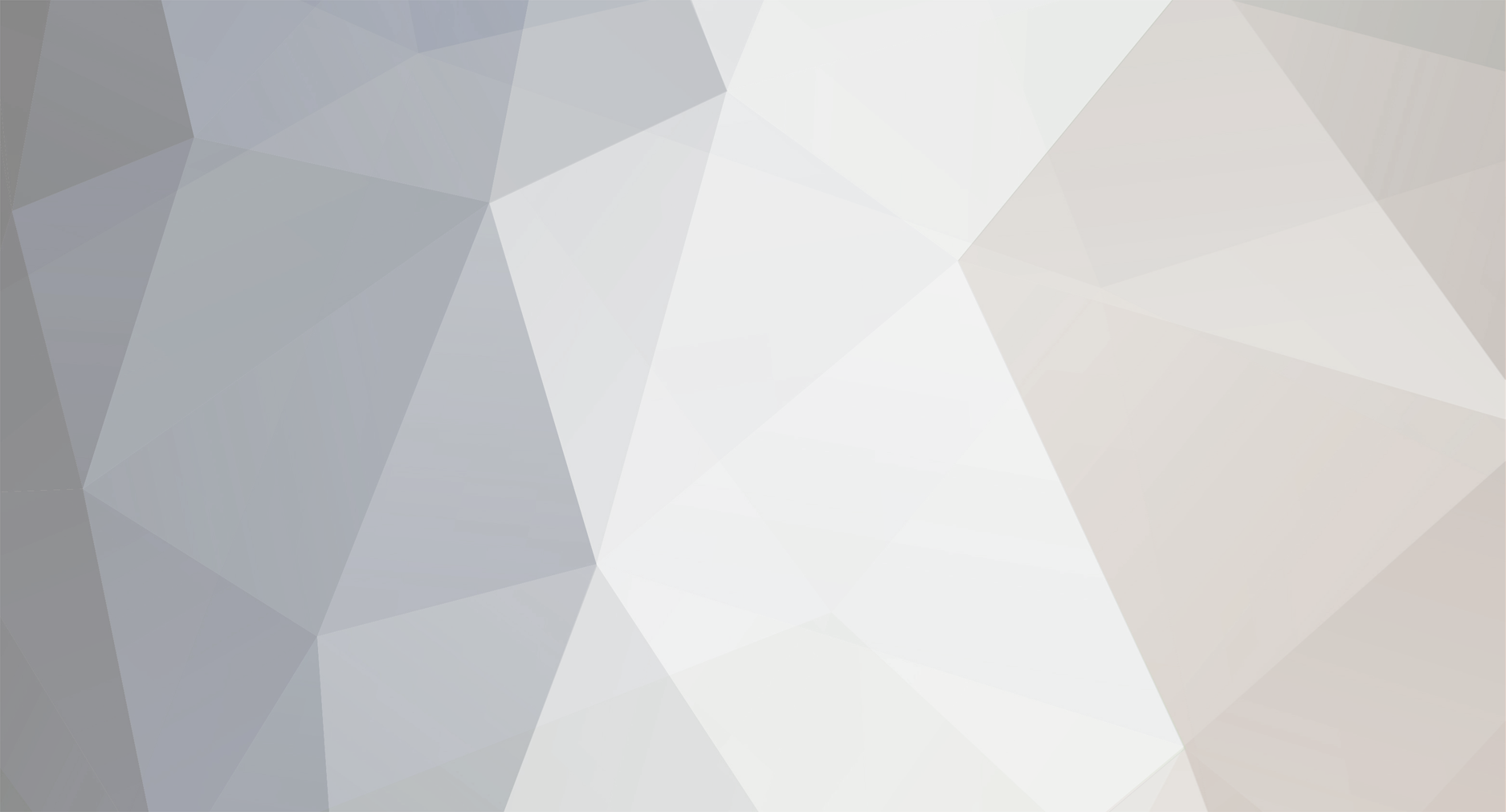
craygo
-
Posts
1,972 -
Joined
-
Last visited
-
Days Won
1
Posts posted by craygo
-
-
Sorry forgot you were using access and odbc
replace with this
$time = array(); $sql = "SELECT Date, Extension, Duration FROM AllInfo WHERE Extension = '$ex' AND Date=#$id# GROUP BY Date, Extension"; $result = odbc_exec($odbc, $sql) or die(odbc_error()); while($r = odbc_fetch_array($result)){ $time[] = $r['Duration']; }
Ray
-
LOL I don't ask how his data is setup, just answer the question. I know the potential problems with something like this, but I just help give the answer.
Ray
-
you would query the database first to get the number of clicks then just use a simple if statement.
<?php $disabled = $r['tablefield'] > 1 ? "disabled=\"disabled\"" : ""; echo "<input type=\"submi\"t name=\"submit\" value=\"submit\" $disabled />\n"; ?>
Ray
-
format is like this
$query = "SELECT player_id, type, price, username, crew, crewname FROM dom_london INNER JOIN user_info ON user_info.id = dom_london.player_id INNER JOIN crews ON user_info.crew = crews.id WHERE dom_london.id = '$section'";
Ray
-
look at your db class and make sure your variables are in the right locations.
$db = new Database('localhost','database name','password','database name');
It is probably a mistype but you have database name in there twice. want to make sure your values are in the right place
Ray
-
<?php $sql = "UPDATE `tablename` SET `Number` = `Number`+1"; mysql_query($sql) or die(mysql_error()); ?>
Ray
-
sanatize() is probably a custom function someone has made, and the @ in front of the POST variable will suppress generating an error if the variable is not set.
Ray
-
It can also be a script which seperates the url into parts then includes a certain part of the url as a page.
example
You start of in www.acme.com/index.php. on that page you have a link which goes to www.acme.com/about. At the top of index.php you have something like this
<?php $uri_split = explode("/", $_SERVER['REQUEST_URI']); $tmp = array(); foreach($uri_split as $key => $val) { if(!empty($val) || $val != "") { $tmp[count($tmp)] = $val; } } $uri_split = $tmp; if(!isset($uri_split[0])) { $uri_split[0] = "about_us"; }?>
Now you just include the page in index.php
include($uri_split[0]);
hope I got that right. Just grabs bits from one of my scirpt.
Ray
-
since you came out of php for your link you have to go back in to get the value
?><a href='menulist.php?resid=<?php echo $row->clientID; ?>'>View menu</a><?
Ray
-
What type of column is the Duration field. If it is just a text or varchar field, you will get the error. problem is the duration column can't be summed because it has those special characters in it. so a function will have to be made to add them up. try this out
<?php function get_seconds($t){ $seconds = 0; if(is_array($t)){ foreach($t as $v){ $array = array(); $pt1 = array(); $pt2 = array(); $array = explode(':', $v); $pt1[] = $array[0]; $pt2 = explode("'", $array[1]); $time = array_merge($pt1, $pt2); $sec = $time[2]; $min = $time[1]*60; $hour = $time[0]*60*60; $seconds += ($sec+$min+$hour); } } else { $array = explode(':', $t); $pt1[] = $array[0]; $pt2 = explode("'", $array[1]); $time = array_merge($pt1, $pt2); $sec = $time[2]; $min = $time[1]*60; $hour = $time[0]*60*60; $seconds += ($sec+$min+$hour); } return $seconds; } $time = array(); $sql = "SELECT Date, Extension, Duration FROM AllInfo WHERE Extension = '$ex' AND Date=#$id# GROUP BY Date, Extension"; $result = mysql_query($sql) or die(mysql_error()); while($r = mysql_fetch_assoc($result)){ $time[] = $r['Duration']; } $seconds = get_seconds($time); echo "<td class=\"borderTable\"><center>".date("H:i:s", mktime(0, 0, $seconds))."</center></td class=\"borderTable\"></table><p></p>"; ?>
Ray
-
my bad I forgot <h> tags don't start a new line.
<?php $s = array(33,34,35,36); $values = implode("', '", $s); $query="SELECT * FROM $tbl_name WHERE id IN ('$values')"; $result=mysql_query($query); while ($row = mysql_fetch_array($result)) { echo '<h5>', ($row['rem1']), '</h5><br />'; } mysql_free_result($result); ?>
Ray
-
-
There is no need to count rows to get the loop to end. The while statement will only run as long as rows are being returned. It will stop on it's own when the end is reached.
Also remember that field names and table names should be in backticks (`) and field values should be in single quotes (').
<?php $totalweight = 0; $query = "SELECT `weight` FROM `sites` WHERE `user` = '$user'"; $result = mysql_query($query) or die(mysql_error()); while ($sites = mysql_fetch_assoc($result)){ $totalweight += $sites['weight']; } $query = "UPDATE `user` SET `weight` = '$totalweight' WHERE `user` = '$user'"; $result = mysql_query($query) or die(mysql_error()); ?>
Ray
-
Thing with checkboxes is they are not passed if they are not checked. so you will have to put a check before you insert into the database
$SubscribeToNewsletter = isset($_POST['SubscribeToNewsletter']) ? "1" : "0";
Now if it is set $subscribeToNewletter will equal 1 if not is will be equal to 0;
Ray
-
First thing you should know is this.
you can get the minutes from using the date and mktime function
echo "<td class=\"borderTable\"><center>".date("g:i", mktime(0, $row["CountOfDuration"]))."</center></td class=\"borderTable\"></table><p></p>"; }
does this return just one row??
Duration contain seconds or minute??
DurationS holds ??
Do you want to show a running total or something like that??
Ray
-
try using 775 not 777. Some hosts won't allow 777.
-
Try using the absolute path for your directory locations.
Example
$path = '/var/www/html/panel/modules/mysql/'.$row_User['homedir'].'/_Backups';
Also when working with directories leave out the last slash
Ray
-
Probably your best bet. Not sure how often the API information changes. You could set up a script to run from a cron job to update the database once a day or something. Then querying the database would take milliseconds.
Ray
-
the query is very simple and should not take any time at all, I would think it is the API's that are taking time to retrieve the information.
-
try this
<?php function get_seconds($time){ $array = explode(':', $time); $pt1[] = $array[0]; $pt2 = explode("'", $array[1]); $time = array_merge($pt1, $pt2); $sec = $time[2]; $min = $time[1]*60; $hour = $time[0]*60*60; $seconds = $sec+$min+$hour; return $seconds; } $time = "2:20'13"; echo get_seconds($time); ?>
Ray
-
Try this out
<?php $query = "SELECT `impressions`, `url` FROM `sites`"; $result = mysql_query($query) or die(mysql_error()); while ($sites = mysql_fetch_assoc($result)){ $url = $sites['url']; $impessions = $sites['impressions']; $weight = ceil($dmozbonus*$indexed*($impressions*$siteprbonus*5996)/(750000 - $impressions)); $update = "UPDATE `sites` SET `weight` = '$weight' WHERE `url` = '$url'"; mysql_query($update) or die(mysql_error()); } ?>
Ray
-
I edited my post above but to help out more you may want to show your table data and let us know what you want to accomplish. Or should I say what your final result should be.
Ray
-
Where is the weight value coming from??
Also where is the url value coming from?? It looks like you are comparing the url to each field in each row that is returned.
Ray
-
You seem to be complicating things here.
Are you trying to update the weight field for all your rows??
[SOLVED] Quick Question
in PHP Coding Help
Posted
Don't forget to mark topic as SOLVED
Ray