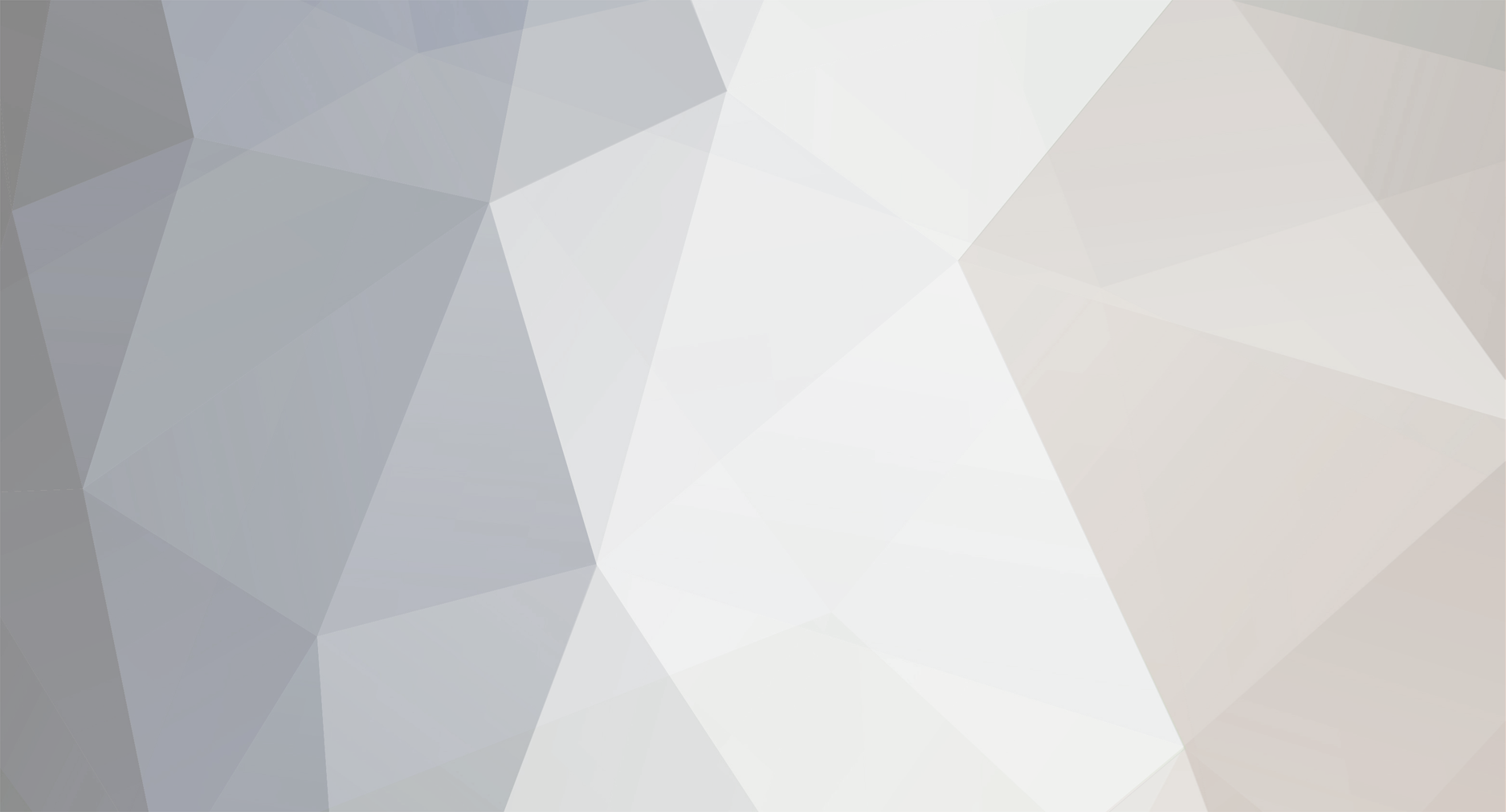
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
[SOLVED] interesting... create your own tag?
wildteen88 replied to optikalefx's topic in PHP Coding Help
My code should be working fine. I even tested it before I posted it and it worked no probs. Do you have access to your php.ini? If you do turn a setting called display_errors on and make sure error_reporting is set to E_ALL. Save the php.ini and then restart your server (Apache, IIS etc). re run your script. if you get any errors post them here in full. I have now added in a status messages. if the script is failing a specifc place you'll now know where it is failing: <?php echo 'Connecting to MySQL...<br />'; $con = mysql_connect('localhost', 'root'); mysql_select_db('test', $con); echo 'Connection established....<br /><br />'; $getImageLog = null; // this function gets called which returns the appripitate image function getImage($img) { global $getImageLog; $getImageLog .= 'Defining $query'; $query = "SELECT pic FROM pics WHERE num ='$img'"; $getImageLog .= ' - (' . $query . ') - Running query...<br />'; $result = mysql_query($query); $getImageLog .= 'Query has ran with no errors<br />Getting the image<br />'; // check there was one result from the query if(mysql_num_rows($result) == 1) { // get the result $row = mysql_fetch_assoc($result); $getImageLog .= 'Image recieved (' . $row['pic'] . ' for img#' . $img . ')<br /><br />'; // return the html for the html return '<img src="http://www.4tenproductions.com/ir/uploadedfiles2/' . $row['pic'] . '">'; } else { $getImageLog .= 'img#' . $img . ' not found<br /><br />'; // image not found in database return '[img#' . $img . ' Not found]'; } } echo 'Defining $txt variable...<br />'; // dummy text $txt = 'Hello world! <include picture# 1> Welcome to my site! <include picture# 6>'; echo '$txt variable defined. Now to parse $txt...<br />'; // use regex to replace <include picture# 123> with the appropiate image // this will call the getImage function. $new_txt = preg_replace('/<include picture# ([0-9]+)>/ise', "getImage($1)", $txt); echo 'getImage() log:<div style="padding:20px;">' . $getImageLog . '</div><br />Result....<br /><br />'; echo htmlentities($txt) . '<hr />' . $new_txt; ?> -
For getting started with using MySQL with the command line and using PHP with MySQL have a read through the free chapters of this book. Note, the first chapter is for setting up PHP and MySQL. As you already have PHP setup jump to the fourth page and read on from the Post-Installation Setup Tasks heading. Also that tutorial is written for Mac and Unix set ups so ignore the bits about unix and mac. That tutorial goes on for four chapters. Summary of Chapters: Chaptet 1: Setting up AMP (Only read from page four for that chapter as discussed above) Chapter 2: MySQL syntax basics via command line - setting up database, creating tables, inserting, deleting, selecting data etc. Chapter 3: Getting started with PHP, learning the basics, such as variables, functions, arrays, loops etc. Chapter 4: Making the MySQL and PHP application (Jokes database webapp)
-
can host more than one website in www root folder?
wildteen88 replied to cluce's topic in Apache HTTP Server
To run multiple websites of the same box you'll want to set virtual hosts. Have read of the manual for setting up virtual hosts. -
Use: $i = 10; $var_10 = 'hello'; echo ${'var_' . $i}; EDIT Beaten to it by tippy, But anyways if you want to use variables value as part of a variables name you'll have to use variable variables, which what the above is
-
A very basic regex pattern: $dob = '02/01/1964'; echo '<b>DOB: </b>' . $dob . '<br />'; if(preg_match('|[0-9]{2}/[0-9]{2}/[0-9]{4}|', $dob)) { echo 'Date of birth is in the correct format'; } else { echo 'Date of birth is not in the correct format'; }
-
[SOLVED] interesting... create your own tag?
wildteen88 replied to optikalefx's topic in PHP Coding Help
I had a slight typo. Try this: <?php $con = mysql_connect("xxxx", "xxxx", "xxxx"); mysql_select_db("update1", $con); // this function gets called which returns the appripitate image function getImage($img) { $query = "SELECT pic FROM pics WHERE num ='$img'"; $result = mysql_query($query); // check there was one result from the query if(mysql_num_rows($result) == 1) { // get the result $row = mysql_fetch_assoc($result); // return the html for the html return '<img src="http://www.4tenproductions.com/ir/uploadedfiles2/' . $row['pic'] . '">'; } else { // image not found in database return '[img#' . $img . ' Not found]'; } } // dummy text $txt = 'Hello world! <include picture# 1> Welcome to my site! <include picture# 6>'; // use regex to replace <include picture# 123> with the appropiate image // this will call the getImage function. $new_txt = preg_replace('/<include picture# ([0-9]+)>/ise', "getImage($1)", $txt); echo $txt . '<hr />' . $new_txt; ?> -
displaying data from mysql on a php page (page.php?ID=)
wildteen88 replied to jdrasq's topic in MySQL Help
You use $_GET to get url parameters from the url, eg mysite.com?page.php?id=1234 to get the id parameter from the url you'd use $_GET['id']. You'd then use $_GET['id'] within your query: // check if id is set and that it only consists of numbers. // You should always validate data before using it if(isset($_GET['id']) && is_numeric($_GET['id'])) { $id = $_GET['id']; $sql = "SELECT * from pages_table WHERE page_id='$id'"; $result = mysql_query($sql); // rest of code here for displaying the page data from the database. } -
OK PHP is not reading your php.ini. Theres the problem. I'm guessing your have your php.ini in C:/php - this is ok however PHP wont be able to read the php.ini in C:/php as it only checks a couple of places for the php.ini which is C:/Windows (or any folder within C:/Windows) the Windows PATH variable or the registry. What I recommend you to do is to add php to the Windows PATH. You can do this by reading this PHP FAQ. Once you have added PHP to the path, PHP should now be able to read your php.ini.
-
[SOLVED] interesting... create your own tag?
wildteen88 replied to optikalefx's topic in PHP Coding Help
What you'll want to do is create a function call getImages which parses the text in $myText to translate <include picture# 15> into the appropriate image. <?php $con = mysql_connect("xxxx", "xxxx", "xxxx"); mysql_select_db("update1", $con); // this function gets called which returns the appripitate image function getImage($img) { $query = "SELECT pic FROM pics WHERE num ='$img'"; $result = mysql_query($query); // check there was one result from the query if(mysql_num_rows($result) == 1) { // get the result $row = mysql_fetch_assoc($result); // return the html for the html return '<img src="http://www.4tenproductions.com/ir/uploadedfiles2/' . $row['pic'] . '">'; } else { // image not found in database return '[img#' . $img' Not found]'; } } // dummy text $txt = 'Hello world! <include picture# 1> Welcome to my site! <include picture# 6>'; // use regex to replace <include picture# 123> with the appropiate image // this will call the getImage function. $new_txt = preg_replace('/<include picture# ([0-9]+)>/ise', "getImage($1)", $txt); echo $txt . '<hr />' . $new_txt; ?> You're best of running this code when you get the page data out of the database. -
You could try addding the following at the top of your script: ini_set('max_execution_time', '300');
-
Have you setup the extension_dir directive within the php.ini. the extension_dir directive must point to PHP's extension folder (ext/), eg: extension_dir = "C:/php/ext" Change C:/php to the path in which you have installed PHP to. Save the php.ini and restart Apache. You must restart Apache when ever you change Apaches or PHP's configuration. If you're still getting the undefined function error then make sure PHP is using the php.ini your are editing by running phpinfo within a script. Check that the Loaded Configuration File (or the Configuration File (php.ini) Path) line is set to the full path in which your php.ini is located. Eg if your php.ini is in C:\php then either line should be set to C:\php\php.ini (don't worry if one line shows C:\WINDOWS as the path. Only one or the other needs to be set to php's php.ini path).
-
how to handle 2 mysql database connections with php
wildteen88 replied to bruckerrlb's topic in PHP Coding Help
bruckerrlbPlease read your other post -
[SOLVED] handling multiple sql statements
wildteen88 replied to bruckerrlb's topic in PHP Coding Help
When you connect to multiple databases you must pass the link identifier (this is returned from mysql_connect) as the second parameter for the mysql_query function when you want to run a query for one of the databases. Try the following: <?php // Always add these lines at the top of script ini_set ('display_errors', 1); error_reporting (E_ALL & ~E_NOTICE); require_once 'auth.php'; // Local Database connection $local_db = mysql_connect('localhost', 'root') or die('localhost connecion failed:<br />' . mysql_error($local_db)); mysql_select_db('users', $local_db) or die('localhost database selection failed:<br />' . mysql_error($local_db)); // Remote Database connection $remote_db = mysql_connect('192.168.1.2', 'fakeusernamehere', 'fakepasswordhere') or die('remote connecion failed:<br />' . mysql_error($remote_db)); mysql_select_db('eisw', $remote_db) or die('remote database selection failed:<br />' . mysql_error($remote_db)); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1" /> <title>Seguimiento de llamadas</title> <link href="style.css" rel="stylesheet" type="text/css" /> </head> <body> <div id="Layer2"></div> <div class="main"> <div class="header" style="text-align: center"> <h1>Cabecera</h1> </div> <div class="toolbar"> <span class="style2">Está Usten En: <a href="principle_menu_user.php" class="style2">Menú Principle</a></span> - <span class="style3">Seguimiento de llamadas</span> </div> <div class="userchooser" style="text-align: center"> <form action="<?php echo $_SERVER['PHP_SELF']?>" method="post"> <table> <tr> <td width="340" height="37">Seleccione el producto:</td> <td width="123"> <select> <?php //if this causes errors, move the variable getting the session id somewhere else $memberid = $_SESSION['SESS_MEMBER_ID']; $query = "SELECT * FROM products WHERE clientid = '$memberid'"; $r = mysql_query($query, $local_db) or die ("Could not perform query:<br />\n$query<br />\n" . mysql_error($local_db)); while ($row = mysql_fetch_array($r)) { print ' <option id="productname">' . $row['productname'] . "</option>\n"; } ?> </select> </td> </tr> <tr> <td height="45">Llamadas realizadas los dÃas:</td> <td><input type="text" id="startdate" name="startdate" /></td> <td width="108"><input type="text" id="enddate" name="enddate" /></td> </tr> <tr> <td height="41">Durante las horas:</td> <td><input type="text" id="starthour" name="starthour" /></td> <td><input type="text" id="endhour" name="endhour" /></td> <td><input type="submit" value="buscar" name="submit" /></td> </tr> </table> </form> </div> <?php // check that form has been submitted // When the form is submitted the followng will be run. if(isset($_POST['submit'])) { ?> <div class="datasectionuser"> <table width="769" height="24"> <tr bgcolor="#bbe0e3"> <td width="141">Nº teléfono cliente</td> <td width="185">Nº teléfono en centralita</td> <td width="184">Producto asociado</td> <td width="34">fecha</td> <td width="80">hora</td> <td width="117">minutos</td> </tr> <?php // declare my variables from the post $startdate = $_POST['startdate']; $enddate = $_POST['enddate']; $starthour = $_POST['starthour']; $endhour = $_POST['endhour']; $productname = $_POST['productname']; //this is from the select option where the user can select his/her option // query a specific table in my database on my server, basically I need to translate product name to product id $query = "SELECT * FROM products WHERE productname = '$productname'"; // bring back the query into a variable $r = mysql_query($query, $local_db); // Get the product id list($productid) = mysql_fetch_array($r); //query the remote database for the specific user $query = "SELECT * FROM ei10800010001200708 WHERE NumeroExterno = '$productid' AND " . "FechaDesde > '$startdate' AND FechaHasta < '$enddate' AND HoraDesde > '$starthour' AND HoraFecha < '$endhour'"; mysql_query($query, $remote_db) or die ("Could not perform query:<br />\n$query<br />\n" . mysql_error($remote_db)); while ($row = mysql_fetch_array($r)) { ?> <tr> <td width="141" align="center"><?php echo $row['NumeroExterno']; ?></td> <td width="141" align="center"><?php echo $row['NumeroExtension']; ?></td> <td align="center"><?php echo $row['productname']; ?></td> <td align="center"><?php echo $row1['FechaDesde']; ?></td> <td align="center"><?php echo $row1['HoraDesde']; ?></td> <td align="center"><?php echo $row1['Duracion']; ?></td> </tr> <?php } ?> </table> <!--I will Figure this export thing out if this query works--> <a href="excel.php" class="createnew">Exportar a Excel</a> </div> <?php } // close if ?> </div> </body> </html> <?php // close connections to database mysql_close($connect1); mysql_close($connect2); ?> I have tidied up your code a bit so its more easer to read. -
Make sure your firewall is not blocking incoming connections on port 80.
-
You need call the news() function in shows.php. PHP wont run that function until you tell it to. PHP wont call functions automatically. <?php include 'includes/config.php'; // make sure do isset if(!isset($_GET['do'])) { die('No action found'); } // make sure id is set and that is of a numrical value if(!isset($_GET['id']) || !is_numerical($_GET['id'])) { die('id not found'); } // call the function switch($_GET['do']) { case 'company': case 'news': case 'sponsor': case 'event': // get the id $id = $_GET['id']; // call the function based _GET['do'] variables value $_GET['do']($id); break; } // function company { // company actions // } function news ($id) { $query_news_rs = "SELECT * FROM news WHERE id=$id"; $news_rs = mysql_query($query_news_rs, $db) or die(mysql_error()); $row_news_rs = mysql_fetch_assoc($news_rs); // *** Get News Details *** $id = $row_news_rs['id']; $channel_id = $row_news_rs['channel_id']; $news_title = $row_news_rs['news_title']; $news_url = $row_news_rs['news_url']; $news_pic = $row_news_rs['news_pic']; $news_date = $row_news_rs['news_date']; $news_content = $row_news_rs['news_content']; $news_display = $row_news_rs['news_display']; // HEREDOC SYNTAX (http://php.net/heredoc) echo <<<EOF <TABLE WIDTH="100%" id="News Details"> <TR> <TD> $id<BR/> $channel_id<BR/> $news_title<BR/> $news_url<BR/> $news_pic<BR/> $news_date<BR/> $news_content<BR/> $news_display<BR/> </TD> </TR> </TABLE> EOF; // DONOT MOVE OR PLACE ANYTHING ON THE LINE ABOVE // *** Free Database *** mysql_free_result($news_rs); } // function sponsor { // sponsor actions // } // function event { // event actions // } ?>
-
This: } </body> </html>' should be: </body> </html>'; }
-
I know this is solved, but Just to let you know in order to get your last setup to work you'd do this: echo '<p>' . ${'slog' . $n} . '</p>'; The technique is called variable variables.
-
If its in the Configure Command line then there is no chance of it being enabled. In order to enable it your host will need to re-compile PHP.
-
The script does nothing of what you say it'll do. What it'll do is define two variables called txt and number. txt holds a string value of "hello world" and number holds a integer value of 16. It'll do nothing else In order for PHP to display the value of the variables you'll use echo: <?php $txt = "Hello World!"; $number = 16; echo '$txt is: ' . $txt . '<br />'; echo '$number is: ' . $number; ?> RESULT $txt is: Hello World $number is: 16
-
mod_rewrite works on the server end and not the client. So you wont have any problems with browser incompatibility issues. I would of thought most hosts would allow for mod_rewrite. You can always check with your host. Also mod_rewrite is an Apache module so it'll only work on Apache servers You can always setup a setting for your app so the user can enable or disable clean urls. SO you're better of coding for both sides.
-
If its disabled then there will be no way to enable this function. Most shared hosts does not allow custom php.ini settings for each site.
-
Looks like you have setup a password for the root user during the installation process for MySQL. You'll have to provide the password you setup for the root user in order for phpMyAdmin to connect to mysql. You define the password for the root user through this line in the config.inc.php: $cfg['Servers'][$i]['password'] = 'password here';
-
If you are on shared hosting then I doubt your host will allow you access to the httpd.conf. What you could do is use .htaccess. .htaccess files allows to change certain aspects of Apache configuration through this file. However not all host allow you to use .htaccess. How come you have to set up a server environment for password and username for your database?
-
The root of phpmyadmin folder, eg if you installed phpmyadmin in C:/phpmyadmin then you place the config.inc.php in C:/phpmyadmin
-
Could you post your code here. Also have you saved your code in a .php file? HTML does not change PHP's behaviour. The PHP code is parsed way before the HTML gets to the browser.