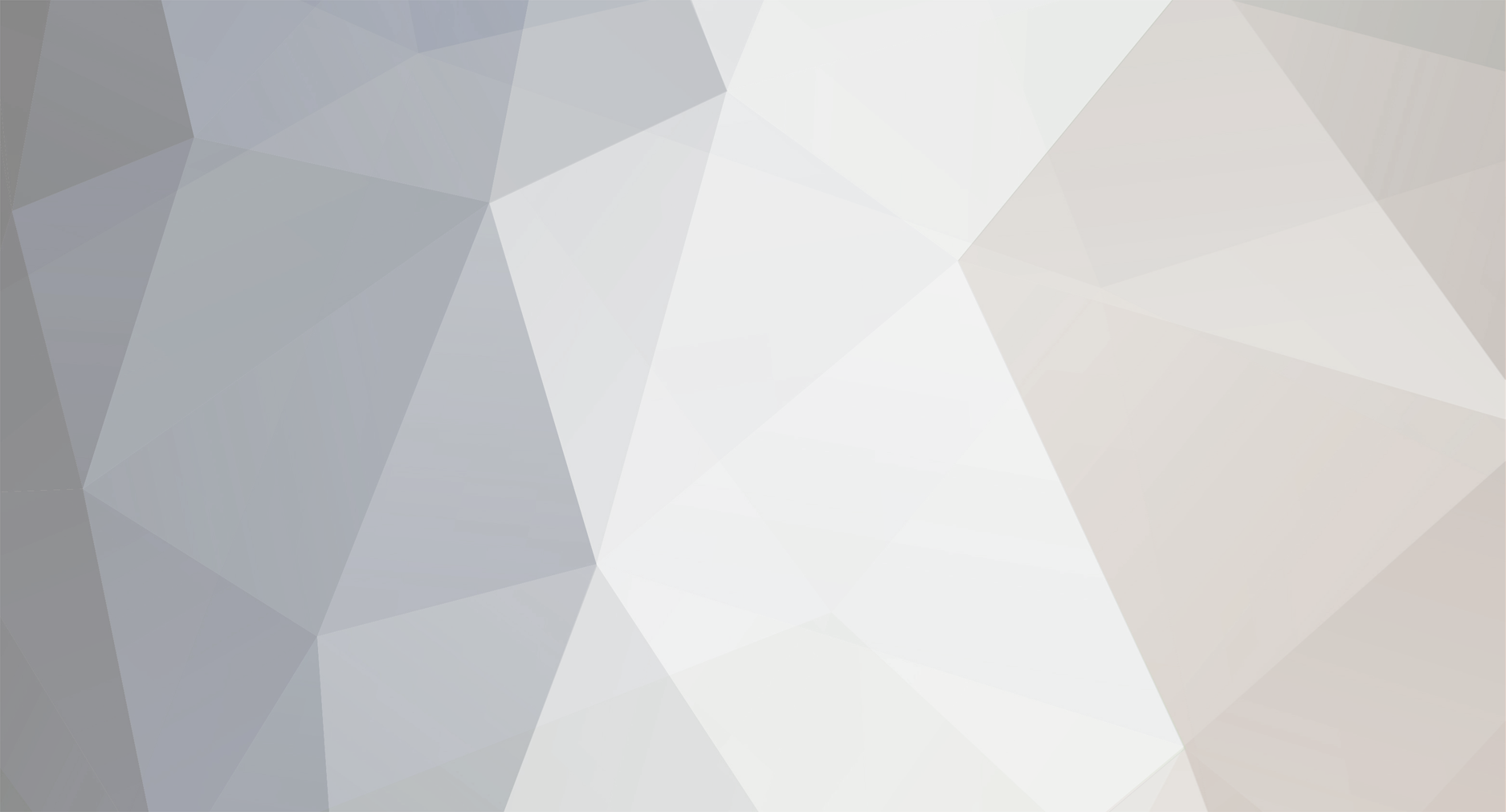
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Is there lots to this code? If not do you think you could post it up inside tags(without the spaces).
-
You need to convert your dates to unix timestamps, either using the mysql UNIX_TIMESTAMP() function, or with the php strtotime() function to allow you to make a comparison: <?php $date1 = '2007-08-01'; $date2 = '2006-08-01'; $diff= strtotime($date1) - strtotime($date2); $days = $diff/(60*60*24); echo $days; ?>
-
I would change your if statement to: if(isset($_POST['submit_x')) { } Again, due to register_globals being off, the variable submit_x isn't defined. It is contained within the $_POST superglobal array. You'll need to change all of your variables that are from your form to be taken from the $_POST array. The reason why i dropped the request method part of your if statement is that if something is set inside the $_POST array, then the method is going to be post.
-
I completely disagree. Why have a while loop when you dont need one? When you come back to look at that, it would be very easy to get confused. You'll be expecting that code block to repeat and it doesn't. Yeah, thats right. Although the braces are unnecessary. Personally, if i'm retrieving just one field from a single row, i tend to use the mysql_result() function - seems to make your intentions clearer that way. However, thats just a matter of opinion.
-
Im a little confused by the last part of your code. However, something like this should do what you are after: <?php $files = array('20070704_A_01.pdf','20070704_B_01.pdf','20070704_A_01.pdf'); $letters = array(); foreach($files as $v){ $bits = explode('_',$v); $letters[] = $bits[1]; } $letters = array_unique($letters); print_r($letters);//with my array, output is Array ( [0] => A [1] => B ) ?>
-
Haha. It won't be long before google do know what you had for breakfast.
-
As was explained, your problems are all down to register_globals. Just thought i would add that the $REQUEST_METHOD variable is part of the $_SERVER superglobal array. So, with register_globals on, you would access its contents with $_SERVER['REQUEST_METHOD'];
-
This isn't possible purely in PHP. Since php is a server side language, it has already been executed once the page has loaded. However, you could possibly achieve this using an ajax technique: 1.) Your php page loads. You record the time of the page load for the user in your database. 2.) You use the javscript onunload event to run a function which makes a request to a php page, which takes the differance between the time it is called, and the time in the database, to work out how long a user has been on a page. Of course, it would be completely useless for people without javascript/who have javascript turned off.
-
Whoops. Forgot some semi colons. And i can't even blame that on it being tired and late - its midday! Try: function get_ads($u) { //connect to db $connection = db_connect(); //retrieve all ads placed by user but not activated. $query="SELECT * FROM ads WHERE user_id='$u'"; $result= mysql_query($query)or die(mysql_error()); $num = mysql_num_rows($result); if($num == 0) { return false; }else{ //parse error return $result; } }
-
Naa, there is a function that takes an array and removes all duplicates from it: array_unique(), but not one that just gives you the duplicates.
-
Thought that might happen when i edited, probably should have added a new reply rather than editing. Ah well. Take a look at the code i suggested.
-
It would be nice if you could tell us where the error occurs? Which line does the error message tell you it happens on? And do you think you could point that line out to us? It'd make it a whole stack easier to help you... Edit: Hmm, i guess i wasn't paying all that much attention - you did sort of say where it happens. I think, you need to change the way your function works slightly. You'll need to pass the result resource back to the script, and then use mysql_fetch_assoc() function in the main script: <?php function get_ads($u) { //connect to db $connection = db_connect(); //retrieve all ads placed by user but not activated. $query="SELECT * FROM ads WHERE user_id='$u'"; $result= mysql_query($query)or die(mysql_error()); $num = mysql_num_rows($result); if($num == 0){ return false }else{ return $result } } ?> $u=$_SESSION['user_id']; $ads=get_ads($u); if ($ads) { echo "<h2>You have the following advertisements pending activation</h2><br/>"; echo '<table id="t4" align="center">'; echo '<caption align="center"><b>Advertisement Summary<b></caption>'; echo '<tr><th>Ad Ref</th><th>Date Placed</th></tr>'; while($row = mysql_fetch_assoc($ads)) { echo '<tr><td>'; echo ($row['ad_ref']); echo '</td>'; echo '<td>'; echo ($row['date_placed']); echo '</td></tr>'; } echo '</table>' ; include ('./includes/footer.html'); } else{ echo "You have no advertisements awaiting activation"; include ('./includes/footer.html'); exit(); }
-
Well, if you're interested: <?php $array = array('J/1' => 'J1' ,'J/2' => 'J2' ,'J/3' => 'J3' ,'J/4' => 'J1' ,'J/5' => 'J3' ,'J/6' => 'J6' ,'J/7' => 'J7'); $duplicate = array(); $t1 = microtime(true);//start timer for($i=0;$i<=10000;$i++){ $duplicate = array(); sort($array);//re-index array with numeric keys $n = count($array); for($x=0;$x<$n;$x++){ for($y=$x+1;$y<$n;$y++){ if($array[$x] == $array[$y]){ $duplicate[] = $array[$x]; } } } } $t2 = microtime(true);//intermediate time for($i=0;$i<=10000;$i++){ $duplicate = array(); foreach($array as $value) { if ( count(array_keys($array, $value)) > 1 && !in_array($value, $duplicate) ) $duplicate[] = $value; } } $t3 = microtime(true);//end time printf ("1st Method: %0.8f<br>2nd Method: %0.8f<br>Ratio (1st method/2nd method): %0.2f", $t2-$t1, $t3-$t2, ($t2-$t1)/($t3-$t2)); Results: 1st Method: 0.38158202 2nd Method: 0.52205086 Ratio (1st method/2nd method): 0.73 Of course, even over 10000 runs of each, there's still only aroun 0.1 of a second differance, so its not exactly all that important. Id probably use yours, for the less typing Lastly, if the array already had numerical indexes and there was no need to sort, then the differance in speed between mine and yours is more noticeable
-
I couldn't get yours to work clearstatcache(which ive just noticed was my fault, whoops!), so here's mine. Looks like more code, but in a topic a while back, Barand showed that it was faster than using in_array(). <?php $array = array('J/1' => 'J1' ,'J/2' => 'J2' ,'J/3' => 'J3' ,'J/4' => 'J1' ,'J/5' => 'J3' ,'J/6' => 'J6' ,'J/7' => 'J7'); sort($array);//re-index array with numeric keys $n = count($array); for($x=0;$x<$n;$x++){ for($y=$x+1;$y<$n;$y++){ if($array[$x] == $array[$y]){ echo 'Duplicate value:'.$array[$x].'<br />'; } } } ?>
-
As far as i'm aware, this shouldn't happen. It was my understanding that you can MAKE it happen, by using the ignore_user_abort() function. Perhaps i'm wrong. Edit: You can also set this in php.ini - perhaps this has been turned on in there for some reason.
-
Yeah, i think we are going to need to see some code. You have other issues too - according to your calender, the 1st of August(today) is a sunday. Also, there is a missing day between each end and start of the month. On your calender, August finishes on a monday, and September starts on a wednesday.
-
Well, being younger than yourself, i can't exactly give any amazing advice; however, you could always look into doing some freelance work to start with. That would allow you to gain experience, build up a portfolio of work and, of course, earn some money!
-
Do you think you could tell us what you mean when you say, its not working? What does it do at the moment? What is it supposed to do? Without that, it's going to be hard for someone to know where to look within your code.
-
Adding to the array is as simple as doing $array[] = $var. There's a few examples in the user comments on the php site for the mt_rand function for random hex codes. Ive used one for this example: <?php $array = array("#ff0000","#ff8800","#0022ff","#33ff33"); for($x=0;$x<9;$x++){ //thanks to zolaar at nothanks dot com - taken from the a comment on http://uk3.php.net/mt_rand $num = mt_rand ( 0, 0xffffff ); $output = sprintf ( "%06x" , $num ); $array[] = '#'.$output; } print_r($array); ?>
-
Sure, use the date and strtotime functions: <?php $month = 01; echo date('F',strtotime("$month/01")); ?>
-
No problem. And if you did understand it, you did better than me. Took me an age to grasp the concept!
-
Tis a job for javascript. Google timed javascript redirect.