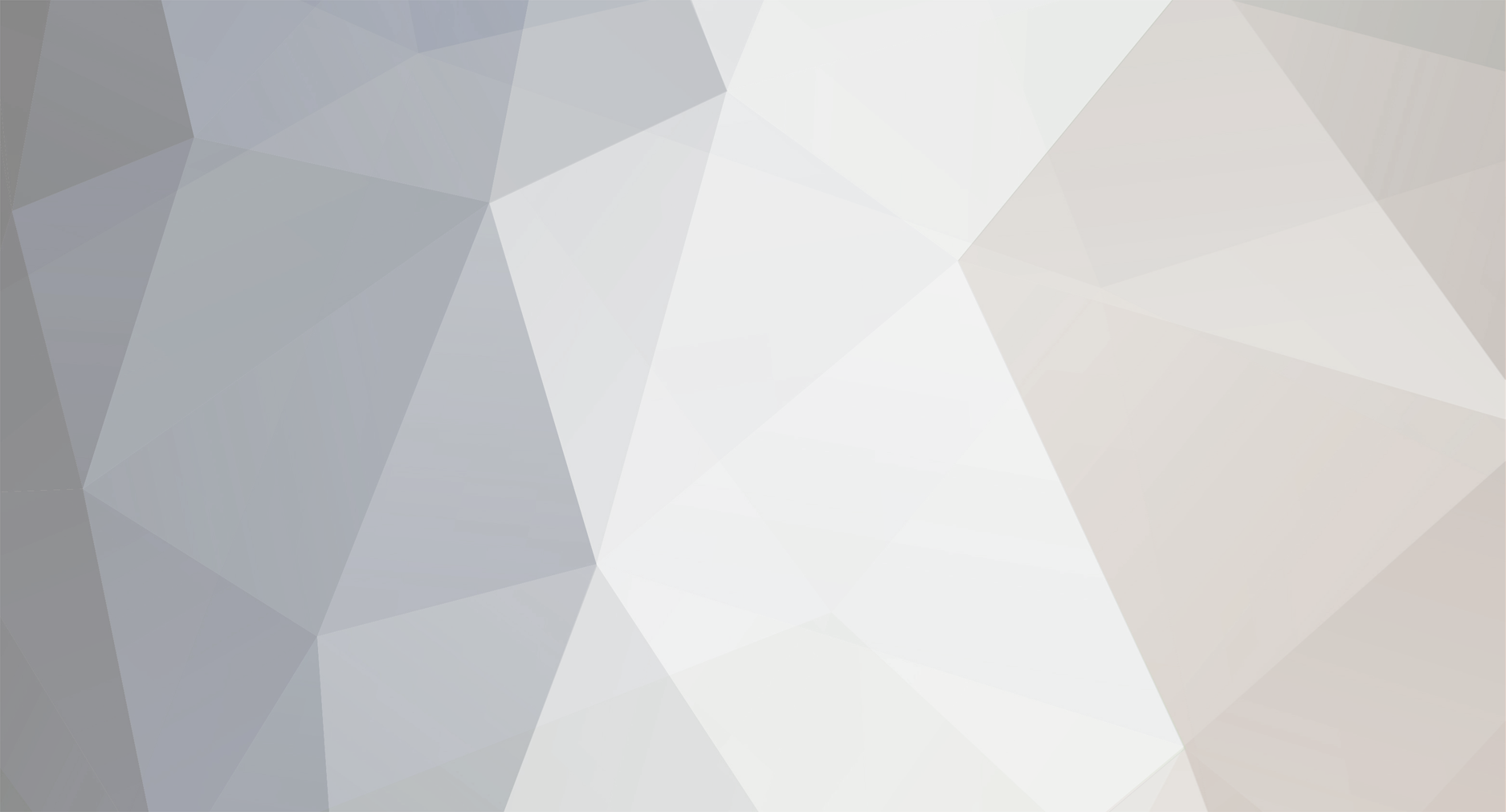
obsidian
Staff Alumni-
Posts
3,202 -
Joined
-
Last visited
Everything posted by obsidian
-
Check the number of records returned before you process the results: <?php $result = mysql_query($query) or die(mysql_error()); if (mysql_num_rows($result) > 0) { while($row = mysql_fetch_array($result, MYSQL_ASSOC)) { /* ... */ } } else { echo "No results"; } ?>
-
Based on the GMarkerOptions API, I believe what you want to do is pass the Icon and zIndexProcess to the GMarker constructor as an object. Try something like this and see if it works (notice especially lines 6-9 where the new GMarker is created): function createMarker(point, html, ba, ov, b) { var mylabel = {"url": overlay[ov], "anchor":new GLatLng(4, 4), "size": new GSize(12, 12)); var Icon = new GIcon(G_DEFAULT_ICON, background[ba], mylabel); var marker = new GMarker(point, { icon: Icon, zIndexProcess: importanceOrder }); marker.importance = b; GEvent.addListener(marker, "click", function() { marker.openInfoWindowHtml(html); }); return marker; } var point = new GLatLng(43.90, -78.0); var marker = createMarker(point, 'asdf', 'Up', 'A', 2); map.addOverlay(marker); Hope this helps!
-
If I'm not mistaken, onsubmit is only a valid event for the form tag itself, not for individual input fields. If you want to capture it on submit, you can take this onsubmit attribute and put it as part of your form tag proper; however, you'll want to change "yourtextboxname.value" to "this.yourtextboxname.value" so the form can locate the field (same with submitbuttonname). Another option would be to handle it in an onclick handler for the submit button instead of the onsubmit. I would do it slightly different than recommended above, but it may just be a preference thing: <input type="submit" name="submitbuttonname" onclick="this.form.textareaname.value = this.value;" value="My Submit" /> Good luck!
-
I have built a content slideshow using the jQuery library as well. When you target a div, you can simply call $('#my-div').fadeOut('slow') or $('#my-div').fadeIn('slow') and even pass a callback function to prep for your next transition. Just another option...
-
[quote author=redbullmarky link=topic=67269.msg987599#msg987599 date=1221079510] haha yeah!!! i remember gorillas (the example sourcecode/game that came with it) very well - loved it! [/quote] Nice. I had forgotten about that, but with your comment, a wave of memories came flooding back. I actually guess I started coding on my TI99 as a kid (whatever language that was), but I distinctly remember my first real interest being when I was using gwbasic and then on to qbasic.
-
So, in your case, you could just assign the value of the referred_to box to the innerHTML of the replace: document.getElementById('replace').innerHTML = document.getElementById('referred_to').value;
-
Hmm... no, it actually returns you an array of the unique elements. You might be better off with manually parsing your array if you simply want a boolean value. Try something like this: <?php function checkUnique($arr) { $tmp = array(); foreach ($arr as $v) { if (in_array($tmp)) { return false; } $tmp[] = $v; } return true; } ?>
-
The best way would be to use an array. You can then use methods such as array_unique() on it to see what you get.
-
Have you tried just accessing the window.title? Try something like this: JavaScript: function updateTitle(el) { window.title = el.value; } HTML: <input type="text" id="my-title" value="" onblur="updateTitle(this);" /> As you can see, I set it to update the title when your focus goes off the text field, but you can change the event to whatever you like.
-
Parent div needs relative positioning while the text at the bottom needs absolute: HTML: <div id="content"> <div id="bottom-align"> Here is my bottom-aligned text </div> </div> CSS: #content { position: relative; border: 1px solid #000000; height: 300px; } #bottom-align { position: absolute; bottom: 0; }
-
Try something like this: HTML: <textarea name="my_area" id="my-area" cols="60" rows="20"></textarea><br /> <input type="button" name="selection" value="Bold" onclick="makeBold('my-area');" /> JavaScript: function makeBold(id) { var el = document.getElementById(id); if (!el.selectionStart) { if (document.selection.createRange().parentElement().tagName != 'TEXTAREA') { el.focus(); return false; } var r = document.selection.createRange().text; document.selection.createRange().text = '<b>' + r + '</b>'; } else { var txt = el.value; var before = txt.substring(0, el.selectionStart); var after = txt.substring(el.selectionEnd); var selection = txt.substring(el.selectionStart, el.selectionEnd); el.value = before + '[b]' + selection + '[/b]' + after; } el.focus(); }
-
Legal issues with linking to google maps!My Boss is driving me mad!
obsidian replied to scotchegg78's topic in Miscellaneous
OK, if she really is having that hard of a time understanding the EULA, here is the only line that pertains to business users: Notice that when the users get to Google Maps, they are considered individual users at that point, and they are bound by the EULA, not your business. Linking to the maps is like linking to an interesting article on a blog or news site: you are providing them with inbound traffic. -
[quote author=micah1701 link=topic=67269.msg838299#msg838299 date=1205328424] php didn't exist when i was 12. ::) (actually, no one knew what the Internet was when i was twelve either) but I did teach myself qbasic around that time. That was my first programing "language." [/quote] LONG LIVE QBASIC!!! ;)
-
Yes I did, I gave the link, you looked at it yourself. I'll try it again to get the divs to work. A link to code you are currently working on doesn't much aid in the debugging process when it's changing, though
-
You keep saying that these examples won't work, but you aren't sharing any code or examples of your implementation with us. I know for a fact these will work in both FF and IE. In fact, I just put this last example together in a sample layout for you to look at in action. This works as-is in both FF and IE6 (just tested). Feel free to pull it apart and do whatever you want with it: http://code.guahanweb.com/test_layout.html
-
Well, here's another way to be sure your center one is truly centered: HTML: <div id="left-col">asdfdf</div> <div id="right-col">adfasdf</div> <div id="center-col">asdfsdaf</div> CSS: #left-col { float: left; width: 100px; } #right-col { float: right; width: 100px; } #center-col { margin: 0 110px; } That way, you float your side columns and just put enough margin on your center content to assure that you clear your sides. Also, this centers your middle column, too.
-
Yes, the Collation column is the order (A is for Ascending), so you have none ordered descending. Also, Fenway is sort of our resident MySql guru, so if he happens to read this, I'd love to get his input to be sure I'm not steering you in the wrong direction, too. I am by no means a DBA
-
That's probably because you have a lot of margin on the divs. Reduce the margin on the facing sides until the combined total of the margin is equal to the distance you want between them. So, for instance, if you wanted 20px between the divs, your margin structure would be something like this: #left-col { margin: 20px 10px 20px 20px; } #center-col { margin: 20px 10px; } #right-col { margin: 20px 20px 20px 10px; } This way, the total margin between all elements is 20px.
-
Hmm... I don't think there is any way to get around that time with an existing table. You could either set up scheduled maintenance, or you could make it a little less invasive by creating the backup table, create the index on the empty table and then let a script run that will throttle the insert of all your friends records into the new table (REPLACE INTO works nicely for this type of thing). This will still take some time, but at least you would not have to be as worried about losing the data... You could even write the contents of the table out to disk and then do an import on the new table instead. The key is, when your index is already in place, each new record is indexed when it is inserted. Applying an index to a table of already 5mil records is just going to take a long time. As for viewing the current index structure, just run this query on the tables you want to examine (SHOW INDEX[/ur]): SHOW INDEX FROM friends;
-
Right. That little bugger actually specifically declares that it be applied to whatever it matches and overrule anything before or after in the cascading precedence. It can be useful occasionally, but more often it causes this type of headache
-
So, have you tried floating all your content/menu divs left and then putting a clear: both on the footer?
-
MySql CREATE INDEX is your friend. Try this out: CREATE INDEX custom_index_01 ON friends (x DESC, timestamp DESC); Then, when you force index, be sure to force it on "custom_index_01" or whatever you decide to call it. When you're dealing with this amount of data, it doesn't hurt to have multiple indexes on a single table in order to help your queries run more optimally. Keep in mind, though, that if you index the columns DESC like I have shown, it will help your current query, but if you ever want to order ASC, this index will not help much.
-
Like I said, the PHP gets processed before the markup hits your screen, so you can only process the data with PHP when the form is submitted to the receiving page. If you are wanting to assign the data to a PHP session variable onsubmit, you need to have a JavaScript call that retrieves the data and submits it via an AJAX request to the receiving script that will then assign it to your session. You cannot access PHP after your page has rendered.