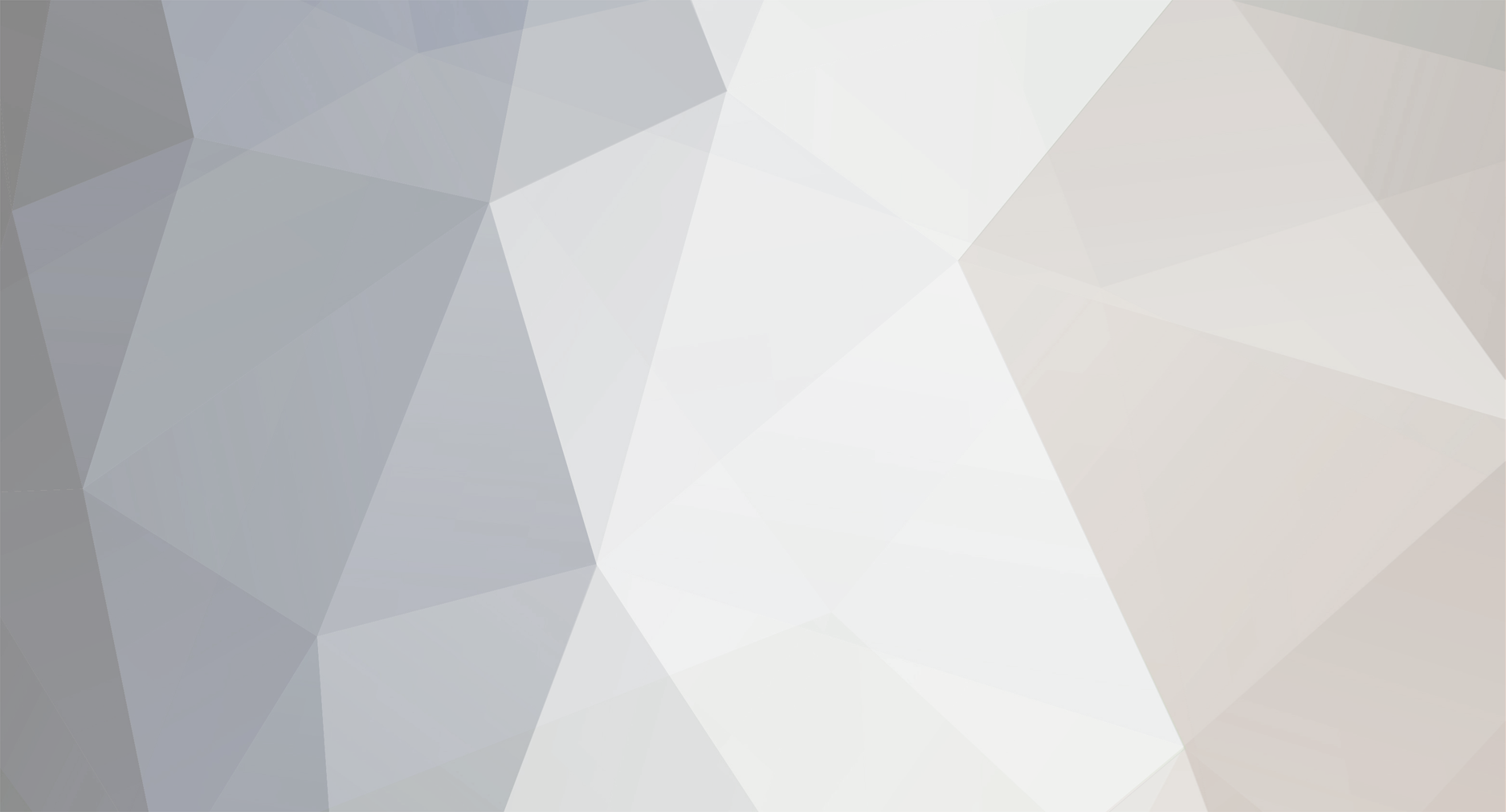
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Try here. Seriously, try actually looking in the manual. Unlike most manuals, it is actually an incredibly useful resource.
-
Though if it's to be a directory tree it may well need to be recursive, which is a little harder. However, there are examples of those in the user comments on the manual page as well.
-
i can not see my barcode and i have error
GingerRobot replied to bakigkgz's topic in PHP Coding Help
A browser is capable of displaying lots of different formats - text files, images, HTML pages etc. You're asking it to display two different types simultaneously - a png image and HTML. It can't do that. To display images in an HTML page, you must use the image tag. You need to move the image creation to a separate file. Let's call it image.php: <?php session_start(); include("dbconfig.php"); $code =$_SESSION['ran_barcode']; //echo "$code"; if (!$_SESSION['loggedIn']) { header("location:login.php"); die (); } else { function UPCAbarcode($code) { $lw = 2; $hi = 100; $Lencode = array('0001101','0011001','0010011','0111101','0100011', '0110001','0101111','0111011','0110111','0001011'); $Rencode = array('1110010','1100110','1101100','1000010','1011100', '1001110','1010000','1000100','1001000','1110100'); $ends = '101'; $center = '01010'; /* UPC-A Must be 11 digits, we compute the checksum. */ if ( strlen($code) != 11 ) { die("UPC-A Must be 11 digits."); } /* Compute the EAN-13 Checksum digit */ $ncode = '0'.$code; $even = 0; $odd = 0; for ($x=0;$x<11;$x++) { if ($x % 2) { $odd += $ncode[$x]; } else { $even += $ncode[$x]; } } $code.=(10 - (($odd * 3 + $even) % 10)) % 10; /* Create the bar encoding using a binary string */ $bars=$ends; $bars.=$Lencode[$code[0]]; for($x=1;$x<6;$x++) { $bars.=$Lencode[$code[$x]]; } $bars.=$center; for($x=6;$x<12;$x++) { $bars.=$Rencode[$code[$x]]; } $bars.=$ends; /* Generate the Barcode Image */ $img = ImageCreate($lw*95+30,$hi+30); $fg = ImageColorAllocate($img, 0, 0, 0); $bg = ImageColorAllocate($img, 255, 255, 255); ImageFilledRectangle($img, 0, 0, $lw*95+30, $hi+30, $bg); $shift=10; for ($x=0;$x<strlen($bars);$x++) { if (($x<10) || ($x>=45 && $x<50) || ($x >=85)) { $sh=10; } else { $sh=0; } if ($bars[$x] == '1') { $color = $fg; } else { $color = $bg; } ImageFilledRectangle($img, ($x*$lw)+15,5,($x+1)*$lw+14,$hi+5+$sh,$color); } /* Add the Human Readable Label */ ImageString($img,4,5,$hi-5,$code[0],$fg); for ($x=0;$x<5;$x++) { ImageString($img,5,$lw*(13+$x*6)+15,$hi+5,$code[$x+1],$fg); ImageString($img,5,$lw*(53+$x*6)+15,$hi+5,$code[$x+6],$fg); } ImageString($img,4,$lw*95+17,$hi-5,$code[11],$fg); /* Output the Header and Content. */ header("Content-Type: image/png"); ImagePNG($img); } UPCAbarcode($code); } ?> You then call the file in an image tag: ... <img src="image.php" /><br /> <input type="text" name="hello" value="hello"> ... Any clearer? -
i can not see my barcode and i have error
GingerRobot replied to bakigkgz's topic in PHP Coding Help
The problem is that you're telling the browser that the content it is expected to receive is a png image. You then try and display HTML too, which it has no idea what to do with. You need to move the image creation to a separate file, and display it using an HTML image tag. -
Is table your actual table name? If so, it's a reserved word and cannot be used without being escaped with backticks (`)
-
If you're trying to delete all data, then try using TRUNCATE: TRUNCATE TABLE numbers Otherwise, can we see the relevant code?
-
Can we see the output from this line? echo $put_CopiedLot; It's usually much easier to see the sql syntax error when you havn't got all the php confusing things.
-
Well what do you have so far? If you've managed to write a registration type page, you're obviously comfortable enough with gathering form data and using it in a query. That's obviously the first part - take whatever information the user types in and query the database with it. If you've found tutorials showing you how to search a database and print out the results then all you need to do is adapt those tutorials so that the results are printed inside the form.
-
Well, you could decide what numbers are likely to represent which characters and perform a conversion. But there's so many different ways in which someone could modify the text slightly: miss-spellings, underscores, dashes etc. A potential solution would be to use the levenshtein distance - though it might be too slow. I'd probably just use someone else's spam filter and let them do the hard work
-
How big is the text file in question?
-
[SOLVED] With Pagination You Have to Run the Same Query Twice?
GingerRobot replied to limitphp's topic in PHP Coding Help
So to actually answer the question: yes, you do. Edit: Well, not quite the same query. The first should simply select a count of the rows. The second should select the fields you wish to display. -
Wouldn't it make more sense to count the number of occurrences of each of the phrases? For example, data containing 10 occurrences of one of the phrases must surely be more likely to be spam than data containing just 1 occurrence?
-
I don't think so. The only alternative would be to have some application run on the server which resizes the images, which could be called by your PHP script. Of course, that would only be better if you could find something that resized whilst using less resources.
-
curl php script works on localhost windows, but not on linux server
GingerRobot replied to jjk2's topic in PHP Coding Help
You're right. I missed that. -
The error is the memory limit one, yes? And what is your memory limit and image size? I just ran a test on a ~3Mb image and just creating the image from the jpeg used nearly 50MBs of memory.
-
curl php script works on localhost windows, but not on linux server
GingerRobot replied to jjk2's topic in PHP Coding Help
The only thing i can obviously see wrong is that all of these test on method: if($method == HEAD) Are using an unquoted string. They should be: if($method == "HEAD") Now, given the fact that you've not mentioned you're getting a warning about that, i'm going to assume that either you don't have error_reporting set to E_ALL or that you don't have display_errors turned on or both. Go do that and run the script again. -
I'm slightly lost as to what you're trying to achieve with all the color and palette functions. As far as i can see, this part: imagetruecolortopalette($simg, false, 256); // Create New Color Pallete $palsize = ImageColorsTotal($simg); for ($i = 0; $i < $palsize; $i++) { // Counting Colors In The Image $colors = ImageColorsForIndex($simg, $i); // Number Of Colors Used ImageColorAllocate($dimg, $colors['red'], $colors['green'], $colors['blue']); // Tell The Server What Colors This Image Will Use Is redundant. I'm not sure why you wanted to convert the original image to a palette? And the next lines really do nothing. ImageColorAllocate() does nothing except to provide color identifiers for use with other functions, except that the first call will fill the background of an image created with imagecreate(). But given that the image identifier you're using is the one you're going to copy to, there doesn't seem much point. Perhaps i've missed something along the way.
-
$s is sitll undefined. In fact, i think you might be causing yourself a lot of headaches with the way you're dealing with the page numbers. Rather than passing around all these different variables (you seen to have $s, and a to and from variable in the URL(which you're doing nothing with)) - just use a single variable to use as the page number. Let's call it $page, and pass it around in the URL. For starters, you'll need to make sure it's set. If it's not, default to page 1: if(isset($_GET['page'])){ $page = (int) $_GET['page']; //type casting to ensure $s is an int }else{ $page = 1; } Now, you're limits of the query can then be ascertained from the page number. The start is ($page-1) * $limit and the number of records is obviously just $limit. Finally, for your pages at the bottom, you can just compare $page with 1 and the number of pages to work out if you should show previous and next links. E.g.: if($page!=1){ //show previous link with page set to $page-1; } if($page > $totalpages){ //show next link with page set to $page+1. $totalpages is simply ceil($rows/$limit) } You might want to take a look at this tutorial if you're still stuck.
-
Yep - you perform the search each time the page loads so you need to know what your searching for everytime.
-
Something like this: $var = 'abc'; if(preg_match("|^[A-Z0-9_]{3,}$|i",$var)){ //valid name } Which would only allow names that are composed of alphanumeric characters and underscores and are at least 3 characters long.
-
Is magic_quotes turned on?
-
adding a email verification to my register script
GingerRobot replied to chris_s_22's topic in PHP Coding Help
As an aside, if you just want to ascertain whether or not the value exists in the database, it is far more efficient to perform a query that selects a count, rather than returning all rows and using the php mysql_num_rows() function. For example: $sql = "SELECT COUNT(*) FROM user WHERE confirm_code ='$passkey'"; $result = mysql_query($sql) or trigger_error(mysql_error(),E_USER_ERROR); if(mysql_result($result,0) == 1){ //code found } -
[SOLVED] Add image upload and insert record to email script.
GingerRobot replied to emediastudios's topic in PHP Coding Help
For a basic file uploader, see the manual page Edit: Apologies redarrow; i missed your last post. -
If you want to be this restrictive, use a whitelist rather than a blacklist. That is, state that filenames must only contain alphanumeric characters, plus underscores for example. You can't forget characters you didn't want to allow this way. It's a much easier regex this way too.
-
Help needed with formatting a DB Query? - Example Shown
GingerRobot replied to Solarpitch's topic in PHP Coding Help
Sure; just change it so the old ticket number is echoed instead of the current one(edit: except the first time through; you'll want the current ticket number then) and remove the check that currently prevents the first line being blank. Edit again: That didn't make much sense. Just have it echo out the current ticket number on a blank line every time it changes. Remove the check that prevents this happening the first time the loop runs.