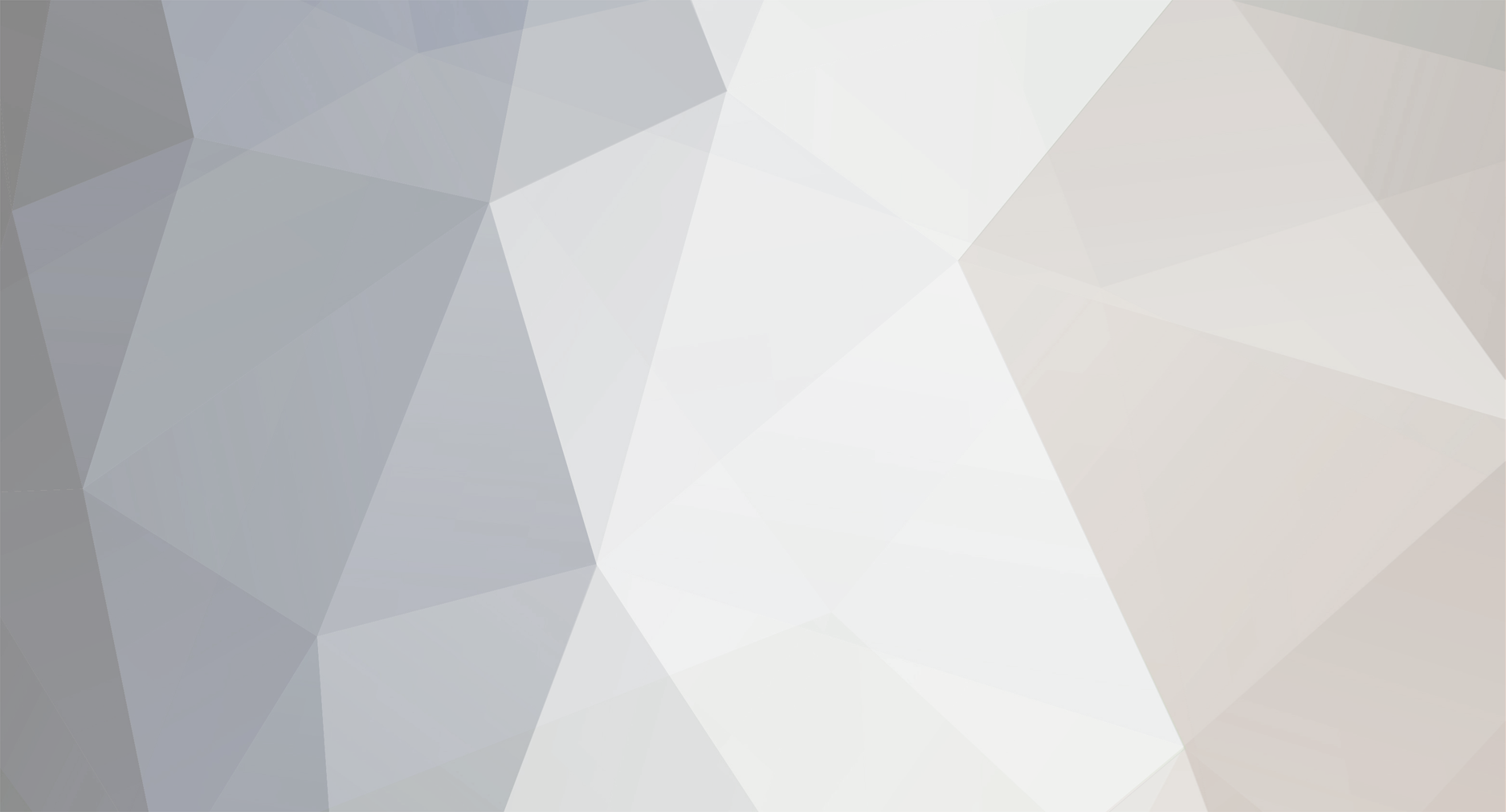
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
If you querys are failing use mysql_error() to retrieve the error from mysql: $resultat = mysql_query("insert into cc_client (NoClient, NoClientA, Nom, Adresse, Ville, CodePostal, Telephone) VALUES ($noenreg, '$enrer[0]', '$nom', '$adresse', '$ville', '$codepostal', '$notel')",$mydb) or die('Query error: ' . mysql_error());
-
When you use sessions, make sure you call the session_start() function on every page that uses sessions, eg: <?php session_start(); // your code ?> Also to check if a session variable exists, use isset: if(isset($_SESSION['FirstName'])) { echo '$_SESSION[\'FirstName\'] exists'; } else { echo '$_SESSION[\'FirstName\'] does not exist'; } session_is_registered is also depreciated I believe.
-
Well as the browser requests for that file, then that is the same as typing yoursite.com/folder/style.css within the address bar. There is no simple solution for protecting the css file. The css file and html file all get downloaded to the browsers cache and is parsed to produce what the user displays sees.
-
If you are including stylesheets into your php script then theres no point in protecting the folder that holds the stylesheet as the user will be able view the css when they go to View > Source To stop people from going a folder and viewing the folder index just add a blank index.html file into the folder, The server will serve index.html file by default. Or turn Indexes of for that folder within the .htaccess file: Options -Indexes The latter may not be possible on some host.
-
You might want to remove the AVG() function. SELECT * FROM site, rating WHERE site.id = rating.sid ORDER BY rating.rating DESC
-
Maximumn size of a url depends on your webbrowser, a simple google search yeilded this. As for max $_POST size this is down to PHPs configuration run phpinfo and search for post_max_size, mines is set to 8MB which I think is the default value. If you are going to send lots of data over the url, then use sessions this will help to increase security as any data your passing from page to page is hidden.
-
What does the $_SESSION['url'] variable hold? What is the result you are expecting?
-
you cannot use a table name within the ORDER BY clause it has to be a fieldname. So to order by a field from the results table you'd results.field_name
-
Umm, you're getting free help from a public community, which members donate their free time to help. Also in order to get you a faster reply would help if you posted your current query and posting your question within the correct board. PHP help is for PHP. Yours is related to MySQL (I presume) so I'll move this tread.
-
Before using any variables which come from the user ($_POST, $_GET or $_COOKIE) then you should always check that they exist first before using then and apply some validation/varification, eg: if(isset($_POST['Catname']) && !empty($_POST['Catname'])) { $Catname = $_POST['Catname']; // place your code here. }
-
A few notes on the Configuration File (php.ini) Path and Loaded Configuration File lines when running phpinfo(). If either of the those two lines do not end in php.ini then PHP is not reading the php.ini from the displayed location. This is where many people get very confused with where to place the php.ini If php cant find the php.ini then above lines will look like the following: Configuration File (php.ini) Path C:/WINDOWS Loaded Configuration File (none) The reason why the Configuration File (php.ini) Path line reads C:/WINDOWS is because that is usually the default location PHP tries to look for the php.ini If PHP is installed in C:\php and you have added C:\php to the System PATH Environment Variable (and not the User Environment Variable which is set in the same location) your php.ini should be in C:\php. In order for the new Environment Variables to work you must restart your computer. Also note that there is no need to move any files outside of the php folder either. If PHP is still not reading the php.ini then ensure the php.ini is the correct file type. As Windows hides the file extension from known file types it can obscure you from the real file extension of files. You maybe seeing php.ini as the file name from Windows Explorer but it may be named as php.ini.txt but Windows only shows the file as php.ini. You can tell this by looking at the file icon for the php.ini. It should have an image of a notepad which has an orange cog wheel on the right hand side of it and the words Configuration Settings underneath the filename in light grey (when viewed in Titled mode - Views > Tiles from Windows Explorer). If none of the above is true then there's your problem.
-
Sorry for the confusion, yes any instance of $REQUEST_METHOD you should change to $_SERVER['REQUEST_METHOD']; As for "$Catname should be $_POST['Catname'];" I forgot to delete that part. Instead do the following:
-
What editor are you using to create your PHP scripts? Most PHP editors have a setting for showing a "Right margin" which will display a grey line up to x amount of characters so when you get up to right margin you can decide whether to carry on or go to the next line. If you are outputting very large strings then use the concatenation to split the string into multiple lines, eg: $str = 'Some very long string here. '. // split long string into multiple lines 'carry on very long string. '. 'and so on'; The above comes in handy with very long MySQL query's. Query's do not need to be defined in one continuous line. This will help to eliminate scrolling. Another example :- If you are defining an array which has a lot of items, I prefer to define each array item on a separate line, eg: $arr = array( 'item1', 'item2', 'item3', 'item4' => array( 'item4_a', 'item4_b', 'etc' ) ); You do not always have to code very long lines. Try to split them up in some way.
-
That script appears to rely on register_globals. Register_globals has been depreciated and is turned off by default, this is why your script is not working. You should attempt to update your script so it doesn't rely on register_globals. $REQUEST_METHOD should be $_SERVER['REQUEST_METHOD']; $Catname should be $_POST['Catname']; Also the following is not secure enough: Catname = str_replace("'","''",$Catname); I'd change it to: Catname = mysql_real_escape_string($_POST['Catname']); $_SERVER and $_POST are predefined variables called superglobals.
-
Yes look into [http://www.php.net/gd]GD[/url] or search the forums. There have been many questions about resizing images with GD. here is the code to get the image name. md5($new_filename)."-".$user.".".getExt($bfilename); as you can see, before the extension is their username, so is there a function that can take last part of the filename before the extension, and tell if it is the user that is trying to upload a new image? To retrieve the username from the filename of a file use: $image = '5c435de5d9db471ca31b5b028eaf2acd-lamez.png'; list($image_name_hash, $username) = explode('-', pathinfo($image, PATHINFO_FILENAME)); echo 'Hash: ' . $image_name_hash . '<br />'; echo 'Username: ' . $username;
-
can someone help pls i cant get sessions to work,
wildteen88 replied to sinista's topic in PHP Installation and Configuration
Try deleting the PHPSESSID cookie and try your script again. Sessions should work fine with the default PHP sessions settinga within the php.ini. I don't know what else to suggest. -
can someone help pls i cant get sessions to work,
wildteen88 replied to sinista's topic in PHP Installation and Configuration
To check whether the PHPSESSID cookie is set is by looking through your browsers cookie manger/internet temporary files Firefox: Tools > Options > Privacy > View Cookies > Type PHPSESSID into Search box. Firefox will show all cookies with the name PHPSESSID scroll through the list of cookies until you come to your sites domain name (eg yourdomain.com) within the Sites column. -
What do you define as closest to 100? You can just do a simple range comparison: // if $total greater than 80, wrap $total in bold tags if($total > 80) { echo '<b>' . $total . '</b>'; } // if $total greater than 50 but less than 80, wrap $total in itialic tags elseif($total > 50 && $total < 80) { echo '<i>' . $total . '</i>'; } // $total is than 50, wrap $total in underline tags elseif($total < 50) { echo '<u>' . $total . '</u>'; }
-
The best way of finding the correct book which will interest you is to go down to your nearest library/book store and have a look. This is what I used to do when I was starting out in PHP and wanting to find books which taught PHP at more intermediate level rather than a beginner level. You should look at the books with titles like Professional PHP5 these types of books will teach the more advanced sides of PHP such as OO concepts.
-
Did you code this yourself? or did you download this script from somewhere or someone else coded it for you? If its any of the latter then I suggest you to either find an updated script or get in contact with the author of the script. We cant really correct the script to work with register globals being off. This is one of the many pains of scripts which rely upon register_globals being off.
-
I am trying it add an alt text to the following image code
wildteen88 replied to blagger's topic in PHP Coding Help
You cannot add a title to background images, which deviltronics_top.gif is. I guess the tep_draw_separator function produces the relevant <img /> HTML code for displaying the pixel_trans.gif image. If you want to add a title to that image then you'll going to need to modify that function. Without seeing any code for that function I can't really suggest any thing. -
can someone help pls i cant get sessions to work,
wildteen88 replied to sinista's topic in PHP Installation and Configuration
Make sure a cookie called PHPSESSID is being set. This cookie should hold a valid 32 character sessions id string (the same as the temporary sess_ file). Eg if you get a a session file created called sess_ra8oanb3qr86t44t9dcg2fhbi5 then the PHPSESSID cookie should be set to ra8oanb3qr86t44t9dcg2fhbi5. This will also mean your browser must have cookie enabled too. Also uncomment this line: ; session.use_only_cookies = 1 by removing the semi-colon at the start of the line. Save your php.ini and restart Apache. -
I am trying it add an alt text to the following image code
wildteen88 replied to blagger's topic in PHP Coding Help
Where is the image you want to add a title to? What have you tried so far? -
Automatically route local sites to correct place
wildteen88 replied to redbullmarky's topic in Apache HTTP Server
I have a read of Dynamically configured mass virtual hosting from the manual. Seems promising. Not sure about the hosts file though, I dont think .localhost will work though. -
If you are distributing your script for other people to install on their websites then you don't need to provide the AMP stack, as it already be setup by the webhost. All you need to do is provide the php scripts.