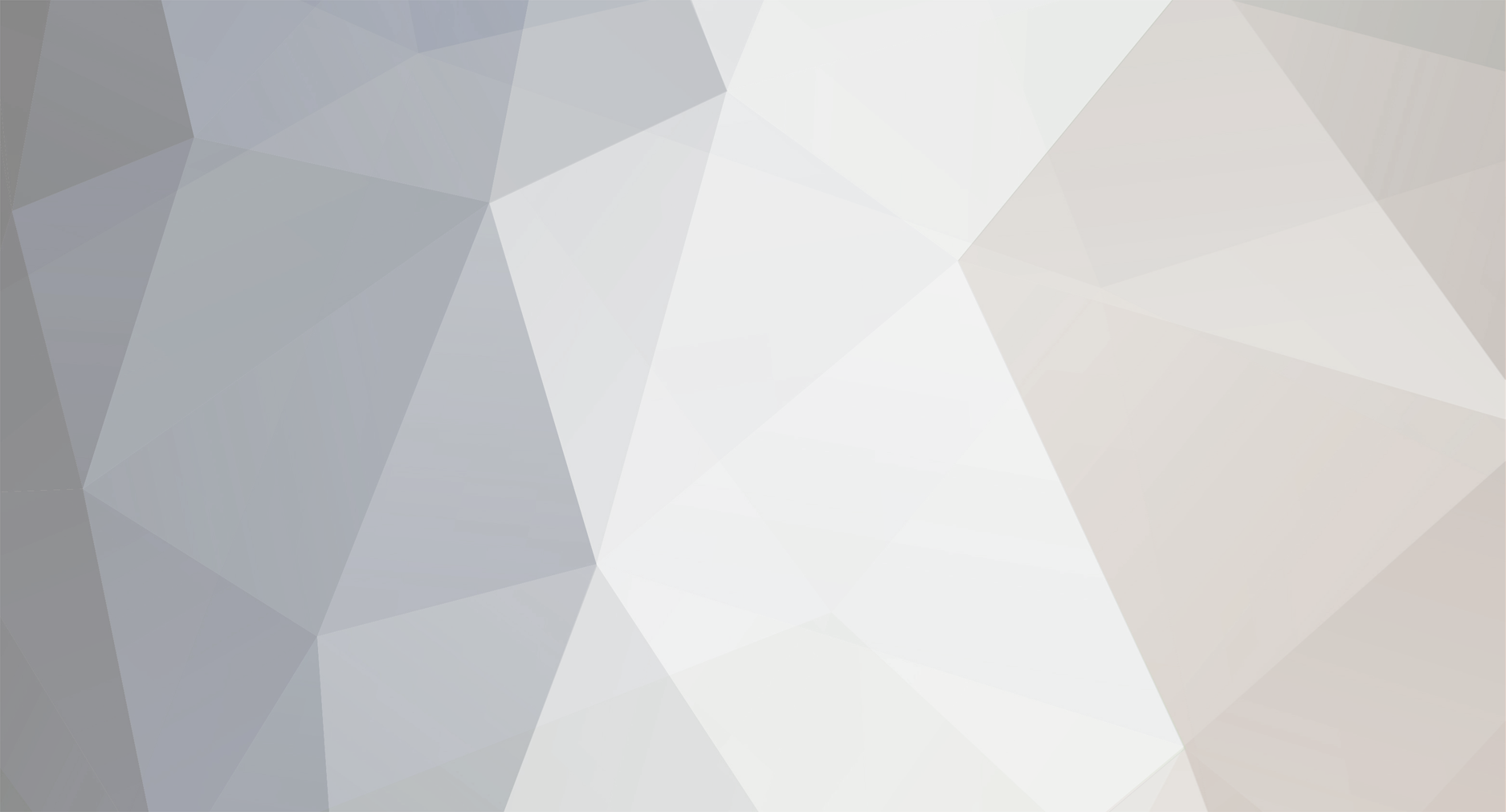
jeremywesselman
-
Posts
154 -
Joined
-
Last visited
Never
Posts posted by jeremywesselman
-
-
They blend right in with the page like they're not even there!
-
Pseudocode yes. But I wouldn't sit there and write down the whole program. Unless it was a simple script and I wasn't near a computer. But even then I don't think I'd write the whole thing out.
And like you mentioned, double the work.
Jeremy
-
Thank you moderators / administrators.
-
I read through all the posts and didn't see e so I'll throw it out there.
[url=http://www.e-texteditor.com/]http://www.e-texteditor.com/[/url]
It's very similar to Textmate, so if you use Windows you have an alternative. It will edit pretty much anything. I use it for Rails development mostly.
Jeremy
-
Of course it is better for the advertiser if the ad is at the top of the page. It is almost guaranteed to be seen even if the person viewing the page glances at it and hits the back button.
-
It happens everytime I start to help. How do you think I got over 3000 posts.
Wasn't it through asking about 100 questions per day? Might not have been that many but it seemed like it.
I was thinking the same thing...
-
Put another minus (-) sign next to the one that's already there.
<?php for ($i = 10; $i > 0; $i--){ print "$i <br>\n"; } // end for loop ?>
Jeremy
-
id int NOT NULL AUTO_INCREMENT PRIMARY KEY user_id int NOT NULL image_name varchar(50) NOT NULL caption varchar(100)
Ok. You'll have the normal 'id' field. The 'user_id' field is the id of the user to which the image belongs.
Then the 'image_name' field. It depends on how you set up your directory. Some people throw them all in one directory and some others set up each member with their own folder under the 'images' directory to keep them kind of organized. However you set it up, when you are linking to the image in HTML, you'll be able to insert the path there and just pull the image name from the table. And if you want to give the user their own folder, you'll be able to throw their username in the path there as well.
Then there is the optional 'caption' field for holding a little description of the pic.
You'll need a separate table for the ratings.
id int NOT NULL AUTO_INCREMENT PRIMARY KEY image_id int NOT NULL rating int NOT NULL
The id field will keep track of the ratings. The image_id field is the id of the image that has been rated. And the rating of course will be the rating, however you set them up.
There might be better ways to do it, but this is what I came up with in a few minutes.
Jeremy
-
That is probably it. Why don't you add them before hand and output as one var and see what happens.
-
<?php session_start(); require("config.php"); require("functions.php"); ?> <table border="0"> <tr> <td>Welcome, <?php echo $_SESSION['s_name']; ?></td> </tr> <tr> <td>You are logged in as <?php echo $_SESSION['s_username']; ?></td> </tr> <tr> <td> </td> </tr> <tr> <td><a href="members.php">Members</a></td> </tr> <tr> <td><a href="inbox.php">Inbox</a> <?php $message = mysql_query("SELECT COUNT(*) FROM myPMs WHERE opened != 'y' AND to_id = '{$_SESSION['s_id']}'") or die ("My SQL Error: <br />". mysql_error()); $msgs = mysql_fetch_array($message); if ($msgs['COUNT(*)'] > 0) { // News Msgs Found echo "<img src=\"images/envelope.gif\" />"; } ?> </td> </tr> <tr> <td><a href="edit_profile.php">My Profile</a></td> </tr> <tr> <td><a href="logout.php">Logout</a></td> </tr> </table>
You're hopping in and out of php and html. Here is your script fixed like it should be. If you are going to use php to echo stuff, you have to echo ALL the HTML, not just the link.
Jeremy
-
What kind of error message do you get?
-
I'd say you could either scan your drawings onto the computer, or get a https://www.digitalgraphicsresources.com/index.asp?PageAction=VIEWCATS&Category=97&gclid=CJmh58eG7IoCFRtAgQodhT-okQ.
(just an example, no affiliation)
Jeremy
-
You need to declare the $messageAry global inside your function to access it outside of your function.
function showImg($filename) { global $messageAry; if($filename != "") { $jpg = $filename; $messageAry['title'] = "woohoo, we have an image"; } else { $jpg = "default.jpg"; $messageAry['title'] = "no image found"; } return $jpg; } // end function
Jeremy
-
$pageviewquery = mysql_query("SELECT * FROM pageviews WHERE pageuserid='45'"); $result = mysql_fetch_array($pageviewquery); echo $result; if ($result == FALSE) { echo "query is false"; } else { echo "query is not false"; }
You need to use mysql_fetch_array() to get the results. Otherwise, it is returning a Resource Id.
Jeremy
-
lol. I can't believe I didn't see it before...
if ($msg['COUNT(*)'] > 0) {You've got a spelling error. $msg should be $msgs...
Jeremy
-
Have you checked to make sure that your query is returning a result?
<?php print_r($msgs); ?>
(might be a dumb question, but let's just be sure here)
-
After running some tests of my own, I have determined that it returns false if there are no rows in the database.
-
<?php $week = date("W"); if ($week<"14") { echo '[<a href="#" onclick="window.open(\'year3/1_10.php\', \'mywindow\', \'width=500,height=350,resizable=no\');"><font color=#808000>First Quarter</font></a>]'; } else { echo '<a href="#" onclick="window.open(\'year3/1_10.php\', \'mywindow\', \'width=500,height=350,resizable=no\');"><font color=#808000>First Quarter</font></a>' ; } ?>
That is one way you can do it.
Another:
<?php $week = date("W"); if ($week<"14"): ?> [<a href="#" onclick="window.open('year3/1_10.php', 'mywindow', 'width=500,height=350,resizable=no');"><font color=#808000>First Quarter</font></a>] <?php else: ?> <a href="#" onclick="window.open('year3/1_10.php', 'mywindow', 'width=500,height=350,resizable=no');"><font color=#808000>First Quarter</font></a> <?php endif; ?>
-
Sounds like all you need to do is change the column name in your query:
$this->dbu->query("select email from ban_emails where email='".$ld['email']."'");
Jeremy
-
[quote]http://www.essentialpim.com/?r=products&pr=screenshots[/quote]
I hate to use the 'M' word, but this just looks like a rip of Microsoft Outlook to me. It is set up exactly like it. Why don't you try Outlook? -
You could use mod_rewrite for Apache. You can take your URL, http://kilbad.com/index.php?id=photos&album=general, and turn it into something like: http://kilbad.com/photos/general/
Here is a link to get you started with the basics.
http://www.yourhtmlsource.com/sitemanagement/urlrewriting.html
There are other resources on the right column of the page to get you further if needed.
Jeremy -
[code]if (isset($HTTP_GET_VARS['delete'])){
$sql = "DELETE FROM web_announcements WHERE id=$id";
$result = mysql_query($sql);
/*********************/
if($result)
{
echo"The announcement has successfully been removed.<br><br>- <a href=./acp.php>Back</a>";
}
/********************/
}
if (isset($HTTP_GET_VARS['announce2'])){
echo"You are now ready to either remove or edit an announcement, please select your options below.<hr color=#990000>";
$query = "SELECT * FROM web_announcements";
$result = mysql_query($query);
while ($results = mysql_fetch_array($result)){
extract($results);
echo"- $title (<a href=>EDIT</a>)/(<a href=$PHP_SELF?delete&id=$id>DELETE</a>)<br>";
}
}[/code]
You need to add another if statement to check if $result returns true or false. Then you can display the success message.
[color=maroon]Jeremy[/color] -
Have you looked at the tutorial here at phpfreaks?
[url=http://www.phpfreaks.com/tutorials/85/0.php]http://www.phpfreaks.com/tutorials/85/0.php[/url]
It is tutorial on a file upload class with file extension validation, getting sizes and many other things.
[color=maroon]Jeremy[/color] -
That is what the '*' does. If you only wanted to select 'id' and 'name', your query would look like this:
[CODE]SELECT id, name FROM table WHERE status = 1[/CODE]
So if you want to pull all columns, use *. The 'WHERE' clause lets you specify certain information about your results.
php print_r
in PHP Coding Help
Posted
You need to drill down through the multi-dimensional array.
Just use the array keys to get their values. I'll let you get the avg because I can't really tell the path to it.
EDIT: I tried to review, but pressed the button too quickly
Jeremy