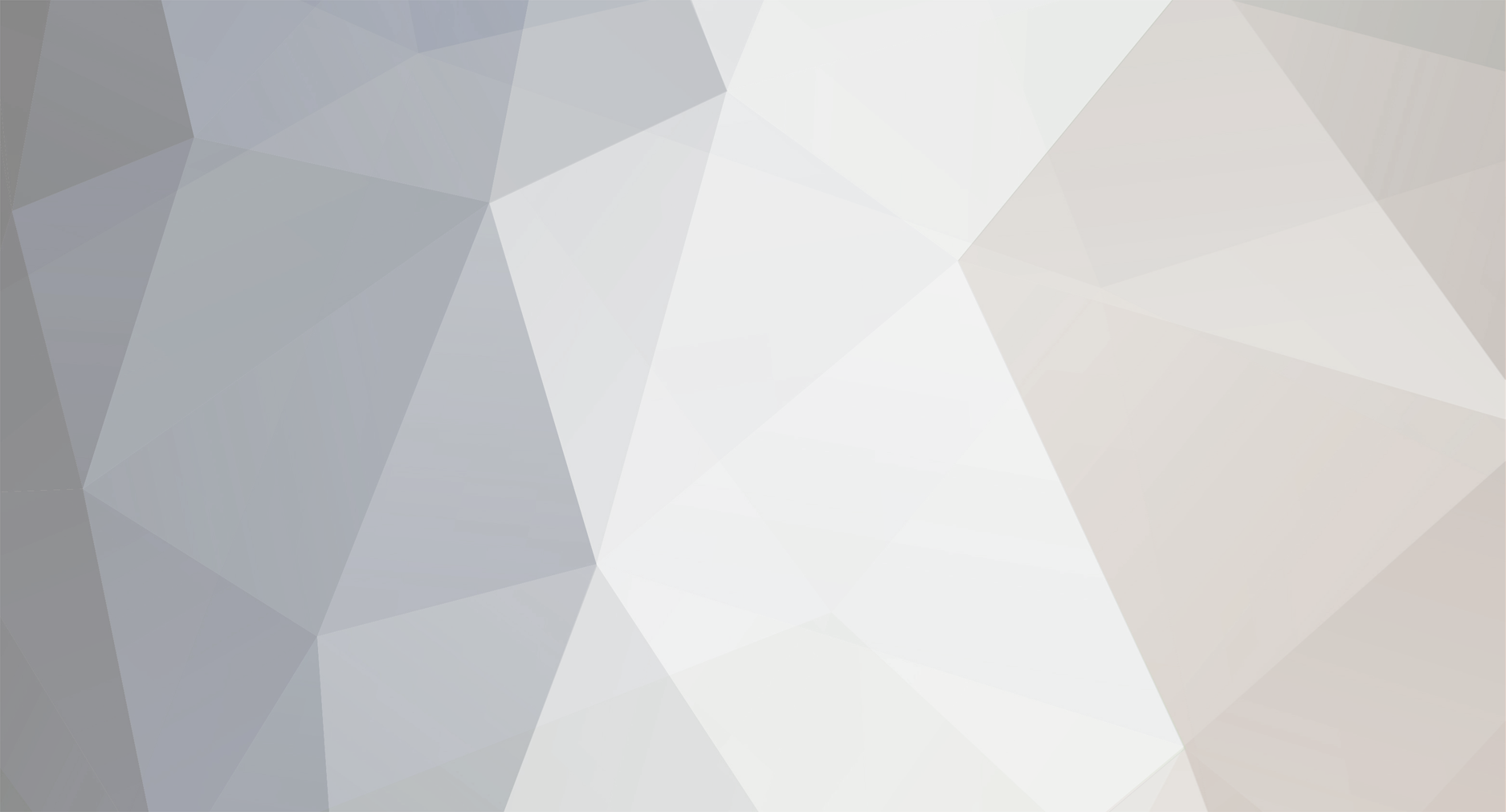
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Adding another table from same database to exsisting result?
GingerRobot replied to JackS's topic in PHP Coding Help
Aside from the fact that you've not told us what happens, what doesn't work etc, MySQL uses a single equals sign for equality, not a double. -
[SOLVED] How can I make this more efficient?
GingerRobot replied to Michdd's topic in PHP Coding Help
Use a multidimensional array? $monsters = array(); $monsters[0] = array('name'=>'spirit','times'=>18); $monsters[1] = array('name'=>'golden spirit','times'=>18); //etc //sample usage foreach($monsters as $monster){ echo $monster['name']; echo $monster['times']; } -
Any chance of you telling us what you mean by 'nothing is working'? Do you get a blank page? What actually happens? If you're getting a blank page, try adding the following two lines to the top of your script: ini_set('display_errors', 'On'); error_reporting(E_ALL);
-
A simple combination of file and array_sum should do the trick.
-
Did you check to make sure the address is passed in the URL? You should see it in the address bar. And how about some debugging on that query: $sql = "DELETE FROM image WHERE address = $address"; $query = mysql_query($sql) or trigger_error(mysql_error().'<br />Query was: '.$sql,E_USER_ERROR);
-
You can pass values in the URL like this: http://www.yourwebsite.com/somepage.php?foo=bar Or multiple items like this: http://www.yourwebsite.com/somepage.php?id=4&foo=bar To get the values in somepage.php, you'll use the $_GET superglobal: $foo = $_GET['foo']; $id = $_GET['id']; If you're using this data to query a database, you'll also need to sanitize your data to prevent SQL injections. You can do this by using the mysql_real_escape_string function.
-
Frankly, google it. You'll find a ton of articles out there on how to send mail with attachments and a further ton on creating excel or csv files. This thread may be helpful for the latter.
-
The syntax of the query is: DELETE FROM yourtable WHERE somefield = 'somevalue' You'll obviously need to use something to uniquely identify the row in the table that you want to delete and pass that in the URL to a script that performs the delete query.
-
Adding another table from same database to exsisting result?
GingerRobot replied to JackS's topic in PHP Coding Help
We'll need to see the code you're trying to use but isn't working in order to help. And we'll need a bit more of an explanation of what didn't work. And burnside: Use mysql_real_escape_string - that's what it's there for. -
Why not generate an excel/csv file and send an email with an attachment?
-
As gets said in every topic like this - if people actually had a good domain name idea, they'd go register it themselves. Good domains names can be hard to come by and so they can be worth a fair amount of money.
-
It looks like you were almost there - just going about accessing the array's value in the wrong way. The -> operator is used in object oriented programming to access properties or methods. You need to use the => operator, though in a slightly different way: $search = ";"; $replace = ""; if (count($tags) > 0) { foreach ($tags as $key => $tag) { echo "LOOP 1:"; echo $tag; $cleantag = str_replace($search, $replace, $tag); $tag = $cleantag; echo "LOOP 2:"; echo $tag; $tags[$key] = $cleantag; echo "LOOP 3:"; echo $tags[$key]; } } echo "<p>TAGS :</p>"; print_r($tags); Basically, the line foreach ($tags as $key => $tag) says that we're going to loop through each element of the $tags array and, for each element, we'll call the key of the array $key and the value of the array $tag. This thread may help.
-
Suggestion: Daily or Weekly PHP Coding
GingerRobot replied to premiso's topic in PHPFreaks.com Website Feedback
You could always extend it to deal with quadratics that have non-real solutions. -
You're imploding the data, which creates (in this case) a comma delimited string containing all of the values in the array. You then loop through the array echoing the string each time. You should either be looping or imploding. Not both.
-
Oh, you should note that the above code isn't very secure. The values of the checkbox aren't verified, so they may not be integers. If this is anywhere live, you should make sure all the values in that array are integers before you execute the query.
-
There's actually a much neater shortcut here. You don't need to loop through your array and execute a separate query for each item. Because you've given the checkboxes a value corresponding to the row's ID, you can do the following: $ids = implode(',',$_POST['archived']; $sql = "UPDATE news SET archived='1' WHERE id IN ($ids)"; mysql_query($sql) or trigger_error(mysql_error(),E_USER_ERROR); $sql = "UPDATE news SET archived='0' WHERE id NOT IN ($ids)"; mysql_query($sql) or trigger_error(mysql_error(),E_USER_ERROR); How does it work? Well, the implode function creates a string from the array using the delimiter to separate each element - in this case, a comma. The IN clause in the mysql query allows you to specify a comma delimited list of possible values. We perform a second query using NOT IN to take care of any items which have been un-archived. Also, notice how the query has been performed. In your previous code, you were suppressing the error with the @ symbol. I've explicitly made it so that errors are shown - it'll make it much easier to find any problems.
-
[SOLVED] passing variables help ... numbers and words ???
GingerRobot replied to imarockstar's topic in PHP Coding Help
There are (most likely) two problems. Firstly, As MatthewJ showed, strings must be delimited by quotes. Otherwise, PHP treats them as constants. Now, if PHP cannot find a constant of that name, it will assume it was supposed to be a string. But, depending on your error reporting level, you may get errors. It's certainly not good practice to rely on this kind of thing. Second, unless you have register_globals turned on (which you shouldn't), you need to retrieve the value from the $_GET array like so: $hai = $_GET['hai']; -
[SOLVED] Can someone help me figure this out?
GingerRobot replied to elentz's topic in PHP Coding Help
Did you try the last query i provided? -
[SOLVED] Can someone help me figure this out?
GingerRobot replied to elentz's topic in PHP Coding Help
1.) Please use the tags. You managed it before, why stop now? 2.) Again, confused. The code you posted doesn't include the DATE_FORMAT part of the query. What are you actually using? 3.) Not having worked with these classes i cant be entirely sure, but you might have to add aliases to your query: $pdf->Table("SELECT vtiger_invoice.invoice_no, vtiger_invoice.invoicestatus, vtiger_invoice.subject, vtiger_invoice.total, DATE_FORMAT(vtiger_invoice.invoicedate,'%m/%d/%Y') AS invoicedate, DATE_FORMAT(vtiger_invoicecf.cf_674,'%m/%d/%Y') AS cf_674 FROM vtiger_invoice Inner Join vtiger_invoicecf ON vtiger_invoicecf.invoiceid = vtiger_invoice.invoiceid WHERE vtiger_invoice.accountid = 4103 ORDER BY vtiger_invoice.accountid ASC",$prop); -
[SOLVED] Can someone help me figure this out?
GingerRobot replied to elentz's topic in PHP Coding Help
It looks from that like you've not put any quotes around the format string. In the code i pasted, i did miss a comma, but it looks like you added that in. This should work: $pdf->Table("SELECT vtiger_invoice.invoice_no, vtiger_invoice.invoicestatus, vtiger_invoice.subject, vtiger_invoice.total, DATE_FORMAT(vtiger_invoice.invoicedate,'%m/%d/%Y'), DATE_FORMAT(vtiger_invoicecf.cf_674,'%m/%d/%Y') FROM vtiger_invoice Inner Join vtiger_invoicecf ON vtiger_invoicecf.invoiceid = vtiger_invoice.invoiceid WHERE vtiger_invoice.accountid = 4103 ORDER BY vtiger_invoice.accountid ASC",$prop); If it doesn't can you paste the exact code you're now using? -
Indeed. The gd library (or, more specifically, the PHP implementation of it) doesn't support animated gifs. I believe imagemagick does, though i've not used it.
-
Problem in using the CURL to get html source code of a url
GingerRobot replied to ammu412's topic in PHP Coding Help
An intermittent fault is more than likely caused by a fault at the receiving site (something which can happen regardless of your method of making the request) and is something that should be checked for and dealt with (again, regardless of your method). By that logic, everything in PHP would be hard to use. Think of a potential problem and there'll be a thread on it. Like cURL you mean? Don't get me wrong, i'm not saying you have to use cURL - i'm just stating that there are many methods of achieving the same thing. When it comes down to it, there's probably not a lot of difference. However, you can't just say that, because you had problems, x method is rubbish.