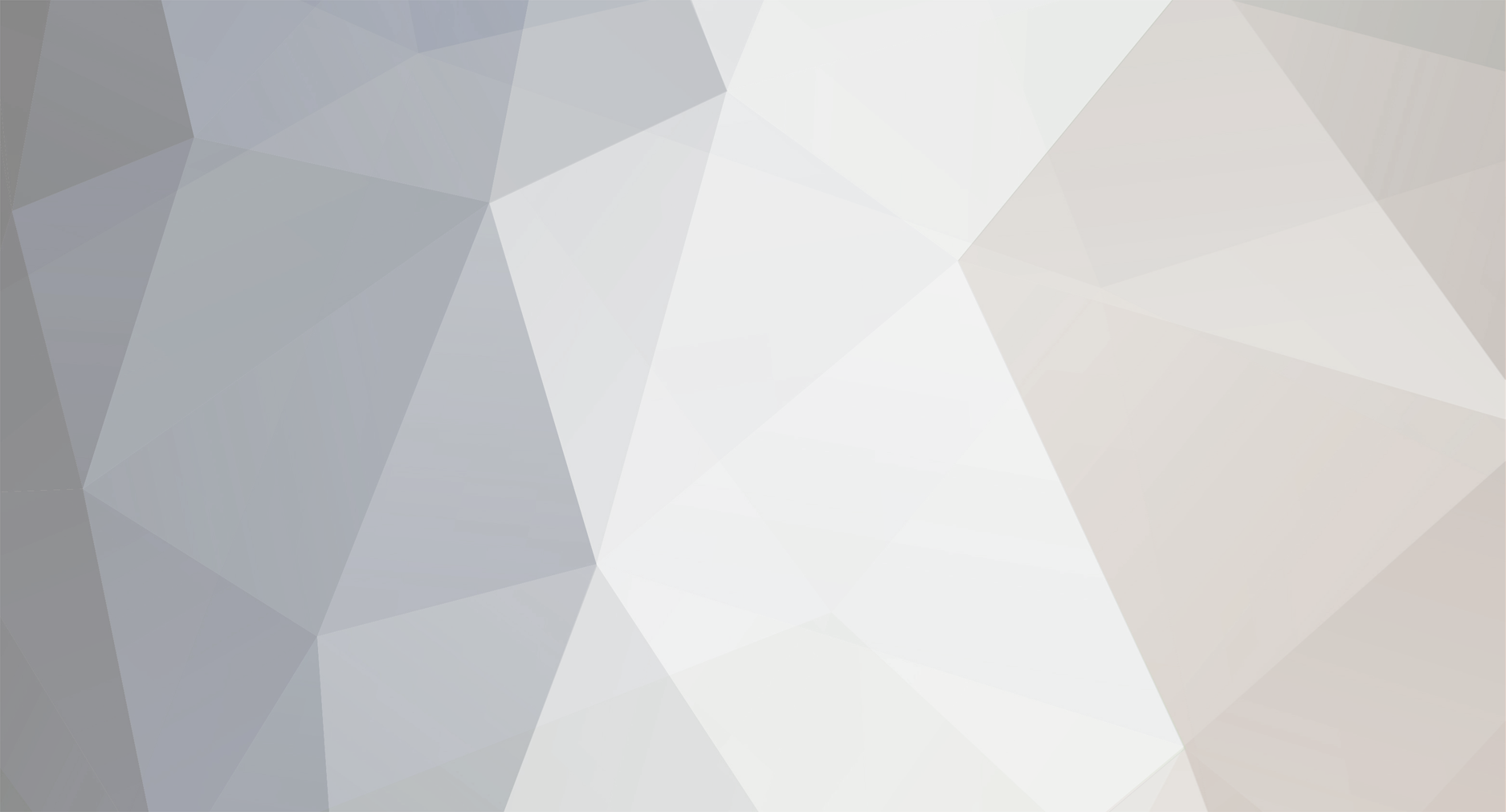
Tom10
Members-
Posts
108 -
Joined
-
Last visited
Everything posted by Tom10
-
Hi, ok so basically i have a forum and want to use a character class to detect if someone is trying to post a thread with a malicious title or message like xss, i have already used a regex in the url to filter characters $url = $_SERVER['SERVER_NAME'] . $_SERVER['REQUEST_URI'] . ''; if(preg_match("#[^\w\s\b\/\&\?\=\-\.\%\_\[\]]#", $url)) { include 'denied.php'; exit(); } But i'm wondering how can i do something like this for a page? if that makes sense All help very much appreciated, i'm not the best at explaining so if something doesn't make sense please ask and i will try to explain in more detail
-
<?php session_start(); error_reporting(E_ALL | E_NOTICE); ini_set('display_errors', 1); require 'connect.php'; // Use $_SERVER['REQUEST_METHOD'] // Here is an article on why you shouldn't use $_POST['submit'] // http://stackoverflow.com/questions/10943060/isset-postsubmit-vs-serverrequest-method-post#comment14373814_10943179 // Read those comments if($_SERVER['REQUEST_METHOD'] == "POST") { $disallowed = "<h1>Your username has disallowed characters!</h1>".PHP_EOL."Please make sure that you have no spaces or characters such as:<h3><li>".PHP_EOL." \"\!\^\$\*\@\#\~ </li></h3>".PHP_EOL."<li><h3>Also make sure that there are no spaces in your username.</h3></li>".PHP_EOL."Thanks, ".PHP_EOL." System Administrator."; $username = $_POST['username']; if(preg_match("#[^\w\b]#", $username)) { echo $disallowed; exit(); } // The above is only needed for the username. By limiting the amount of characters the user can use can result in weak passwords. By allowing them to use such characters !^$*@#, they strengthening their password. You don't need to whitelist these characters because SQL injections does not come from user input, it comes from bad codes. $stmt = $con->prepare("SELECT username, password, status FROM users WHERE username = ?"); // Just only need the username so we can verify the password $stmt->bind_param("s", $username); // Bind the variables so there won't be any SQL injections $stmt->execute(); // Executes the query $stmt->store_result(); // Stores the results for later checking // Checks to see if the query has returned a row if($stmt->num_rows) { // The username exists $post_password = $_POST['password']; // We finally check for the password because we only needed the username in the beginning $hash = password_hash($post_password, PASSWORD_DEFAULT); $stmt->bind_result($username, $password, $status); while($stmt->fetch()) if(password_verify($post_password, $password)) { echo "Success!"; // The account exists with the right password } else { echo "Wrong password"; // The wrong password has been typed in } } else { echo "No Account"; // This should be a custom error saying that the account does not exist or something like they have typed int he wrong password and username } } ?> <html lang="en"><meta charset="utf-8"><head></head><title>Login to your account!</title> <body> <center> <span style="font-size: 32px; font-weight: bold; font-family: Arial; padding; 10px;"> Please Login </span> </center> <link rel="stylesheet" href="css/login.css" /> <div class="login"> <center> <form action="" method="POST"> <br><br>Username: <br><input type="text" name="username" placeholder="Username" required /> <br><br>Paswsord: <br><input type="password" name="password" placeholder="Password" required /> <br><br><input type="submit" name="submit" value="Login to your account!" /> </center> </div> </form> </body> </html> It says wrong password
-
Also i've never understood while loops
-
But your code makes a lot of sense and i will make changes
-
I got my code to work, i'm having problems with the status though <?php session_start(); error_reporting(E_ALL | E_NOTICE); ini_set('display_errors', 1); require 'connect.php'; if(isset($_POST['submit'])) { $disallowed = "<h1>Your username or password has disallowed characters!</h1>".PHP_EOL."Please make sure that you have no spaces or characters such as:<h3><li>".PHP_EOL." \"\!\^\$\*\@\#\~ </li></h3>".PHP_EOL."<li><h3>Also make sure that there are no spaces in your username or password.</h3></li>".PHP_EOL."Thanks, ".PHP_EOL." System Administrator."; $username = $_POST['username']; $password = $_POST['password']; if(preg_match("#[^\w\b]#", $username)) { echo $disallowed; exit(); } if(preg_match("#[^\w\b]#", $password)) { echo $disallowed; exit(); } $password = hash('gost-crypto', $password); $stmt = $con->prepare("SELECT username, password FROM users WHERE username = ? AND password = ?"); $stmt->bind_param("ss", $username, $password); $stmt->execute(); $stmt->store_result(); $status = $stmt->fetch(); if($stmt->num_rows > 0) { if($status < 1) { header("Location: banned.php"); } else { header("Location: user.php"); } } else { echo "Your username or password is incorrect. Please try again."; } } $con->close(); ?> <html lang="en"><meta charset="utf-8"><head></head><title>Login to your account!</title> <body> <center> <span style="font-size: 32px; font-weight: bold; font-family: Arial; padding; 10px;"> Please Login </span> </center> <link rel="stylesheet" href="css/login.css" /> <div class="login"> <center> <form action="" method="POST"> <br><br>Username: <br><input type="text" name="username" placeholder="Username" required /> <br><br>Paswsord: <br><input type="password" name="password" placeholder="Password" required /> <br><br><input type="submit" name="submit" value="Login to your account!" /> </center> </div> </form> </body> </html>
-
<?php session_start(); error_reporting(E_ALL | E_NOTICE); ini_set('display_errors', 1); require 'connect.php'; if(isset($_POST['submit'])) { $disallowed = "<h1>Your username or password has disallowed characters!</h1>".PHP_EOL."Please make sure that you have no spaces or characters such as:<h3><li>".PHP_EOL." \"\!\^\$\*\@\#\~ </li></h3>".PHP_EOL."<li><h3>Also make sure that there are no spaces in your username or password.</h3></li>".PHP_EOL."Thanks, ".PHP_EOL." System Administrator."; $username = $_POST['username']; $password = $_POST['password']; if(preg_match("#[^\w\b]#", $username)) { echo $disallowed; exit(); } if(preg_match("#[^\w\b]#", $password)) { echo $disallowed; exit(); } $password = hash('gost-crypto', $password); $stmt = $con->prepare("SELECT username, password FROM users WHERE username = ? AND password = ?"); $stmt->bind_param("ss", $username, $password); $stmt->execute(); $stmt->store_result(); if($stmt->num_rows) { $stmt->bind_result($username, $password, $status); while($stmt->fetch()) if($status == 0) { echo "Banned"; } else { echo "regular user"; } } else { echo "no"; } } ?> <html lang="en"><meta charset="utf-8"><head></head><title>Login to your account!</title> <body> <center> <span style="font-size: 32px; font-weight: bold; font-family: Arial; padding; 10px;"> Please Login </span> </center> <link rel="stylesheet" href="css/login.css" /> <div class="login"> <center> <form action="" method="POST"> <br><br>Username: <br><input type="text" name="username" placeholder="Username" required /> <br><br>Paswsord: <br><input type="password" name="password" placeholder="Password" required /> <br><br><input type="submit" name="submit" value="Login to your account!" /> </center> </div> </form> </body> </html>
-
This part is not working correctly if($status == 0) { echo "banned."; } else { echo "notbanned."; } the status column in the table is set to 0 and it says the account is not banned.
-
$password = hash('gost-crypto', $password); $stmt = $con->prepare("SELECT username, password, status FROM users WHERE username=? AND password=? AND status=?"); $stmt->bind_param("ssi", $username, $password, $status); $stmt->execute(); $res = $stmt->get_result(); $status = $res->fetch_assoc(); $res->num_rows; if($res->num_rows > 0) { if($status = "0") { echo "banned."; } else { echo "notbanned."; } I can't get this to work i'm trying to check the status of an account
-
Never mind i found what i did wrong i changed if($stmt) to if($res->num_rows > 0) and also i forgot to add the hash algorithm in the login script
-
I'm trying to make a login script using prepared statements $stmt = $con->prepare("SELECT username, password FROM users WHERE username=? AND password=?"); $stmt->bind_param("ss", $username, $password); $stmt->execute(); $res = $stmt->get_result(); $res->num_rows; if($stmt) { echo "yes"; } else { echo "no"; } Since i cannot actually get it to login i'm just getting it to echo yes or no to check if the statement is working, if i type the correct username but the wrong password it echo's yes
-
Thanks, Barand i appreciate your help
-
I'm trying to use a prepared statement to check if a username exists please can someone explain prepared statements to me i've been on php.net and still don't understand the statement: $ucheck = $con->prepare("SELECT * FROM users WHERE username=?"); $ucheck->bind_param("s", $username); $ucheck->execute(); $ucheck->num_rows(); if(mysqli_num_rows($con) > 0) { echo "The username:".$username."already exists!"; exit(); } $username = $_POST['username'];
-
Okay forget my register.php page turns out the server needed time to update the file
-
Doesn't work mate thanks a lot though i appreciate any help
-
Thanks man , i'll try it in a sec
-
Also in my register.php page i just did what you said $currentpass = hash('ripemd128', $currentpass); $newpass = hash('ripemd128', $newpass); $cpass = hash('ripemd128', $cpass); but i have if(strlen($username) <3 || strlen($username) >30) { die("Your username must be 3 - 30 characters."); } else if(strlen($password) <3 || strlen($password) >30) { die("Your password must be 3 - 30 characters."); } And because the password is hashed it's bigger than 30
-
Sorry $cpass is confirming the new password
-
Not sure if this would work but $username = $_SESSION['username']; echo $username; Would that work in the chat page? In a table or something
-
Hi, i'm making a change password script which works fine, it changes the password but i want to check the old password before setting a new one and it keeps saying the old password is incorrect. Here is my script: if(isset($_POST['updatepass'])) { $currentpass = $_POST['oldpassword']; $newpass = $_POST['newpassword']; $cpass = $_POST['cpassword']; $currentpass = htmlspecialchars($currentpass, ENT_QUOTES); $currentpass = mysqli_real_escape_string($con, $currentpass); $currentpass = strip_tags($currentpass, ENT_QUOTES); $currentpass = filter_var($currentpass, FILTER_SANITIZE_STRING, FILTER_FLAG_ENCODE_HIGH); $currentpass = htmlentities($currentpass, ENT_QUOTES); $newpass = htmlspecialchars($newpass, ENT_QUOTES); $newpass = mysqli_real_escape_string($con, $newpass); $newpass = strip_tags($newpass, ENT_QUOTES); $newpass = filter_var($newpass, FILTER_SANITIZE_STRING, FILTER_FLAG_ENCODE_HIGH); $newpass = htmlentities($newpass, ENT_QUOTES); $cpass = htmlspecialchars($cpass, ENT_QUOTES); $cpass = mysqli_real_escape_string($con, $cpass); $cpass = strip_tags($cpass, ENT_QUOTES); $cpass = filter_var($cpass, FILTER_SANITIZE_STRING, FILTER_FLAG_ENCODE_HIGH); $cpass = htmlentities($cpass, ENT_QUOTES); $cpass = hash('ripemd128', $cpass); $currentpass = hash('ripemd128', $cpass); $oldpasswd = "SELECT password FROM users WHERE username='$username' AND password='$password'"; $opwd = mysqli_query($con, $oldpasswd); if($currentpass != $password) { die("Your old password is not correct."); } else { $query = "UPDATE users SET password='$cpass' WHERE username='$username'"; $UPDATE = mysqli_query($con, $query); if($UPDATE === TRUE) { echo "<div style='color: red; font-family: sans-serif; font-size: 18px;'>Your password has been updated!</div>"; } else { echo "Password could not be changed."; echo var_dump($UPDATE); } } } All help is very much appreciated
-
Hi, i am sending a query to my database, to check if my website is under maintainance, it's ment to display an access denied page if maintainance in the table is equal to 1 but it's not working properly. Here is the code: <?php error_reporting(E_ALL | E_NOTICE); require 'connect.php'; session_start(); $pageMaintain = "SELECT maintainance FROM config"; $result = mysqli_query($con, $pageMaintain); if(mysqli_num_rows($result) == 1) { include 'accessdenied.php'; die(); } else { } ?> And a picture of the table: It doesn't matter if it's 0 or 1 it still includes the access denied page.
-
Ah, Thanks Ch0cu3r and sorry gingerjm i wasn't quite sure what you meant.
-
$sql = "INSERT INTO `users` (username, password) VALUES ('$username', '$cpassword')";
-
Would it not be easier to use html and include the readonly attribute?