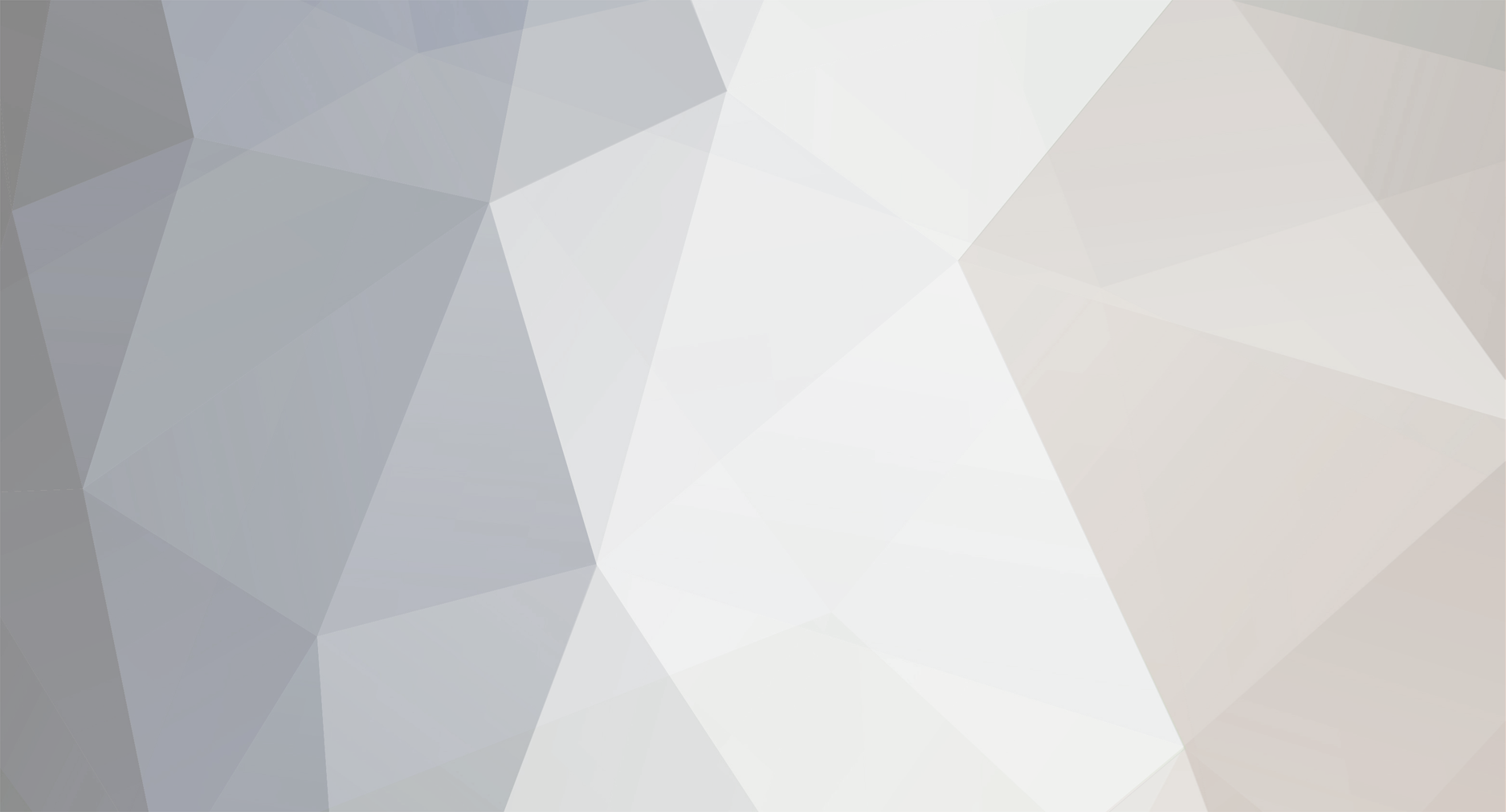
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
[SOLVED] password protection in phpmyadmin
wildteen88 replied to dazzclub's topic in PHP Coding Help
Modified your code abit: <?php require_once 'includes/connection.php'; // check that the form is submitted if(isset($_POST['submit'])) { // validate username if(isset($_POST['username']) && !empty($_POST['username'])) { // use the built in mysql real escape string function to protect agains SQL Injection $username = mysqli_real_escape_string($connection, $_POST['username']); } else { // username does not validate, define an error $errors[] = 'You have forgotton to include your username.'; } // we apply the same for the password field. if(isset($_POST['password']) && !empty($_POST['password'])) { $username = mysqli_real_escape_string($connection, $_POST['password']); } else { $errors[] = 'Password not provided'; } // chekc that no errors have been set, if so display them if(isset($errors) && is_array($errors)) { echo 'Errors: <ul><li>' . implode('</li><li>', $errors) . '</li></ul>'; } // no errors are set so we'll continue else { // run query $sql = "SELECT * FROM members WHERE username='$username' and password='$password'"; $result = mysqli_query($connection, $sql); // check that the query return only ONE result if(mysqli_num_rows($result) == 1) { $_SESSION['is_logged_in'] = true; // get result set from the query and assign it to the 'user' session. $row = mysqli_fetch_assoc($result); $_SESSION['user'] = $row; // redirect to the login_success.php header('Location: login_success.php'); exit; } // query failed, display error echo "Wrong Username or Password"; } } // for was not submitted, display error else { echo 'Please use the login form for logging in'; } ?> <?php session_start(); // check that the user has logged in if(isset($_SESSION['is_logged_in']) && $_SESSION['is_logged_in'] !== true || !isset($_SESSION['is_logged_in'])) { die('You must be logged in to view this page!'); } ?> <html> <head> <title>Order form</title> <link rel="stylesheet" type="text/css" href="styles/style.css" /> <head> <body> <div id="container"> <div id="holder"> <div id="header">header goes here</div> <div id="main"> You are logged in! Session data: <?php echo '<pre>' . print_r($_SESSION['user'], true) . '</pre>'; ?> </div> <div id="footer"></div> <div> </div> </div> </body> </html> I have modified it so all user details get saved to a session variable called 'user'. This will save you from have to run an sql query to retrieve the user details from the database for every page. -
Users will not be able to override your variables within your scripts provided register_globals is disabled (which it should be by default).
-
That should work, aslong the .noBoarder selector is set after the h3 selector, eg: h3 { /* css for <h3></h3> tags } h3.noBorder { border-style: none; } if it still doesnt work then re-set the boarder: border: 0px solid #FFF;
-
I re-edited my post. read above
-
verticle-align does not work the same with divs as it does with tables. If you only want the images to be vertically aligned then apply vertical-align: middle; to the .filmstrip class
-
Is the cache folder inside your public_html folder? If it isn't then it wont work. You are only allowed access to files within the public_html folder
-
You must have some kinda bug in your code which causes this. Try setting error_reporting to E_ALL and enabling display_errors within the php.ini Your session settings are fine, they should not need to be modified.
-
The reason you're getting that error is because something else is already running on port 3306. This port is the default port mysql uses for listening for incoming tcp/ip requests. It appears the original mysql process must be still running. How come you appear to think mysql is currupt? Are you using MySQL database with a PHP script and the PHP script no longer works?
-
You could just use the modulus (%) operator instead: $i = 0; while($i < 10) { $color = ($i%2) ? 'red' : 'green'; echo '<span style="color:'.$color.'">Line #' . ($i+1) . '</span><br />'; $i++; }
-
It is better to use an array then separate variables. I'm asumming you're using my code So if someone entered "one two three" into the text box (Minus the quotes). Then to get the first word you'd use $textarea_text_array[0], to get the second you'd use $textarea_text_array[1] and finally $textarea_text_array[2] to get the last. Arrays start at zeros. I know when using arrays for the first time it seems confusing however arrays can very helpful when used in the correct way. My code is just an example, you can change the variable $textarea_text_array to shorter one instead, by simple changing, this line: $textarea_text_array = explode("\n", $textarea_txt); to: $words = explode("\n", $textarea_txt); Then use $words[0], $words[1], $words[2] to retrieve the words. To understand arrays further I encourage you to have a read of the manual on arrays.
-
What's the advantage of a do / while loop as opposed to just a regular while loop? PHP will always execute the code in the do clause first regardless of the argument in the while statement. eg: $i = false; do { echo 'While loop ran'; } while($i == true);
-
You can do this with javascript: setTimeout("submitForm()", 60000); function submitForm() { document.form_name.submit(); } change form_name to the name of your form.
-
Use a JOIN: SELECT * FROM members m, readings r WHERE m.CNumber='$CNumber' AND r.CNumber='$CNumber' AND r.RType='$RType'"
-
Getting information from the site address
wildteen88 replied to netpsycho's topic in PHP Coding Help
The only difference is index.php has to be present in the url, it is still as effective as mod_rewrite. -
Getting information from the site address
wildteen88 replied to netpsycho's topic in PHP Coding Help
One method is to use mod_rewrite which will allow you to create clearn urls like http://www.giantbomb.com/category/arcade/ or you can go the PHP route, example: <?php function get_path() { $path_bits = false; if(isset($_SERVER['PATH_INFO']) && $_SERVER['PATH_INFO'] != '/') { // get the path info // path format: index.php/module/page/params1/params2/ etc $path = $_SERVER['PATH_INFO']; // check that the last character in the path is not a backslash // if it is we'll remove it if(substr($path, strlen($path)-1, 1) == '/') { $path = substr($path, 0, strlen($path)-1); } // explode the bits from the path, eg module, page and parameters $path_bits = explode('/', $path); // drop index.php array_shift($path_bits); } return $path_bits; } $path_bits = get_path(); echo '<pre>' . print_r($path_bits, true) . '</pre>'; ?> -
mod re-write - nearly there, but a bit oif help please ;-)
wildteen88 replied to Mr Chris's topic in Apache HTTP Server
as $_GET['story_id'] holds your headline man-walks-in-park you'll need to use the headline field rather than the story_id field in your query eg: $headline = mysql_real_escape_string(str_replace('-', ' ', $_GET['story_id'])); $result = mysql_query("SELECT * FROM cms_stories WHERE headline='$headline'"); -
You might want to apply a rewrite condition: RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteRule ^(.+)/(.+)/(.+)\.png /image.php?type=$1&style=$2&tag=$3 Also it may because you're using (.+) which I believe is greedy. What you should do is use character ranges: Full code RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteRule ^([a-z]+)/([a-z]+)/([a-z]+)\.png$ /image.php?type=$1&style=$2&tag=$3 [NC]
-
mod re-write - nearly there, but a bit oif help please ;-)
wildteen88 replied to Mr Chris's topic in Apache HTTP Server
My rewrite rule is working fine for me. If I create a file called section.php and place it in my root and add the following code: <?php echo '<pre>' . print_r($_GET, true) . '</pre>'; ?> and go to mysite.com/article/2/man-walks-in-park/ then I get this output: Array ( [s] => 2 [story_id] => man_walks_in_part ) -
All it'll take is one line of javascript, something like: <textarea onkeyup="document.textarea2.value=this.value"></textarea> Create another textarea and give it an id of textarea2, eg: <textarea id="textarea2"></textarea> It may not work, it is only for an example code. However do test it.
-
[SOLVED] Offsetting server time ahead to local time using date();
wildteen88 replied to Dale_G's topic in PHP Coding Help
Use the set_default_time_zone function or use date('h:i:s A', strtotime('+3 hours')); -
Yes it is possible.
-
<?php if(isset($_POST['submit'])) { echo '<pre>' . print_r($_POST, true) . '</pre>'; } ?> <form method="post"> <table border="0" cellspacing="2" cellpadding="4"> <tr> <th>Item</th> <th>Qty</th> <th>Unit Price</th> <th>Vat Rate</th> </tr> <?php for ($i=1;$i<=10;$i++): ?> <tr> <td><input type="text" name="item_code[]" size="12" maxlength="12"></td> <td><input type="text" name="item_quantity[]" size="11" maxlength="11"></td> <td><input type="text" name="item_price[]" size="11" maxlength="11"></td> <td><input type="text" name="item_rate[]" size="8" maxlength="8"></td> </tr> <?php endfor; ?> </table> <input type="submit" name="submit" value="submit" /> </form>
-
mod re-write - nearly there, but a bit oif help please ;-)
wildteen88 replied to Mr Chris's topic in Apache HTTP Server
Try: RewriteRule ^article/([0-9]+)/([a-z0-9\-]+)/$ section.php?s=$1&story_id=$2 [QSA,NC,L] -
If you are getting that error, then that suggests A) You have setup a password for the root account but you have forgotten it B) MySQL is refusing connections from localhost. To see if a password is set for the root user open a MySQL terminal by going to Start > Run and typing in cmd into the run box. Click ok to open the command line Now type the following command: mysql -u root and press enter. If no password is set for the root user you should be shown the MySQL Monitor. If there is a password set you'll receive the Access denied error message. If you have forgotten the password for the root user then you'll need to reset it.