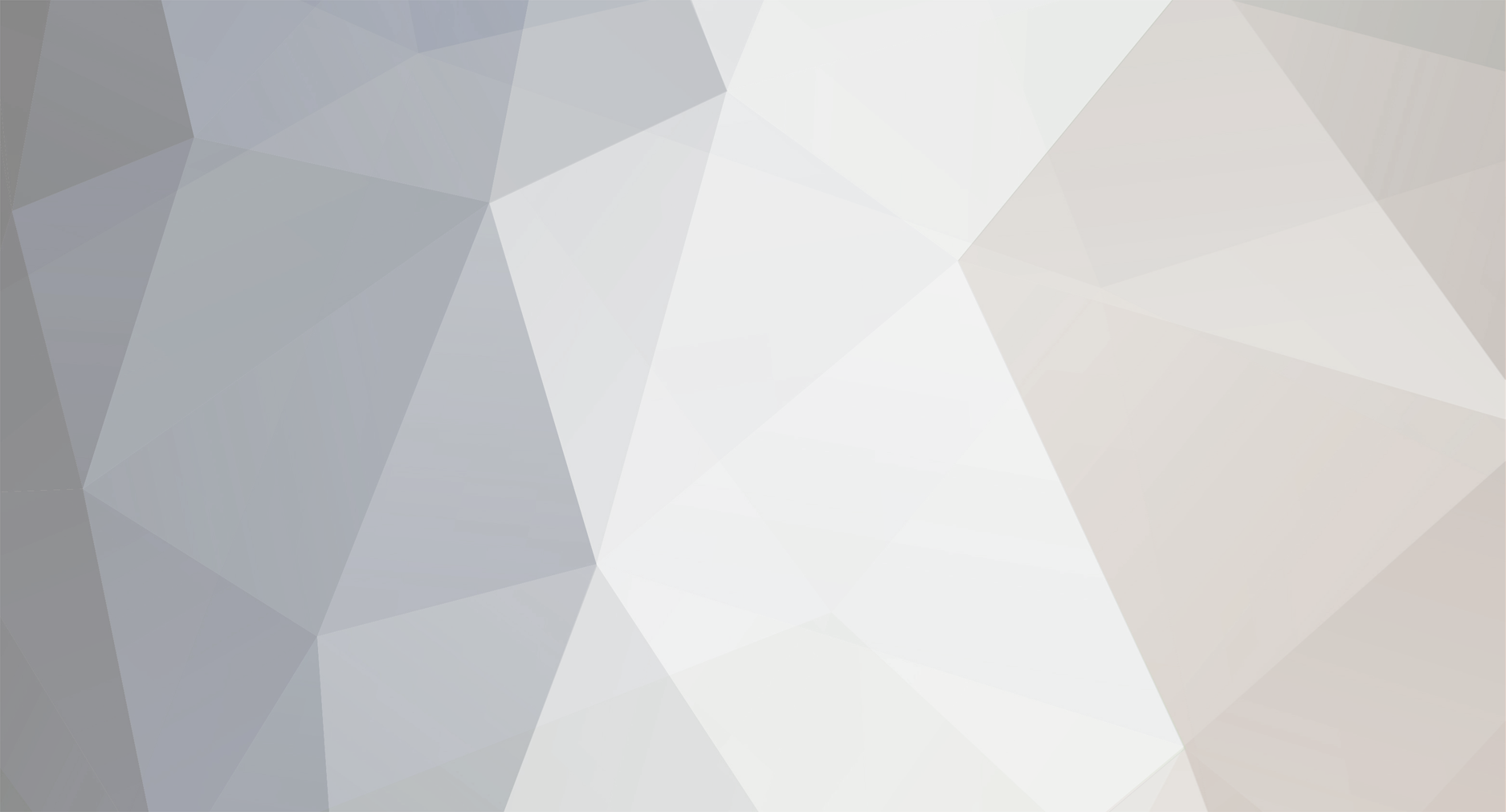
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Something like this: $arr = array('Value1', 'Value2', 'Value3'); $sql = "INSERT INTO tbl_name (`Col1`, `Col2`, `Col3`) VALUES('" . implode("','", $arr) . "')"; echo $sql; // Result: INSERT INTO tbl_name (`Col1`, `Col2`, `Col3`) VALUES('Value1','Value2','Value3') mysql_query($sql);
-
In that case phpMyAdmin is not reading your configuration you setup when you ran the PMA setup script. When you have finished the setup script a file is created in the scripts folder. You then have to move this file to the root of the phpMyAdmin folder. Either that or you can just copy config.default.php from the libraries folder and place it in the root of the phpMyAdmin folder and then rename it to config.inc.php and edit that file manually. You'll only have to change a couple of lines.
-
PHP is server side. You will need a server that has PHP setup. If you ahve a server with PHP then make sure you save your php code in .php files and not .html files.
-
You should check whether the variables have values first before using them and never use raw _POST/_GET data in a query. <?php // check that the form has been submitted // normally you'd do this by checking whether the variable // _POST['submit'] exists which will correspond to the forms submit button // for this to work make sure you ngive your submit button the name 'submit', eg: // <input type="submit" name="submit" value="Submit" /> if(isset($_POST['submit'])) { $errors = null; // check that variables exist first before using the if(!isset($_POST['university']) || empty($_POST['university'])) { // if the variable doesn't exist or the variable holds an empty value // set an error message $error[] = 'Univeristy not supplied'; } if(!isset($_POST['colledge']) || empty($_POST['colledge'])) { $error[] = 'Colledge not supplied'; } if(!isset($_POST['institute']) && empty($_POST['institute'])) { $error[] = 'Institute not supplied'; } // check that the errors variable is not any array if(!is_array($errors)) { // error variable is not an array // connect to mysql and select database $db = mysql_connect('localhost', 'root') or die('not connected'); mysql_select_db('trials', $db); // process submitted data // before you use raw _POST data in an query it is recommended to run data strings // from the user through mysql_real_escape_string function to pretect against SQL Injection attacks $uni = mysql_real_escape_string($_POST['university']); $col = mysql_real_escape_string($_POST['colledge']); $inst = mysql_real_escape_string($_POST['institute']); // setup the query $query = "INSERT INTO organizations VALUES('$uni','$col}','$inst')"; // run the query $result = mysql_query($query); // check for result if($result) { echo 'Data inserted into database'; } else { echo 'Error: Unable to add data into database<br />' . mysql_error(); } } // if the error variabble is not an array we'll display the errors: else { echo "Please correct the following errors:<br />\n!"; echo "<ul>\n<li>\n"; echo implode("</li>\n<li>", $errors); echo "</li>\n</ul>\n\n"; } } ?>
-
Rather than turn regsiter_globals on you should set error_reporting to E_ALL and turn display_errors on. That way PHP will spit out errors if there is any when the script runs.
-
You should not have to configure anything if you have XAMPP installed. What do you mean by "Its that extensions error."
-
If it cant start the service then there is most probably an existing service already setup with the same name as the one the installer is trying to start. Or there is something running on port 3306 (default mysql port) and service cannot start because of this. What I'd do is change the service, on the screen where you enter the root password there is an option for setting up a service and then a pull downmenu for service choose a different service name instead. When you uninstalled MySQL last time the uninstaller probably didn't remove the service.
-
Yes. By default Apache (the A in Xampp) serves htdocs folder as the document root. You can change this if you want to a different folder by editing the httpd.conf. There is no standard for what folders should be named.
-
[SOLVED] How do I break up using nl2br?
wildteen88 replied to westmatrix99's topic in PHP Coding Help
The record "about" just holds demo data. By code I ment the code you currently have for pulling AboutUs outof the database. You'll need to use tihs code: <table width="300" border="0" cellspacing="0" cellpadding="2"> <tr> <td><?php echo nl2br($row_rsabout['AboutUs']); ?></td> </tr> <tr> <td> </td> </tr> </table> Within a while loop when you have ran your sql query. -
[SOLVED] How do I break up using nl2br?
wildteen88 replied to westmatrix99's topic in PHP Coding Help
Oh you want to create the HTML for that page from the database. How is the $row_rsabout['about'] created what data does it hold. It might be helpful if you could post more code. -
This line: 'surname' => array mssql_bind(($query, "@Surname", $surname, SQLVARCHAR)); Needs to be 'surname' => array (mssql_bind($query, "@Surname", $surname, SQLVARCHAR))); You had your braces in the wrong placeand you forgot to add a closing ) brace for the parent array
-
Rather than check for which button has been pressed just make sure the form fields exist than run your code. Then after check which button has been submitted and redirect to the correct page, eg: if (isset($_POST['sectDropdown']) && isset($_POST['weight']) && isset($_POST['question']) && isset($_POST['quesType'])) { // run what ever code here for processing the form data // after processing the form data now check to see what button has been submitted if(isset($_POST['SubmitNext'])) { // rediect here when submitNext button has been pressed } elseif(isset($_POST['SubmitProceed'])) { // rediect here when submitProceed button has been pressed } }
-
[SOLVED] How do I break up using nl2br?
wildteen88 replied to westmatrix99's topic in PHP Coding Help
OK... and where do you want the data to be shown when you get it from the database? Can you highlight it on the page. -
You'll probably want to do some string processing on the $name variable, Not sure but try: $tmp_name = $_FILES['pictures']["tmp_name"][$key]; $name = $_FILES['pictures']["name"][$key]; list($filename, $ext) = explode('.', $name); $name = $filename . $key . $ext; move_uploaded_file( $tmp_name, "$garage_dir/$name" );
-
Use strip_html tags function. this function will strip all html tags posted.
-
Change this line: $rs_name = mysql_query($query_rs_name, $staff) or die(mysql_error()); to this: $rs_name = mysql_query($query_rs_name, $staff) or die('Error with query:<br>' . $query_rs_name . '<br>' . mysql_error()); The error should now show you the generated query from your code. Is the query correct?
-
[SOLVED] How do I break up using nl2br?
wildteen88 replied to westmatrix99's topic in PHP Coding Help
How is the tables laid out. Could you provide some example html or a simple image of what you mean. -
Need help..my script didn't work in PHP 5.2.1
wildteen88 replied to marmozzz's topic in PHP Coding Help
Looks like your site has a setting called regsiter_globals on. See if you can disable this setting as it is affecting your sessions. When register_globals is on PHP automatically registers variables from user data, eg instead of using $_SESSION['basket'] you can just use $basket straight away. Having regsiter_globals on can cause security exploits within your code. For now though change all $basket variables to $basket_id and your script should function as normal. But you should still try and get register_globals disabled. -
Those aren't mysql extensions and dont require to be enabled for use with MySQL. If you want to use those extensions then they will require third party libraries in order to work. You'll have to go to php.net and see what requirements those extensions requires.
-
Did you try it a url instead? I am pretty sure you can only use url parameters (?channel=$id - thats what I mean by a url parameter) within includes if you use a url path.
-
Do you need those extensions? If you don't just disable them in the php.ini by adding a ; in front of extension=php_xxx.dll line(change xxx to the extension name eg oci8 Save the ini and try again.
-
What happens if you use a web address in the include $id = trim($_GET['id']); include("http://mysite.com/includes/function_channels.php?channel=$id"); Change http://mysite.com/ to your websites address. I think you can only use url parameters if you are using a url path rather than a filesystem path.
-
Is the format of the number going to be xxx-xxxx, eg three numbers followed by a dash then four numbers If is you could use a bit regex: $var = '555-1234'; if(preg_match("/^([0-9]+){0,3}\-([0-9]+){0,4}/", $var)) { echo 'number'; } else { echo 'image'; }
-
Surely you'll need to return on this line: $this->getChildMess($mid, $userid, $x); as akitchin said. When PHP calls the new instance of the getChildMess function PHP will run through that function again and again causing an infinity recursion. I cannot see any logic
-
Can you run a php script with just phpinfo() in it for me and then scroll down and look for the following lines: url_fopen url_include (if it exists) What are their values?