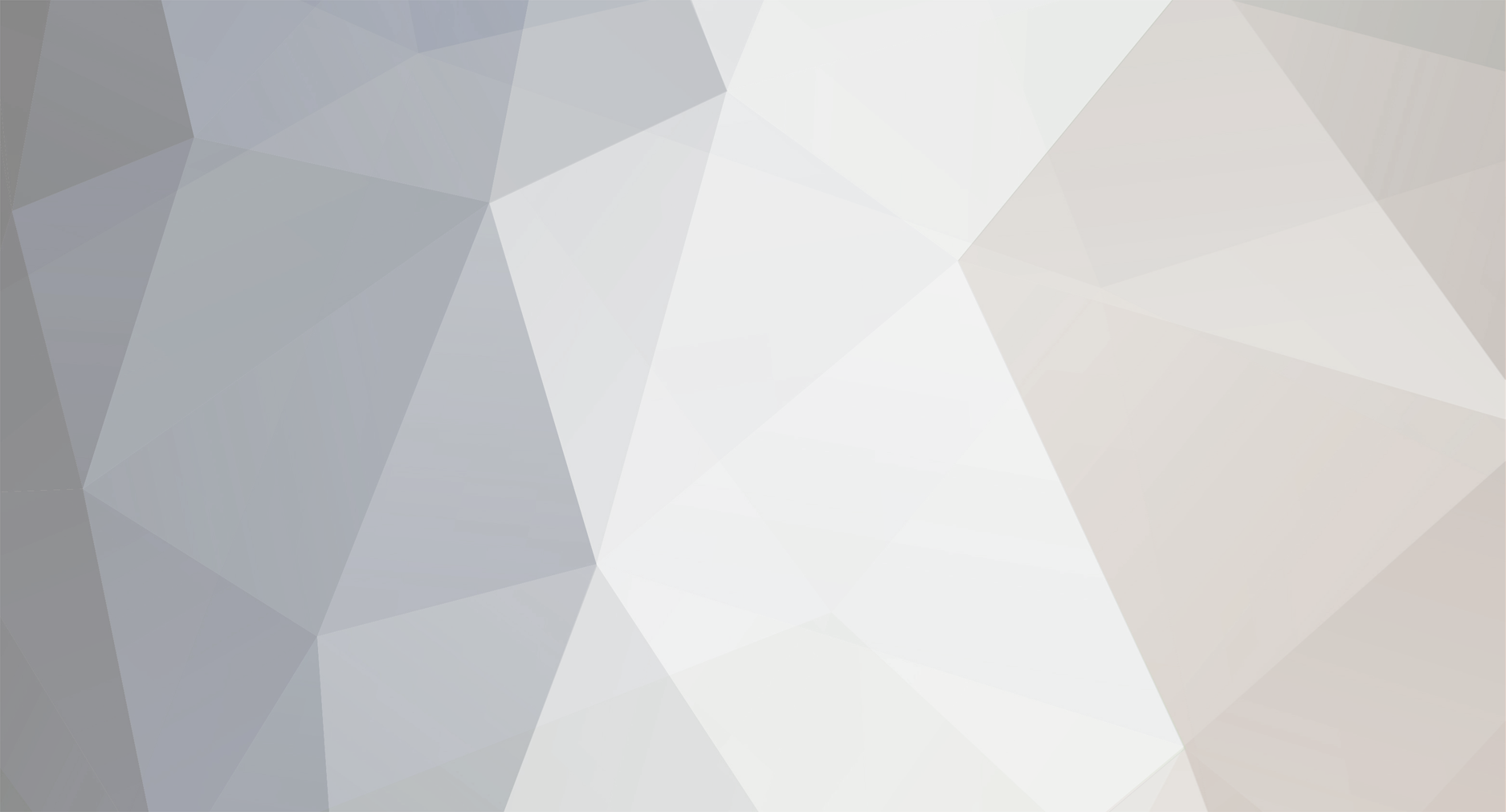
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
[SOLVED] Sorting offices and regions into 3D Array ?
GingerRobot replied to scotchegg78's topic in PHP Coding Help
Hmm, i thought the table prefixes would be preserved in the selected column names, but apparently not. Looks like you'll have to add all of the fields form one of the tables with a prefix manually (i've started this with the office table, adding o before each field name). <?php $array = array(); $sql = "SELECT o.address1 as oaddress1,o.address2 as oaddress2, o.officeID as oofficeID, r.* FROM cr_office as o,cr_region as r WHERE o.regionID=r.regionID ORDER BY r.regionID"; //add tables from office and add a prefix of o to each field name $result = mysql_query($sql) or die(mysql_error()); $lastid = ''; while($row = mysql_fetch_assoc($result)){ if($lastid != $row['regionID']){ $array[$row['regionID']] = array('address1'=>$row['address1'],'address2'=>$row['address2']); //fill in with all other fields from region table - note: no 'r.' required $lastid = $row['regionID']; } $array[$row['regionID']]['offices'][$row['oofficeID']] = array('oaddress1'=>$row['oaddress1'],'oaddress2'=>$row['oaddress2']); //fill in with all other fields from office table (e.g. o.*); } echo '<pre>'.print_r($array,1).'</pre>'; ?> -
How about posting some sample code?
-
[SOLVED] Sorting offices and regions into 3D Array ?
GingerRobot replied to scotchegg78's topic in PHP Coding Help
Yeah, it's more complicated. This *should* do the trick, untested though: <?php $array = array(); $sql = "SELECT o.*,r.* FROM cr_office as o,cr_region as r WHERE o.regionID=r.regionID ORDER BY o.regionID"; $result = mysql_query($sql) or die(mysql_error()); $lastid = ''; while($row = mysql_fetch_assoc($resut)){ if($lastid != $row['r.regionID']){ $array['r.regionid'] = array('r.address1'=>$row['r.address1'],'r.address2'=>$row['r.address2']); //fill in with all other fields from region table (e.g. r.*); $lastid = $row['r.regionID']; } $array['r.regionid']['offices'][$row['o.officeID']] = array('o.address1'=>$row['o.address1'],'o.address2'=>$row['o.address2']); //fill in with all other fields from office table (e.g. o.*); } echo '<pre>'.print_r($array,1).'</pre>'; ?> -
array_intersect()? Looking in the manual at the function you know about (e.g. http://www.php.net/array_diff) will help you find similar functions by looking under the section 'See Also'.
-
[SOLVED] Sorting offices and regions into 3D Array ?
GingerRobot replied to scotchegg78's topic in PHP Coding Help
I think i know what you want, if so its actually pretty simple: <?php $array = array(); $sql = "SELECT * FROM cr_office"; $result = mysql_query($sql); while($row = mysql_fetch_assoc($result)){ $array[$row['regionID']][] = $row; } echo '<pre>'.print_r($array,1).'</pre>'; ?> -
1.) Dont surpress the errors if you're looking for them. 2.) The formatting has already been done for you. Try this: $sql = "SELECT *, DATE_FORMAT(timeStampStart,'%b-%Y') as x FROM dental_holidayrequest GROUP BY x ORDER BY x"; $result = mysql_query($sql) or die(mysql_error()); while($row = mysql_fetch_assoc($result)){ echo $row['x'].'<br />'; }
-
I would imagine the field type you're trying to insert into is incorrect. Try changing it to a DATETIME field.
-
If you would like someone to do this for you, head over to the freelance section. This part of the forum is for helping people with what they are trying to do. If you'd like to do it yourself, i suggest you google for pagination tutorials to start with...there are hundreds of them out there.
-
Did you want something like this? SELECT *,DATE_FORMAT(dental_holidayrequest,%b-%Y) as x FROM yourtable GROUP BY x ORDER BY x Im assuming that dental_holidayrequest was the field with the date in. What field type is it?
-
Minimium posts on some boards
GingerRobot replied to The Little Guy's topic in PHPFreaks.com Website Feedback
So don't bother? There will naturally be people who use that kind of thing as a way of advertising their site. And, frankly, good luck to them. It's your choice wether or not you visit a site and take a look round. Personally, i very rarely do for the reasons you mentioned and i'd be far more likely to take the time if it were for someone who i know contributes to the site. -
No, i was actually just suggesting (as i posted) this: if (preg_match("|\.gif$|i", $filename)){ I notice you would have had a parse error in the above. I wonder if you have the display of errors turned off. Add this: error_reporting(E_ALL); ini_set('display_errors','On'); To the top of your code. I also notice you're not setting the correct header. You'll need to tell the browser what it's expecting. Thus the code is: <?php error_reporting(E_ALL); ini_set('display_errors','On'); resize("./", "http://images.wsdot.wa.gov/nwflow/flowmaps/sysvert.gif", "538", "./"); function resize($cur_dir, $cur_file, $newwidth, $output_dir) { $dir_name = $cur_dir; $olddir = getcwd(); $dir = opendir($dir_name); $filename = $cur_file; if (preg_match("|\.gif$|i", $filename)) { $format = 'image/gif'; } if($format!='') { list($width, $height) = getimagesize($filename); $newheight=$height*$newwidth/$width; switch($format) { case 'image/gif'; $source = imagecreatefromgif($filename); break; } $dimg = imagecreatetruecolor(640,538); imagealphablending($dimg, false); $source = imagecreatefromgif($filename); imagecopyresized($dimg, $source, 0,0,0,438, $newwidth, $newheight, $width, $height); header("Content-type: image/gif"); imagegif($dimg); } } ?>
-
Whats this line then? resize("./", "http://images.wsdot.wa.gov/nwflow/flowmaps/sysvert.gif", "538", "./"); As for the problem: 1.) Remove the error surpressors (@) -- how do you hope to find errors if you're surpressing them? 2.) You're creating from a gif (imagecreatefromgif) yet appear to want support for other image types. 3.) Your regex is going to be problematic. Taking your regex for png, for example: "/.jpg/i" This will match any string with the text jpg appearing after any character. That is, both of the following would match: image.jpg, ajpg.png. The . character is a special character, meaning any character. You should escape is and make the regex check only the end of the string: if(preg_match("/\.jpg$/i", $filename)) And finally, you should get in the habit of posting your updated code. Its much easier for people to follow.
-
Of course: <?php if($condition===true){ header("location:true.php"); }else{ header("location:false.php"); } ?> You should note that you cannot send any output to the browser prior to changing location like this.
-
1.) I don't think you can -- you're limited by the upload speed of the user, which, for most, isn't that great. 2.) I think you could make it more dynamic. I notice the background is static -- perhaps have that change a little. You could also experiement with using varying fonts and font colours. Or you could try having the letters positioned in something other than a straight line. Basically, the less predictable (whilst still being readable) the better really. p.s. Welcome to the forums!
-
Making an image shop up on a dynamic image
GingerRobot replied to shadysaiyan's topic in PHP Coding Help
Did you even read the the above liink? It allows you to copy one image onto another. -
Making an image shop up on a dynamic image
GingerRobot replied to shadysaiyan's topic in PHP Coding Help
imagecopymerge()? -
Ok, well there lies your problem. The library isn't enabled by default. http://uk.php.net/manual/en/zip.installation.php
-
Yeah, mine should have been: <?php $sql = "SELECT sku FROM admin WHERE sku BETWEEEN 1000 and 9999"; $result = mysql_query($sql) or die(mysql_error()); $taken = array(); $possible = range(1000,9999); while($row=mysql_fetch_row($result)){ $taken[] = $row[0]; } $unused = array_diff($possible,$taken); echo '<pre>'.print_r($unused,1).'</pre>'; ?>
-
Hmm, can't think of an easy way to do it all though mysql, but you could do this: <?php $sql = "SELECT sku FROM admin WHERE sku BETWEEEN 1000 and 9999"; $result = mysql_query($sql) or die(mysql_error()); $taken = array(); $possible = range(1000,9999); while($row=mysql_fetch_row($result)){ $taken[] = $row[0]; } $unused = array_diff($range,$possible); echo '<pre>'.print_r($unused,1).'</pre>'; ?>
-
First step of debugging -- echo the problem part: if(!in_array($_FILES[$name]['type'], $allowed_ext_array)) { $error_messages .= 'Sorry, you are only allowed to upload '.$names.' file types'.'<br />'; echo $_FILES[$name]['type']; }
-
Well, the first thing you'd need are the latitudes and longitudes of the four corners of your map. Without that, you cant really do anything -- you need a reference point to be able to plot the others. I suppose you could go for a rough estimate of the latitude and longitude of, say, central London and then estimate it's position on the map and go from there. You'll then need to convert your latitudes and longitudes to positions (e.g. pixels) on the map by using a scale. Then you'd need the gd library functions (http://www.php.net/gd) to be able to put something on top of the image. In short, its not that easy
-
Perhaps you have the display_errors off? Add this: ini_set('display_errors','On'); To the top of your script.
-
See this post
-
Yes, sorry, it was supposed to be $result. As for the . - that joins table and field names. For example, friends.friendname means the friendname field in the friends table. Whilst not strictly necessary in this query (since friendname doesn't appear in the other table), I find it best to always do it this way since it makes the query clearer.