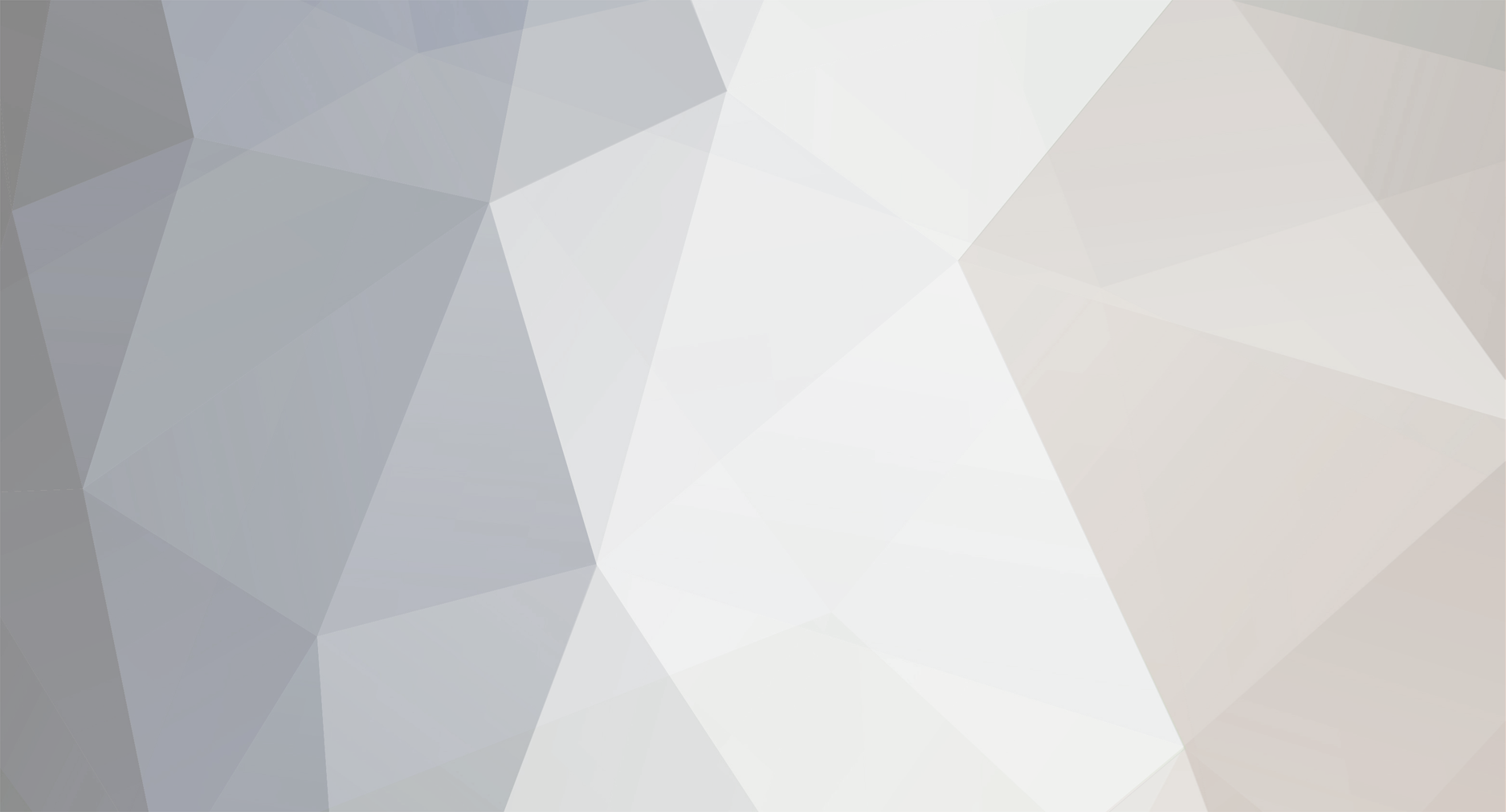
mogosselin
-
Posts
116 -
Joined
-
Last visited
-
Days Won
1
Posts posted by mogosselin
-
-
Did you check the errors in the PHP log files?
Did you try to debug the HTTP headers you get? If you don't know how, check out this page : https://developer.chrome.com/devtools/index
In chrome, just check the Network tab of the developer tools, click on the filter icon and choose "documents". When you load your page, your should see your "Location: ..." in the "headers" tab. If not, it didn't get there somehow.
If you're not sure about php.ini, you can add this line to your code:
ini_set('error_reporting', E_ALL);
Just be sure to remove it when you put your code online.
-
Try using trigger_error() instead of "echo" when you have an error. Because when you echo errors like that and an error occurs, the app will be in an unknown states and the code will continue anyway, meaning that you'll get a bug latter that is harder to find.
And, it's also possible that the "echoed" error message won't be visible, depending on where it is printed in the HTML code.
Try to print the value of the $db array that is initialized with the file "contra.conf", I suspect that there is an error there... And change that "echo "Failed to connect... " for a trigger_error.
You could also add this at the end of the _db() method or just before calling $this->_db->query($sql). That way you'll see if it's an object or a variable (probably holding the value false from an error somewhere?)
if (!is_object($this->_db)) { trigger_error("DB isn't an object!", E_USER_ERROR); }
-
Hi! It looks like a specific problem with PHPmotion. Did you try to ask in their forum at http://www.phpmotion.com/forum/ ?
-
Hi bbalet! Seems to me that you know what you're doing!
I looked at the code a bit and the only thing I could say is that some methods are really long.
For example, this one : https://github.com/bbalet/lms/blob/master/application/models/leaves_model.php / get_user_leaves_summary
The first step to break it up would be to make a new method where you put a comment.
For example, where you put the comment "//Fill a list of all existing leave types", you could have a method named "fillList()" and another one "getLeavesExistingTypes()" or something like that. Dunno if it make sense there but you know what I mean.
Remember, the first rule is that methods should be small. The second rule is that methods should be smaller than that (from the book Clean Code)
-
Hey Miles!
IMO, you should learn HTML, CSS and a bit of JavaScript if it's not already the case. Then, (again IMO), you should try to make a simple app with vanilla PHP. Something like a really basic blog, or something like that. Just to see how everything works. Then, when you feel comfortable, go for a framework.
-
You can join W3School forum and also vist their site to improve your programming skills.
Beware of w3schools, it's often outdated and are not associated with the w3c. check out http://www.w3fools.com/
-
Hi, you could try something like that with mysqli.
I used mysqli prepared statements to prevent SQL injections.
As for the dynamic table name, you should check it against a white list of allowed tables, again to prevent SQL injections.
<?php define('DB_SERVER', "localhost"); define('DB_USER', "root"); define('DB_PASSWORD', "123"); define('DB_TABLE', "db_test"); $conn = mysqli_connect(DB_SERVER, DB_USER, DB_PASSWORD, DB_TABLE); // check valid tables where you could insert something. You want to prevent SQL injections somehow... $tables = array("table1", "table2", "table3"); $table = $_SESSION["use"][0]; if (!in_array($table, $tables)) { trigger_error('Invalid table from session: ' .$table, E_USER_ERROR); } // The 2 ? will be replace by actual values, we call this a prepared statement. It will prevent SQL injections $query = 'INSERT INTO `'.$table . '` (ID,Amic) VALUES (?, ?)'; if (mysqli_connect_errno($conn)) { trigger_error('Database connection failed: ' . mysqli_connect_error(), E_USER_ERROR); } if ($stmt = mysqli_prepare($conn, $query)) { $value1 = $_SESSION["person"][0]; $value2 = "1"; mysqli_stmt_bind_param($stmt, "ss", $value1, $value2); mysqli_stmt_execute($stmt); mysqli_stmt_close($stmt); } else { trigger_error('Error with SQL query' . $query, E_USER_ERROR); } mysqli_close($conn); ?>
-
Really weird behavior. I never experienced that bug before. If you have a smple code, it would be nice
-
Well, the problem with starting from nothing to PHP is not that PHP is hard to learn, it's that you start from so far and a "web language" comes with everything around it:
- HTML
- JavaScript
- CSS
- Sessions
- The HTTP protocol
- Cookies
- etc...
So maybe you should learn how to make normal static websites first. I wrote an article a couple of weeks ago that could help you to make sense of all this : http://www.mogosselin.com/learning-php-real-starting-point/ (/shameless plug)
-
I read a lot of good reviews of Larry Ullman's book from beginners. I didn't read them myself, but if you check them on amazon, you'll see that the reviews are mostly postiives one.
And, if you want to learn to code better, I highly recommend "clean code". It's not a PHP book, but you'll learn a lot from it. Every developer should have to read it before coding anything IMO
-
Hi Ben!
I read a lot of good reviews about Larry Ullman's books! Since they are mostly beginners book, I didn't need to read them, but I would like to know what's so good about them?
And, as for PHP, I think that you made the right choice. Honestly, all the stuff around PHP (HTML, JavaScript, CSS, etc.) you'll need to learn isn't lost. When you'll feel comfortable with PHP, learning another web language like Java, Ruby, etc, will be 10x easier than the first web language you learn because you have to deal with everything around.
Good luck!
-
Hi! In your courses, do they teach you about HTML, HTTP, websites, etc? Or is it "basic" programming? What languages are you studiying?
-
Hi, and welcome! I hope you'll have fun learning PHP
Do you already know HTML, CSS, etc?
-
@mrfdes
http://www.phptherightway.com/ isn't a tutorial, it's more of stuff that you should be careful with or know when using PHP.
It's just like if you started to paint a house. A tutorial would be "How to paint a wall with a brush".
And "painting the right way" would be more like : Use a brush #34 with Xyz type of paint. Be careful not to use the ABC outdated paint. You could also use a paint roller. etc.
You'll still need to do some reasearch and read tutorials, but at least you know the pitfalls and what to be careful with
-
Or, you could do like @Jacques1 said, but without the Ajax call. If you already have the data when the page is requested, you could:
- Transform your PHP into an array
- Transform the array into json using json_encode()
- Use JQuery.parseJson() to create a JS object
It depends if you need the info right away a later on click or something.
I found this link that states the pros and cons of both methods (plus another one):
http://stackoverflow.com/questions/23740548/how-to-pass-variables-and-data-from-php-to-javascript
-
You can also use mysqli instead of PDO if it's too confusing to you. PDO is more "object oriented" and if you didn't already learn that, it could be confusing. Anyway, that you use one or the other, don't forget to use "prepared statements".
The "quotes" and potential problematic characters will be dealt with.
Good luck with your application!
-
Being a newbie I'll explain my side:
2000 lines of code; as keeping to simplified thigs like else, if, else if is easier than creating and learning custom functions.
I use HTML and PHP as I didn't know there was anything wrong with it?
<html>
<title>Example</title>
<body>
<h1>Example</h1>
<php
Echo "hello world"
#insert php script
<?
</body>
</html>
What is wrong with this other than my bad syntax and bad spelling?
The other points are great. Even I don't do that. I guess because lack of knowledge and/or care of their work?
What is wrong with this way of doing thing? The thing is that it could be OK if you know when this is good and when this is bad. Most of the beginners seems to think that this is the only way.
If you make a small file of 40 lines and that's it, who cares. But if you try to make a real application with a DB behind, a login, users, authentication, etc etc... This is when it will get messy and hard to debug.
-
I'm not here to criticize anybody, I'm just trying to understand why a lot of beginners are using a lot of bad practices, especially obvious ones. BTW, I'm not telling that every beginners use bad practices or all of the bad practices around.
Anyway, I've been helping in a couple of forums and other websites since 3-4 months now and I always see the same bad practices used by beginners, and I don't understand why they use that kind of coding style.
I'm talking about:
- Writing a PHP file of 2000 lines without any functions (so it's like basically like writing a 2000 lines function)
- Mixing HTML with PHP, PHP with HTML
- Not using a debugger to debug their code
- Not knowing how to use the official doc (or not knowing about the official doc at all)
- SQL Injections
Is it because they don't know about those practices?
Is it because they don't understand it?
Or maybe all the tutorials they used to learn are crap (or just written to provide examples, not good practices)?
Or is it just because they are beginners and it's the "normal" way of learning things?
Is there something we can do about it?
Like I said, I'm not trying to discourage anyone or judge anybody, I'm just trying to understand so that I can help those beginners better
By the way, I probably programmed like this at the beginning, but it was more than a decade ago, so I don't really remember
P.S. I wasn't sure where to post it, thanks for moving it to the right place
-
Welcome! It's really great that you're starting this young. And you're also lucky that "nerds" and "geeks" are more popular than they were when I was your age
Good luck with your coding
-
Did the code you had worked and just stoped working for an unknown reason? Or is it "new code"? Or did you change your server or some other configurations?
The only thing I can think of is checking the upload file limit in your php.ini if you're uploading the file to your server before "ftp uploading" it elsewhere. If you do upload a file to your server before "ftp-ing" it and there is an error, the filename will be empty.
-
Well, nobody is paid here to answer questions (contrary to that farmer). We are doing it to help people and for fun. And learning to code has nothing to do with hiring somebody and making him make the job at your place. If you want somebody to do all the job at your place, you're going to have to hire somebody.
That being said, if you want to learn to develop web applications, it seems that you are stuck at the beginning. What you probably want to do is to check how PHP works with MySQL. There are a lots of books and tutorial out there. If you prefer a book, I suggest that you check Amazon and search for PHP Mysql. If you prefer free tutorials, Google something like "php and mysql tutorial". Be sure to add a filter by date, you don't want to get tutorials of 10 years ago.
What is your goal with this application? Learn how to code for fun? You want to become a professional web developer?
-
You just need to check if the use exists, first. Add a method that just check that. For example:
function userExists($id) { .... if (!empty($rows)) { return true; } return false; }
I don't know what is the framework you use so you might have to change the line with "!empty($rows)"...
Then, you'll be able to do something like this:
if (userExists($userid)) { ... transfer money... } else { ... error ... }
Some free tips:
I think that you will have to read a little bit about SQL injections (one of my post I made).
Also, I think that an MMO RPG is a really ambitious project to make for a beginner. Is it 100% web based? If you're going to make a big application, you should also read about good practices, specifically how to sperate your presentation from your code. It's really hard to maintain and read code that has HTML mixed in with PHP (here's a link on stack overflow: http://stackoverflow.com/questions/18641738/how-i-separate-logic-from-presentation).
-
For the single sign on, there are a couple of ways you could do that, depending on how your 2 websites are made and also where they are (both on Azure?).
And, where is your AD server?
-
What do you mean by "placeholder"? Do you mean the default text that is displayed in an input field? If yes, you can use the property "placeholder" in an input field to display default value. It won't be displayed if the value is not empty. For example:
<input type="text" value="" placeholder="Default value">
If something exists in value="", then the value inside placeholder won't be displayed. Since you have labels besides your form, you don't need a place holder.
Note : You could use the <label> tag for your form labels. The way it works is something like this:
<label for="email">Your Email</label> <input id="email" value="" name="email" placeholder="email@company.com">
The "for" attribute of the "label" tag indicates the "id" of the tag the label is form (in this case, "email"). This is better for accessibility.
Spelling Suggestor like google's did you mean
in PHP Coding Help
Posted
Check out Solr or Elastic Search. They probably have components or plugin for that.