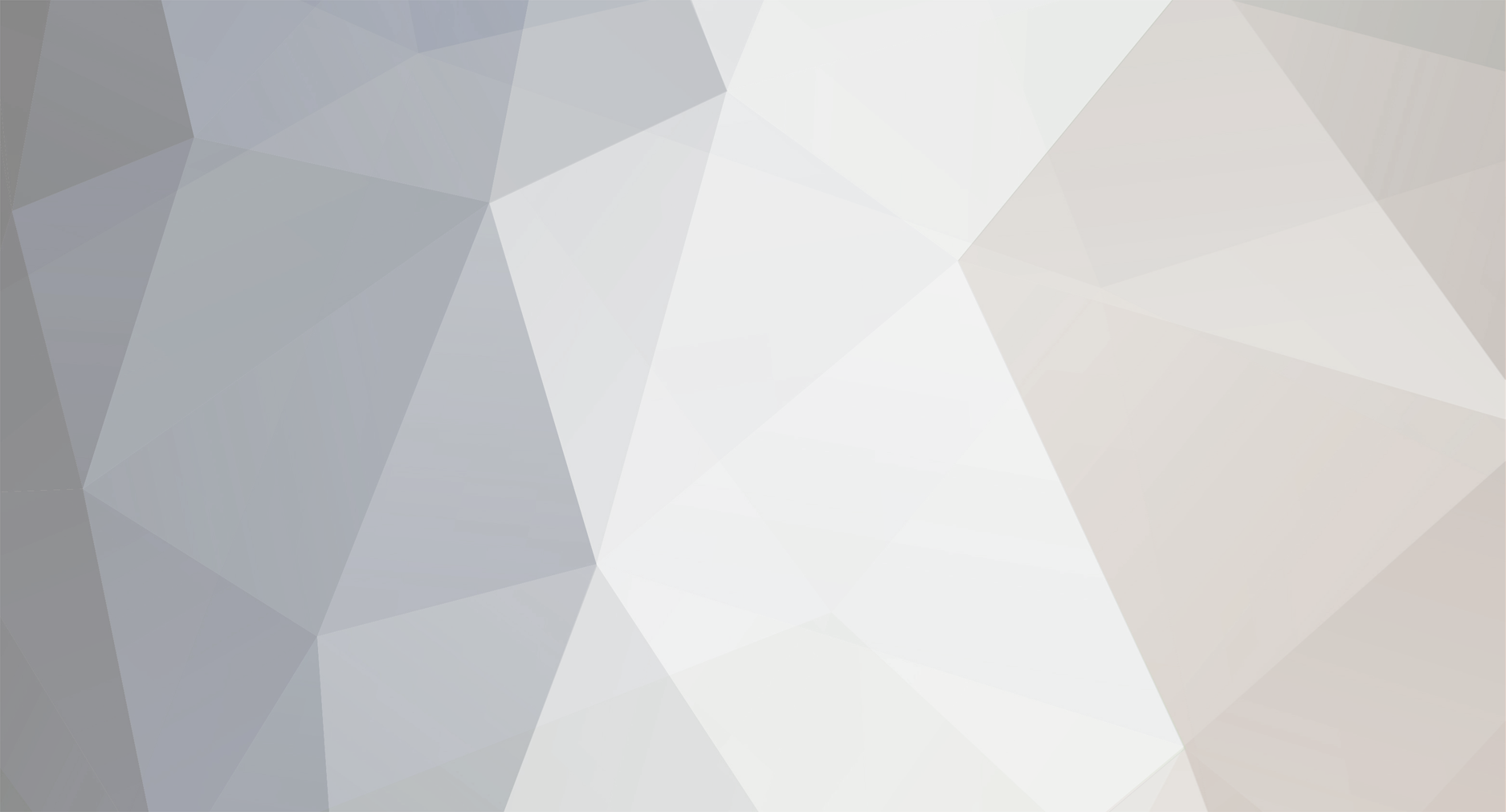
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
[SOLVED] problem with regular expressions or something
wildteen88 replied to psychohagis's topic in PHP Coding Help
If you are changing a timestamp into the following date format: DDth MMMM YYYY Then just change the format options in the first parameter for the date function. So if you want it in the above format do this: <?php $timestamp = time(); $date = date("jS F Y", $timestamp); // Output example: 1st January 2007 // OR // $date = date("D jS F Y", $timestamp); // Output example: Mon 1st January 2007 echo $date; ?> -
Have you checked that any of the above extensions need any more requirements. Such as external libraries (.dll) or extra packages to be installed. You might want to check over at php.net or whereever you got those extensions from. From looking at the list you are using extensions that are not included by default, looks like you have downloaded them from pecl.php.net
-
Open your php.ini file and turn display_errors on and make sure error_reporting is set to E_ALL Save the php.ini and restart IIS. Re-run your script. If there is any errors PHP finds they will now be displayed to the browser. Post all error messages you get here in full.
-
Your are best of adding the PHP folder (C:\php) to the windows PATH. To do so. Go to start > control panel > system Click the advanced tab and then click the environment variables button. Next scroll down the list of the environment variables until you find the PATH variable. Select this variable and then press the Edit button. A dialog box should open up allowing you to edit the variable. Now press the End key on your keyboard and type the following exactly: ;C;\php Make sure you do not delete anything that is already in the text box. Close all open dialog boxes, making sure your click OK and not cancel to close them. When all dialog boxes are closed restart Windows. When you restart windows PHP should now be able to access itself. All extensions you load up in the php.ini should now load with out problems.
-
Are the variables you use in the function defined outside of the function? if they are then this is why. Functions have there own variable scope. Functions cannot use functions that was defined outside of it, unless they passed as a parameter to the function or you define the variables as global when you set the function. Examples: Global <?php $myvar = 'hello world'; function myfunc() { global $myvar; // myvar is now global echo $myvar; } myfunc(); ?> Parameter <?php $myvar = 'hello world'; function myfunc($var1) { // $var is a parameter. Functions can have multipl parameters. // each parameter is separated by a comma. echo $var1; } myfunc($myvar); ?>
-
Don't use a system path when displaying the image. Use a HTML path. System path: C:/path/to/something HTML path: http://www.mysite.com/path./to/something (absolute) OR ./path/to/something (relative) It is fine using a system path for uploading the image, but not displaying it. Also firefox uses the file:// protocol in order to access files off of the users file system.
-
[SOLVED] quite tricky, .. can you please help me ..
wildteen88 replied to jaikar's topic in PHP Coding Help
That is correct. PHP will treat any PHP code coming out of a database literally. As when you fetch something from the database PHP will treat it as a string, depending on the data type you use for your columns. -
You have the rewrite rule set-up wrong. It should be: RewriteRule ^page/([A-Za-z]+)/$ page\.php?name=$1
-
[SOLVED] quite tricky, .. can you please help me ..
wildteen88 replied to jaikar's topic in PHP Coding Help
You can only call variables from within double quotes and not single quotes. If you are storing PHP code in a database then you will need to use eval() in order to process the PHP code in the database. -
I forgot to add an example in my post earlier. Re-check my post. It has an example now. If you want to round up change floor to ceil instead.
-
If you want to get whole numbers and not decimal numbers use ceil (for rounding up) or floor (rounding down). Eg: $sum = 10 / 3; echo floor($sum); // result: 3
-
How to remove links from a layer containing php
wildteen88 replied to adamddickinson's topic in PHP Coding Help
You can use PHP to remove html from strings. PHP has a few methods to do this. It depends on how you get the data. -
You place all .php (this is what you save your php scripts as not as .html) files in Apaches htdocs folder (Default path: C:/Program Files/Apache Group/Apache2/htdocs). Whenever you want to run your PHP script you go to http://localhost/path/to/script.php You can place all other files too in htdocs folder if you wish. If the books good enough it should explain to you all this, most bocks have an extra chapter in the Appendix for setting up AMP.
-
That made me giggle when I saw that on digg. If you want to print any page of that site it will cost you $5k!
-
Umm, you can clearly see who's a master in regex here.
-
isset checks to see whether the variable exists or not. It does not check the variables value. Umm, frost beat me \0/
-
Something like this: <?php $string = "7/2"; preg_match("#([\d]+)/([\d])([A-Z]+){0,}#", $string, $matches); echo '<pre>' . print_r($matches, true) . '</pre>'; echo ' x = ' . $matches[1] . '<br /> y = ' . $matches[2] . '<br /> z = ' . (empty($matches[3]) ? 'N/A' : $matches[3]); ?>
-
Not php for this. Just a bit of javascript. Hop over to dynamicdrive.com for such a script. If I remember correctly they have plenty of image loading scripts.
-
If you want to create an editor like this search google for BBCode editors. The editor uses javascript to insert the bbcodes in to the textarea. However in order to parse the BBCodes that will require PHP. Look in to the regex board and you see a few topics about BBCode parsers.
-
You sure products.product_cat holds a keyword. Could you post your table scheme for the products table here. If product_cat holds a keyword then it should work. Make sure the keywords you use in the URL and the product_cat column is in the same case. Database matches are not case-insensitive. Type your keywords in lowercase to be on the safe side.
-
So what's wrong with your query? Earlier you was talking about products.product_dis
-
Why out the link in a code box for monkey? Code boxes are for code not links
-
Something like this?: <?php if(isset($_POST['cities']) && !empty($_POST['cities'])) { $cities_list = $_POST['cities']; // put each city on its own in an array. $cities = explode("\n", $cities_list); // now we format how we want the cities to be added to the txt file $city_list = ''; foreach($cities as $city) { $city_list .= '"' . trim($city) . '", '; } //clean up the cities_listed sting $city_list = substr($city_list, 0, (strlen($city_list)-2)); echo 'Cities to be added:<br />' . $city_list . '<br /><hr />'; // open file read for writing $city_file = fopen('cities.txt', 'a'); // make sure file is writeable if(is_writable('cities.txt')) { // write cities list to file fwrite($city_file, $city_list . "\r\n"); // close the file fclose($city_file); } else { echo 'Unable to write to file!'; } } ?> <form action="" method="post"> Cities:<br /><textarea name="cities"></textarea><br /> <input type="submit" name="submit" value="Add Cities!" /> </form> Add each city on a new line in the textarea. Submit the form it will format the string to be entered into the text file for you, the format entered into the text file would be like this: "city1, "city2", "city3", "exct...." It will add each group of cities you submit on a new line in the text file too.
-
what does products.product_cat hold? You are using that column in your WHERE clause. DO you mean products.product_dis instead? Also make sure you validate what is coming from _GET['id']. A malicious user could do SQL Injection attacks. Never use raw user input _POST, _GET etc in SQL queries.
-
How do you get the list of cities? Does it come from a textarea which the user submits and each city is on a new line? I need more info in order to provide working code for you.