == $currentpage) { // 'highlight' it but don't make a link echo " [<b>$x</b>] "; // if not current page... } else { // make it a link echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$x'>$x</a> "; } // end else } // end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $currentpage + 1; // echo forward link for next page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$nextpage'>></a> "; // echo forward link for lastpage echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$totalpages'>>></a> "; } // end if /****** end build pagination links ******/ ?><br> <html> <body> <form name="StringyChat_form" method="POST" action="<? echo $_SERVER['REQUEST_URI']; ?>"> <br> <input type="hidden" name="name" class="StringyChatFrm" value="<?php $name ?>" size="20" > <br> <i>Type your Message here...</i>:<br> <textarea name="message" class="StringyChatFrm" cols="20" rows="4"></textarea> <br> <input type="hidden" name="form_token" value="<?php echo $form_token; ?>" /><br> <input name="StringyChat_submit" class="StringyChatFrm" type="submit" value="Post Message"> </form> </body> </html>
-
session_start() must be used before you send anything else to the browser. it must be the first thing in your code on the page.
Ok so I moved it to the top of my script but still the same message
<html> <?php /*** begin the session ***/ session_start(); /*** create the form token ***/ $form_token = uniqid(); /*** add the form token to the session ***/ $_SESSION['form_token'] = $form_token; define('TIMEZONE', 'Africa/Harare'); date_default_timezone_set(TIMEZONE); // database connection info $conn = mysql_connect('********','********','*********') or trigger_error("SQL", E_USER_ERROR); $db = mysql_select_db('u506124311_cobus',$conn) or trigger_error("SQL", E_USER_ERROR); // find out how many rows are in the table $sql = "SELECT COUNT(*) FROM StringyChat"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); $r = mysql_fetch_row($result); $numrows = $r[0]; // number of rows to show per page $rowsperpage = 20; // find out total pages $totalpages = ceil($numrows / $rowsperpage); // get the current page or set a default if (isset($_GET['currentpage']) && is_numeric($_GET['currentpage'])) { // cast var as int $currentpage = (int) $_GET['currentpage']; } else { // default page num $currentpage = 1; } // end if // if current page is greater than total pages... if ($currentpage > $totalpages) { // set current page to last page $currentpage = $totalpages; } // end if // if current page is less than first page... if ($currentpage < 1) { // set current page to first page $currentpage = 1; } // end if // the offset of the list, based on current page $offset = ($currentpage - 1) * $rowsperpage; $ip = $_SERVER["REMOTE_ADDR"]; $name = $_SERVER["HTTP_X_MXIT_USERID_R"]; $msg = $_POST['message']; $time = date("U"); $mxitid = $_SERVER["HTTP_X_MXIT_USERID_R"]; if(!isset($mxitid, $name )) { $mxitid = "DEFAULT"; $name = "SYSOP"; } $sqli = "INSERT INTO StringyChat (StringyChat_ip, StringyChat_name, StringyChat_message, StringyChat_time, mxit_id) VALUES ('$ip', '$name', '$msg', '$time', '$mxitid')"; $result = mysql_query($sqli, $conn) or trigger_error("SQL", E_USER_ERROR); // get the info from the db $sql = "SELECT StringyChat_time, StringyChat_name, StringyChat_message FROM StringyChat ORDER BY id DESC LIMIT $offset, $rowsperpage"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); function filterBadWords($str) { $result1 = mysql_query("SELECT word FROM StringyChat_WordBan") or die(mysql_error()); $replacements = ":-x"; while($row = mysql_fetch_assoc($result1)) { $str = eregi_replace($row['word'], str_repeat(':-x', strlen($row['word'])), $str); } return $str; } // while there are rows to be fetched... while ($list = mysql_fetch_assoc($result)) //while (($pmsg = $list['StringyChat_message'] == $bwords) ? ":-x" : $list['StringyChat_message']) { // echo data //echo ($pmsg = ($list['StringyChat_message'] == $bwords) ? ":-x" : $list['StringyChat_message']) print '<span style="color:#828282">' . '(' . date( 'D H:i:s', $list['StringyChat_time'] ) . ') ' . '</span>' . '<b>' . $list['StringyChat_name'] . '</b>' . ' : ' . filterBadWords($list['StringyChat_message']) . '<br />'; } // end while /****** build the pagination links ******/ // range of num links to show $range = 3; // if not on page 1, don't show back links if ($currentpage > 1) { // show << link to go back to page 1 echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=1'><<</a> "; // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$prevpage'><</a> "; } // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($x == $currentpage) { // 'highlight' it but don't make a link echo " [<b>$x</b>] "; // if not current page... } else { // make it a link echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$x'>$x</a> "; } // end else } // end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $currentpage + 1; // echo forward link for next page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$nextpage'>></a> "; // echo forward link for lastpage echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$totalpages'>>></a> "; } // end if /****** end build pagination links ******/ ?><br> <body> <form name="StringyChat_form" method="POST" action="<? echo $_SERVER['REQUEST_URI']; ?>"> <br> <input type="hidden" name="name" class="StringyChatFrm" value="<?php $name ?>" size="20" > <br> <i>Type your Message here...</i>:<br> <textarea name="message" class="StringyChatFrm" cols="20" rows="4"></textarea> <br> <input type="hidden" name="form_token" value="<?php echo $form_token; ?>" /><br> <input name="StringyChat_submit" class="StringyChatFrm" type="submit" value="Post Message"> </form> </body> </html>
-
there are two things to do to address re-submitting form data. the first one address preventing the data from being processed again. the second one is to make the 'user' experience better (i.e. prevent the browser from displaying any of the resubmit form data/expired page-form messages.)
1) each time you output the form, you need to produce a unique-random one-use 'token' that's put into a hidden form field and stored in a session variable. when the form is submitted, you test that the session variable exists, is not empty, and that it matches the value from the hidden form field to serve as a condition for even processing the form data. you clear the session variable in the form processing code, which causes the form processing code to skip processing any re-submission of the form data. this also helps to prevent a bot script/someone from requesting your form once and using it to keep submitting comments. they must actually receive your form with a new token value to be able to submit a comment.
2) after you have successfully processed the form data (inserted it into the database table), you need to do a header() redirect to the exact same url that the form submitted to. this will cause the last action in the browser for that url to be a GET request for the page and the browser won't attempt to resubmit the form data due to a refresh of the page or navigating to that url.
there are some things your current code needs to do that it isn't already doing. your form processing code needs to check that a post method form was submitted at all, so that the form processing code only runs if there is $_POST data and you need to validate that the required form fields are at least not empty. your current code will insert a row with an empty message field every time the page gets requested.
Ok So I went and added a session so my form as said above
<html> <?php define('TIMEZONE', 'Africa/Harare'); date_default_timezone_set(TIMEZONE); // database connection info $conn = mysql_connect('*****','**********','*********') or trigger_error("SQL", E_USER_ERROR); $db = mysql_select_db('u506124311_cobus',$conn) or trigger_error("SQL", E_USER_ERROR); // find out how many rows are in the table $sql = "SELECT COUNT(*) FROM StringyChat"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); $r = mysql_fetch_row($result); $numrows = $r[0]; // number of rows to show per page $rowsperpage = 20; // find out total pages $totalpages = ceil($numrows / $rowsperpage); // get the current page or set a default if (isset($_GET['currentpage']) && is_numeric($_GET['currentpage'])) { // cast var as int $currentpage = (int) $_GET['currentpage']; } else { // default page num $currentpage = 1; } // end if // if current page is greater than total pages... if ($currentpage > $totalpages) { // set current page to last page $currentpage = $totalpages; } // end if // if current page is less than first page... if ($currentpage < 1) { // set current page to first page $currentpage = 1; } // end if // the offset of the list, based on current page $offset = ($currentpage - 1) * $rowsperpage; $ip = $_SERVER["REMOTE_ADDR"]; $name = $_SERVER["HTTP_X_MXIT_USERID_R"]; $msg = $_POST['message']; $time = date("U"); $mxitid = $_SERVER["HTTP_X_MXIT_USERID_R"]; if(!isset($mxitid, $name )) { $mxitid = "DEFAULT"; $name = "SYSOP"; } $sqli = "INSERT INTO StringyChat (StringyChat_ip, StringyChat_name, StringyChat_message, StringyChat_time, mxit_id) VALUES ('$ip', '$name', '$msg', '$time', '$mxitid')"; $result = mysql_query($sqli, $conn) or trigger_error("SQL", E_USER_ERROR); // get the info from the db $sql = "SELECT StringyChat_time, StringyChat_name, StringyChat_message FROM StringyChat ORDER BY id DESC LIMIT $offset, $rowsperpage"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); function filterBadWords($str) { $result1 = mysql_query("SELECT word FROM StringyChat_WordBan") or die(mysql_error()); $replacements = ":-x"; while($row = mysql_fetch_assoc($result1)) { $str = eregi_replace($row['word'], str_repeat(':-x', strlen($row['word'])), $str); } return $str; } // while there are rows to be fetched... while ($list = mysql_fetch_assoc($result)) //while (($pmsg = $list['StringyChat_message'] == $bwords) ? ":-x" : $list['StringyChat_message']) { // echo data //echo ($pmsg = ($list['StringyChat_message'] == $bwords) ? ":-x" : $list['StringyChat_message']) print '<span style="color:#828282">' . '(' . date( 'D H:i:s', $list['StringyChat_time'] ) . ') ' . '</span>' . '<b>' . $list['StringyChat_name'] . '</b>' . ' : ' . filterBadWords($list['StringyChat_message']) . '<br />'; } // end while /****** build the pagination links ******/ // range of num links to show $range = 3; // if not on page 1, don't show back links if ($currentpage > 1) { // show << link to go back to page 1 echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=1'><<</a> "; // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$prevpage'><</a> "; } // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($x == $currentpage) { // 'highlight' it but don't make a link echo " [<b>$x</b>] "; // if not current page... } else { // make it a link echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$x'>$x</a> "; } // end else } // end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $currentpage + 1; // echo forward link for next page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$nextpage'>></a> "; // echo forward link for lastpage echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$totalpages'>>></a> "; } // end if /****** end build pagination links ******/ ?><br> <?php /*** begin the session ***/ session_start(); /*** create the form token ***/ $form_token = uniqid(); /*** add the form token to the session ***/ $_SESSION['form_token'] = $form_token; ?> <body> <form name="StringyChat_form" method="POST" action="<? echo $_SERVER['REQUEST_URI']; ?>"> <br> <input type="hidden" name="name" class="StringyChatFrm" value="<?php $name ?>" size="20" > <br> <i>Type your Message here...</i>:<br> <textarea name="message" class="StringyChatFrm" cols="20" rows="4"></textarea> <br> <input type="hidden" name="form_token" value="<?php echo $form_token; ?>" /><br> <input name="StringyChat_submit" class="StringyChatFrm" type="submit" value="Post Message"> </form> </body> </html>
But now I'm receiving the error
Warning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at /home/u506124311/public_html/ag/page.php:2) -
Hi I made a simple chat script with pagination in MySQL (yes I know I should change to MySQLi) but just bare with me please
My script is working fine when I post messages, but I have a problem.. Each time I refresh my page my previous message gets reposted again. Is there maybe a way I can fix this problem?
<html> <?php define('TIMEZONE', 'Africa/Harare'); date_default_timezone_set(TIMEZONE); // database connection info $conn = mysql_connect('****','******','*****') or trigger_error("SQL", E_USER_ERROR); $db = mysql_select_db('*****'',$conn) or trigger_error("SQL", E_USER_ERROR); // find out how many rows are in the table $sql = "SELECT COUNT(*) FROM StringyChat"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); $r = mysql_fetch_row($result); $numrows = $r[0]; // number of rows to show per page $rowsperpage = 20; // find out total pages $totalpages = ceil($numrows / $rowsperpage); // get the current page or set a default if (isset($_GET['currentpage']) && is_numeric($_GET['currentpage'])) { // cast var as int $currentpage = (int) $_GET['currentpage']; } else { // default page num $currentpage = 1; } // end if // if current page is greater than total pages... if ($currentpage > $totalpages) { // set current page to last page $currentpage = $totalpages; } // end if // if current page is less than first page... if ($currentpage < 1) { // set current page to first page $currentpage = 1; } // end if // the offset of the list, based on current page $offset = ($currentpage - 1) * $rowsperpage; // INSERT INTO DATABASE $ip = $_SERVER["REMOTE_ADDR"]; $name = $_SERVER["HTTP_X_MXIT_USERID_R"]; $msg = $_POST['message']; $time = date("U"); $mxitid = $_SERVER["HTTP_X_MXIT_USERID_R"]; if(!isset($mxitid, $name )) { $mxitid = "DEFAULT"; $name = "SYSOP"; } $sqli = "INSERT INTO StringyChat (StringyChat_ip, StringyChat_name, StringyChat_message, StringyChat_time, mxit_id) VALUES ('$ip', '$name', '$msg', '$time', '$mxitid')"; $result = mysql_query($sqli, $conn) or trigger_error("SQL", E_USER_ERROR); // get the info from the db $sql = "SELECT StringyChat_time, StringyChat_name, StringyChat_message FROM StringyChat ORDER BY id DESC LIMIT $offset, $rowsperpage"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); function filterBadWords($str) { $result1 = mysql_query("SELECT word FROM StringyChat_WordBan") or die(mysql_error()); $replacements = ":-x"; while($row = mysql_fetch_assoc($result1)) { $str = eregi_replace($row['word'], str_repeat(':-x', strlen($row['word'])), $str); } return $str; } // while there are rows to be fetched... while ($list = mysql_fetch_assoc($result)) //while (($pmsg = $list['StringyChat_message'] == $bwords) ? ":-x" : $list['StringyChat_message']) { // echo data //echo ($pmsg = ($list['StringyChat_message'] == $bwords) ? ":-x" : $list['StringyChat_message']) print '<span style="color:#828282">' . '(' . date( 'D H:i:s', $list['StringyChat_time'] ) . ') ' . '</span>' . '<b>' . $list['StringyChat_name'] . '</b>' . ' : ' . filterBadWords($list['StringyChat_message']) . '<br />'; } // end while /****** build the pagination links ******/ // range of num links to show $range = 3; // if not on page 1, don't show back links if ($currentpage > 1) { // show << link to go back to page 1 echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=1'><<</a> "; // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$prevpage'><</a> "; } // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($x == $currentpage) { // 'highlight' it but don't make a link echo " [<b>$x</b>] "; // if not current page... } else { // make it a link echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$x'>$x</a> "; } // end else } // end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $currentpage + 1; // echo forward link for next page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$nextpage'>></a> "; // echo forward link for lastpage echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$totalpages'>>></a> "; } // end if /****** end build pagination links ******/ ?><br> // FORM <body> <form name="StringyChat_form" method="POST" action="<? echo $_SERVER['REQUEST_URI']; ?>"> <br> <input type="hidden" name="name" class="StringyChatFrm" value="<?php $name ?>" size="20" > <br> <i>Type your Message here...</i>:<br> <textarea name="message" class="StringyChatFrm" cols="20" rows="4"></textarea> <br> <input name="StringyChat_submit" class="StringyChatFrm" type="submit" value="Post Message"> </form> </body> </html>
-
Yes, but you put them inside parentheses. Look at the examples in manual for the ON DUPLICATE KEY UPDATE clauses - not a "(" or ")" to be seen
Thank you it solved my problem
$query = mysqli_query($con,"INSERT IGNORE INTO mxit (ip,time,user_agent,contact,userid,id,login,nick,location,profile) VALUES ('$ip','$post_time','$mxitua','$mxitcont','$mxituid','$mxitid','$mxitlogin','$mxitnick','$mxitloc','$mxitprof') ON DUPLICATE KEY UPDATE ip='$ip',user_agent='$mxitua',contact='$mxitcont',login='$mxitlogin',nick='$mxitnick',location='$mxitloc',profile='$mxitprof'") or die(mysqli_error($con));
-
Parentheses!
Ok I tried
$query = mysqli_query($con,"INSERT IGNORE INTO mxit (ip,time,user_agent,contact,userid,id,login,nick,location,profile) VALUES ('$ip','$post_time','$mxitua','$mxitcont','$mxituid','$mxitid','$mxitlogin','$mxitnick','$mxitloc','$mxitprof') ON DUPLICATE KEY UPDATE (ip,user_agent,contact,login,nick,location,profile) VALUES ('$ip','$mxitua','$mxitcont','$mxitlogin','$mxitnick','$mxitloc','$mxitprof')") or die(mysqli_error($con));
-
I don't get it I used commas
ON DUPLICATE KEY UPDATE (ip=$ip,user_agent=$mxitua,contact=$mxitcont,login=$mxitlogin,nick=$mxitnick,location=$mxitloc,profile=$mxitprof)")
-
No you haven't. You are getting that error because you have given the ON DUPLICATE KEY UPDATE clause an incorrect value.
The value you pass it is the unique primary key that identifies the row you are going to update when a duplicate entry occurs.
My Unique field is the userid field and I didnt include it as you can see above. I only included certain fields to update?
-
You use "... ON DUPLICATE KEY UPDATE .."
http://dev.mysql.com/doc/refman/5.6/en/insert-on-duplicate.html
ok I followed the example, but receiving an error message
$query = mysqli_query($con,"INSERT IGNORE INTO mxit (ip,time,user_agent,contact,userid,id,login,nick,location,profile) VALUES ('$ip','$post_time','$mxitua','$mxitcont','$mxituid','$mxitid','$mxitlogin','$mxitnick','$mxitloc','$mxitprof') ON DUPLICATE KEY UPDATE (ip=$ip,user_agent=$mxitua,contact=$mxitcont,login=$mxitlogin,nick=$mxitnick,location=$mxitloc,profile=$mxitprof)") or die(mysqli_error($con));
the error message is as follow
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near '(ip=197.79.26.192,user_agent=PURPLE,contact=guniverse,login=cobusbo,nick=~cobusb' at line 2 -
Hi I'm currently experiencing a problem with my query. I've used the `INSERT IGNORE` option in my query and it works pretty well not to add duplicates, but the problem is that if my unique field match it doesn't update any other info in the row, is there maybe another option to update a row if an existing field exists but insert new row if it doesn't exists?
my current query is as follow
$query = mysqli_query($con,"INSERT IGNORE INTO mxit (ip,time,user_agent,contact,userid,id,login,nick,location,profile) VALUES ('$ip','$post_time','$mxitua','$mxitcont','$mxituid','$mxitid','$mxitlogin','$mxitnick','$mxitloc','$mxitprof')") or die(mysqli_error($con));
-
Hi, Ive stumbled apon a Mongo database connected to a broadcast script. I would like to change it to a Mysql database can anybody please show me an example how it will look in Mysql format
<?php /* Require the PHP wrapper for the Mxit API */ require_once ('MxitAPI.php'); /* Function to count the number of users in MongoDB */ function count_users() { $mongo = new Mongo('127.0.0.1'); $collection = $mongo->sampleapp->users; $collection->ensureIndex(array('uid' => 1)); return $collection->count(); } /* Function to get batches of users from MongoDB */ function get_users($skip=0, $limit=50) { $mongo = new Mongo('127.0.0.1'); $collection = $mongo->sampleapp->users; $collection->ensureIndex(array('mxitid' => 1, 'created_at' => 1)); $users = $collection->find()->sort(array('created_at' => 1))->skip($skip)->limit($limit); return iterator_to_array($users); } /* Instantiate the Mxit API */ $api = new MxitAPI($key, $secret); /* Set up the message */ $message = "(\*) Congratulations to our winners (\*)\n\n"; $message .= "1st place - Michael with 100 points\n"; $message .= "2nd place - Sipho with 50 points\n"; $message .= "3nd place - Carla with 25 points\n\n"; $message .= 'Good Luck! $Click here$'; /* Mxit Markup is included in the message, so set ContainsMarkup to true */ $contains_markup = 'true'; /* Count the number of users in the database */ $count = count_users(); /* Initialise the variable that counts how many messages have been sent */ $sent = 0; /* Keep looping through the user list, until the number of messages sent equals the number of users */ while ($sent < $count) { /* Get users in batches of 50 */ $users = get_users($sent, 50); /* The list where the user MxitIDs will be stored */ $list = array(); foreach ($users as $user) { $list[] = $user['mxitid']; $sent++; } /* If there is a problem getting an access token, retry */ $access_token = NULL; while (is_null($access_token)) { /* We are sending a message so request access to the message/send scope */ $api->get_app_token('message/send'); $token = $api->get_token(); $access_token = $token['access_token']; // Only attempt to send a message if we have a valid auth token if (!is_null($access_token)) { $users = implode(',', $list); echo "\n$sent: $users\n"; $api->send_message($app, $users, $message, $contains_markup); } } } echo "\n\nBroadcast to $sent users\n\n";
-
Perhaps a combination of
- file(), to read the csv file into an array and
- array_unique(), to remove any duplicates
Thank you It solved my problem
<?php $myfile = fopen("users.csv", "a+") or die("Unable to open file!"); $mxituid = $_SERVER["HTTP_X_MXIT_USERID_R"]; $txt = "$mxituid\n"; fwrite($myfile, $txt); fclose($myfile); $list = file('users.csv'); $list = array_unique($list); file_put_contents('unique.csv', implode('', $list)); ?>
-
Only possible if you first read the users.csv into memory and then scan every entry to see if there is a match. You should better store this into a database.
It doesn't have any private info in it the reason I want to store it as this file type is because I need to link the file to another website to publish something to all users saved in this file. So I don't want duplicate records
-
Hi Im trying to write info to a text file and it works perfectly with the following script, but it writes duplicate info, how can I change it so that no duplicate records are being added to my file and just ignore inserting it without giving a message?
<?php $myfile = fopen("users.csv", "a+") or die("Unable to open file!"); $mxituid = $_SERVER["HTTP_X_MXIT_USERID_R"]; $txt = "$mxituid\n"; fwrite($myfile, $txt); fclose($myfile); ?>
-
Never used INSERT IGNORE, what insertion result do you get?
If a duplicate record in your database Unique field is being detected it will just ignore inserting another row in the background without giving a message error or anything
-
Ok I've manage to get my entries to work, but I'm currently experiencing a problem checking for duplicates. I want to use my userid column and check if a duplicate exist and if it does it should just ignore the entry in the background.
My current code is
<?php $con=mysqli_connect("sql305.mzzhost.com","mzzho_15247412","92295454","mzzho_15247412_mxit"); // Check connection if (mysqli_connect_errno()) { echo "Failed to connect to MySQL: " . mysqli_connect_error(); } ?> <? define('TIMEZONE', 'Africa/Harare'); date_default_timezone_set(TIMEZONE); $ip = $_SERVER["REMOTE_ADDR"]; $post_time = date("U"); $mxitua = $_SERVER["HTTP_X_DEVICE_USER_AGENT"]; $mxitcont = $_SERVER["HTTP_X_MXIT_CONTACT"]; $mxituid = $_SERVER["HTTP_X_MXIT_USERID_R"]; $mxitid = $_SERVER["HTTP_X_MXIT_ID_R"]; $mxitlogin = $_SERVER["HTTP_X_MXIT_LOGIN"]; $mxitnick = $_SERVER["HTTP_X_MXIT_NICK"]; $mxitloc = $_SERVER["HTTP_X_MXIT_LOCATION"]; $mxitprof = $_SERVER["HTTP_X_MXIT_PROFILE"]; if(!isset($mxitid)) { $mxitid = "DEFAULT"; } $query = mysqli_query($con,"INSERT IGNORE INTO mxit (ip,time,user_agent,contact,userid,id,login,nick,location,profile) VALUES ('$ip','$post_time','$mxitua','$mxitcont','$mxituid','$mxitid','$mxitlogin','$mxitnick','$mxitloc','$mxitprof')") or die(mysqli_error($con)); ?>
As you can see I tried to use the INSERT IGNORE option but it doesn't seem to work... any help please...
Nevermind I changed my Userid from text to varchar then I could make it an unique identifier and it solved my problem, thank you
-
Ok I've manage to get my entries to work, but I'm currently experiencing a problem checking for duplicates. I want to use my userid column and check if a duplicate exist and if it does it should just ignore the entry in the background.
My current code is
<?php $con=mysqli_connect("sql305.mzzhost.com","mzzho_15247412","92295454","mzzho_15247412_mxit"); // Check connection if (mysqli_connect_errno()) { echo "Failed to connect to MySQL: " . mysqli_connect_error(); } ?> <? define('TIMEZONE', 'Africa/Harare'); date_default_timezone_set(TIMEZONE); $ip = $_SERVER["REMOTE_ADDR"]; $post_time = date("U"); $mxitua = $_SERVER["HTTP_X_DEVICE_USER_AGENT"]; $mxitcont = $_SERVER["HTTP_X_MXIT_CONTACT"]; $mxituid = $_SERVER["HTTP_X_MXIT_USERID_R"]; $mxitid = $_SERVER["HTTP_X_MXIT_ID_R"]; $mxitlogin = $_SERVER["HTTP_X_MXIT_LOGIN"]; $mxitnick = $_SERVER["HTTP_X_MXIT_NICK"]; $mxitloc = $_SERVER["HTTP_X_MXIT_LOCATION"]; $mxitprof = $_SERVER["HTTP_X_MXIT_PROFILE"]; if(!isset($mxitid)) { $mxitid = "DEFAULT"; } $query = mysqli_query($con,"INSERT IGNORE INTO mxit (ip,time,user_agent,contact,userid,id,login,nick,location,profile) VALUES ('$ip','$post_time','$mxitua','$mxitcont','$mxituid','$mxitid','$mxitlogin','$mxitnick','$mxitloc','$mxitprof')") or die(mysqli_error($con)); ?>
As you can see I tried to use the INSERT IGNORE option but it doesn't seem to work... any help please...
-
Try to pass the link identifier as a parameter.
.................or die(mysqli_error($con));
Ok this is the error that appears
You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near '.12.51,1411750180,,,,DEFAULT,,,,)' at line 2
-
most likely the column names are spelt incorrectly in the query or incorrect data type you're inserting to db. Have you tried to debug it using a mysqli_error() function. Something like
$query = mysqli_query($con,"INSERT INTO mxit (ip,time,user_agent,contact,userid,id,login,nick,location,profile) VALUES ($ip,$post_time,$mxitua,$mxitcont,$mxituid,$mxitid,$mxitlogin,$mxitnick,$mxitloc,$mxitprof)") or die(mysqli_error());
ok the following error appears
Warning: mysqli_error() expects exactly 1 parameter, 0 given in /home/vol1_1/mzzhost.com/mzzho_15247412/htdocs/try/mxit.php on line 44 -
Hi I'm trying to insert unique info retrieved to my database but seems like I'm doing something wrong with my quary my current setup is as follow
mxit.php
<?php $con=mysqli_connect("*****","*******","*******","******"); // Check connection if (mysqli_connect_errno()) { echo "Failed to connect to MySQL: " . mysqli_connect_error(); } mysqli_close($con); ?> <? define('TIMEZONE', 'Africa/Harare'); date_default_timezone_set(TIMEZONE); $ip = $_SERVER["REMOTE_ADDR"]; $post_time = date("U"); $mxitua = $_SERVER["HTTP_X_DEVICE_USER_AGENT"]; $mxitcont = $_SERVER["HTTP_X_MXIT_CONTACT"]; $mxituid = $_SERVER["HTTP_X_MXIT_USERID_R"]; $mxitid = $_SERVER["HTTP_X_MXIT_ID_R"]; $mxitlogin = $_SERVER["HTTP_X_MXIT_LOGIN"]; $mxitnick = $_SERVER["HTTP_X_MXIT_NICK"]; $mxitloc = $_SERVER["HTTP_X_MXIT_LOCATION"]; $mxitprof = $_SERVER["HTTP_X_MXIT_PROFILE"]; if(!isset($mxitid)) { $mxitid = "DEFAULT"; } mysqli_query($con,"INSERT INTO mxit (ip,time,user_agent,contact,userid,id,login,nick,location,profile) VALUES ($ip,$post_time,$mxitua,$mxitcont,$mxituid,$mxitid,$mxitlogin,$mxitnick,$mxitloc,$mxitprof)"); mysqli_close($con); ?>
and ive included the above file on my index.php
<?PHP include "mxit.php"; ?>
but after I've opened up my index page I get an errorAnd another question is how can I check the field contact in my databases and if the name already exists not to add the record to my database? Since I don't want duplicate records...
ok so I removed the
mysqli_close($con);
at the top connection, but still no records has been added to my database
-
Hi I'm trying to insert unique info retrieved to my database but seems like I'm doing something wrong with my quary my current setup is as follow
mxit.php
<?php $con=mysqli_connect("*****","*******","*******","******"); // Check connection if (mysqli_connect_errno()) { echo "Failed to connect to MySQL: " . mysqli_connect_error(); } mysqli_close($con); ?> <? define('TIMEZONE', 'Africa/Harare'); date_default_timezone_set(TIMEZONE); $ip = $_SERVER["REMOTE_ADDR"]; $post_time = date("U"); $mxitua = $_SERVER["HTTP_X_DEVICE_USER_AGENT"]; $mxitcont = $_SERVER["HTTP_X_MXIT_CONTACT"]; $mxituid = $_SERVER["HTTP_X_MXIT_USERID_R"]; $mxitid = $_SERVER["HTTP_X_MXIT_ID_R"]; $mxitlogin = $_SERVER["HTTP_X_MXIT_LOGIN"]; $mxitnick = $_SERVER["HTTP_X_MXIT_NICK"]; $mxitloc = $_SERVER["HTTP_X_MXIT_LOCATION"]; $mxitprof = $_SERVER["HTTP_X_MXIT_PROFILE"]; if(!isset($mxitid)) { $mxitid = "DEFAULT"; } mysqli_query($con,"INSERT INTO mxit (ip,time,user_agent,contact,userid,id,login,nick,location,profile) VALUES ($ip,$post_time,$mxitua,$mxitcont,$mxituid,$mxitid,$mxitlogin,$mxitnick,$mxitloc,$mxitprof)"); mysqli_close($con); ?>
and ive included the above file on my index.php
<?PHP include "mxit.php"; ?>
but after I've opened up my index page I get an errorWarning: mysqli_query(): Couldn't fetch mysqli in /home/vol1_1/mzzhost.com/mzzho_15247412/htdocs/try/mxit.php on line 44
Warning: mysqli_close(): Couldn't fetch mysqli in /home/vol1_1/mzzhost.com/mzzho_15247412/htdocs/try/mxit.php on line 45And another question is how can I check the field contact in my databases and if the name already exists not to add the record to my database? Since I don't want duplicate records...
-
Your mysql query is failing, thereby returning false instead of a resource ID. This is likely because of a scope issue. When you have a function, any variables inside of the function must be created in the function itself, OR be passed in the arguments (I purposely steered clear of explaining globals here). Going further with the explanation, I can tell you that $dbTable, and $db are unknown variables in your function checkban(). You will either have to hard code them, or pass them in the arguments.
Since you hard coded the column names, it wouldn't be to hard to hard code the table name. Then either create a db connection inside of the function (useless, unless that is the only db call), or pass it in.
My suggestion would be:
function checkban($mxitid,$db) {
Ok so I changed my func.php to
<?php $mxitid = $_SERVER["HTTP_X_MXIT_USERID_R"]; require("admin_code_header.php"); function checkban($mxitid) { // querys database $q2 = mysql_query("SELECT 1 FROM StringyChat WHERE unban_time > NOW() AND mxit_id = $mxitid"); $get1 = mysql_num_rows($q2); // if found if ($get1 == "1") { // deny user access $r=mysql_fetch_array($q2); die("You have been banned from this website until . If you feel this is in error, please contact the webmaster at ."); } } ?>
But now I'm still receiving this error on my Admin page
and the same error on my index page
Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in /home/u506124311/public_html/ag/func.php on line 10And it seems like my option to insert the ban time to my Database don't work as well...
if ($_POST["1h"]) { $mxitid1= $_POST["1h"]; if(!$mxitid1) { echo "you must put in an MXIT ID"; } $sql1 = "INSERT StringyChat SET unban_time = DATE_ADD(NOW(), INTERVAL 1 DAY) WHERE mxit_id = $mxitid1)"; $result1 = mysql_query($sq1l); }
from my admin page...
My unban_time field in my database has been set as INT (10) and no default value...
-
The errors are self explanatory.
You are trying to call your checkban() function which you have not been defined! You have only defined this function your admin script. PHP will not be aware of that. So you need to make that function accessible from index1.php. I would suggest moving any common used functions into a separate file and then include that file when you are going to use a common function.
You have defined this function more than once. Function names must be unique.EDIT: You are getting that error because you have this code within your while loop
if ($_POST["1h"]) { $mxitid1= $_POST["1h"]; if(eregi("^[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}$", $IP_To_Add)) { $sql1 = "UPDATE ".$dbTable." SET unban_time = DATE_ADD(NOW(), INTERVAL 1 DAY) WHERE mxit_id = $mxitid1)"; $result1 = mysql_query($sq1l); } else { echo "Error: Cannot Kick: ".$IP_To_Add; } } function checkban($mxitid) { // querys database $q = mysql_query("SELECT 1 FROM ".$dbTable." WHERE unban_time > NOW() AND mxit_id = '$mxitid'",$db); $get = mysql_num_rows($q); // if found if ($get == "1") { // deny user access $r=mysql_fetch_array($q); die("You have been banned from this website until $r[legnth]. If you feel this is in error, please contact the webmaster at ."); } }
Move that code so it is not within thewhile loop. For example move it so it is before this line in your admin script
while ($myrow = mysql_fetch_array($result)) {
You are getting that error because the regex pattern used on the line below does not match the value in $IP_To_Add
if(eregi("^[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}$", $IP_To_Add))
NOTE: You should not be using ereg*() functions they are deprecated and no longer supported. You need to convert any use of ereg*() functions to use the PCRE functions. For example if your are using eregi() you need to use preg_match() applying the i pattern modifier to the regex pattern.
Ok after implementing the above I'm receiving the following error on my admin page
Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in /home/u506124311/public_html/ag/func.ban.php on line 9
and my Admin page looks like this
<? include "./emoticon_replace1.php"; if ($_POST["DeletePost"]) { $id = $_POST["id"]; $query = "DELETE FROM ".$dbTable." WHERE id='".$id."'"; mysql_query($query); echo "ID removed from system: ".$id; } if ($_POST["BanIP"]) { $IP_To_Add = $_POST["ip"]; if(eregi("^[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}$", $IP_To_Add)) { $sql = "INSERT INTO ".$IPBanTable." (ip) VALUES (\"$IP_To_Add\")"; $result = mysql_query($sql); } else { echo "Error: Not a valid IP: ".$IP_To_Add; } } if ($_POST["purge"]) { $query = "TRUNCATE TABLE ".$dbTable; mysql_query($query); echo "StringyChat purged"; } if(!$_POST["update"] || !$_POST["StringyChat_name"] || !$_POST["StringyChat_message"]) { } else { $id = $_POST["id"]; $name = $_POST["StringyChat_name"]; $message = $_POST["StringyChat_message"]; include("emoticon_replace.php"); $query = "UPDATE ".$dbTable." SET StringyChat_name='$name', StringyChat_message='$message' WHERE id='".$id."'"; $result = mysql_query($query, $db) or die("Invalid query: " . mysql_error()); } if ($_POST["EditPost"]) { $id = $_POST["id"]; $result = mysql_query("SELECT * FROM ".$dbTable." WHERE id='".$id."'", $db); $myrow = mysql_fetch_array($result); ?> <form name="StringyChat_form" method="POST" action="?mode=postman"> Name:<br> <input name="StringyChat_name" class="StringyChatFrm" type="text" size="20" maxlength="<? echo $name_size; ?>" value="<? echo $myrow["StringyChat_name"]?>"> <br> Message:<br> <textarea name="StringyChat_message" class="StringyChatFrm" cols="20" rows="4"><? echo $myrow["StringyChat_message"]?></textarea> <br> <input type="hidden" name="id" value="<? echo $id ?>"> <input name="update" class="StringyChatFrm" type="submit" value="Update"> </form> <? } ?> <a href="<? echo $_SERVER['REQUEST_URI']; ?>&m=purge">Purge StringyChat</a><br> <br> <? // Load up the last few posts. The number to load is defined by the "ShowPostNum" variable. $result = mysql_query("SELECT * FROM ".$dbTable." ORDER BY StringyChat_time DESC",$db); while ($myrow = mysql_fetch_array($result)) { $msg = $myrow["StringyChat_message"]; $msg = strip_tags($msg); $msg = eregi_replace("im#([a-z]{3})", "<img src=\"/stringychat/images/\\1.gif\" alt=\"emoticon\">",$msg); printf("<div class=\"StringyChatItem\"><h4>%s<br>\n", $myrow["StringyChat_name"]); printf("%s<p>\n",$myrow["StringyChat_ip"],"%s</p>\n"); printf("%s</h4>\n", date("H:i - d/m/y", $myrow["StringyChat_time"])); printf("%s</div>\n", $msg); ?> <form name="form<? echo $myrow["id"];?>" method="post" action="?mode=postman"> <input name="id" type="hidden" value="<? echo $myrow["id"];?>"> <input name="ip" type="hidden" value="<? echo $myrow["StringyChat_ip"];?>"> <input name="EditPost" type="submit" id="EditPost" value="Edit"> <input name="DeletePost" type="submit" id="DeletePost" value="Delete"> <input name="BanIP" type="submit" id="BanIP" value="Ban <? echo $myrow["StringyChat_ip"];?>"> <input name="1h" type="submit" id="1" value="Kick <? echo $myrow["mxit_id"];?>"> <input name="1d" type="submit" id="1d" value="Kick <? echo $myrow["StringyChat_ip"];?> for 24 hours "> <input name="7d" type="submit" id="7d" value="Kick <? echo $myrow["StringyChat_ip"];?> for 7 days "> </form> <? } if ($_POST["1h"]) { $mxitid1= $_POST["1h"]; if(!ip) { echo "you must put in an MXIT ID"; } $sql1 = "UPDATE ".$dbTable." SET unban_time = DATE_ADD(NOW(), INTERVAL 1 DAY) WHERE mxit_id = $mxitid1)"; $result1 = mysql_query($sq1l); } ?>
and on my index page I receive the following error
Warning: mysql_query(): supplied argument is not a valid MySQL-Link resource in /home/u506124311/public_html/ag/func.php on line 9
Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in /home/u506124311/public_html/ag/func.php on line 10And my Index file looks like the following
<?php require_once('common.php'); include "func.php"; checkban($_SERVER['HTTP_X_MXIT_USERID_R']); checkUser(); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "DTD/xhtml1-transitional.dtd"> <html> <head> <title>Galaxy Universe Chat</title> <link href="style/style.css" rel="stylesheet" type="text/css" /> </head> <body><br> <div id="main"> <div class="caption">Galaxy Universe Chat</div> <div id="icon"> </div> <div id="result"> <span style="color:lime">Hello <?php echo $_SESSION['userName']; ?> ! </span><br/> <div style="color:red"><b>Please keep it clean and in English or you will be banned!</b></div> <br> <?PHP include "./page.php"; include "./stringychat.inc.php"; ?> <br> <p><a href="index1.php">Refresh</a> | <a href="logout.php">Log Out</a></p> </div> <div id="source">Galaxy Wars chat @ cobusbo</div> </div> </body>
and lastly my func.php looks like
<?php $mxitid = $_SERVER["HTTP_X_MXIT_USERID_R"]; require("admin_code_header.php"); function checkban($mxitid) { // querys database $q2 = mysql_query("SELECT 1 FROM ".$dbTable." WHERE unban_time > NOW() AND mxit_id = '$mxitid'",$db); $get1 = mysql_num_rows($q2); // if found if ($get1 == "1") { // deny user access $r=mysql_fetch_array($q2); die("You have been banned from this website until . If you feel this is in error, please contact the webmaster at ."); } } ?>
Any help? -
And you think something's wrong because...
What's happening and what's supposed to be happening?
trying to load my index.php file i get the error
trying to load my admin page
and when i press kick button it says
So my problem is within my queries and form but not sure what I should do...
Need help to not resubmit info when refreshing
in PHP Coding Help
Posted
Ok I checked the token but seems like I'm still doing something wrong
I'm getting error