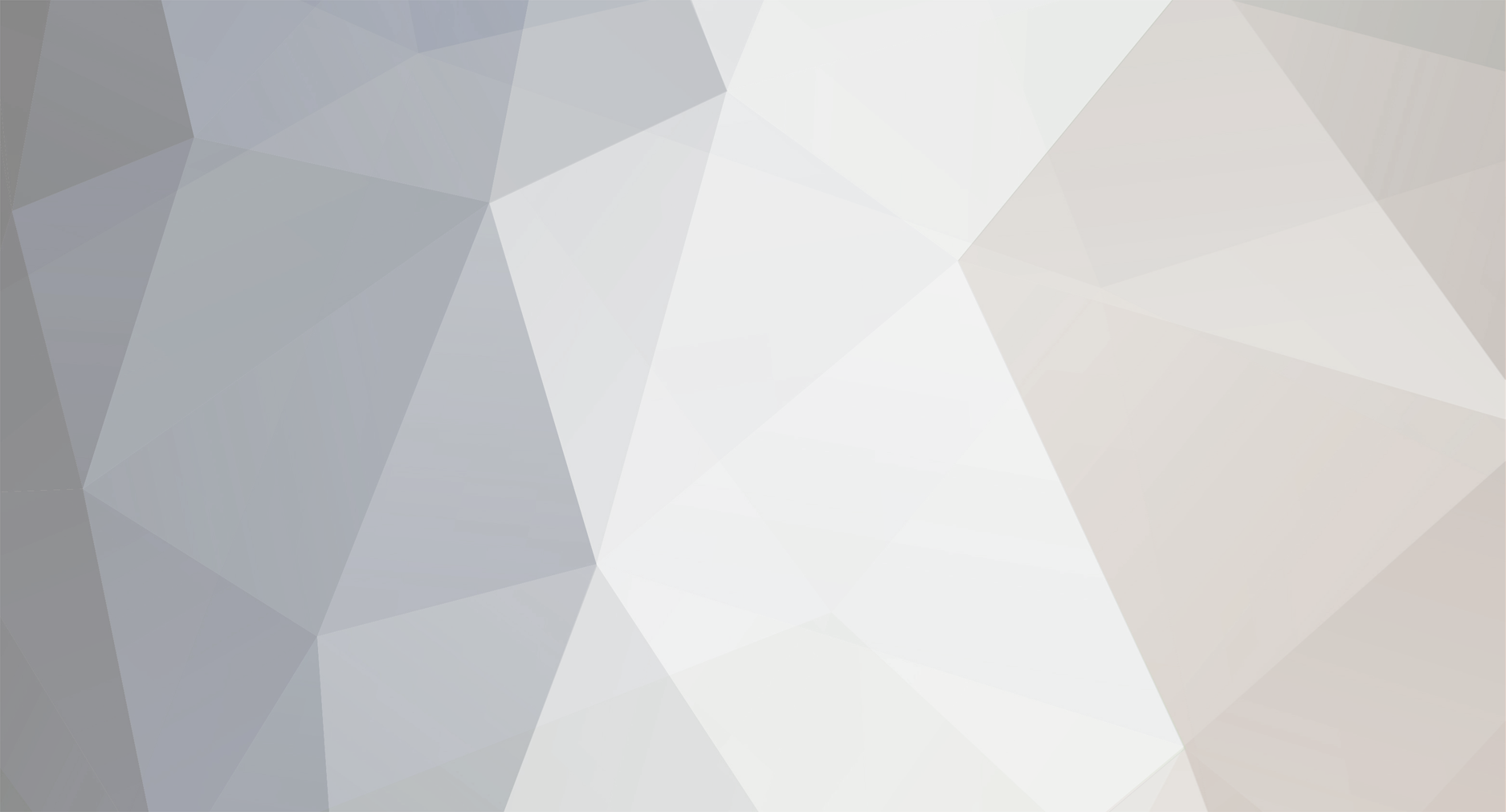
jeremywesselman
-
Posts
154 -
Joined
-
Last visited
Never
Posts posted by jeremywesselman
-
-
I was going to suggest CakePHP also. It's a rapid development framework based on the concept of Ruby on Rails. There is also PHP on Trax, but I haven't really messed around with it. There are a lot of other frameworks around too.
Personally, I use a framework that consists of smarty, pear and a good directory structure.
Jeremy -
Try this:
[code]<?php
echo $row['name'] . "<br><table border='0' width='100%' id='table1'><tr>";
?>[/code]
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
[code]<?php
echo "$row['name']
<br>
<table border='0' width='100%' id='table1'>
<tr>";
?>[/code]
If you use double quotes to echo with, you DO NOT need to concatenate your variable into your string.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
Ok. Single quotes and double quotes have a slight difference in PHP. There is more than one way to code your example.
Code 1:
[code]<?php
echo "<a href=\"$row['link']\">$row['thumb']</a>";
?>[/code]
Code 2:
[code]<?php
echo '<a href="' . $row['link'] . '">' . $row['thumb'] . '</a>';
?>[/code]
When you use double quotes while echoing a string, you don't need to concatenate the string with your variables. You can just echo them within the string. But with this technique, you do need to escape other double quotes in the string with a backslash(\).
If you use single quotes while echoing a string, you have to concatenate(.) the string together with the variables, as in Code 2.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
You have your quotes messed up.
[code]<?php
echo '<a href="' . $row['link'] . '">' . $row['thumb'] . '</a>';
?>[/code]
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
It is because you are trying to put HTML code inside PHP tags without using print or echo to print the HTML code.
It looks like you need to close the PHP tag right before the output of the HTML and get rid of the closing tag at the bottom.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
You will need to use the 'LIMIT' keyword in MySQL.
[!--sql--][div class=\'sqltop\']SQL[/div][div class=\'sqlmain\'][!--sql1--][span style=\'color:blue;font-weight:bold\']SELECT[/span] Sender, Subject , Date [color=green]FROM[/color] [color=orange]inbox[/color] [color=green]LIMIT[/color] [color=orange]0, 20[/color] [!--sql2--][/div][!--sql3--]
This query will return from row 0 to row 20. The 0 represents the starting row, and the 20 represents the quantity of rows to be returned. To show records 21 - 40, your query would look something like this:
[!--sql--][div class=\'sqltop\']SQL[/div][div class=\'sqlmain\'][!--sql1--][span style=\'color:blue;font-weight:bold\']SELECT[/span] Sender, Subject , Date [color=green]FROM[/color] [color=orange]inbox[/color] [color=green]LIMIT[/color] [color=orange]21, 20[/color] [!--sql2--][/div][!--sql3--]
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
You have posted this in the wrong section. This has nothing to do with PHP! Mods, please move to the Javascript Forum.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
You will need to put whatever you want to display inside the echo() function.
[code]<?if ($friend[online]) echo('<img src "images/star.gif" length="16" width ="16">');?>[/code]
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
I think that if you are on a deadline and come across a script that does what you are looking to do, then use it. But I think it makes a programmer feel good to say that they coded the script on their own. I know it makes me feel a lot better about being me :)
And it also looks nice on your resume. To say you coded the whole thing and all the features.
But that's just me...
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
You have the right idea. Right after $address1, you will need an if statement to determine whether or not to display $address2.
[code]<?php
...
echo($address1);
if($address2 != "")
{
echo(', ' . $address2);
}
...
?>[/code]
Or something similar to that.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
CakePHP is as fast as I need it to be. I haven't really put a large load on it yet. I have just been messing around with it a little. It is very fast to develop with. They built in it on the Ruby on Rails concept, except using PHP. It is very easy to get an application up and running quickly.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
I have used PEAR DB on a couple of projects. It's alright. I use it mostly when separating content from logic. But lately I have been messing around with CakePHP. I think it is really neat how everything works together like it does.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
Start coding!!!
You've read about it, now do it. The best way to learn is to do. Do a little bit, check it in browser, do a little more, check again. Until you make something that looks like your template. I'm not going to lie to you. This will probably take you a while. You will be refering to many XHTML and CSS sites along the way.
At this time, if you haven't even coded a single example, you need to google some XHTML and CSS tutorials and follow them.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
Then why don't you just pass it into the constructor when you instantiate it.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
How do you expect to call $MyNewClassObject->getVarName(); when you are trying to find out what $MyNewClassObject is? Think through your question before you ask.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
You can start at [a href=\"http://www.w3schools.com/\" target=\"_blank\"]http://www.w3schools.com/[/a]. You need to learn XHTML for laying out the site and CSS for controling the layout and styling of the site.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
If you want something that is descriptive of the company, you could go with:
- www.ibc.com
- www.ibusinessconsultants.com
- www.ibconsultants.com
But you have probably already gone through these and rejected them :)
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
Why did you make it when you don't know what to do with it? It sounds like you need to do a lot of reading.
You need to slice and export it in photoshop. Then you need to use XHTML and CSS to recreate the psd. You really need to read up on XHTML and CSS to learn how to do it properly. You will learn nothing throwing it into frontpage.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
I'm sure you can just put it in the root directory and use it in all applications, but I haven't tried that. But with the way that I have mine set up, I put a copy in each project folder, because I bury the template_c and configs folder in the library itself because you never really need to use anything in these folders.
Give it a shot. And if it don't work, you'll have to put a separate library in each app folder.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
I think it returs a string every time because it is in quotes. I did a few tests and this:
[code]<?php
$text = 23;
?>[/code]
returns integer. But if I put it in quotes, it returns a string.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
You can do that by not setting the category_id variable or by setting it to 'all'. Then you will need to do a check on it to determine which sql statement you will need to run.
[code]product.php?category_id=all[/code]
Then you would check it like this.
[code]<?php
if(isset($_GET['category_id']))
{
$category_id = $_GET['category_id'];
if($category_id == 'all')
{
$sql = "SELECT * FROM products";
}
else
{
$sql = "SELECT * FROM products WHERE category_id = $category_id";
}
}
?>[/code]
And then you can run your query with $sql.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
You need to go through your code and delete all the \ before the ". That is why it is not working correctly. Unless the " is inside a set of "", you need to remove the \.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
It is because you are escaping all of quotation marks. Unless it is inside a string that is being printed, they do not need to be escaped.
[!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
PHP/MySQL Issue
in PHP Coding Help
Posted