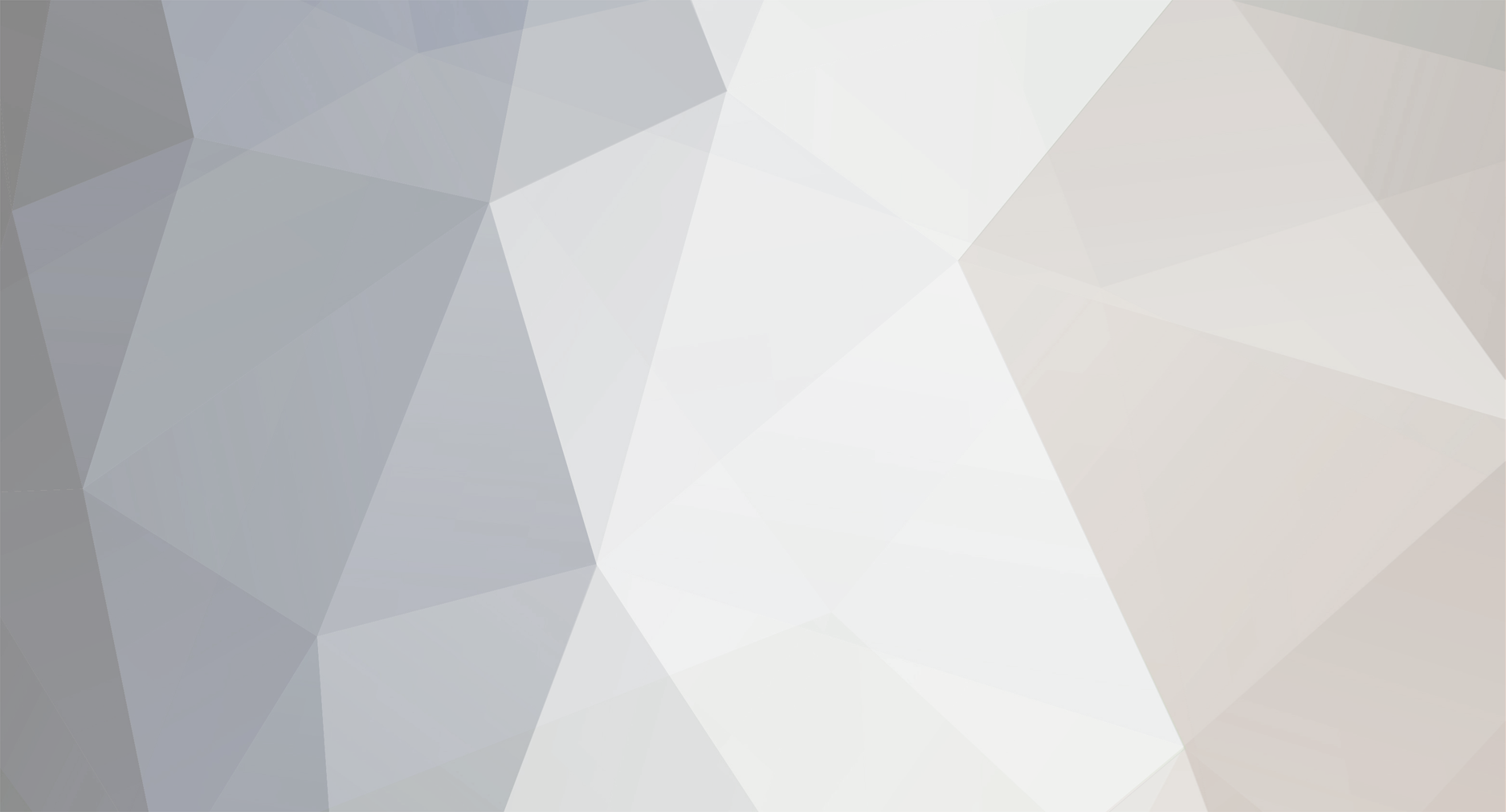
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
This would work: [code] <?php $curr_year = date('Y'); $curr_month =date('m'); $old_year = 2004; $old_month = 8; $months_passed = ($curr_year-$old_year)*12+$curr_month-$old_month; echo $months_passed; ?> [/code] Purely based on calendar months.
-
I would think you would be better creating the thumbnail when the image is uploaded. That way you are only doing this piece of processing once, rather than doing it every time someone views the image.
-
What you will need to do is create another table in your database, perhaps called unique_hits. You would need 3 fields: ip, tutorial_id and time. then, when someone views the page, you will check to see if an entry on the same IP for that tutorial exists. If it does, then dont add to the hit counter, if it doesn't, add to the counter and insert an entry into the new table. The reason why i say include a time field is because, if i were you, i would remove entries from this new table that are an hour/day old otherwise you are going to have a huge table on your hands.
-
Yes. In your log in process you need to set a session. So, if the user should be logged in, use: [code] <?php $_SESSION['username'] = $username;//change to whatever variable contains the username ?> [/code] You will also need to put: [code] <?php session_start(); ?> [/code] At the top of the log in script. Then, i would recommend enclosing the following in a new php file, perhaps called logincheck.php: [code] <?php session_start(); if(empty($_SESSION['username']){//check to see if there is anything in the session; if not, redirect to the log in page header("location:login.php"); exit; } ?> [/code] Then, all of your files that require a user to be logged in, use: [code] <?php include("logincheck.php"); ?> //all of the rest of the html [/code] You will also need to change all of these to php files.
-
I think it might just be a problem with your HTML. You have a hidden field called action, but it has no value. So change: <input type="hidden" name="action"> to: <input type="hidden" name="action" value="register"> Hopefully its something as simple as that. Cant see anything wrong with your switch statement.
-
Would it not be confusing for a user to send a 404 error for something like a bad query string? Would it not be better to give them a message saying what the problem was etc?
-
From the manual: require() and include() are identical in every way except how they handle failure. include() produces a Warning while require() results in a Fatal Error. In other words, don't hesitate to use require() if you want a missing file to halt processing of the page. include() does not behave this way, the script will continue regardless. Be sure to have an appropriate include_path setting as well.
-
Copying an old mysql column to a new column?
GingerRobot replied to extrovertive's topic in PHP Coding Help
Out of interest, why does it sleep for 1 second after 20 entries? What purpose does that serve? -
trying to build a myspace-like site... help?
GingerRobot replied to CircularStopSign's topic in PHP Coding Help
There are a couple of tutorials on here which could help you get started. They are for membership systems which will be the main part of your site http://www.phpfreaks.com/tutorials/65/0.php http://www.phpfreaks.com/tutorials/78/0.php The second one is a follow up and talks about image uploading. -
Login to Password Protected Directories using PHP and MYSQL
GingerRobot replied to Wildhalf's topic in PHP Coding Help
Why is that you need to password protect directories? A login system would usually use sessions. There are a couple of tutorials on here which could help you: http://www.phpfreaks.com/tutorials/40/0.php http://www.phpfreaks.com/tutorials/65/0.php They show how to create membership systems. -
You will need to use a differant variable name to store it all for later, plus you can use substr() to take off the last character. [code] <?php //Select User Email from Database $rsUserEmail = mysql_query("SELECT * FROM contacts WHERE carrier_id = '$cid' AND contacts.rates = '1'") or die(mysql_error()); // store the record of the "example" table into $row $allemails = '';//give it some value other wise php5 gives a notice while($row = mysql_fetch_array($rsUserEmail)){ // Print out the contents of the entry $email = $row['contact_email']; echo "$email; "; // Here I get result a@aol.com; b@aol.com; c@aol.com; $allemails = $allemails.$email.';'; } $allemails = $str = substr($allemails,0,strlen($allemails)-1); echo '<br />'; echo $allemails; ?> [/code]
-
The number_format() function is your friend here, you can use it show 1000 separators, specify the number of decimal places and the synbol used to show a decimal place http://uk.php.net/number_format
-
What is it supposed to do?
-
Hey im new and looking for book suggestions
GingerRobot replied to PigsHidePies's topic in PHP Coding Help
I have bought PHP and MySQL Web Development By Luke Welling and Laura Thompson and i have found that it is very good. I use it both as a general reference book and to learn about specific things. It also includes some real life examples(one of which is building a CMS), and whilst i have not tried these, the other code in the book is well explained and very useful so i am confident that these will be good too. -
Sorry when i said change the variables i meant change the ones you are using when you connect to the database. I imagine that you have in your functions page: $username = 'nicky'; This will be overwriting the $_SESSION['username'];
-
redirect to original page on login...not working :(
GingerRobot replied to bcamp1973's topic in PHP Coding Help
try: [code] <?php if(!empty($_POST['redir'])) { header('Location: '.$_POST['redir']); } else { header('Location: http://my.server.domain/?pg=default_page'); } exit(); ?> [/code] -
Can we see what code you are using? Im a little bit mistified by what you mean by the header-Location. if you are doing something like this: header("location:connectionpage.php"); Then thats not a very good way to do it. You should be using include: include("connectionpage.php"); But im not sure. You might mean something differant.
-
redirect to original page on login...not working :(
GingerRobot replied to bcamp1973's topic in PHP Coding Help
And what happens when you try this? -
register_globals is a php setting. You can only change it if you have ability to change you php.ini settings
-
probably not a good idea to show your database username and password on the forum. But yes, this is due to register_globals. If you have a session called $_SESSION['var'] and then in a page use the variable $var, it will overwrite the contents of $_SESSION['var']; Ideally, you'd want to turn register_globals off if possible. Otherwise, change the database username and password variables to something like: $dbusername $dbpassword
-
It is probably the max_execution_time again. Try raising that some more.
-
"When I run the form right now" So you manage to use the form the first time the page loads, but after you submit it, you get that message? Or do you never see the form at all? Sorry, im just a bit unclear on that.
-
Im struggling to understand why you'd need a loop; surely one person isn't going to sign up more than one account? Try something like this: [code] <html> <body> <?php $host= 'localhost'; $user = 'root'; $passwd = ''; $my_database = 'login'; $userid = $_POST['userid']; $username = $_POST['username']; $password = $_POST['password']; $firstname = $_POST['firstname']; $lastname = $_POST['lastname']; $address = $_POST['address']; $city = $_POST['city']; $postcode = $_POST['postcode']; $connect = mysql_connect($host, $user, $passwd); mysql_select_db($my_database);//moved this here becuase i would say it is confusing to have it further down the script. $table_name = 'user'; $sql= "INSERT INTO `user` ( `userid` , `username` , `password` , `firstname` , `lastname`, `address`, `city`, `postcode` ) " . " VALUES ( '$userid', '$username', '$password', '$firstname', '$lastname', '$address', '$city', '$postcode');"; } $results_id = mysql_query($sql); if ($results_id) { print 'SignUp Successfull!<p />'; } else { die ("Query=$sql failed! <p />"); } echo "<form name='add' method='POST' action='login.php'>"; echo "<input type='submit' value='Back'>"; echo "</form>"; mysql_close($connect); ?> </table> </body> </html> [/code]
-
So what is it actualyl happening at the moment? Also, that still doesn't define $work. Unless you have register_globals on im pretty sure you're going to need to do $work = $_POST['work']; Unless $work isn't supposed to be the same as the work field in the form.
-
I dont really have any ideas, but someone else might want to see the code so you could start by posting that. Also, perhaps it is the webhost? Are your other pages working ok?