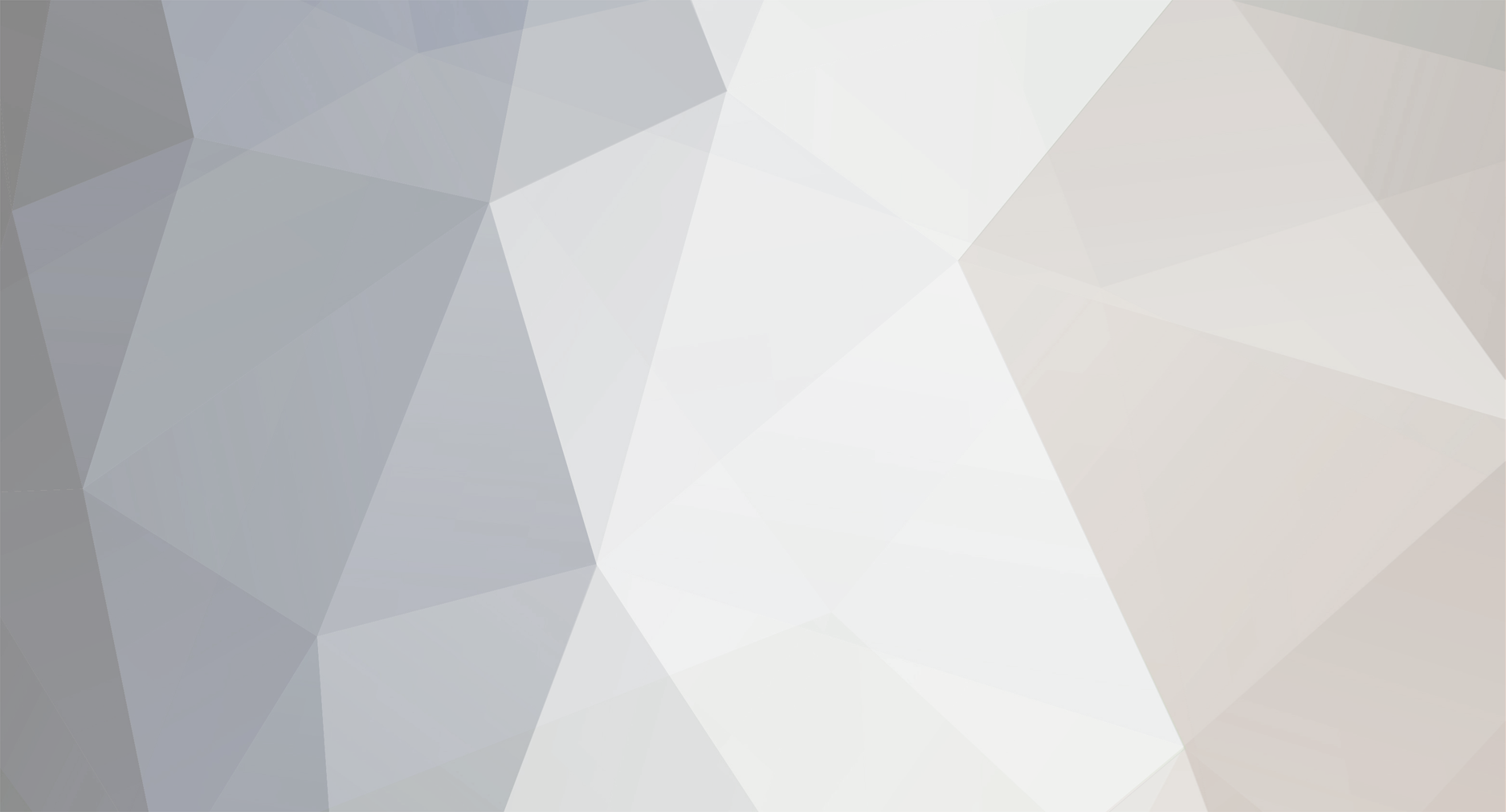
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
You should use the following conditions instead RewriteCond %{REQUEST_FILENAME} !-d RewriteCond %{REQUEST_FILENAME} !-f
-
Noob help to extract username and password from api reply
wildteen88 replied to thernes's topic in PHP Coding Help
If the username and password are separated by a comma use explode Example: $text = 'username,password'; list($username, $password) = explode(',', $text); echo 'Username is: ' . $username . '<br />'; echo 'Password is: ' . $password; -
You can define the width of the table using the width html attribute. The same attribute can be used to define the width of your columns too.
-
To add up arrays use array_sum. Also when use variables with functions you should pass them as paramaters. Making functions use variables in the global scope is bad practice. $start = array($melon,$pear); $main = array($chicken,$pork); sum($start, $main) function sum($start, $main) { $total = array_sum($start) + array_sum($main); echo $total; }
-
The message you linked to copied 'n pasted fine for me. I sometimes get odd occurrences where code is massively spaced out too. I guess there is a bug in the code parser or something. We can only hope the SMF devs fix this issue on the next release candidate.
-
Your code will not output anything as all you're doing is defining a bunch of variables based on what was selected in your form. You have defined a function called sum which is not being called.
-
No you wont need to check that the $error variable exists when resetting the password. All your code for resetting the password will be placed in the else statement else { // A match was found, reset the password for the given username here } The else statement will ONLY be executed if the query returned a match on the username/email address.
-
You should modify your where clause so it also checks the email $query = mysql_query("SELECT * FROM Users WHERE Username = '$username' AND Email='$email") or trigger_error("Query failed: ".mysql_error()); Now to see if the your query matched a row that contains the same username/email combination provided you'd use mysql_num_rows $query = mysql_query("SELECT * FROM Users WHERE Username = '$username' AND Email='$email' LIMIT 1") or trigger_error("Query failed: ".mysql_error()); // if no rows was returned, the display an error. Username/email does not exist if(mysql_num_rows() == 0) { echo 'The Username/Email address provided does not exist'; } else { // A match was found, reset the password for the given username here }
-
Use strpos
-
Although this function is for parsing configuration files. You could use the parse_ini_file function if your text file is in this format myContent = Hello, World anotherLine = Testing 123.. oneMoreLine = 'A multi-line something here' justForLuck = whatever here With the following code $line = parse_ini_file('yourfile.txt'); You can easily display a certain line using the $line variable, eg echo $line['myContent'] // first line echo $line['justForLuck'] // last line etc
-
This is should work fine RewriteEngine On RewriteCond %{REQUEST_FILENAME} !-d RewriteCond %{REQUEST_FILENAME} !-f RewriteRule ^([a-zA-Z0-9-]+)/([a-zA-Z0-9]+)/?$ /index.php?page=$1&act=$2 [L] RewriteRule ^([a-zA-Z0-9-]+)/?$ /index.php?page=$1 [L]
-
This is causing the error //RewriteRule ^([A-Za-z0-9-]+)/?$ index.php?page=$1 [L] You use # to comment out lines, not //
-
Oh, I thought you where reading the lines from a file. You'd want to use explode instead $words = str_replace(array("\r\n", "\r"), "\n", $_POST['words']); // convert the list of words into an array $wordsArray = explode("\n", $words); echo '<pre>'.print_r($wordsArray, true).'</pre>';
-
Have a look in to the file function.
-
Name you check boxes as delete[] <input type="checkbox" name="delete[]" value="2"> This will make the $_POST['delete'] variable hold an array of selected checkboxes. Now use the following code to delete the comments that are selected if(is_array($_POST['delete'])) { $ids = implode(',', $_POST['delete']); $sql = 'DELETE FROM comments_table WHERE id IN('.$ids.')'; mysql_query($sql); }
-
No it doesn't. Did you look in DB-1.7.14RC1/DB folder. You'll see there is a load of .php files there. Move the DB folder to your PEAR folder.
-
"DAY 2", "1 book read" and STILL showing ERROR, Please Help?
wildteen88 replied to Modernvox's topic in PHP Coding Help
Just looking at your code I can see multiple syntax errors First is line 1 <php That is an invalid tag, It should be <?php Line 21 - 22 is incorrect mysql_select_db($database); if (!mysql_select_db) It should be Line 41 ); I don't know what that is? Delete it You have a missing closing brace } after line 45 44. if (!$result){ 45. die ("Could not query the database: <br />". mysql_error()); 46. } Line 46 is incorrect mysql_close)$connection) It should be mysql_close($dbx); -
You have an error with your SQL query. Use mysql_error to find out why.
-
You need to pass your sql query to mysql_query first! $sql="SELECT amount FROM payments WHERE user='".$_SESSION['user']."' AND member_id='".$_SESSION['id']."'"; if (mysql_num_rows($sql) > 0) {
-
PHP code does not need to be placed within the HTML head tags. Move your PHP code before the <html> tag, eg <?php require("config.php"); session_start(); if ($_POST['submit']=='login') { $username="$_POST[username]"; $password="$_POST[password]"; $sql="SELECT username FROM users where username='$username' AND password='$password'"; $result = mysql_query($sql) or die (mysql_error()); $num = mysql_num_rows($result); if ( $num != 0 ) { // A matching row was found - the user is authenticated. list($username) = mysql_fetch_row($result); // this sets variables in the session $_SESSION['user']= $username; header("Location: Content.html"); //echo "Logged in..."; exit(); } header("Location: login.php?msg=Invalid Login"); } ?> <html> <head> <meta http-equiv="Content-Language" content="en-us"> <meta name="GENERATOR" content="Microsoft FrontPage 5.0"> <meta name="ProgId" content="FrontPage.Editor.Document"> <meta http-equiv="Content-Type" content="text/html; charset=windows-1252"> <title>Login Page</title> You cannot use session_start or header before any output is sent to the browser. Moving the php code to the top of the page will fix your error.
-
Add a ? after \s That way it will match either as1233 or as 1233
-
To output the contents of an array you need to use print_r eg echo '<pre>'.print_r($file,true).'</pre>';
-
\s doesn't strip white space. \s matches any whitespace character, eg a space, newline, tab etc. To match a space add \s before [0-9] eg ("^[[a-zA-Z]{2}\s[0-9]{4}]$") Alternatively you could just add a space instead ("^[[a-zA-Z]{2} [0-9]{4}]$")
-
I cant tell you what is wrong without seeing your code.