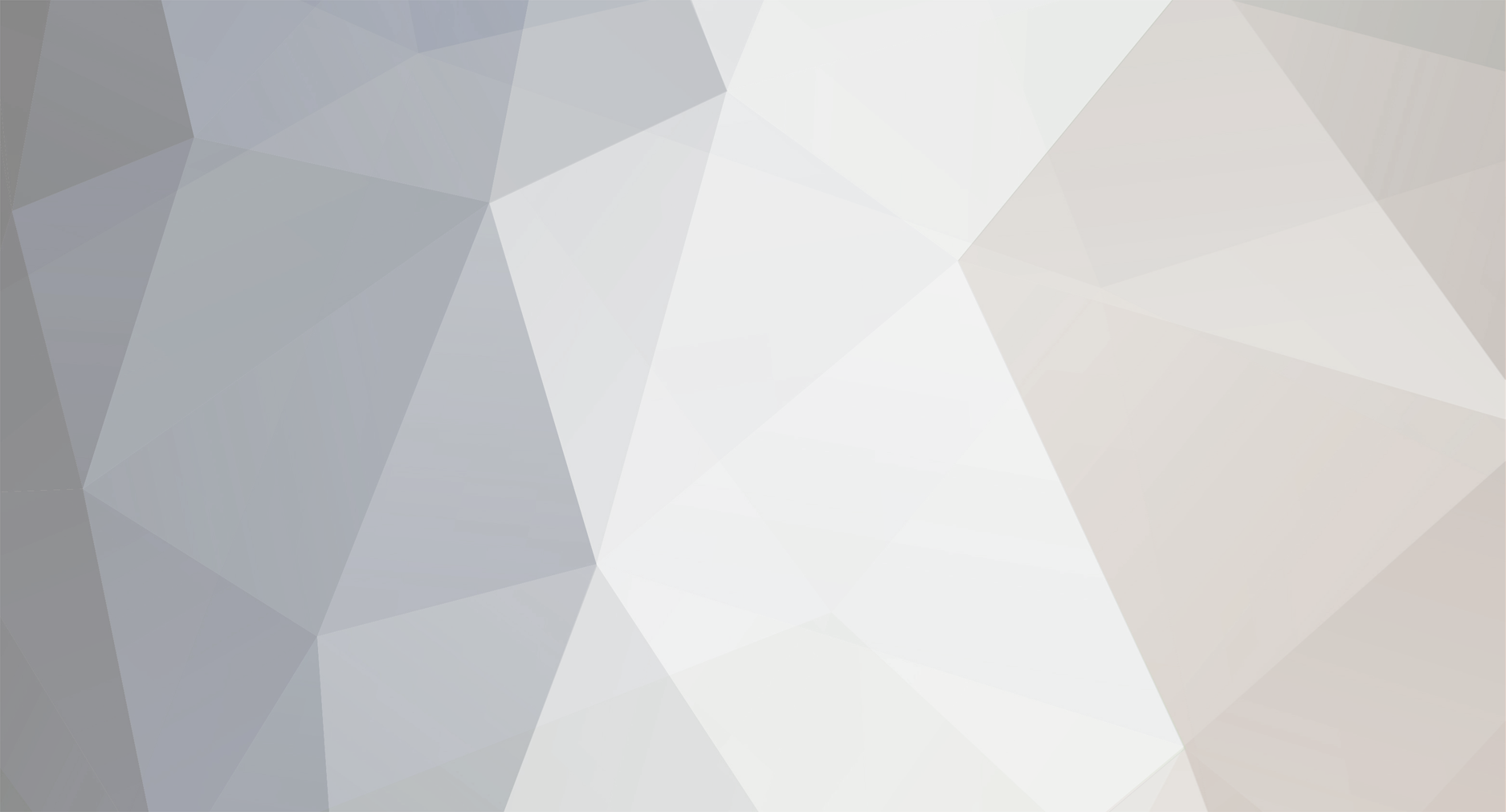
Supervan
-
Posts
52 -
Joined
-
Last visited
Posts posted by Supervan
-
-
Also, the PDOExceptions would probably be more useful info than your custom ones.
I think i must use a combination of messages. End-user messages and system PDOExceptions thats get directed to the admins
-
Your call to a 'query' function is not going to return an exception, as boompa tried to say. The 'query' function of yours returns the object (??). Try adding your exception check to the actual query execution statement if you want to see the exception.
Kind of a lot of code to perform a simple pdo query.
Hi, could you please help me to simplify my code.
Using 2 classes
1. Db Class...........public function query
2. User class ...............public function updatename
-
Assuming the query function being called is the one you posted (which doesn't necessarily make sense because it looks like both of these functions are in the same class, but you're invoking the query function on $this->_db rather than $this), you are returning $this from your query function, which shouldn't be falsy.
Thanks...I should have mentioned...
Using 2 classes
1. Db Class...........public function query
2. User class ...............public function updatename
-
You're not getting YOUR exception message or you are not getting the exception error message?
Thanks for responding.
Im not getting "throw new Exception('There was a problem updating.');"
-
Hi, please help.
I deliberately made a mistake in my query… can’t get the Exception to display an error message.
public function updatename($name = null, $id = null) { if (!$id && $this->isLoggedIn()) { $id = $this->data()->id; } $parms = array(); $parms[] = array(':name', $name, PDO::PARAM_STR); $parms[] = array(':id', $id, PDO::PARAM_INT); if (!$this->_db->query("UPDATE users " . "SET name = :this_variable_not_found" . " WHERE id = :id", $parms)) { throw new Exception('There was a problem updating.'); } } public function query($sql, $data_in = array()) { $this->_error = false; if ($data_in) {// prepared query $this->_query = $this->_pdo->prepare($sql); // this example extends the pdo class foreach ($data_in as $arr) { if (isset($arr[2])) {// type supplied $this->_query->bindValue($arr[0], $arr[1], $arr[2]); } else {// no type supplied $this->_query->bindValue($arr[0], $arr[1]); // defaults to string type } } if ($this->_query->execute()) { $this->_results = $this->_query->fetchAll(PDO::FETCH_OBJ); $this->_count = $this->_query->rowCount(); } else { $this->_error = true; } } else {// non-prepared query $this->_query = $this->_pdo->prepare($sql); if ($this->_query->execute()) { $this->_results = $this->_query->fetchAll(PDO::FETCH_OBJ); $this->_count = $this->_query->rowCount(); } else { $this->_error = true; } }// code to retrieve the result from the query.... return $this; }
-
I not fond of repetition in code, any way of using abbreviation shortening the array code.
':id',$idnr,INT
':name',$name1,STR
$parms = array(); $parms[] = array(':id',$idnr,PDO::PARAM_INT); $parms[] = array(':name',$name1,PDO::PARAM_STR);
Im trying to create a generic class that I can use with all my queries CRUD.
Can someone please assist.
$idnr = 123; $name1 = "tom"; $parms = array(); $parms[] = array(':id',$idnr,PDO::PARAM_INT); $parms[] = array(':name',$name1,PDO::PARAM_STR); $users = DB::getInstance()->query("SELECT * FROM users WHERE id = :id AND name = :name",$parms); class DB { private static $_instance = null; private $_pdo, $_query, $_error = false, $_results, $_count = 0; private function __construct() { try { $this->_pdo = new PDO('mysql:host=' . Config::get('mysql/host') . ';dbname=' . Config::get('mysql/db'), Config::get('mysql/username'), Config::get('mysql/password')); // echo "connected"; } catch (PDOException $e) { die($e->getMessage()); } } public static function getInstance() { if (!isset(self::$_instance)) { self::$_instance = new DB(); } return self::$_instance; } public function query($sql, $data_in = array()) { $this->_error = false; if ($data_in) {// prepared query $this->_query = $this->_pdo->prepare($sql); // this example extends the pdo class foreach ($data_in as $arr) { if (isset($arr[2])) {// type supplied $this->_query->bindValue($arr[0], $arr[1], $arr[2]); } else {// no type supplied $this->_query->bindValue($arr[0], $arr[1]); // defaults to string type } } if ($this->_query->execute()) { $this->_results = $this->_query->fetchAll(PDO::FETCH_OBJ); $this->_count = $this->_query->rowCount(); } else { $this->_error = true; } } else {// non-prepared query $this->_query = $this->_pdo->prepare($sql); if ($this->_query->execute()) { $this->_results = $this->_query->fetchAll(PDO::FETCH_OBJ); $this->_count = $this->_query->rowCount(); } else { $this->_error = true; } }// code to retrieve the result from the query.... return $this; } public function results() { return $this->_results; } public function first() { return $this->results()[0]; } public function error() { return $this->_error; } public function count() { return $this->_count; } }
-
This is untested, but should work:
$parms = array( array( ':id', $idnr, PDO::PARAM_INT ), array( ':name', $name1, PDO::PARAM_STR ) );
Of course, whether it's actually shorter or not is up for debate....
thx
-
The original is simplest in my view, however a wrapper class for this specific query could look like this (untested):
class my_xyz_query{ private $id = null; private $name = null; private $parms = null; public function__construct($name="",$id=""){ $this->parms=array(); $this->parms[] = array(':id',$id,PDO::PARAM_INT); $this->parms[] = array(':name',$name,PDO::PARAM_STR); } public function set_name($name){ $this->name=$name; } public function set_id($id){ $this->id=$id; } public function get_parms(){ $this->parms[0][1]=$this->id; $this->parms[1][1]=$this->name; return $this->params; } }
thx
-
Thanks for your time and feedback. Trying to create generic classes for CRUD operations...
-
Hi,
Im using OOP and PDO prepared statement.
Will it be possible to construct a class and shorten the following 3 lines of code, the array portion of the code.
A lot of repetition.$parms = array(); $parms[] = array(':id',$idnr,PDO::PARAM_INT); $parms[] = array(':name',$name1,PDO::PARAM_STR);
Code Sample
$idnr = 123; $name1 = "tom"; $parms = array(); $parms[] = array(':id',$idnr,PDO::PARAM_INT); $parms[] = array(':name',$name1,PDO::PARAM_STR); // then use the $parms array as the second parameter in your query calling statement - $users = DB::getInstance()->query("SELECT * FROM users WHERE id = :id AND name = :name",$parms);
-
Thanks to all, who have commented... OOP gives structure to php and code reuse ability
-
Hi
I’m looking for a standalone PHP OOP framework or code that follows best practices
using
- PDO prepared statements- Singleton Design Pattern
Not looking for a massive library, something short and sweat straight to the point
Any comments, feedback would be appreciated
-
Thank you all, greate help.
-
It's still not clear what you want. What does JavaScript insertion have to do with database queries?
If you want to somehow magically “clean” the user input before you store it in the database, this is neither possible nor sensible. Cross-site scripting is an output problem, not an input problem. You leave the input as is when you store it in the database. But when you output the data, you escape it for the specific context.
As a concrete example: Let's say you have a form on your site where people can enter an arbitrary comment. When you store the comment, you don't do anything with it. No escaping, no “sanitizing” (whatever that means). You just store the raw text. But when you put this comment into your HTML page, you escape it for the specific target context. For example, if the comment is supposed to go into a simple HTML element like div or p, you need htmlspecialchars().
It's very important to understand that escaping is done when you use the data, not when you receive or store it. You should avoid terms like “sanitizing”, because they carry the idea that you could just take the input, apply some magical cleaning function and then safely use the result in any context. This is wrong.
Ok, how would I protect my site's output from Cross-site scripting?
Some example code would be appreciated.
-
Hmm.. I see what you mean. To be honest I haven't heard of either of those and didn't even know things like that were out there. I'll definitely look into them.
Do you mean create the insert query (or whatever it happens to be) outside of the class, and then inside the class do something like this:
$stmt = $dbh->prepare($sql);
Is it better practice to do it that way?
look at this online training, regarding oop
http://www.youtube.com/playlist?list=PLfdtiltiRHWF5Rhuk7k4UAU1_yLAZzhWc
-
No, it doesn't. It escapes “<”, “>”, “&”, single and double quotes and a whole lot of harmless characters.
If you need anything else, you're doing it wrong. Are you trying to insert user input into an existing script element? This is simply wrong and mustn't be done at all.
Hi
Im trying to sanitize user input, i bit corncerned about java script insertion.
Using pdo and prepared queries.
Thanks
-
Hi,
This escape function will only block quotes
How would you stop java script insertion?
function escape($string) { return htmlentities($string, ENT_QUOTES, 'UTF-8'); }
Thanks
-
Good to know.. thanks
-
The error im getting.
2002 An attempt was made to access a socket in a way forbidden by its access permissions.If i allow access to sourceforge it then works... I dont like this...
:o
-
Please help.
The latest Wampserver (64 bits & PHP 5.5) 2.5 requires authentication on SourceForge before you can open phpmyadmin.
Does anyone know why is this required. I blocked access to the internet and phpmyadmin would not start.
Any ideas?
Thanks
-
Hi,
Any other way to import the native xlsx excel file into PHPmyadmin.
I know this function was supported in the older versions prior to phpMyAdmin 3.4.5.
I don't want to convert the excel file to csv format.
Thanks -
the array structure would be - array(array(':id',$idnrr,PDO::PARAM_INT),array(':name',$name1,PDO::PARAM_STR))
or more clearly -
$parms = array(); $parms[] = array(':id',$idnrr,PDO::PARAM_INT); $parms[] = array(':name',$name1,PDO::PARAM_STR); // then use the $parms array as the second parameter in your query calling statement - $users = DB::getInstance()->query("SELECT * FROM users WHERE id = :id AND name = :name",$parms);
This worked like a charm... Thank you so much.
Now I need to try and solve the rest. Update/ Insert and delete..
-
I really appreciated your help. I tried, but missing something.
Error... Warning: PDOStatement::bindValue() expects parameter 3 to be long
$idnrr = 1; $name1 = "tom12345"; $users = DB::getInstance()->query("SELECT * FROM users WHERE id = :id AND name = :name", array(':id'=>$idnrr,PDO::PARAM_INT,':name'=>$name1,PDO::PARAM_STR)); if ($users->count()) { foreach ($users->results() as $result) { echo $result->name . "<br />"; } }
class DB { private static $_instance = null; // use $_ notation for private private $_pdo, $_query, $_error = false, $_results, $_count = 0; public function query($sql, $data_in = array()) { $this->_error = false; if ($data_in) {// prepared query $this->_query = $this->_pdo->prepare($sql); // this example extends the pdo class foreach ($data_in as $arr) { if (isset($arr[2])) {// type supplied $this->_query->bindValue($arr[0], $arr[1], $arr[2]); } else {// no type supplied $this->_query->bindValue($arr[0], $arr[1]); // defaults to string type } } if ($this->_query->execute()) { $this->_results = $this->_query->fetchAll(PDO::FETCH_OBJ); $this->_count = $this->_query->rowCount(); } else { $this->_error = true; } } else {// non-prepared query $this->_query = $this->_pdo->prepare($sql); if ($this->_query->execute()) { $this->_results = $this->_query->fetchAll(PDO::FETCH_OBJ); $this->_count = $this->_query->rowCount(); } else { $this->_error = true; } }// code to retrieve the result from the query.... return $this; } public function results() { return $this->_results; } public function first() { return $this->_results[0]; } public function error() { return $this->_error; } public function count() { return $this->_count; } }
-
imo, it doesn't matter if you use named place holders or ? place holders. you have to keep track of how many, what they are used for, and supply an input parameter for each one.
btw - your existing code will work for either type of place holder. for a ? place holder, your query calling statement would become - $users = DB::getInstance()->query("SELECT * FROM users WHERE id = ?", array($idnrr));
however, your current method of supplying the parameters in the ->execute($params) method won't work for things like LIMIT x,y clauses (i.e. you are dynamically getting the y value from the user), because using ->execute($params) treats all the parameters as strings, which produces a sql syntax error when used in a LIMIT clause.
a general purpose solution would need a way of (optionally) supplying the input type (in each element in your $params array) and would specifically bind each input by looping over the elements of the $params array. see the following example -
public function query($query,$data_in=array()){ if($data_in){ // prepared query $stmt = parent::prepare($query); // this example extends the pdo class foreach($data_in as $arr){ if(isset($arr[2])){ // type supplied $stmt->bindValue($arr[0],$arr[1],$arr[2]); } else { // no type supplied $stmt->bindValue($arr[0],$arr[1]); // defaults to string type } } $stmt->execute(); } else { // non-prepared query $stmt = parent::query($query); } // code to retrieve the result from the query.... }
to specifically supply an integer data type, the $params array entry, corresponding to your supplied example, would look like - array(':id',$idnrr,PDO::PARAM_INT). to use a ? place holder, this would become - array(1,$idnrr,PDO::PARAM_INT)
Thanks for your help..
How would I remove an instance of a class inside a array
in PHP Coding Help
Posted
How would I remove an instance of a class inside a array
The content is stored inside an array. and the array inside $_SESSION ['cart']
I will have have Multiple instances of $item inside the array. Cant use unset because its protected