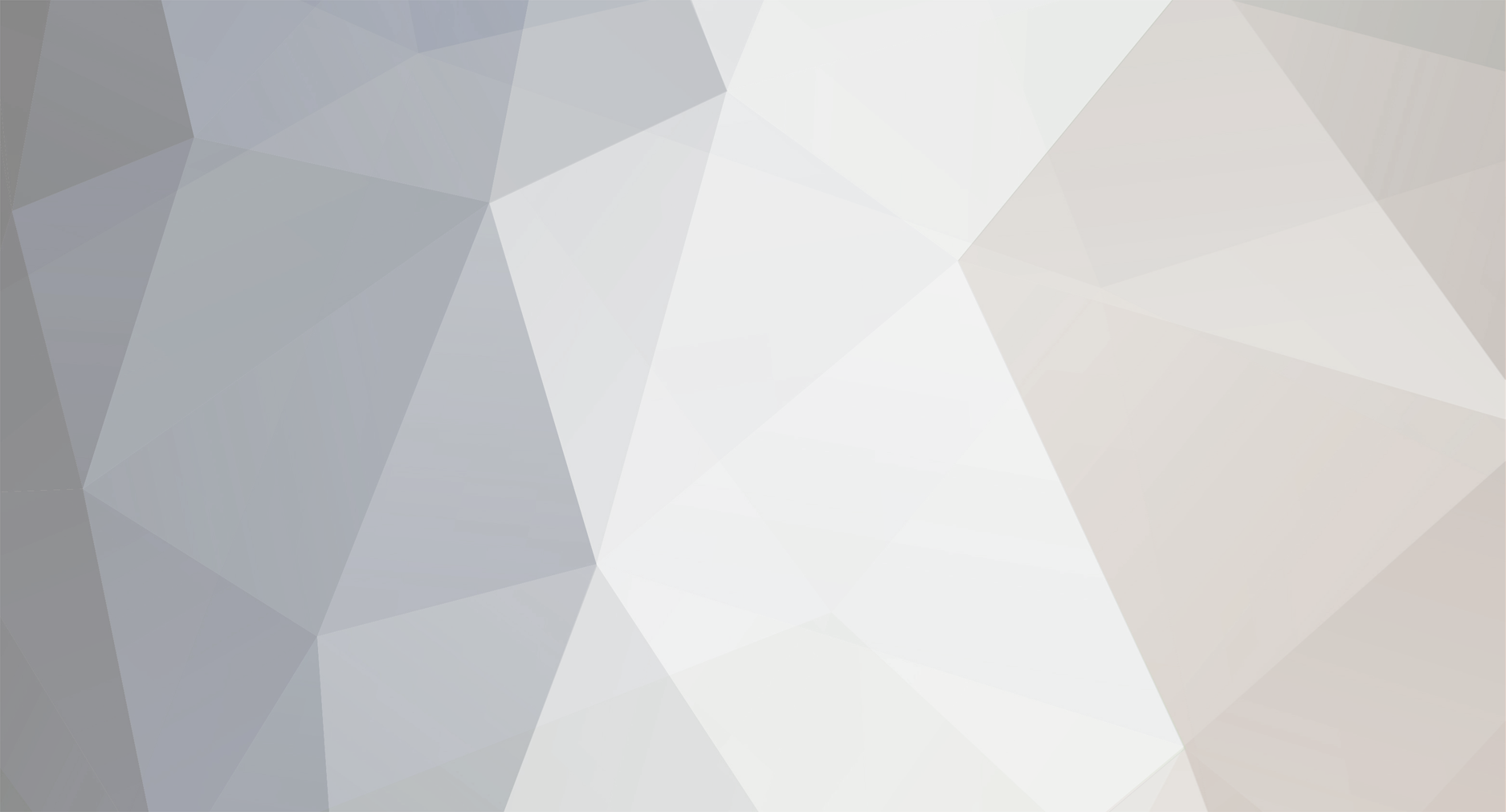
jeremywesselman
Members-
Posts
154 -
Joined
-
Last visited
Never
Everything posted by jeremywesselman
-
You will want to use the 'id' column to select the user because you will never have the same 'id', but you could have the same 'name'. So. When populating your select box, you'll want to put the user's 'id' in the value of the <option> and the user's name in the display part. [code]<?php echo "<option value='{$nt["id"]}'>{$nt["username"]}</option>"; ?>[/code] Then when you want to pull the user from the db you will use a query like this: [code]<?php $query = "SELECT * FROM member_messages WHERE id = $id"; ?>[/code] Where * is whatever columns you want to select. It will then find the 'id' that is equal to $id and give you all the columns requested. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
As yonta has already stated, your constructor will be named after your class with PHP4. Also, you will use [b]var[/b] to declare your variables instead of [b]public[/b] or [b]private[/b]. [code]<?php class Gallery { var $thumbdir = "photos/".$_GET['folder']."thumbs/"; var $imagedir = "photos/".$_GET['folder']; var $image = $imagedir.$_GET['image']; var $MAX = 200; var $start = $_GET['start']; var $end; function Gallery($thumbdir,$imagedir,$image,$start,$end = $start+$max) { $this->thumbdir = $thumbdir; $this->imagedir = $imagedir; $this->image = $image; $this->MAX = $end; $this->displayStart = $start; $this->displayEnd = $end; }// end construct function display() { return $this->thumbdir; } } //end the class $gal = new Gallery($thumbdir,$imagedir,$image,$start,$MAX); $gal->display(); ?>[/code] [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
When updating a record, you have to tell it which record to update. You are not doing this. You will tell it which record you want to update by using your table's primary key. Which should be 'id' if your table is set up correctly. [code]<?php ... $query="UPDATE member_info SET name='$name', password='$password', email='$email', band_name='$band_name', music_played='$music_played', number_of_musicans='$number_of_musicans', playing_history='$playing_history', band_personalty='$band_personalty', number_of_played_venues='$number_of_played_venues', club='$club' , pub='$pub' , partys='$partys', functions='$functions', house_party='$house_party', weedings='$weedings', churches='$churches', how_much_per_hour_do_you_charge='$how_much_per_hour_do_you_charge', cash='$cash', credit_card='$credit_card', both='$both', terms_condition='$terms_condition', not_play_out_date='$not_play_out_date', dates1='$dates1', dates2='$dates2', dates3='$dates3', dates4='$dates4', date_added='$date_added', end_date='$end_date', user_ip='$user_ip', level='$level' WHERE id = $id"; ... ?>[/code] [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
What version of PHP are you using? Because PHP4 OOP and PHP5 OOP have some syntax differences. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
This is the part you will use in your form processor. [code]<?php $featured = $_POST['featured']; if($featured == "Yes") $price = $config_price_featured; else $price = $config_price; ?>[/code] And you would set up your select box like this. [code]... <select name="featured"> <option value="Yes">Yes</option> <option value="No">No</option> </select> ...[/code] I think this is what you're lookin' for. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
The easiest way to do this by your specifications would be to use an 'id' column in your database table, assuming that you have one. Just select the last x records from your table, order by 'id' descending. [code]<?php $query = "SELECT * FROM table ORDER BY id DESC LIMIT 10"; ?>[/code] Your query would look something like that. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
I think what you are saying is that you want to be able to edit all of the values from the admin table and update them all together. And the 'config' table has two columns. A 'property' and a 'value'. The only way that I can think of to update a table like this is to update each value separately. At least that's the only simple way to do it. I'm not saying it can't be done, I just can't think of a more simple way. An easier way to do this would be to make just one row in the 'config' table and make the column titles the property and the first row table cell the value. This way you'll be able to pull them all out by one query and update them all with one query. Hope this helps... [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
[code] <?php ... for($i = 0; $i < 9; $i++) mysql_query($query[i]) or die(mysql_error() . ". Report this to blah@blah.com"); ... ?>[/code] You need to add a $ in front of the $query[i]. It should be $query[$i]. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
can anyone help .....
jeremywesselman replied to the apprentice webmaster's topic in PHP Coding Help
Can you give us the code that you are using to connect? Thx. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--][b]Jeremy[/b][!--colorc--][/span][!--/colorc--] -
PHP MVC Section
jeremywesselman replied to jeremywesselman's topic in PHPFreaks.com Website Feedback
Alright. Maybe when the technique of coding in MVC becomes more popular. I was just throwin' out an idea. Thanks for all those who replied. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
PHP MVC Section
jeremywesselman replied to jeremywesselman's topic in PHPFreaks.com Website Feedback
Yes, you could. But I was thinking more of a 'Help' section dedicated to just MVC. Whether it is in a framework or not. Things like techniques and such. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
I was thinking that you could add a section for the discussion and for assistance with PHP MVC frameworks. I think it could help anybody looking to start developing in MVC frameworks. What do you think? [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
[!--quoteo(post=358387:date=Mar 25 2006, 05:45 PM:name=neewah)--][div class=\'quotetop\']QUOTE(neewah @ Mar 25 2006, 05:45 PM) [snapback]358387[/snapback][/div][div class=\'quotemain\'][!--quotec--] You probarbly shouldn't post 18 days later especially with a useless reply :\ [/quote] You don't say!
-
I just wanted to thank all the mods for the quick turnaround in the freelancing forum. You all are doing a great job keeping up with it. Thanks for all of your help in every forum on the site. I will be making another donation to phpfreaks very soon. Another way would be to only let the member who posted the topic originally edit and add new replies. This probably wouldn't be too difficult to implement. Just add some code to disable the reply button unless you are a mod or the topic starter. Thanks again, [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
Thanks mods. You took care of the problem very quickly. I can understand how difficult it must be to keep up with all the posts that are being posted. A suggestion might be to get some more moderators, but that is probably up to phpfreak. Anyways, thanks for taking care of the freelancing section and all the sections for that matter. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
I just wanted to comment on how users are not reading the rules for posting in the freelancing section. I don't know about a lot of people, but I check phpfreaks.com often for freelancing work. About 15 - 20 times a day actually. And lately, there have been a lot of people replying in the freelancing section which is a big no no. But it seems lately that the mods aren't maintaining it like they used to. They used to edit every post with a message explaining to users that they should not post replies and that they should contact the author of the post via an email or by pm. It seems that they are not editing these posts anymore and the replies are building up. I just wanted to see what was going on and if anybody else gets aggravated by this. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--]
-
Dreamweaver Newbie Needs Help!
jeremywesselman replied to EdgeWalker's topic in Editor Help (PhpStorm, VS Code, etc)
What "mode" are you typing the code in? There is a "Code", "Both" OR "Design". Just checking all of the facts... FYI: You'll want to be in "Code" Mode if you don't already know. [!--coloro:#990000--][span style=\"color:#990000\"][!--/coloro--]Jeremy[!--colorc--][/span][!--/colorc--] -
You don't need a special program to copy and paste. Jeremy
-
deleting user id from DB
jeremywesselman replied to xtiancjs's topic in Editor Help (PhpStorm, VS Code, etc)
Well, on your logout page or in your logout function, just have a query to delete the current userid. Here is the sql syntax you would use to delete the user "DELETE FROM table WHERE userid = user_id" Jeremy -
You'll need to go into your cpanel(if you have one) and add your ip address to the "access hosts" list in the mysql databases page. It should be close to the bottom. You might have to create a new username and password to access from your computer also. Jeremy
-
First, you need to change the "name" property of your textboxes to something that describes what is in them. So, if the first textbox is "name", you will need to change "name = 'textfield'" to "name='name'". Do this for the rest of the form. Then you are going to start off by setting up the process part of the page. [!--PHP-Head--][div class=\'phptop\']PHP[/div][div class=\'phpmain\'][!--PHP-EHead--][span style=\"color:#0000BB\"]<?php [/span][span style=\"color:#007700\"]if(isset([/span][span style=\"color:#0000BB\"]$_POST[/span][span style=\"color:#007700\"][[/span][span style=\"color:#DD0000\"]\'Submit\'[/span][span style=\"color:#007700\"]])) { [/span][span style=\"color:#FF8000\"]//Enter form processing here [/span][span style=\"color:#007700\"]} else { [/span][span style=\"color:#FF8000\"]//Your form could go here so it doesn\'t show up when you submit it. [/span][span style=\"color:#007700\"]} [/span][span style=\"color:#0000BB\"]?>[/span] [/span][!--PHP-Foot--][/div][!--PHP-EFoot--] So after this, we will need to get all the values of the textboxes on your form. [!--PHP-Head--][div class=\'phptop\']PHP[/div][div class=\'phpmain\'][!--PHP-EHead--][span style=\"color:#0000BB\"]<?php [/span][span style=\"color:#007700\"]if(isset([/span][span style=\"color:#0000BB\"]$_POST[/span][span style=\"color:#007700\"][[/span][span style=\"color:#DD0000\"]\'Submit\'[/span][span style=\"color:#007700\"]])) { [/span][span style=\"color:#0000BB\"]$name [/span][span style=\"color:#007700\"]= [/span][span style=\"color:#0000BB\"]$_POST[/span][span style=\"color:#007700\"][[/span][span style=\"color:#DD0000\"]\'name\'[/span][span style=\"color:#007700\"]]; [/span][span style=\"color:#0000BB\"]$email [/span][span style=\"color:#007700\"]= [/span][span style=\"color:#0000BB\"]$_POST[/span][span style=\"color:#007700\"][[/span][span style=\"color:#DD0000\"]\'email\'[/span][span style=\"color:#007700\"]]; [/span][span style=\"color:#FF8000\"]//etc... You get the point. [/span][span style=\"color:#007700\"]} else { [/span][span style=\"color:#FF8000\"]//form [/span][span style=\"color:#007700\"]} [/span][span style=\"color:#0000BB\"]?>[/span] [/span][!--PHP-Foot--][/div][!--PHP-EFoot--] After you collect all of your data, go ahead and do any error checking you want on it. (Check if it is empty, correct, etc). Then comes the mail part. [!--PHP-Head--][div class=\'phptop\']PHP[/div][div class=\'phpmain\'][!--PHP-EHead--][span style=\"color:#0000BB\"]<?php [/span][span style=\"color:#007700\"]... [/span][span style=\"color:#FF8000\"]//the mail function [/span][span style=\"color:#0000BB\"]$to [/span][span style=\"color:#007700\"]= [/span][span style=\"color:#DD0000\"]\"\" [/span][span style=\"color:#FF8000\"]//The person you are sending it to [/span][span style=\"color:#0000BB\"]$subject [/span][span style=\"color:#007700\"]= [/span][span style=\"color:#DD0000\"]\"\" [/span][span style=\"color:#FF8000\"]//The subject of the email [/span][span style=\"color:#0000BB\"]$message [/span][span style=\"color:#007700\"]= [/span][span style=\"color:#DD0000\"]\"\" [/span][span style=\"color:#FF8000\"]//The message you want to appear in the body of the email [/span][span style=\"color:#0000BB\"]$email [/span][span style=\"color:#007700\"]= [/span][span style=\"color:#DD0000\"]\"\" [/span][span style=\"color:#FF8000\"]//The person email address who is sending email [/span][span style=\"color:#0000BB\"]mail[/span][span style=\"color:#007700\"]([/span][span style=\"color:#0000BB\"]$to[/span][span style=\"color:#007700\"], [/span][span style=\"color:#0000BB\"]$subject[/span][span style=\"color:#007700\"], [/span][span style=\"color:#0000BB\"]$message[/span][span style=\"color:#007700\"], [/span][span style=\"color:#DD0000\"]\"FROM: $email\"[/span][span style=\"color:#007700\"]); ... [/span][span style=\"color:#0000BB\"]?>[/span] [/span][!--PHP-Foot--][/div][!--PHP-EFoot--] The mail part goes in the processing part of the script. Jeremy
-
Need help......
jeremywesselman replied to johndoedoedoe's topic in Editor Help (PhpStorm, VS Code, etc)
What you want done is very easy to do. I have looked a couple places but I have found nothing to show you exactly how to do it exactly like you want it. If you need help, email me at jeremywesselman [at] insightbb [dot] com and I could walk you through it so we don't clog the board up. Jeremy -
inserting date into database
jeremywesselman replied to mattennant's topic in Editor Help (PhpStorm, VS Code, etc)
Yes, it all goes on the same page. I tested on my own server before I posted here and it works for me. Jeremy -
inserting date into database
jeremywesselman replied to mattennant's topic in Editor Help (PhpStorm, VS Code, etc)
[!--PHP-Head--][div class=\'phptop\']PHP[/div][div class=\'phpmain\'][!--PHP-EHead--][span style=\"color:#0000BB\"]<?php [/span][span style=\"color:#007700\"]if(isset([/span][span style=\"color:#0000BB\"]$_POST[/span][span style=\"color:#007700\"][[/span][span style=\"color:#DD0000\"]\'submit\'[/span][span style=\"color:#007700\"]])) { [/span][span style=\"color:#0000BB\"]$month [/span][span style=\"color:#007700\"]= [/span][span style=\"color:#0000BB\"]$_POST[/span][span style=\"color:#007700\"][[/span][span style=\"color:#DD0000\"]\'month\'[/span][span style=\"color:#007700\"]]; [/span][span style=\"color:#0000BB\"]$day [/span][span style=\"color:#007700\"]= [/span][span style=\"color:#0000BB\"]$_POST[/span][span style=\"color:#007700\"][[/span][span style=\"color:#DD0000\"]\'day\'[/span][span style=\"color:#007700\"]]; [/span][span style=\"color:#0000BB\"]$year [/span][span style=\"color:#007700\"]= [/span][span style=\"color:#0000BB\"]$_POST[/span][span style=\"color:#007700\"][[/span][span style=\"color:#DD0000\"]\'year\'[/span][span style=\"color:#007700\"]]; [/span][span style=\"color:#0000BB\"]$eventDate [/span][span style=\"color:#007700\"]= [/span][span style=\"color:#0000BB\"]$month [/span][span style=\"color:#007700\"]. [/span][span style=\"color:#DD0000\"]\" \" [/span][span style=\"color:#007700\"]. [/span][span style=\"color:#0000BB\"]$day [/span][span style=\"color:#007700\"]. [/span][span style=\"color:#DD0000\"]\", \" [/span][span style=\"color:#007700\"]. [/span][span style=\"color:#0000BB\"]$year[/span][span style=\"color:#007700\"]; echo([/span][span style=\"color:#0000BB\"]$eventDate[/span][span style=\"color:#007700\"]); } else { [/span][span style=\"color:#0000BB\"]?> [/span]<form action=\"[span style=\"color:#0000BB\"]<?=$_SERVER[/span][span style=\"color:#007700\"][[/span][span style=\"color:#DD0000\"]\'PHP_SELF\'[/span][span style=\"color:#007700\"]][/span][span style=\"color:#0000BB\"]?>[/span]\" method=\"post\"> Enter Event Date: <select name=\"month\"> <option value=\"Jan\">Jan</option> <option value=\"Feb\">Feb</option> <option value=\"Mar\">Mar</option> <option value=\"April\">April</option> </select> <select name=\"day\"> <option value=\"1\">1</option> <option value=\"2\">2</option> <option value=\"3\">3</option> <option value=\"4\">4</option> <option value=\"5\">5</option> <option value=\"6\">6</option> <option value=\"7\">7</option> </select> <select name=\"year\"> <option value=\"2005\">2005</option> <option value=\"2006\">2006</option> </select> <input type=\"submit\" value=\"Submit\" name=\"submit\"> </form> [span style=\"color:#0000BB\"]<?php [/span][span style=\"color:#007700\"]} [/span][span style=\"color:#0000BB\"]?>[/span] [/span][!--PHP-Foot--][/div][!--PHP-EFoot--] All you have to do is concatenate all of the values of the selects. You can format it however you want. Jeremy -
Navigation
jeremywesselman replied to keeper.garrett1's topic in Editor Help (PhpStorm, VS Code, etc)
[!--PHP-Head--][div class=\'phptop\']PHP[/div][div class=\'phpmain\'][!--PHP-EHead--][span style=\"color:#0000BB\"]<?php [/span][span style=\"color:#FF8000\"]//Use the switch() to set up navigation [/span][span style=\"color:#007700\"]switch([/span][span style=\"color:#0000BB\"]$_GET[/span][span style=\"color:#007700\"][[/span][span style=\"color:#DD0000\"]\'id\'[/span][span style=\"color:#007700\"]]) { case [/span][span style=\"color:#DD0000\"]\'1\'[/span][span style=\"color:#007700\"]: { include([/span][span style=\"color:#DD0000\"]\'page1.php\'[/span][span style=\"color:#007700\"]); } break; case [/span][span style=\"color:#DD0000\"]\'2\'[/span][span style=\"color:#007700\"]: { include([/span][span style=\"color:#DD0000\"]\'page2.php\'[/span][span style=\"color:#007700\"]); } break; default: { include([/span][span style=\"color:#DD0000\"]\'home.php\'[/span][span style=\"color:#007700\"]); } break; } [/span][span style=\"color:#0000BB\"]?>[/span] [/span][!--PHP-Foot--][/div][!--PHP-EFoot--] Then have your menu set up like "index.php?id=1" and it will load page1.php. Jeremy