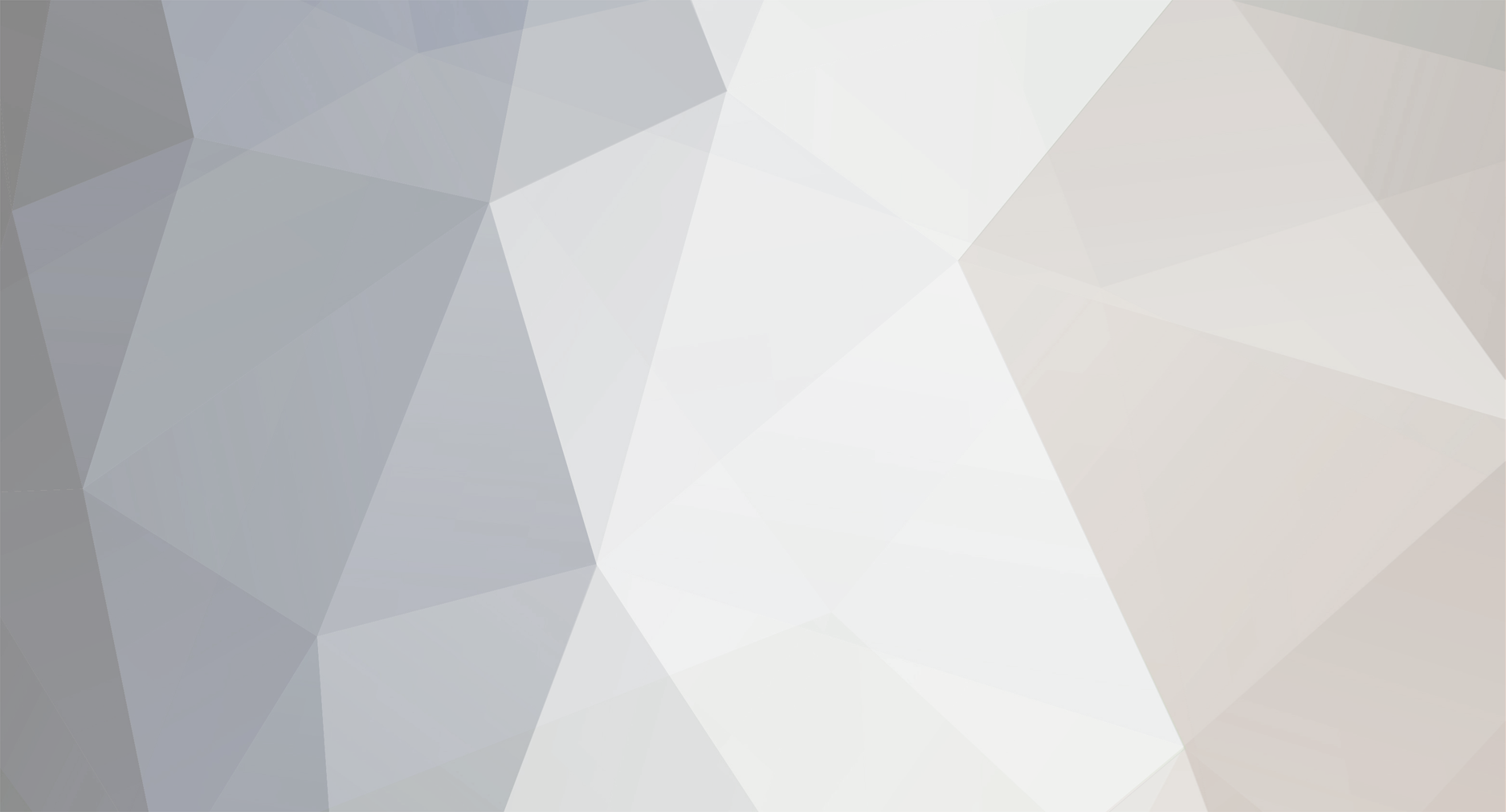
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
The problem is each Operating Systems defines a new line in different ways. For example a new line in Windows is defined by \r\n, On Mac OS its \r and Unix its \n You may want to use regular expressions for this instead. Eg $article = substr($article, 0, 1000); $article = "<p>" . preg_replace("~(\r\n|\r|\n){1,}~s", "</p>$1<p>", $article) . "</p>";
-
Functions have their own variable scrope. Which means any variables defined outside of them will not be accessible. When using variables with functions you should pass them as arguments. For example $ids= isset($_GET['ids'])?(int) $_GET['ids']:null; $idc= isset($_POST['idc'])?(int)$_POST['idc']:null; function ShowCart($ids, $idc) { // $ids and $idc are not numbers if(!is_numeric($ids) || !is_numeric($idc)) { // exit the function, return false return false; } // continue with function }
-
Or you could use nl2br. Which converts new lines to <br /> tags.
-
Display PHP Array Value Inside HTML Form Text Box
wildteen88 replied to jponte's topic in PHP Coding Help
That is completely wrong. The reason why 'Sander' will only appear in the input box is probably because you're not wrapping your HTML attribute values in quotes. Wrong $somevar = "Sander van Doorn"; echo "<input type=text name=somename value=$somevar />"; The above will result in just 'Sande' being showing in the text box. It will treat 'van' and 'Doorn' as HTML attributes. All attribute values SHOULD be wrapped in quotes to prevent problems like this. The correct way is $somevar = "Sander van Doorn"; echo "<input type=\"text\" name=\"somename\" value=\"$somevar\" />"; //OR echo '<input type="text" name="somename" value="'.$somevar.'" />'; I doubt it. Do you even know what nl2br is used for. -
This line has a syntax error. Remove the ; (semi-colon) after the else else;{ Other than that issue above your code will function as you described.
-
Display PHP Array Value Inside HTML Form Text Box
wildteen88 replied to jponte's topic in PHP Coding Help
No the correct way is <td width="274"><input name="ShipAttention" type="text" size="45" VALUE="<?php echo $info['Cust_ID'] ?>"></td> NOTE: Tags such as <? ?> and <?= ?> may not always work as a setting called short_open_tag may be disabled. Always use the full PHP tags, eg <?php ?> -
Delete $row = mysql_fetch_array($result); Now the following line while($row) should be while($row = mysql_fetch_array($result))
-
header can also not be used after any output. Line 182 header((($myusername == "AdMiN") ? "location:my_account.php" : "location:my_account.php")); Also don't use these functions, they are no longer supported and soon to be removed completely. session_register("myusername"); session_register("mypassword");
-
Your have output on line 114 in check_login.php You cannot use session_start() after any output, this also includes any files being included too.
-
Really need some help with this one Guyz??
wildteen88 replied to Modernvox's topic in PHP Coding Help
Its probably a pain the ass to you because you don't understanding it. To me CSS is actually quite simple. I wont say any more. -
Change <form id="form1" name="form1" method="post" action=""> To <form id="form1" name="form1" method="post" action="comment2.php?id=<?php echo $id; ?>"> Now change if(isset($_POST['submit'])) to if(isset($_POST['submit'], $_GET['id']) && is_numeric($_GET['id'])) Also change $postid = (int) $_POST['id']; to $postid = (int) $_GET['id'];
-
How do I extract the data from my database query?
wildteen88 replied to Canadian's topic in PHP Coding Help
If you're reading the book it should be explaining to you how to use the mysqli class properly, maybe you skipped too far in the book or missed a chapter. If you're stuck at any time on how to use a PHP function then have read of the PHP manual on that particular function. So for all documention on mysqli is here. You can use the built in mysqli class or use mysqli procedurally. -
strtotime() will only work properly on US English date formats, for example 15 January 2009 08:41:03. So you will have to expand the month to its full name, so instead of feb it will need to be February. You'll also need to loose the ordinal suffix (st, nd, rd or th) too from your original dates. With your dates now in the format strtotime expects you can do this $fromDate = '15 February 2010 05:00:00'; $toDate = date('j F Y H:i:s', strtotime('+23 hours 59 minutes', strtotime($fromDate))); echo "$fromDate to $toDate";
-
Really need some help with this one Guyz??
wildteen88 replied to Modernvox's topic in PHP Coding Help
Yes that would be the correct code. However not sure what '<font size=\"3\"></font>' is for. You should use CSS for formatting your text. Just echo out the necessary variable, eg $venue['field_name_here'] -
Ops. All instances of mysql_real_escape_sting should be mysql_real_escape_string I mistype the function name.
-
How do I extract the data from my database query?
wildteen88 replied to Canadian's topic in PHP Coding Help
I guess so. I cant tell you the exact method you need to use in order to extract your results from your query. You seem be using a database class object. What is the database class you're using? Is it a custom class or the built in mysqli class? -
As you are getting the id from the url? All values in the query string are sent as strings. is_int will expect an integer value. So you're better of using is_numeric when checking to see if $_GET['id'] is a number. You'd then type cast $_GET['id'] (or use intval) to convert it to an integer. For example if(isset($_GET['id']) && is_numeric($_GET['id'])) { $id = (int) $_GET['id']; }
-
Really need some help with this one Guyz??
wildteen88 replied to Modernvox's topic in PHP Coding Help
File path should be enclosed in quotes. This is wrong require_once(db_cred_all.inc); it should be require_once('db_cred_all.inc'); This portion of code is incorrect $category= isset($_POST['category']); if ($category== "venues") $result = mysql_query(SELECT * FRom venue WHERE state = '$st'"); While($venue = mysql_fetch_array($result)) { echo $title['title'] . " " . $actual_location['actual_location']; echo "<br />"; //////How to only display Title and actual_location initially as a link and than the rest on next page? } It should be if(isset($_POST['category'])) { $category = $_POST['category']; if ($category == "venues") { $result = mysql_query("SELECT * FROM venue WHERE state = '$st'"); While($venue = mysql_fetch_array($result)) { echo $venue['title'] . " " . $venue['actual_location']; echo "<br />"; } } } -
The main problem is this error For some reason your code is not connecting to your database. Try the following code, I have merged comment.php with write-comment.php. Place the following code in comment.php <?php $con = mysql_connect("localhost","username","password"); if (!$con) die('Could not connect: ' . mysql_error()); mysql_select_db("databasename", $con); if(isset($_POST['submit'])) { $name = mysql_real_escape_sting($_POST['name']); $mail = mysql_real_escape_sting($_POST['mail']); $commen = mysql_real_escape_sting($_POST['comment']); $postid = (int) $_POST['id']; if( (!empty($name)) && (!empty($mail)) && (!empty($comment)) ) { $sql = "INSERT INTO prototype (name, mail, comment, postid) VALUES('$name', '$mail', '$comment', '$postid')"; mysql_query($sql) or die('Unable to insert comment: '. mysql_error()); } } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> </head> <body> <?php if(isset($_REQUEST["id"]) && is_numeric($_REQUEST["id"])) { $id = (int) $_REQUEST["id"]; $result = mysql_query ("SELECT * FROM commenttable WHERE postid='$id' ORDER BY id desc") or die ('Unable to grab comments: '.mysql_error()); while($row = mysql_fetch_array($result)); { echo $row['name']; echo $row['mail']; echo $row['comment']; echo $row['timestamp']; } } ?> <form id="form1" name="form1" method="post" action=""> Name: <input name="name" type="text" /> <br /> Mail: <input name="mail" type="text" /> <br /> Kommentar: <textarea type="text" name="comment" cols="50" rows="6"></textarea> <br /> <input name="submit" type="submit" class="submit" value="Send"/> </form> </body> </html>
-
Yes ofcourse. Look at this FAQ post for an example.
-
That can be used for PHP too. Rather then setting the link to remote.html change it to remote.php
-
And... the problem is what? What are you trying to do? What errors are you getting etc. Need more information about your problem
-
If you're getting a blank screen then it is more than likely there is an error in your code. In order to see the error you need to enable a PHP setting call display_errors and set error_reporting to E_ALL within your php.ini. If your host doesn't allow access to the php.ini then add the following two lines at the top of your php script (on the line after the opening PHP tag <?php) error_reporting(E_ALL); ini_set('display_errors', 1); Post the errors you're getting here.
-
You need to include the Price_ExVat column in your SELECT statement, eg: $sqlSelect = "SELECT Prod_ID, Price_ExVat ...etc Now in your while loop echo out $row['Price_ExVat'] where you want the price to be displayed. Like so while ($row = mysql_fetch_assoc($result)) { echo "£{$row['Price_ExVat']} <a href=\"basket.php?src=".urlencode($_SERVER['REQUEST_URI'])."&productID=" . $row["Prod_ID"] . "\"><img src=\"images/addtobasket.jpg\" width=\"55\" height=\"28\" border=\"0\"></a>"; //end make while }
-
Using data entered in text field to redirect page
wildteen88 replied to nerotic's topic in PHP Coding Help
You need to be using $_POST['storeentry'] not $storeentry