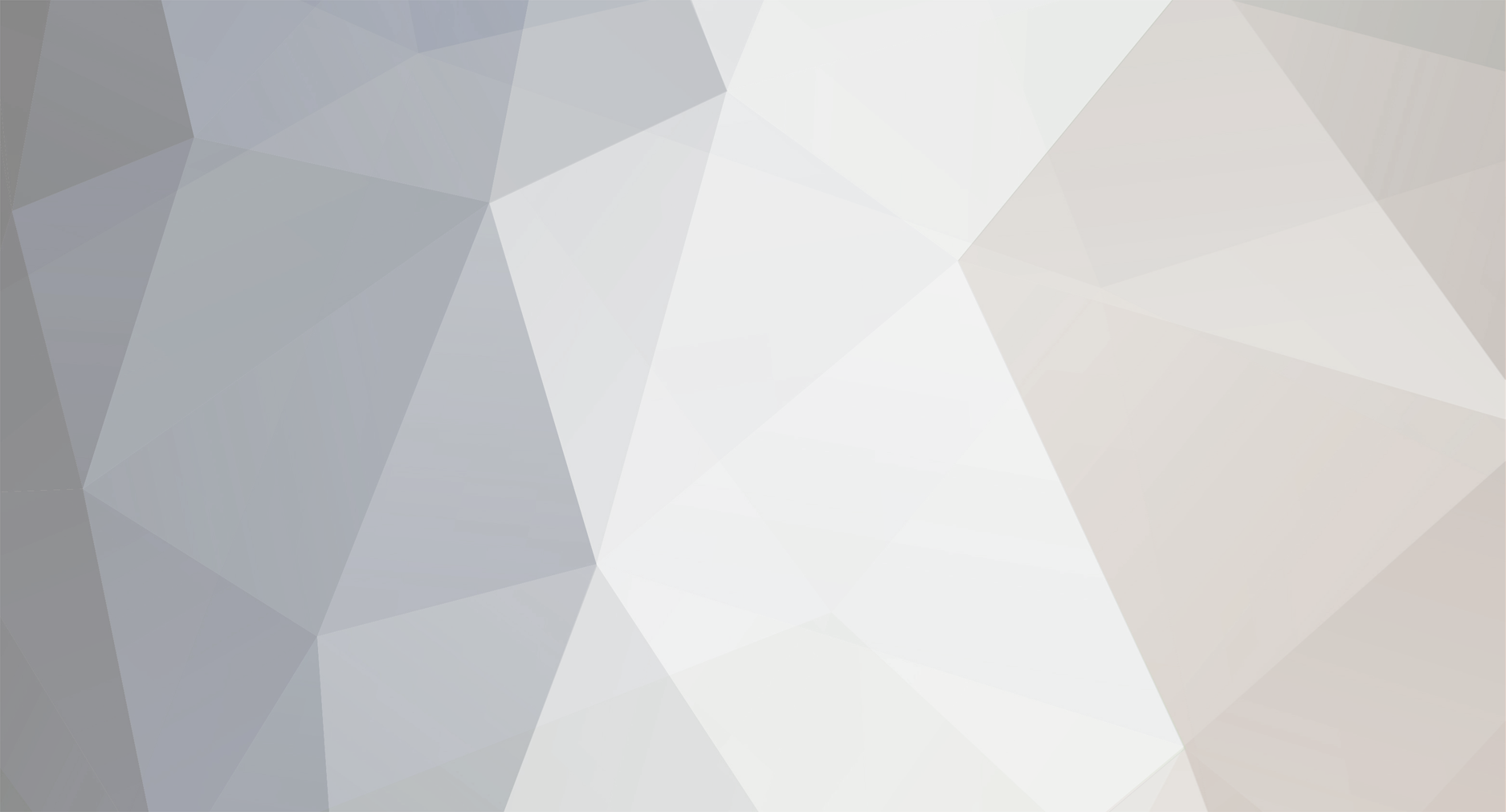
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Well you probably dont need all of those lines of code for the query, but aside from that, if you are wanted to retreive all the boxers that match your criteria, and show their details, then try: [code] <?php $age=$_POST['age']; $weight=$_POST['weight']; $bouts=$_POST['bouts']; ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> <title>Untitled Document</title> </head> <body> <?php $link = mysql_connect('mysql2.streamline.net', 'smallheath', '-') or die('Could not connect: ' . mysql_error()); $db_selected = mysql_select_db('smallheath', $link) or die ('Error while connecting to database: ' . mysql_error()); ?> <!--set up the table --> <table border="1" cellpadding="5"> <tr> <th>First Name</th> <th>Last Name</th> <th>Age</th> <th>Weight</th> <th>Bouts</th> <th>Senior</th> <th>Gender</th> </tr> <?php // build and execute the query //Age Query $query= "SELECT * FROM boxers WHERE age="; $query .= $age; $query .= "-1"; $query .= " or age="; $query .= $age; $query .= " or age="; $query .= $age; $query .= "+1"; //Weight Query $low=$weight-3; $high=$weight+3; $query= "SELECT * FROM boxers WHERE weight >="; $query .= $low; $query .= " and weight <="; $query .= $high; //Bout Query $low= "$bouts-3"; $high= "$bouts+3"; $query = "SELECT * FROM boxers WHERE bouts >="; $query= "$low"; $query= "and bouts <="; $query= "$high"; $results = array(mysql_query($query)); //loop through results while ($row = mysql_fetch_array($results)) { // print out the code for each row echo <<<END <tr> <td>$row['first_name']</td> <td>$row['last_name'</td> <td>$row['age']</td> <td>$row['weight']</td> <td>$row['bouts']</td> <td>$row['senior']</td> <td>$row['gender']</td> </tr> END; } ?> <!-- Close Table --> </table> </body> </html> [/code] Previously, i think you got a little confused with this part: [code] <?php $row = $fn['first_name']; $row = $ln['last_name']; $row = $age['age']; $row = $weight['weight']; $row = $bouts['bouts']; $row = $sen['senior']; $row = $gen['gender']; ?> [/code] I think you meant to write: $fn = $row['first_name']; etc Ive not bothered to assign the contents to shorter variable names - unless you're going to be using them later on, it seems like a waste of time. I think thats what you were wanting.
-
So you want to multiply everysingle person's drugs fact by 100? I would do it like: [code] <?php $sql = "UPDATE `players` SET `UTCQ`=`UTCQ`*'100'"; mysql_query($sql) or die(mysql_error() . ' in <br />' . $sql); ?> [/code]
-
How to run a script when a hyperlink is pressed
GingerRobot replied to pantinosm's topic in PHP Coding Help
Well then you make the link direct to the page that contains the the query? Or is the point that you are redirecting to a page/site out of your control and are, perhaps, recording which pages/sites a user has viewed? In which case, i think yes, you would have to use ajax. -
Problem with backslash before every " and '...Help
GingerRobot replied to itsureboy's topic in PHP Coding Help
Yes, by default on most hosts, the php setting magic_quotes is turned on. This automatically excapes information from get and post data. Characters such as quotes, semi colons etc can either cause problems with your code or can be used for a malicious purpose. The magic_quotes setting prevents this. To remove the slashes for outputing the data etc, use the function stripslashes() http://uk2.php.net/stripslashes -
This is called pagination. Take a look at this tutorial here: http://www.phpfreaks.com/tutorials/147/0.php
-
Yes, you could always set up a function. However, you shouldn't use ereg_replace or preg_replace for this kind of thing, str_replace(or str_ireplace if you want it to be case insensitive) is faster. The other two should be used if you want to use regular expressions.
-
Well it looks to me like you are searching for the whole of the text within the variable result, and you are searching within the same variable, so it will replace the whole thing. Here is a sample of how you should use it, im not using your variables because i dont know what they are [code] <?php $text = "A test sentence"; $search_term = "test"; $output = str_replace($search_term,"<font style='background-color:yellow;'>$search_term</font>",$text); echo $output; ?> [/code] So you look for the search term, replace it with the search term against a background in yellow, but you look for the search term within the whole text.
-
How to run a script when a hyperlink is pressed
GingerRobot replied to pantinosm's topic in PHP Coding Help
Im not quite sure what you are asking here. Do you think you could give a bit more information? Is it that you have lots of information and want to have links for searching and sorting this data? What type of searches would this be? -
If you simply want to show all of the available pages, then just use a loop: [code] <?php $pages = ceil($numrows/5); $i = 0; while($i <= $pages){ $rownumber = $i * 5; echo $rownumber; echo " <A HREF='$php_self?pfg=$rownumber'>Page $i</A> "; $i++; } ?> [/code] If you wanted to show, say 2-3 pages each side of the current page then it gets a little more complicated, but its just going to require a few if statements.
-
So your code is just: [code] <?php while( $youdata > 0 && $odata > 0) { $opponents_health -= rand(5,10); } [/code] With nothing else before the start of this? If so i dont really understand. There is no syntax error there
-
Well that code should not produce the error you have given at the moment. Im assuming that you have cut something out of the while statement otherwise that will produce an error - as at the moment it will enter an infinite loop. Are you sure you are still getting the exact same error?
-
Can you please show us the new code then?
-
while($youdata > 0 && $odata >) Your while statment is incompete. There is nothing for $odata to be greater than.
-
I wonder if you could write the string contents to a file then include the file? Not sure if there is an easier way of doing it though...
-
The . is used as the concatenation operator(had to get my book out for the spelling!) It is used to append text/variables to another variable. The .= appends the following to the original variable. for instance: [code] <?php $a = 'some text'; $b = ' more text'; $c = $a.$b; echo $c;//echos some text more text echo '<br />'; $c.= ' even more text'; echo $c;//echos some text more text even more text ?>[/code]
-
So you have links similar to <a href="yourpage.php?sortal=a">A</a> etc? Well then this is how i would do it: <?php $letter = mysql_real_escape_string($_GET['sortal']);//using mysql_real_escape_string to prevent any malicous use of the sort $sql = "SELECT * FROM `yourtable` WHERE `userfield` LIKE '$letter%'"; mysql_query($sql) or die (mysql_error()); ?> You use LIKE to allow you to place wildcard matches in your search term. A % siginfies any number of any character, and an underscore is used for one character of any type. Here, you want the letter that has been selected followed by any number of other characters. If no letter has been selected, then you would have a searchterm of just % in which case all results would be found.
-
"Column count doesn't match value count at row 1"
GingerRobot replied to oskare100's topic in PHP Coding Help
You've enclosed num_logins and plus 1 in single quotes - you could either leave out the quotes in this section entirely, or to make it obvious what you are doing, place the field name in backticks (`) and the number in single quotes. Backticks are used to refer to a field within mysql - they are not necessary for the script to work, but they can help you to see what you are doing. "(CURRENT_DATE,`num_logins`+'1') where user_id = '$vuserid'"; As for your second question i would take a look at this page: http://uk.php.net/manual/en/function.mysql-real-escape-string.php It is for the function that you should use to prevent these types of attacks and also gives you an example of how they would work. It probably explains it better than i could. -
Well it would seem that it is your host then. Perhaps you could contact them?
-
Im having problems with the onDrag event in firefox - it seems to do absolutely nothing. Im wondering if this has an obvious solution - perhaps firefox doesn't support the onDrag event? If that's the case, does anyone know of an equivilant? Any help would be much appreciated - as much as i hate IE, its doing me a favour by working at the moment and i dont like that :p
-
Im definately getting there...its not a problem with my ajax as if i use your stylesheet and html with my javascript/ajax, it seems to work fine... So ive just got to work from yours to mine to find out whats not working. Ben
-
Ok, i shalll have a look at that in more detail when i have some more time later. Thank you very much for posting that. Ben
-
Ok, its going to be easier for me to show you whats happening here: http://www.kingpins-london.com/chat/mainpage.php Just type a name in to start the "chat". Its set up at the moment so that it will just show content from a php page, but it checks that every 2 seconds. If you try it in firefox, everything works fine - because the content doesn't change it looks like its not even trying to refresh it. But if you try in IE, it appears to flicker and the scroll bars jump up and down - im wondering if this is something to do with IE not cutting the text off properly at the end of the cell. Im using CSS to achieve the scroll bar inside the table with overflow: auto; Im not sure if my problem lies with my ajax or with my css/javascript or perhaps it is just IE If anyone can point me in the right direction it would be much appreciated.
-
It doesn't matter. We just need to see what the query actually is doing.
-
Ah i only just noticed that you had commented this line out: // echo $sql; for testing purposes remove the comments to see what the query is showing.
-
What is shown after $sql is echoed?