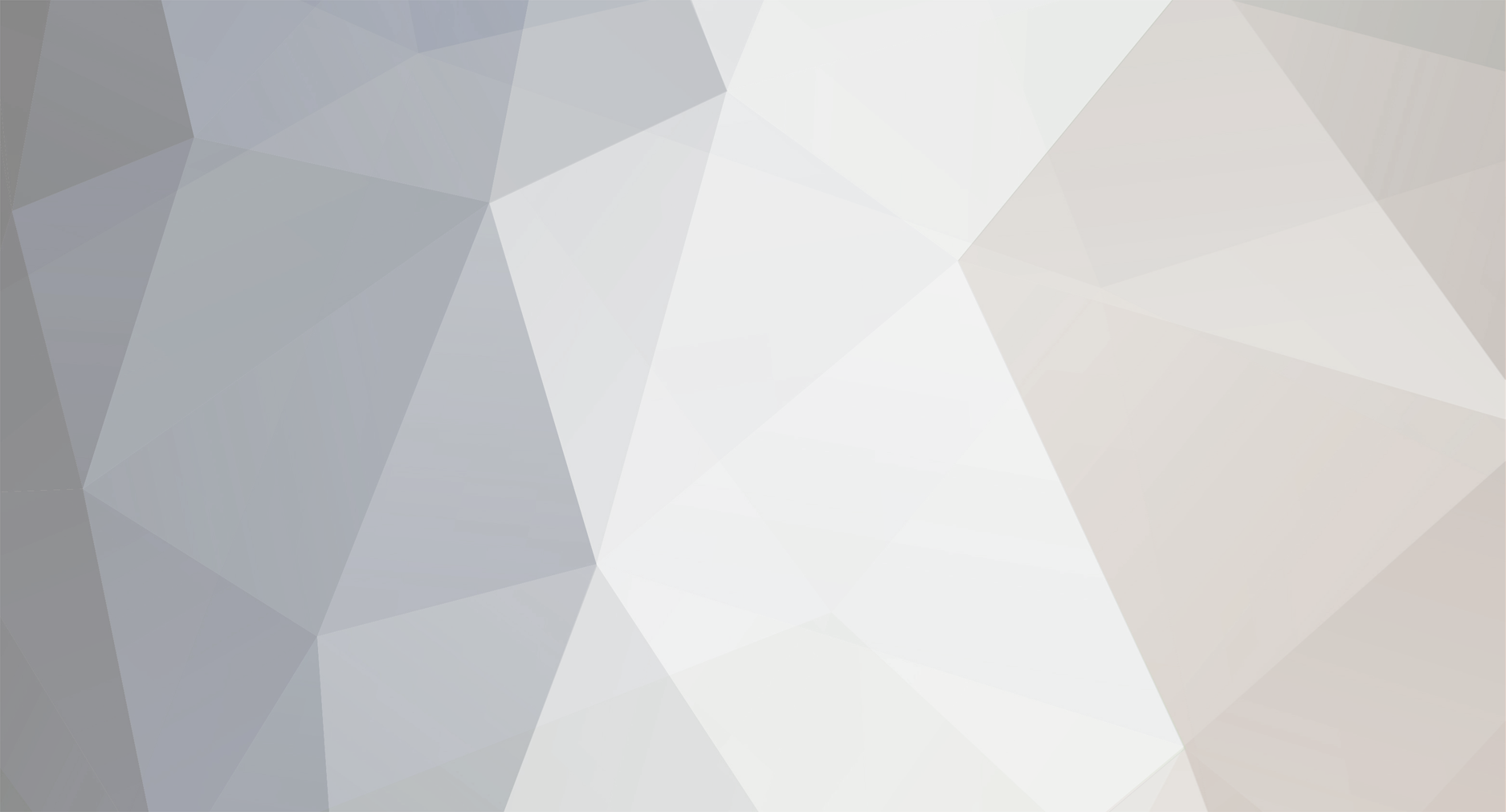
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
[SOLVED] Preventing [spaces] on a subject or message.
GingerRobot replied to Gath's topic in PHP Coding Help
Try stripping out any unneeded white space with trim before you check the length: <?php $str = ' '; $str = trim($str); if(strlen($str) < 3){ //invalid title }else{ //title is fine } ?> Of course, you'll also be wanting to use something like mysql_real_escape_string to prevent any sql injection. -
You're missing the braces around your else statement starting on line 149. Try: else{ foreach ($errors as $msg) echo " - $msg<br/>\n"; } You'll also need to add in something to show an error mesage if the user gets the captcha wrong.
-
PHP comparing time for custom session timeouts
GingerRobot replied to JoelRocks's topic in PHP Coding Help
Why store days/minutes/hours/seconds? Surely you're better off storing a unix timestamp? -
Unless im being completely blind, you've not included the part of the code that sets the cookie. Therefore, we can't suggest where the output is coming from. Also, the error message will tell you what line the header was already sent by. It would be useful to have that information. Help us help you.
-
Try: <?php session_start(); session_id($_GET['PHPSESSID']);//set php session id from URL $hostname= "localhost"; $user= "remotepa_framewo"; $password = "-"; $conn = @mysql_connect( $hostname, $user, $password ) or die ("Could not connect to server"); $db = @mysql_select_db("remotepa_framework", $conn) or die ("Could not connect to database"); $sql = "SELECT * FROM users WHERE username='".$_SESSION['username']."'"; $result = mysql_query( $sql, $conn) or die ("Could not execute query"); $output = mysql_fetch_assoc($result); if(isset($_SESSION['username'])) { if($output['active'] < 1) { echo ("Please reset your details"); echo ("Hello " .$_SESSION['username']); echo ("<br />"); echo ("You are logged in successfully"); } } else { echo ("Sorry you are not logged in"); exit; } ?> You need to start the session before any output. You also need to start it before making use of the $_SESSION array. The reason for the mysql_fetch_assoc error is because there are no results being returned by your query, because $_SESSION['username'] is undefined without starting the session previously
-
Well, if thats all the code in the script, $connect in undefined. If its not, then the error could be generated elsewhere in the script.
-
[SOLVED] Multidimensional Array - Breadcrumb Menu
GingerRobot replied to tarun's topic in PHP Coding Help
Well, with the array set up as you have it, you'll run into problems. You can't have an element of an array as BOTH a string and an array. For instance, you have $submenu["Contact Us"]["Live Chat"] equal to the string "/chat/index.php" and it is also an array. If you were to modify your $submenu array so that it only ever has two dimensions, the first being the parent node, the second the child, you can write a recursive function similar to: <?php function menu($menu,$index='Parent'){ foreach($menu[$index] as $k => $v){ echo "<li><a href='$v'>$k</a></li>"; if(is_array($menu[$k])){ echo '<ul>'; menu($menu,$k); echo '</ul>'; } } } $menu = array(); $menu["Parent"]["Home"] = "/index.php"; $menu["Parent"]["Contact Us"] = "/contactus.php"; $menu["Parent"]["Members"] = "/members/index.php"; $menu["Home"]["About"] = "/about.php"; $menu["Contact Us"]["Live Chat"] = "/chat/index.php"; $menu["Live Chat"]["Live Help"] = "/chat/connected.php"; $menu["Contact Us"]["Email"] = "/emailform.php"; echo '<ul>'; menu($menu); echo '</ul>'; ?> Give that a shot. P.S. 1000th post EDIT: Reposted with a neater function. -
Perhaps an example? <?php if(isset($_POST['submit1'])){ echo 'You clicked the first submit button!'; }elseif(isset($_POST['submit2'])){ echo 'You clicked the second submit button!'; }else{ echo 'You havn\' pressed a button yet'; } ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="POST" /> <input type="submit" name="submit1" value="Click Me!" /><br /> <input type="submit" name="submit2" value="No, Click Me!" /> </form>
-
Presumably there are two queries running here, one of which generates the total, the other the list of players. I assume there must be a slight difference in the two queries.
-
Just to add, you can connect a wireless router to a wired router...thats how mine's set up at home. We already had a wired modem router, then got a laptop. My dad bought a wireless router thinking it also had a modem in it, but it didn't. Never got round to buying a wireless modem router, so it's stayed that way ever since.
-
Well, if you mean that a sentence could end with a ?/!/. then i would suggest you have two options. 1.) Regular expressions. 2.) Cycle through the string character by character and break it apart where necessary. Since regular expressions are annoying me at the moment: <?php $str = "Hello! Is anybody out there? Obviously not. I'm all alone"; $i=0; $z = 0; $sentences = array(); while($i<strlen($str)){ $sentences[$z] .= $str[$i]; if($str[$i] == '!' || $strlen[$i] == '.' || $strlen[$i] == '?'){ $z++; } $i++; } print_r($sentences); ?> If thats not the issue, then im not sure how you would tell where a sentence ends.
-
Getting external data from a logged in user
GingerRobot replied to FalcorTheDog's topic in PHP Coding Help
http://www.google.co.uk/search?hl=en&q=php+cURL+login+to+yahoo&meta= That should get you started. -
Getting external data from a logged in user
GingerRobot replied to FalcorTheDog's topic in PHP Coding Help
Yeah, it is a job for cURL. This does mean that you would have to get the login details from the user, which they might not be happy to do. -
The time() function doesn't accept any parameters. You just ned to change the format you are using for your date function: $mydate = date("U", mktime(18, 30, 0, 8, 24, 2007));
-
Autoselecting a value in a dropdown menu
GingerRobot replied to HaLo2FrEeEk's topic in PHP Coding Help
Well, if its all going through index.php and you pass an action to it through the URL, then it would be something like: <?php $action = $_GET['action']; $pages = array('news','roster');//fill the array with all of your pages echo '<select name="page">'; foreach($pages as $v){ echo "<option value='$v'"; echo $action==$v? "selected='selected'": ""; echo ">$v</option>"; } echo '</select>'; ?> Makes it much easier by putting all the possible pages in an array. -
Variable variables?: <?php $query = "SELECT city FROM cities ORDER BY city"; $results = mysql_query($query); while ($row = mysql_fetch_array ($results, MYSQL_ASSOC)) { $$row['city'] = $row['city']; } ?>
-
explode?
-
There seems to be a few people Who Type Like That. Am i the only one who finds it damn hard to read? I can't quite put my finger on why, but it irritates me beyond belief. Surely it must also take a lot of effort to type like that?!
-
Further maths is a bit of a bitch i have to say. Managed to get the A in it, but im really not looking forward to FP2. FP1 was hard enough!
-
Reading Material - General Computing not PHP
GingerRobot replied to GingerRobot's topic in Miscellaneous
Thanks a lot for the input - certainly gives me some topics to look for. Haha. Im now waiting for the inevitable post that says exactly that. Or perhaps no-one will now ive said this. Perhaps ive changed the future... Perhaps i should shut up and go to bed. -
Not that im aware of. A google define seems to suggest they are the same too
-
Hmm, didn't notice that was a php 4 function too. Its exactly the same, just much shorter!
-
[SOLVED] Arrays (3D array? or just value change?)
GingerRobot replied to colombian's topic in PHP Coding Help
You can nest your foreach loops: <?php $forms = array(array( 'fname' => "First name",'type' => "text"),array( 'lname' => "Last name", 'type' => 'text') ); foreach($forms as $k => $v){ foreach($v as $k2 =>$v2){ echo $k2.' : '.$v2.'<br />'; } } ?> Remember, the whole point of multidimensional arrays is that each element within the array is an array in itself. Therefore, you work with each value of the array as you would any other single dimensional array. Hmm, i wonder if i could have put 'array' into those two sentences anymore often that i did. -
I dont suppose i can explain this any better than the manual can: www.php.net/explode I guess ill try anyway The explode function splits a string by whatever you put in the first parameter(the delimiter). It creates an array of all the pieces of the string between all delimiters found within the string. In your exact example: <?php $comp_code = '<some code>£$@</some code>.'; $i_titl = 'foo bar'; $code = explode("£$@", $comp_code); echo $code[0] . $i_titl . $code[1]; //produces <some code>foobar</somecode>. ?> Beaten to it, thought id post with the example using the posted code. Completely agree that the manual explains it though.
-
Rather like: <?php $timestamp = time(); $offset = date('Z'); $gmt_timestamp = $timestamp - $offset; echo date('H:i:s',$gmt_timestamp); ?>