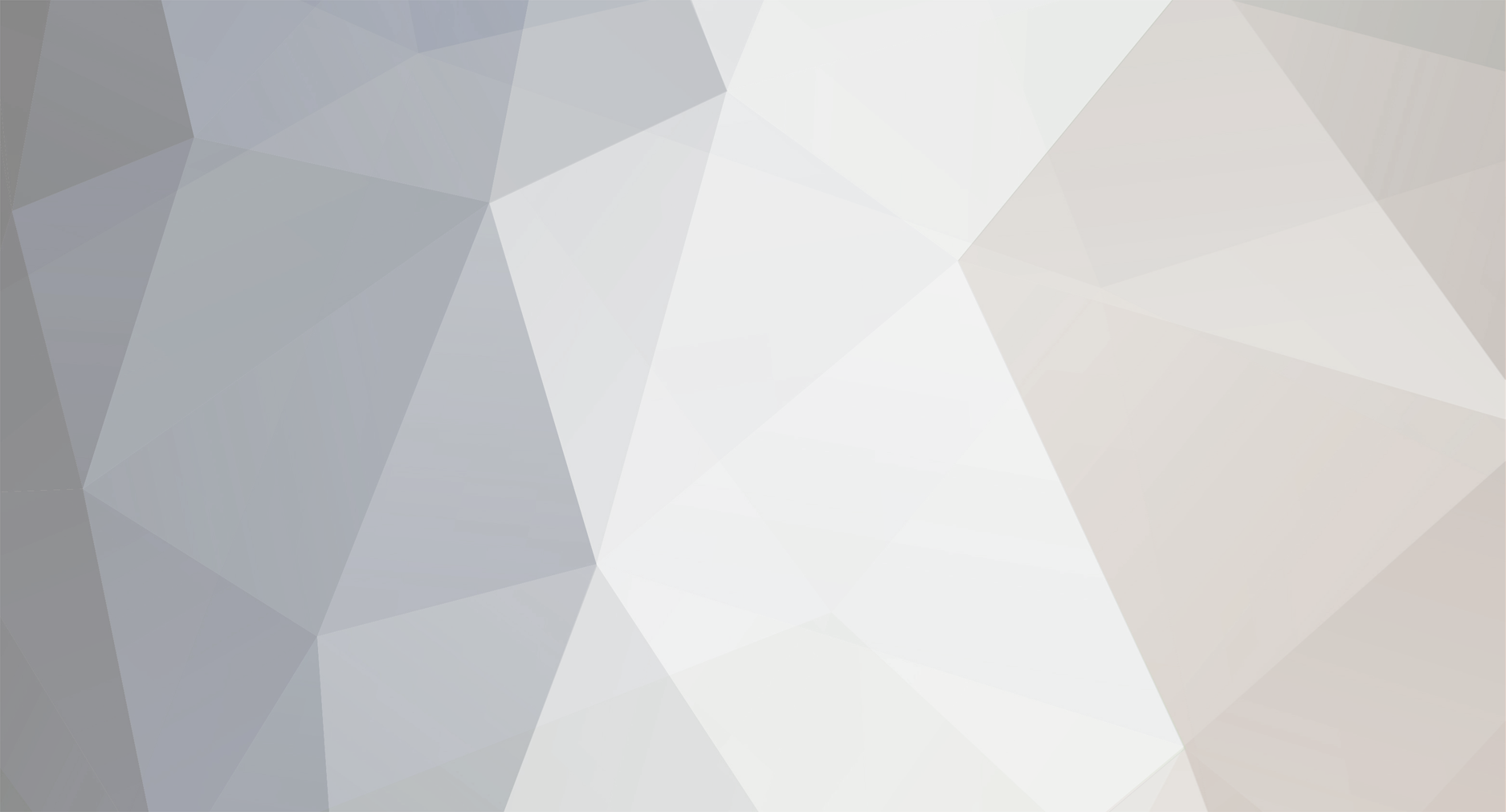
mogosselin
-
Posts
116 -
Joined
-
Last visited
-
Days Won
1
Posts posted by mogosselin
-
-
I found this function that would download an image, individually:
http://edmondscommerce.github.io/php/curl/php-save-images-using-curl.html
Or this tutorial that seems to download all images of one page. I didn't try it, but here you go:
http://snipplr.com/view/69459/
To find it, I simply searched for 'curl php get images in page'
-
@Beanmen : When you post code in the forum, you can use the 'code' button and paste your code in the popup that will appear, this way the code will have colour and will have a better format.
That being said, you should tell us:
- The example you provided only has HTML, no PHP code. Do you have PHP installed on your computer? Or do you plan to code directly on a web server, for example by uploading PHP files with FTP? If you don't have PHP installed, you should check out XAMP (for Mac) or WAMP (for Windows), it will install PHP on your machine for you.
- Do you want to create a contact form that will be send by email? It looks like this from your field names. If that's what you want, you can check out my tutorial: http://www.mogosselin.com/simple-php-contact-form/ (shamelessplug) or check Google for 'php contact form', I'm sure there is a ton of those.
-
Thank you for your answers.
1.
mogosselin, As you said that “returned value is something unexpected, you should kill your app”
Are you telling me that I need to check the return value?
2.
Before we return the value we are checking it then returning it.
How is it secure?
Well, you would need to check the value before you return it if you think it could have a value that it shouldn't return.
For example, if you return the column ID from a database, you probably don't need to check if it's a valid number.
But, if you want to return a value of a PHP function directly that could, in some situation, return something unexpected (like NULL, or false, or whatever)... Then yes, I would check the value before returning it.
If it's something unexpected, I would then kill my app (with throw new Exception() or trigger_error()) and display a friendly error message to the user (or display an error message in dev mode).
-
@ginerjm : If it's a standard small site/1 server setup, you're right, and that's what you assumed the OP has probably.
But, if you have more 'front servers' than 'DB servers', you could want to unload the DB. It all depends on your setup. I doubt that's what the OP has, but you never know
@gizmola : great post!
-
From what you said, something like 'web.config' equivalent would simply be a file that your PHP code would read.
There are no default/native application variables in PHP due to it's nature (how it runs requests, start its process, etc.). It's not like C# or Java where you can have application variables.
So, you could setup an ini file and read it with parse_ini_file
If it's a matter of performance, you'll need to setup distributed cache server like Memcached or Reddis, for example. But this is another ball game
-
Why do you need to process so many rows at the same time? To me, it looks like the wrong solution and it doesn't seems to scale really well. Do you need to process the rows one by one for a report or something like that?
-
At first glance, the only thing that I could say is that it's hard to 'read', IMO. Personally, I would add functions with names of what the code really does.
For example:
$k = str_replace( [ '\(\:any\)', '\(\:alnum\)', '\(\:num\)', '\(\:alpha\)', '\(\:segment\)' ], [ '(.+)', '([[:alnum:]]+)', '([[:digit:]]+)', '([[:alpha:]]+)', '([^/]*)' ], $k );
What is it doing?
Also, I don't like using variable with only letters (like $k), except when it's a counter. I would probably use $uriRule or something like that. But, this is maybe just a personal view.
I guess that it's not the kind of criticism you were looking for? Why are you 'concerned' with this piece of code? Performance?
-
So, what kind of return value are you talking about? A return value that you manage, something coming from a native function or an external library?
Anyway, if it's a function you control and the returned value is something unexpected, you should kill your app as soon as possible.
For example:
<?php function getUserInfo($userId) { $rows = getUserFromDatabase($userId); if (sizeof($rows) < 0 or sizeof($rows) >1) { // Here we try to get the logged in user information, but we get more than 1 results from the DB or we get 0, which is not normal trigger_error('Got 0 or more than 1 row for user info!', E_USER_ERROR); } return $rows[0]; } ?>
That way, you don't need to check if the value is valid at every points where you call this function.
Also, you could raise an Exception and try/catch it if you can gracefully recover from it.
-
Hello,
I tested it on my machine and it works great.
File #1: header.php at the root
File #2: index.php in a sub folder.
Here's the content of index.php:
<?php include('../header.php'); ?>
Are you sure you don't get a permission error or a syntax error? Did you check your logs?
Are you on Windows or Linux?
Alternatively, you could try this code:
<?php include($_SERVER['DOCUMENT_ROOT'] . "/header.php"); ?>
-
I would like to generate a constant value that change from a website to website but have an identical value for a single website.
Like the website URL?
$_SERVER['REQUEST_URI']
Like others said, it's not really clear what you want to acheive. Do you need something so that you can hardcode conditions in a development/test/production website?
-
One of the benefits of namespaces is that they prevent collisions. For example, you can use a code library that has a class named 'User' and you could also have a class named 'User' in your code base.
Also, I find that it's a nice way to organize your code and see if you know what you're doing. For example, if a namespace has 100 classes, it's a good sign that you probably need to do some refactoring.
And, it helps you see where the class that you use is coming from. An external library? Your own code? If so, where? Is it normal that you import a view class from a model class? etc...
That being said, use namespaces all.. the... time...
-
So, with Apache, you can use a file named .htaccess. You put that file in your directory where your PHP resides and this will gives Apache instructions.
Apache is responsible for 'forwarding' your request to the PHP 'engine'.
By default, all it will do is check the URL. If the filename ends with .php, it will try to tell PHP to execute that file.
But, with mod_rewrite (an Apache module), you can configure apache to change the URLs. For example, you can tell it to rewrite URLs with a certain pattern to another.
Here's a tutorial I found:
http://www.sitepoint.com/apache-mod_rewrite-examples/
Here's one I wrote myself (you can give me your comments if you read it):
http://www.mogosselin.com/friendly-url-for-php-with-apache/
If it doesn't work for you, you can query Google for 'php mod_rewrite tutorial' or 'php mod_rewrite example', etc.
-
Hello there!
To me, your description wasn't really clear. What is not working exactly? Displaying the right status 'Online' or 'Offline' at the right time? Which part of the code do you think doesn't work?
-
@vpetkovic Exactly. You'll have just one JS file with PHP variables in it. The PHP variable values will probably come from a database. So, if you need to change the logic in the JS file, you'll just have one file to change.
One way to do it cleanly would be to initialize the JS variables in the PHP script:
<script>
var variable1 = '<?= $var1 ?>';
var variable2 = '<?= $var2 ?>';
...
</script>
<script src="your_js_file_here.js"></script>
Then, all you need to do in your JS file is to use the variable1, variable2, etc...
Note that it could be not the 'super clean' solution in JavaScript to have that kind of global variables, but I'm not that experienced with JS. But I guess it's a start
-
'unexpected T_ENDWHILE' really means 'Dude, I see an 'endwhile' and I didn't expect it at all... what the hell is that?'. Sometimes, those error messages means that you are missing a ';' character at the end of a line and then PHP sees the next 'statement' (which is not a ';') and will spit this error.
But, like ParvinS said, in your case, you have two 'endwhile' and just one 'while loop'. PHP saw your last 'endwhile' and tought 'what the hell is that? I didn't see any 'while' and now you want to end it?'.
-
Or with path info, even better (as suggested by @ginerjm)
<?php $path_parts = pathinfo('/www/htdocs/inc/lib.inc.php'); echo $path_parts['dirname'], "\n"; echo $path_parts['basename'], "\n"; echo $path_parts['extension'], "\n"; echo $path_parts['filename'], "\n"; // since PHP 5.2.0 ?>
Code from the example in the official doc: http://php.net/manual/en/function.pathinfo.php
-
You'll need to separate the path of the file from the name of the actual file, and just change the name.
With the following method, you can get the file name only:
$filePath = '/usr/local/test.txt'; $file = basename($filePath); echo $file; // should give test.txt
And, with the following, you can extract the directory name:
$filePath = '/usr/local/test.txt'; $dir = dirname($filePath); echo $dir; // should give /user/local
-
Hello vpetkovic! Welcome to the wonderful world of web development
It's normal that you think about these kind of 'application designs' (or how you will program and structure your app) when you start up. But, it's not a good way to resolve these kind of problems.
Here's a couple of problems I can think of you will face if you continue with this kind of app design:
- You'll end up with a lot of duplications in your JS files (all the same code everywhere, only a bit of it changing)
- If you need to change your JS code, you'll need to change all of the JS files of every users.
- If you have a bug, you'll have a lot of fun trying to find what happens... Is it a bug in the JS file of this user? Are all the users affected? How will I correct this bug everywhere? Will I have to update one or more JS files?
- If you want to evolve your JS, you'll have problems maintaining all the new and old JS files
- If you want to store events or something like this, you'll need to write code that will parse and change your JS files, which won't be easy nor fun (lots of potential bugs, etc.)
Now, lets say that you want to accomplish something like this in JavaScript:
alert('Hello ...username...');
The text '...username...' should be replaced by the current logged in user.
A way to do it (without having to create new JS file for each users), would be something like this:
<?php $username = getUserName(); // some kind of function that gets the logged in user's name somewhere (like from a data base) ?> <script> alert('Hello <?= $username ?>'); </script>
Everything between PHP tags (<?php and <?=) will be executed and 'changed' on the server side, meaning it will become text and then this will be sent to your browser.
Lets say that the username is 'Louie', then this will be sent to your browser by the server (after executing the PHP):
<script> alert('Hello Louie'); </script>
Then, you browser will take this and execute the code between the <script> tags.
Remember, Javascript is executed by your browser, the PHP is executed by the server hosting the PHP files.
Does it make sense to you?
-
Ljike @Jacques1 said, you need to DELETE everything related to one user and his subscription, it's the easiest way to do it.
For example, imagine that I have a table with these entries:
idUser / idSubscription
1 - 1
1 - 2
1 - 3
2 - 2
3 - 1
3 - 3
So, it basically means that the user #1 is subscribed to the feed #1, 2 and 3.
The user #2 subscribed to the feed #2 only.
And the user #3, subscribed to the feed #3.
Now, imagine that you are logged in as the user #2 and you have those checkboxes on the page:
Feed #1 [ ]
Feed #2 [X]
Feed #3 [ ]
The Feed #2 is already 'ticked'. But lets say that I want to subscribe to the Feed #3 also, I'll get this form:
Feed #1 [ ]
Feed #2 [X]
Feed #3 [X]
And that's what I'm going to submit to the server.
Then, on the server side in your PHP code, you loop trough all the checkboxes you received and try to insert all of them. When you get to Feed #2 for the current user, you get this error message because the entry 'user #2 / feed #2' already exists in the subscription table.
Now, before inserting the data, you could check if it already exists. Imagine something like this:
For each subscription received
Check if already exists
If not, insert the new row
This would work, but it's not efficient. The easiest and fastest way would be something like this:
Delete every subscriptions for user #2
For each subscription received
Insert new subscription
So, you have to run this query first:
DELETE FROM member_feed WHERE member_id = 2
Then, after this, you can loop trough all the subscription and insert them again.
-
Do you have any JavaScript in your 'form' page?
What I would do would be to create a simple, mostly empty, HTML page with your needed form (without any code) that posts to your PHP file. Then, 'echo()' the string that was cut.
If it works, the problem is with the 'client side script, meaning probably some JavaScript that does something weird before posting the data.
If this doesn't work, the problem is not the client, you'll then know that you need to search on the server or 'in between' (a proxy, a browser pluging, something else).
Did anybody else tried your form or only you? Did you try on different browsers and computers?
-
The error probably comes from the fact that your condition isn't true:
if(isset($_POST['invite'])){ foreach ( (array)$subs as $subscribed_feed) { $subscription_stmt->execute(array( 'member_id' => $_SESSION['member_id'], 'feed_id' => $subscribed_feed )); } }
What this IF does, is it check if 'invite' (which is an index), exists in the array $_POST. My guess is that when you post your form, it's always false anyway.
do a var_dump($_POST) to see what's inside the $_POST variable. If 'invite' never exists, that's your problem. Also, checks if the code goes into your 'foreach'. You could do a var_dump($subs) just to be sure.
-
Here's an example of what your 'contextData' array should contain in the header:
$contextData = array( 'method' => 'POST', 'header' => "Content-type: application/x-www-form-urlencoded\r\n [other header stuff here...]", 'content' => $postdata );
-
This is an example with mysqli_ + OOP style:
<?php define('DB_SERVER', "localhost"); define('DB_USER', "user"); define('DB_PASSWORD', "password"); define('DB_TABLE', "db_name"); $conn = new mysqli(DB_SERVER, DB_USER, DB_PASSWORD, DB_TABLE); if ($conn->connect_error) { trigger_error('Database connection failed: ' . $conn->connect_error, E_USER_ERROR); } $query = "SELECT * FROM users"; $result = $conn->query($query); if($result === false) { trigger_error('Wrong SQL: ' . $query . ' Error: ' . $conn->error, E_USER_ERROR); } else { $result->data_seek(0); while($row = $result->fetch_assoc()){ echo $row['username'] . '<br>'; } } $result->free(); $conn->close(); ?>
Here's an example with mysqli_, procedural style:
<?php define('DB_SERVER', "localhost"); define('DB_USER', "user"); define('DB_PASSWORD', "password"); define('DB_TABLE', "db_name"); // The procedural way $mysqli = mysqli_connect(DB_SERVER, DB_USER, DB_PASSWORD, DB_TABLE); if (mysqli_connect_errno($mysqli)) { trigger_error('Database connection failed: ' . mysqli_connect_error(), E_USER_ERROR); } $query = "SELECT text AS txt FROM `table_test`"; $result = mysqli_query($mysqli, $query) or trigger_error("Query Failed! SQL: $query - Error: ". mysqli_error($mysqli), E_USER_ERROR); if($result) { while($row = mysqli_fetch_assoc($result)) { echo $row['txt'] . '<br>'; } } mysqli_close($mysqli); ?>
This is an example with PDO:
$db = new PDO("server", "username", "password"); $statement = $db->prepare("select * from users"); $statement->execute(); $row = $statement->fetch();
- Please consider specifying the names of the fields instead of SELECT *. This is a, generally, a bad practice to use * (security & performance wise).
- As it's already been said, don't use mysql_ but mysqli_ or PDO.
-
Hello Oxfo7d!
Your code is a little bit hard to read because there are no indentation.
But, I've seen that you seems to do SQL queries in a loop:
$res1 = mysql_query("SELECT * FROM `places` ORDER BY `name` desc"); while($row1 = mysql_fetch_array($res1)) { ... }
So all the queries will inside the while will be run for each places. How many 'places' do you have? Because it looks like you have about 15 queries with sub selects (SELECT * FROM (SELECT ...)) which are not the most performant type of queries you could do.
I think that's mostly your problem. You should try to do less queries and less queries with sub-select. 11.5k rows isn't big at all, it shouldn't even be a problem.
php sitemap script help - Last Mod Function not working properly
in PHP Coding Help
Posted
The code that doesn't work seems to be in this part:
First, it will try to use filemtime(). If it doesn't work, it'll try filectime. If filectime doesn't work, well, that's what you'll get anyway.
So, just try to debug your code and see what happens. Is it going to the 'if' or the 'else'?
The 'if' would be if the first filemtime() doesn't work. If it goes there, try to output the $mod variable and see what it is.
If it goes on the 'else' try to output the $mod variable.
Then, tell use what happens