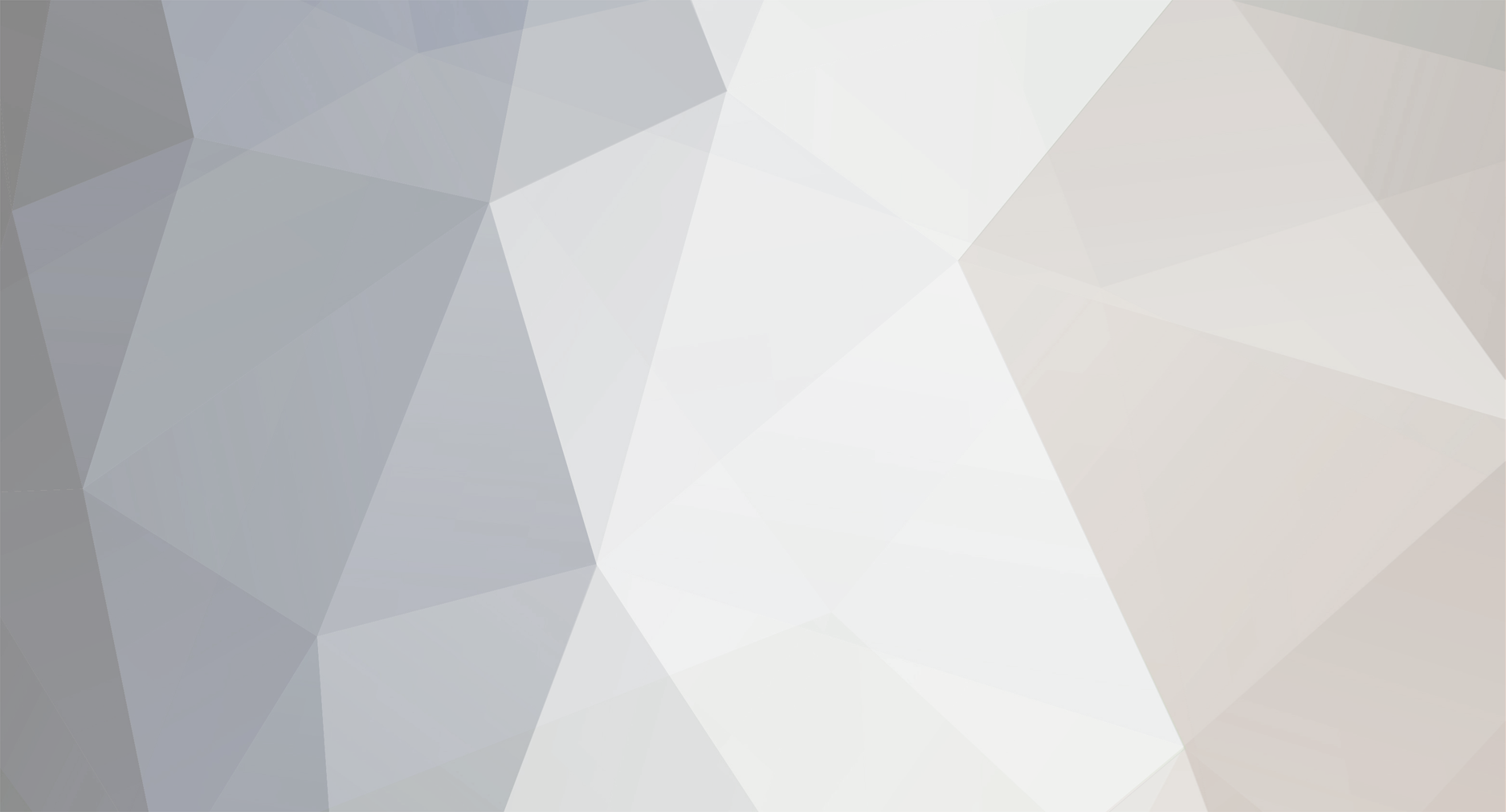
craygo
-
Posts
1,972 -
Joined
-
Last visited
-
Days Won
1
Posts posted by craygo
-
-
Change the login.php to this
<?PHP session_start(); //check that the user is calling the page from the login form and not accessing it directly //and redirect back to the login form if necessary if (!isset($_POST['username']) || !isset($_POST['password'])) { header( "Location: http://localhost/test2/login.htm" ); } //check that the form fields are not empty, and redirect back to the login page if they are elseif (empty($_POST['username']) || empty($_POST['password'])) { header( "Location: http://localhost/test2/login.htm" ); } else{ //convert the field values to simple variables //add slashes to the username and md5() the password $user = addslashes($_POST['username']); $pass = md5($_POST['password']); //set the database connection variables $dbHost = "localhost"; $dbUser = "root"; $dbPass = ""; $dbDatabase = "randv"; //connet to the database $db = mysql_connect("$dbHost", "$dbUser", "$dbPass") or die ("Error connecting to database."); mysql_select_db("$dbDatabase", $db) or die ("Couldn't select the database."); $result=mysql_query("select * from users where username='$user' AND password='$pass'", $db); //check that at least one row was returned $rowCheck = mysql_num_rows($result); if($rowCheck > 0){ while($row = mysql_fetch_array($result)){ //egister a variable $_SESSION['username'] = $row['username']; $_SESSION['logged_in'] = 1; //successful login code will go here... echo 'Success!'; //we will redirect the user to another page where we will make sure they're logged in header( "Location: checkLogin.php" ); } } else { //if nothing is returned by the query, unsuccessful login code goes here... echo 'Incorrect login name or password. Please try again.'; } } ?>
Ray
-
ok couple things
you need to add this
//$query="SELECT * FROM contacts, booking_form WHERE contacts_id = $record AND bf_id = $bf_record"; $query="SELECT * FROM contacts WHERE contacts_id = $record"; $result=mysql_query($query); if(!$result) { print("Query Error: ".mysql_error()); } $row = mysql_fetch_assoc($result); // add this line
Also need to change the variable for the issue loop
while($irow=mysql_fetch_assoc($issues_result)) { // change this to $irow and the variables below $selected = in_array($irow['issue_number'], $bf_issues_booked) ? "checked=\"checked\"" : ""; $issues[] = '<input name="issue_number[]" type="checkbox" value="' .$irow['issue_number']. '" tabindex="11" ' . $selected . ' />Issue <strong>' .$irow['issue_number'] . '</strong> ' . $irow['issue_month'] . ' ' . $irow['issue_year'] ; }
That is what I found so far, let me know
Ray
-
look like it will run youjust have so syntax errors
//Run the count information $count = 10; for($i = 1; $i <= $count; $i++) { $cost = number_format($_REQUEST["cost_".$i], 2, ".", ""); $size = $_REQUEST['size_'."$i"]; if ($cost > 0 && $size > 0){ mysql_query("UPDATE `sizes` SET `size` = '$size', `cost` = '$cost' WHERE `refnum` = '$sizeref'"); } } mysql_close($con);
Also how do you know the loop needs to run 10 times?? The only thing is you will be updating the same record on every loop. Why would you do this??
You may want to pass the count value from the form to the update script.
Ray
-
where is the list of magazine issues being held??
-
well you would have to post your entire code, because the process actually takes 2 queries to do what you want
-
you can do something like
if(strtolower("value") == strtolower("VALUE")){ echo "the same value"; }
Ray
-
change the action in the form tag
<form method="post" action="somepage.php">
What was the problem??
Ray
-
you are closing your loop before you echo anything out, so when you do echo anything it will be the last row in the table
<?php $links = @mysql_query("SELECT * FROM ".$mysql_pretext."_documents WHERE document_type_id = '2'"); if (!$links) { echo("Error retrieving student life documents from the database!<br>Error: " . mysql_error()); exit(); } $number = 0; ?> <table> <tr> <td height="30" colspan="2" bgcolor="#A0C4EC" class="search"><span class="style1">Student Life Library</span></td> </tr> <?php while ($link = mysql_fetch_array($links)) { $ids1 = $link["ID"]; $titles1 = $link["title"]; $type = $link["type"]; $date = $link["date"]; $filename = $link["name"]; $addedby = $link["added_by"]; $number++; ?> <tr> <td width="6%" height="30" valign="middle"><img src="images/pdf_icon.png" width="20" height="20" /></td> <td width="94%" height="30" valign="middle" class="text"><a href="getdocument.php?id=<?php echo($ids1); ?>"><?php echo($filename); ?></a> - <?php echo($titles1); ?></td> </tr> <?php } ?> </table>
Ray
-
Not sure what else it can be. I have this
<?php import_request_variables("pg", "form_"); $hostname = "localhost"; $db_user = "root"; $db_password = "*******"; $db_table = "customer"; //$db = mysql_connect($hostname, $db_user, $db_password); //mysql_select_db("rdms",$db); ?> <html> <head> <title>Adding New Contact in Record</title> </head> <link rel="stylesheet" type="text/css" media="screen" href="abc.css" /> <body> <fieldset> <legend> New Customer Entry </legend> <img src="header.jpg" align="top"> <h2>Adding New Contact in Record</h2><?php if (isset($_REQUEST['Submit'])) { $form_cust_country = $_POST['customer_country']; $sql = "INSERT INTO $db_table(customer_id, customer_name, customer_add, customer_add1, customer_phone, customer_mobile, customer_fax, customer_city, customer_county, customer_postcode, customer_country, customer_email, customer_position, customer_website, customer_comment) values ('$form_cust_id', '$form_cust_name','$form_cust_add','$form_cust_add 1','$form_cust_phone', '$form_cust_mobile','$form_cust_fax','$form_cust_city','$form_cust_county','$form_cust_postcode', '$form_cust_country','$form_cust_email','$form_cust_position','$form_cust_website','$form_cust_comment')"; echo $sql."<br />"; if($result = mysql_query($sql ,$db)) { echo "Thank you, Your information has been entered into our database"; } else { echo "ERROR: ".mysql_error(); } } else { ?> <form method="post" action=""> <table width="470"> <tbody> <tr> <td>ID:</td> <td><input type="text" name="cust_id" class="abc"></td> </tr> <tr> <td> Name:</td> <td><input type="text" name="cust_name" class="abc"></td> </tr> <tr> <td>Address:</td> <td><input type="text" name="cust_add" class="abc"></td> </tr> <tr> <td>Line 2:</td> <td><input type="text" name="cust_add1" class="abc"></td> </tr> <tr> <td>Phone:</td> <td><input type="text" name="cust_phone" class="abc"></td> </tr> <tr> <td>Mobile:</td> <td><input type="text" name="cust_mobile" class="abc"></td> </tr> <tr> <td>Fax: </td> <td><input type="text" name="cust_fax" class="abc"></td> </tr> <tr> <td>City:</td> <td><input type="text" name="cust_city" class="abc"></td> </tr> <tr> <td>County:</td> <td><input type="text" name="cust_county" class="abc"></td> </tr> <tr> <td>Post code:</td> <td><input type="text" name="cust_postcode" class="abc"></td> </tr> </tbody></table> <table width="450"> <tbody> <tr> <td>Country:</td> <select name="customer_country" class="abc"> <option value="" selected="selected">Select a Country</option> <option value="United States">United States</option> <option value="United Kingdom">United Kingdom</option> </select> </tr> <tr> <td>Email:</td> <td><input type="text" name="cust_email" class="abc"></td> </tr> <tr> <td>Position: </td> <td><input type="text" name="cust_position" class="abc"></td> </tr> <tr> <td>Website:</td> <td><input type="text" name="cust_website" class="abc"></td> </tr> <tr> <td>Comments:</td> <td><input type="text" name="cust_comment" class="abc"></td> </tr> </tbody></table> </fieldset> <input type="submit" name="Submit" value="Submit"> </form> <?php } ?> </body> </html>
and when I echo the sql I get this
INSERT INTO customer(customer_id, customer_name, customer_add, customer_add1, customer_phone, customer_mobile, customer_fax, customer_city, customer_county, customer_postcode, customer_country, customer_email, customer_position, customer_website, customer_comment) values ('1', 'Hello','222 nnjjh ave','222 nnjjh ave 1','4015554444', '5555555555','1544455654','somecity','some county','025558', 'United States','sjklkgh@jklghlkjh.com','position','www.domain.com','jklglkg')
Ray
-
try and echo out the query and see what you get
$sql = "INSERT INTO $db_table(customer_id, customer_name, customer_add, customer_add1, customer_phone, customer_mobile, customer_fax, customer_city, customer_county, customer_postcode, customer_country, customer_email, customer_position, customer_website, customer_comment) values ('$form_cust_id', '$form_cust_name','$form_cust_add','$form_cust_add 1','$form_cust_phone', '$form_cust_mobile','$form_cust_fax','$form_cust_city','$form_cust_county','$form_cust_postcode', '$form_cust_country','$form_cust_email','$form_cust_position','$form_cust_website', '$form_cust_comment')"; echo $sql."<br>"; $result = mysql_query($sql ,$db); if($result) { echo "Thank you, Your information has been entered into our database"; } else { echo "ERROR: ".mysql_error(); }
Ray
-
post code for config.php
-
$time = time(); //now in seconds $formatted_date = date("Y-m-d H:i:s", $time); echo $formatted_date;
Ray
-
Hey don't get technical with me
I just hope giraffemedia gets the point
Ray
-
what is the structure of the table??
-
Thanks I am so use to using double quotes I forget sometimes. good catch
-
The only thing that would cause that is the database table. What type is the field and how long is it?
do you get any error??
try this to check
$result = mysql_query($sql ,$db); if($result) { echo "Thank you, Your information has been entered into our database"; } else { echo "ERROR: ".mysql_error(); }
-
What you need to do is grab the comma seperated values then create an array from them I use a function for this to get the data. Loop through all your issues then check to see if the id is in the array that you created. use the in_array() command to see if is is in the array.
// Define the array variable $bf_issues_booked = explode(",",$row['bf_issue_number']); // just to see if there is any result echo $bf_issues_booked; // A loop used to populate my form with the checkboxes and issue name, number etc. This works fine while($row=mysql_fetch_assoc($issues_result)) { $selected = in_array($row['issue_number'], $bf_issues_booked) ? "selected" : ""; $issues[] = '<input name="issue_number[]" type="checkbox" value="' .$row['issue_number']. '" tabindex="11" $selected />Issue <strong>' .$row['issue_number'] . '</strong> ' . $row['issue_month'] . ' ' . $row['issue_year'] ; }
Ray
-
you have to give it a name
echo "<a href=\"pest.php?id=".$row['id']."\" />".$row['Name']."</a>\n";
Then on the processing page
$id = $_GET['id'];
Then run your query
Ray
-
your values are messed up. there are spaced in your values and you forgot a comma.
$sql = "INSERT INTO $db_table(customer_id, customer_name, customer_add, customer_add1, customer_phone, customer_mobile,
customer_fax, customer_city, customer_county, customer_postcode, customer_country, customer_email,
customer_position, customer_website, customer_comment)
values ('$form_cust_id', '$form_cust_name','$form_cust_add','$form_cust_add 1','$form_cust_phone',
'$form_cust_mobile','$form_cust_fax','$form_cust_c ity','$form_cust_county','$form_cust_postcode',
'$form_cust_country','$form_cust_email','$form_cus t_position','$form_cust_website,''$form_cust_comme nt')";
Ray
-
session_register is may not work, it requires register_globals to be on and they are off by default
http://us.php.net/manual/en/function.session-register.php
what you want to so is use $_SESSION instead
<?PHP //check that the user is calling the page from the login form and not accessing it directly //and redirect back to the login form if necessary if (!isset($username) || !isset($password)) { header( "Location: http://localhost/test2/login.htm" ); } //check that the form fields are not empty, and redirect back to the login page if they are elseif (empty($username) || empty($password)) { header( "Location: http://localhost/test2/login.htm" ); } else{ //convert the field values to simple variables //add slashes to the username and md5() the password $user = addslashes($_POST['username']); $pass = md5($_POST['password']); //set the database connection variables $dbHost = "localhost"; $dbUser = "root"; $dbPass = ""; $dbDatabase = "randv"; //connet to the database $db = mysql_connect("$dbHost", "$dbUser", "$dbPass") or die ("Error connecting to database."); mysql_select_db("$dbDatabase", $db) or die ("Couldn't select the database."); $result=mysql_query("select * from users where username='$user' AND password='$pass'", $db); //check that at least one row was returned $rowCheck = mysql_num_rows($result); if($rowCheck > 0){ while($row = mysql_fetch_array($result)){ //start the session and register a variable session_start(); $_SESSION['username'] = $row['username']; $_SESSION['logged_in'] = 1; //successful login code will go here... echo 'Success!'; //we will redirect the user to another page where we will make sure they're logged in header( "Location: checkLogin.php" ); } } else { //if nothing is returned by the query, unsuccessful login code goes here... echo 'Incorrect login name or password. Please try again.'; } } ?>
and
<?php //start the session session_start(); //check to make sure the session variable is registered if(isset($_SESSION['username']) && $_SESSION['logged_in'] == 1){ //the session variable is registered, the user is allowed to see anything that follows echo 'Welcome, you are still logged in.'; } else{ //the session variable isn't registered, send them back to the login page header( "Location: http://localhost/test2/login.htm" ); } ?>
Also look here for how to properly destroy a session
http://us.php.net/manual/en/function.session-destroy.php
Ray
-
First fix your query and get back to us, cause once I fixed it everything is fine
$sql = "INSERT INTO $db_table(customer_id, customer_name, customer_add, customer_add1, customer_phone, customer_mobile, customer_fax, customer_city, customer_county, customer_postcode, customer_country, customer_email, customer_position, customer_website, customer_comment) values ('$form_cust_id', '$form_cust_name','$form_cust_add','$form_cust_add 1','$form_cust_phone', '$form_cust_mobile','$form_cust_fax','$form_cust_city','$form_cust_county','$form_cust_postcode', '$form_cust_country','$form_cust_email','$form_cust_position','$form_cust_website', '$form_cust_comment')";
Ray
-
sorry my bad
-
where are you setting $count??
-
What OS, Webserver, php version mysql version you using??
[SOLVED] Please help - i'm stumped
in PHP Coding Help
Posted
if you give me a dump of your 3 tables I can help you much better. just an sql dump from phpmyadmin