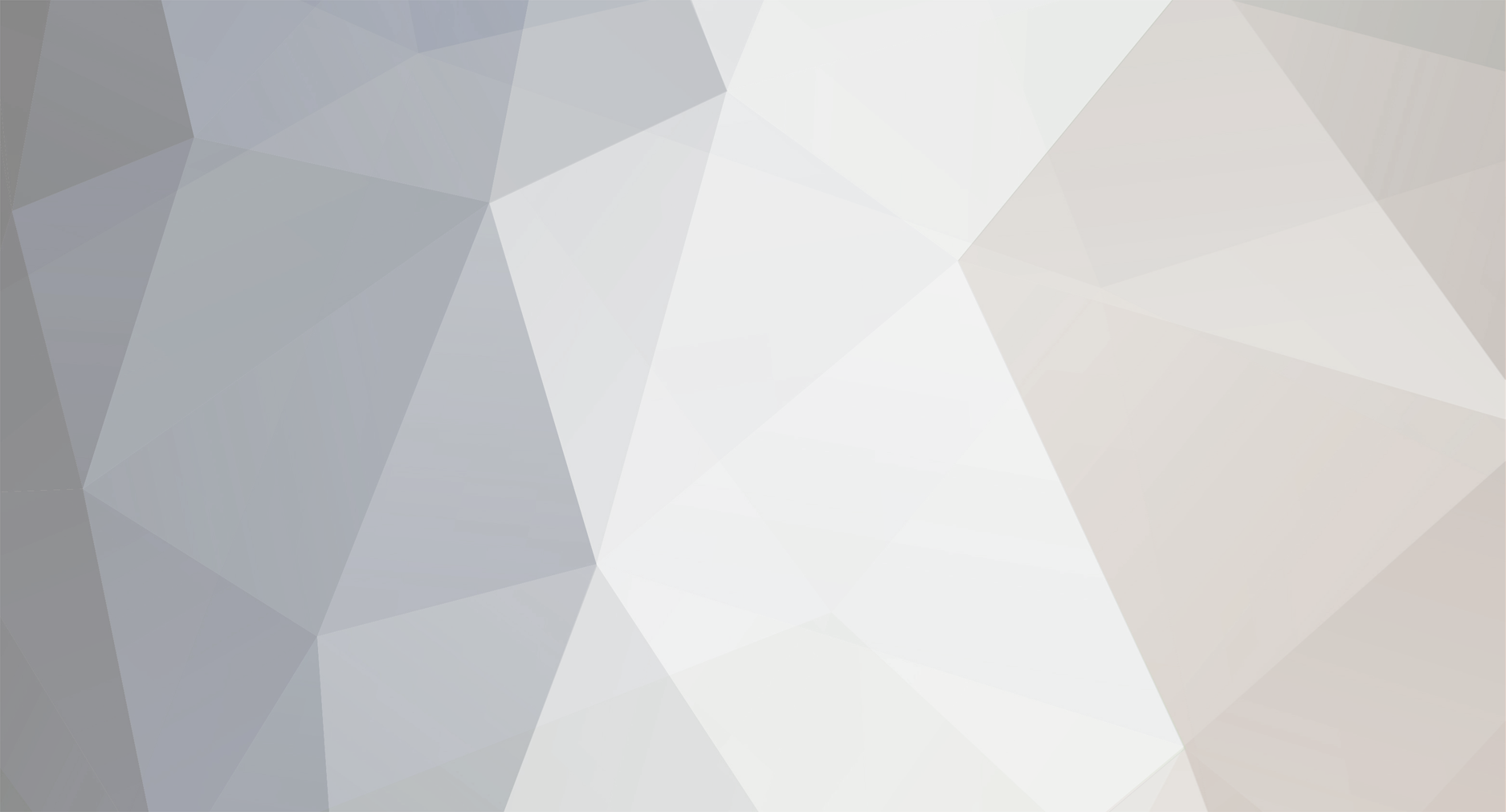
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
I can help yes. However I need to know the output of the code I provided earlier.
-
Ohh! Another syntax error escaped passed me again, must slow down on my typing however you managed to correct it. Glad I could help. Dreamweaver is not the best tool in the box when it comes to PHP. I only use it for HTML/CSS I use a separate PHP IDE for my PHP coding.
-
Tried the php gtk documentation?
-
The very basic code, What does this output: $query = "SELECT * FROM sections s, products p WHERE p.pSection=s.sectionID ORDER BY sectionOrder ASC"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { echo '<pre>' . print_r($row, true) .' </pre>'; } The major step here is using an SQL join to query two tables at once.
-
You wouldn't need to submit the form to edit.php, just submit the form to it's self. Then in the edit section for the switch/case statement you'll have this: case 'edit': // check that form has been submitted: if(isset($_POST['submit']) && $_POST['submit'] == 'Apply Changes') { $id = $_POST['id']; foreach($_POST as $field => $value) { if($field != 'submit' && $field != 'id') { $fields_list[] = "`$field`='" . mysql_real_escape_string($value) . "'"; } } $sql = 'UPDATE ' . $tbl_name . ' SET ' . implode(', ', $fields_list) . ' WHERE id=' . $id; echo "<pre>$sql</pre>"; } // form has not been submitted, display form elseif(isset($_GET['id']) && is_numeric($_GET['id'])) { // get the id from the url // url example: ?action=edit&id=1 $id = $_GET['id']; // get data from table based on id $sql = 'SELECT * FROM '.$tbl_name.' WHERE id='.$id; // perform query $result = mysql_query($sql); // check that the quewry return one result if(mysql_num_rows($result) == 1) { $row = mysql_fetch_assoc($result); $form = '<form action="?action=edit" method="post">'; foreach($row as $field_name => $field_value) { $form .= '<b>' . ucwords($field_name) . '</b>: <input type="text" name="'. $field_name .'" value="' . $field_value . '" /><br />'; } $form .= '<input type="submit" name="submit" value="Apply Changes" /></form>'; echo $form; } } break;
-
It'll be better if you looked into converting your for loops and mysql_result functions calls into a nice simple while loop: while($row = mysql_fetch_assoc($sql_query_result)) { echo '<pre>' . print_r($row, true) .' </pre>'; } That will require far less coding. You should also look into using SQL Joins. Rather than calling multiple queries you'd just have one query and a single while loop, which will make for some nice clean code.
-
And you have uncommented the mysql extension in the php.ini and setup the extension_dir path correctly? Looks like php is is still having issues loading the mysql extension. Scan your computer for any files called php_mysql.dll and libmysql.dll Windows should only find one instance of php_mysql.dll which should be in your php ext folder (C:\Program files\php\ext) however for libmysql.dll only two instances should be found, one in the root of the php folder and the other in the bin folder for mysql. If Windows finds any extract files, either delete them or temporarily rename them. Also ensure you are restarting Apache when you make any changes to the php.ini and/or have removed any files called php_mysql.dll and libmysql.dll You might also want to enable a setting called display_start_errors too within the php.ini
-
Use SELECT DISTINCT or GROUP BY field_name in your query
-
empty($_POST['rating'][$key]) it is not checking the key it is checking the value of the item in the rating array. Also I modified your code a bite: makeDropListkey function function makeDropListKey($name, $list, $selected='') { // $name select name // $list array of value, label pair // $selected selected value global $x; // for the tab index global $key; // gets this value from the foreach loop while(list($value, $label) = each($list)) { if($selected == $value) { $options .= '<option value="'.$value.'" selected="selected">'.$label.'</option>'; } else { $options .= '<option value="'.$value.'">'.$label.'</option>'; } } $dropList = '<select name="'.$name.'['.$key.']" tabindex="'.$x.'">'.$options.'</select>'."\n"; return $dropList; } Form processing code: if(isset($_POST['form2_submit'])) { $label = array( "" => "Rate this video:", "1" => "1 Poor", "2" => "2", "3" => "3", "4" => "4", "5" => "5 Excellent"); // default for empty selected foreach($_SESSION['products'] as $key => $value) { if(!isset($_POST['Rating'][$key]) || !empty($_POST['Rating'][$key])) { echo '<font face="Arial" color="#FF0000" size="+1"><b>' . $name . '</b></font>'; } else { echo $name . '</td>'; } //more code goes here that does not apply (for the $value) //used makeDropListKey which adds $key to make the Rating[$key] <-- dyanamic ability to function $list = makeDropListKey('Rating', $label, $_SESSION['Rating'][$key]); echo $list; } }
-
Have a read of this tutorial on sessions.
-
[SOLVED] password protection in phpmyadmin
wildteen88 replied to dazzclub's topic in PHP Coding Help
Then use: <?php session_start(); // check that the user has logged in if(isset($_SESSION['is_logged_in']) && $_SESSION['is_logged_in'] !== true || !isset($_SESSION['is_logged_in'])) { die('You must be <a href="login.php">login</a> to view this page!'); } require_once 'includes/connection.php'; // no need to query members table, query the contacts table as you already have the username in the 'user' session $sql = "SELECT * FROM contacts WHERE username='{$_SESSION['user']['username']}'"; // perform the query $result = mysqli_query($connection, $sql); // as you are only returning 1 row from the contact tables you don't need a while loop $row = mysqli_fetch_assoc($result); // display contact data for user echo '<pre>' . print_r($row, true) . '</pre>'; ?> <html> <head> <title>Order form</title> <link rel="stylesheet" type="text/css" href="styles/style.css" /> <head> <body> <div id="container"> <div id="holder"> <div id="header">header goes here</div> <div id="main"> You are logged in! Session data: <?php echo '<pre>' . print_r($_SESSION['user'], true) . '</pre>'; ?> <a href="logout.php">Logout</a> </div> <div id="footer"></div> <div> </div> </div> </body> </html> -
An else can only be after an if statement so common sense will tell you it needs to be this: Reformated your code: $mysite_username = $_COOKIE['mysite_username']; if (!isset($_COOKIE['loggedin'])) { echo "<a href=\"register.html\">Register</a> <a href=\"login.html\">Login</a>"; } else { echo "<a href=\"logout.php\">Logout</a> <a href=\"usercp/index.html\">UserCP</a>"; } Also I highly suggest that use sessions rather than cookies, because at the moment I can easily by pass the need to log in, by simply setting the loggedin cookie. If you use sessions then it will prevent the user from tampering with the cookies.
-
correct! Also when retrieving cookies use $_COOKIE not $HTTP_COOKIE_VARS (this variable is depreciated).
-
[SOLVED] password protection in phpmyadmin
wildteen88 replied to dazzclub's topic in PHP Coding Help
The username is already in the session!, no need to perform the sql query to get the username. In login_success.php you'll see this code: Session data: <?php echo '<pre>' . print_r($_SESSION['user'], true) . '</pre>'; ?> That will produce this result, if the user logged in successfully Session data: Array ( [id] => their_id [username] => their_username [password] => their_hashed_password ) To get the username from the session use $_SESSION['user']['username'] variable. If you look in login.php we set up the session so it stores the username from database if they login successfully. -
To check the value of form field use: !empty($_POST['Rating'][$key])
-
Here is a template. Code is not complete, all you need to do is put the necessary code bits in the switch statement (add, edit and update) <?php $host = 'localhost'; $user = 'username'; $pass = 'password'; $db_name = 'database'; $tbl_name = 'database_table'; // connect to mysql and select database mysql_connect($host, $user, $pass); mysql_select_db($db_name); // get the required action if(isset($_GET['action']) && !empty($_GET['action'])) { // call code based on action switch(strtolower($_GET['action'])) { case 'edit': // code here to update data in table break; case 'delete': // code here to delete data from a table break; case 'insert': // code here to insert data into a table break; default: echo 'Invalid action permitted'; } } else { // get all data from the table $sql = 'SELECT * FROM ' . $tbl_name; $result = mysql_query($sql); // get the number of field from the table $num_fields = mysql_num_fields($result); // display results with table fieldnames and "add" link echo '<a href="?action=add">Add Record</a> <table border="1" cellspacing="2" cellpadding="5"> <tr>'."\n"; // first the field names from table for($i = 0; $i < $num_fields; $i++) { echo ' <th>' . ucwords(mysql_field_name($result, $i)) . "</th>\n"; } // append the Action column echo " <th>Action</th>\n"; echo " </tr>\n"; // now display all content to screen while($row = mysql_fetch_assoc($result)) { // use implode to create new cell for each item in the row echo " <tr>\n <td>" , implode("</td>\n <td>", $row) . "</td>\n "; // display our action links (edit and delete) echo '<td><a href="?action=edit&id='.$row['id'].'">EDIT</a> | <a href="?action=delete&id='.$row['id'].'">DELETE</a></td>'."\n </tr>\n"; } // close table echo "</table>\n"; } ?>
-
[SOLVED] password protection in phpmyadmin
wildteen88 replied to dazzclub's topic in PHP Coding Help
I provided the script so you didn't have to perform the operation manually using phpmyadmin. My script will loop through all rows in the members table applying an md5 hash to the passwords in the password field. When inserting a password into the database, just use the md5() php function, just like I did when encrypting the $_POST['password'] variable in login.php. Example code for insert new username and encrypted password into members table; // setup username and password $username '= foo'; $password = 'bar'; // md5 encrypt the password $password = md5($password); // insert new username and password into members table: $sql = "INSERT INTO members (`username`, `password`) VALUES ('$username', '$password')"; mysqli_query($connection, $sql) or die(mysql_error()); -
Is each piece of data (EL/93.00) separated by commas? If thats the case you'll first have to use explode(',',$data) to get each piece of data on its own then use a foreach loop to loop through the bits of data. Finaly within the loop use explode("/", $data) to separate the initials (EL or RLM) and 0.00 on their own. Put it all together and you end up with this: <?php $datas = 'EL/93.00,EL/93.00,RLM/0.00,RLM/0.00,RLM/0.00,RLM/0.00,RLM/0.00,RLM/0.00,RLM/0.00'; // sperarate each peice of data from comma delimited list $bits = explode(',', $datas); // loop through the bits of data foreach($bits as $data) { // separate the initials (EL or RLM) and 0.00 into $udac array $udac = explode('/', $data); echo '<p>' . $udac[0] . '<br />' . $udac[1] . '</p>'; } ?>
-
[SOLVED] password protection in phpmyadmin
wildteen88 replied to dazzclub's topic in PHP Coding Help
If you are running the script to hash the passwords in the database, you'll need to undo what you did here: -
There is not a function as such to insert a character between another set of characters However you can do it this way: $chars = 'pp'; $new_str = $chars{0}.'h'.$chars{1}; echo $new_str;
-
php5 is backwards compatible with php4. However this will only work if your php4 scripts does not rely on depreciated php settings such as register_globals. Before upgrading php4 run phpinfo() and save the outputted html code to an external .html file call this php4info.html then when you upgrade php to php5 compare php4info.html to php5 configuration when you run phpinfo() on your php5 setup.
-
Do you mean joining two strings together, if so use the concatenation operator (.) $string1 = 'Hello world!'; $string2 = ' Nice to see you!'; // concatenate the two string together echo $string1 . $string2;
-
[SOLVED] password protection in phpmyadmin
wildteen88 replied to dazzclub's topic in PHP Coding Help
I see you attached your database schema. It is because you don't store the md5 hash for the passwords in the password field for your members table. You only store it as raw text. The script is encrypting the password when the form is submitted and is comparing an unencryupted password (in the table) to an encrypted password (from the script). You'll need to change the passwords in your database to a md5 hash in order for the script to work. For a simple fix, run this script only once! <p><font color=red>RUN THIS SCRIPT ONCE! RUNNING THIS SCRIPT MORE THAN ONCE WILL RE-ENCRYPT THE MD5 HASHES!</font></p> <?php require_once 'includes/connection.php'; $sql = 'SELECT * FROM members'; $result = mysqli_query($connection, $sql) or die(mysqli_error($connection)); while($row = mysqli_fetch_assoc($result)) { $sql = "UPDATE members SET `username`='".$row['username']."', `password`='".md5($row['password'])."' WHERE id=".$row['id']; echo '<pre>' . $sql . '</pre>'; echo 'MD5 Hashed password for "' . $row['username'] . '"<br />'; mysqli_query($connection, $sql) or die(mysqli_error($connection)); } echo 'Affected rows: ' . mysqli_affected_rows($connection); ?> <p><font color=red>RUN THIS SCRIPT ONCE! RUNNING THIS SCRIPT MORE THAN ONCE WILL RE-ENCRYPT THE MD5 HASHES!</font></p> -
[SOLVED] password protection in phpmyadmin
wildteen88 replied to dazzclub's topic in PHP Coding Help
Wheres the quotes gone? The query code should be: $sql= "SELECT * FROM members WHERE username='$username' AND password='$password'"; Did you modify the code in some way? All string values need to be wrapped within quotes in a query. Otherwise MySQL will think you're referencing a column, which is why you're retrieving the error. -
Edit Ignore that didn't read your post properly. I used regex instead of a simple explode: $lines = file('welcome.txt'); foreach($lines as $line) { if(preg_match("|^1([a-z0-9]+)\s+([a-z]+)\s+([0-9\.]+)|i", $line, $matches)) { list(,$word1, $word2, $word3) = $matches; echo '<p>Word1: ' . $word1 . '<br />'; echo 'Word2: ' . $word2 . '<br />'; echo 'Word3: ' . $word3 . '</p>'; } }