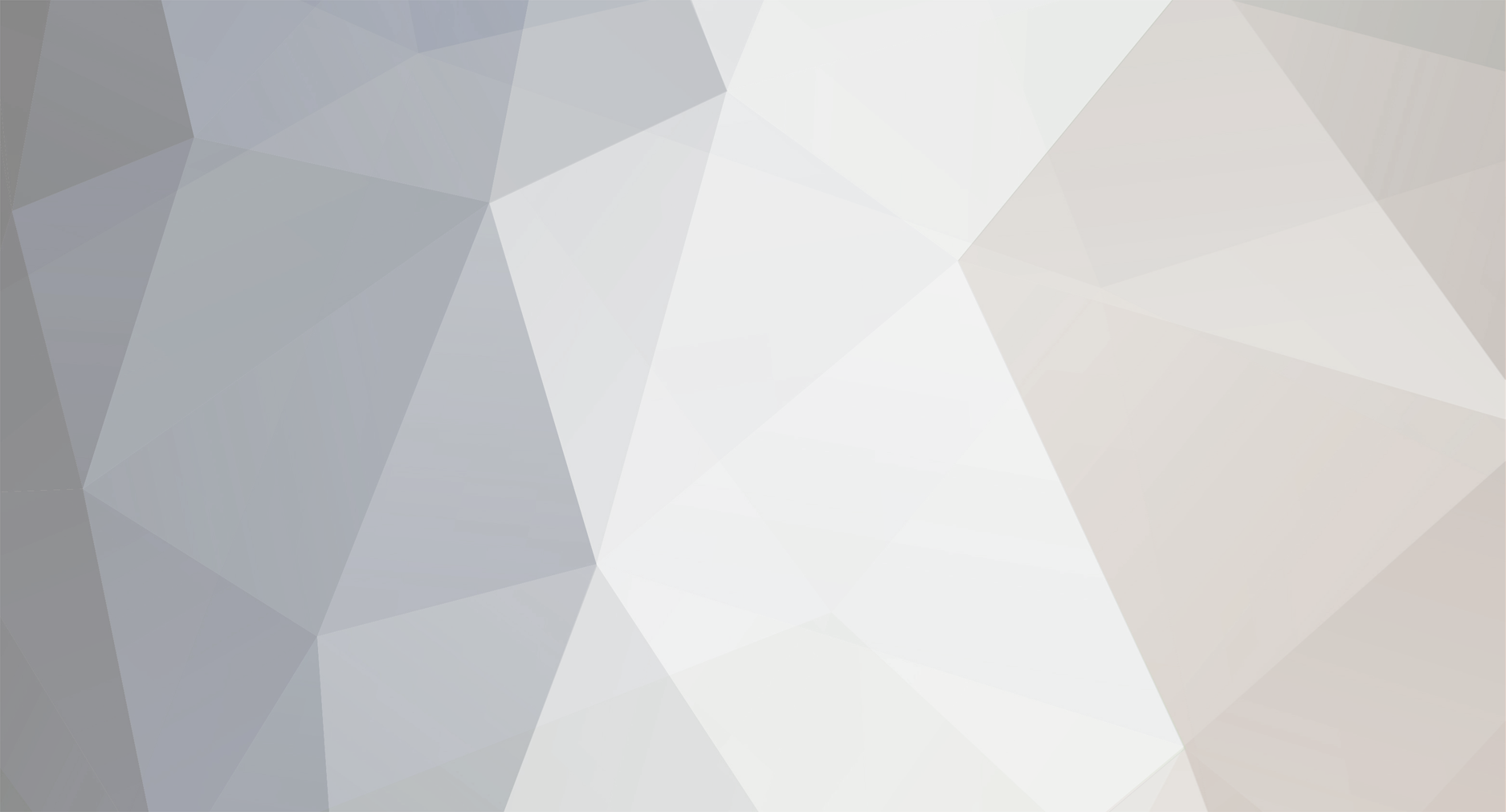
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
Never use .inc -- ever. Unless you have your sever set up correctly, the source code would be displayed if someone navigated directly to these pages. And even then, you leave yourself open to problems in the future should the server set up change. It's more personal preference with regard to .inc.php or .php -- i personally would use .php, though store the files in a separate includes folder. Seems less messy, but that's just opinion.
-
I would say it depends on what data you are getting from the user. Are you getting the date they worked too? Maybe if we saw your form it would help.
-
Why are we selecting all the rows from a table and then looking for a match? Use a database the way it's designed to be used: $sql = "SELECT COUNT(*) FROM badisp WHERE isp='$hostname'"; $result = mysql_query($sql) or die(mysql_error()); if(mysql_result($result,0) > 0){ echo 'invalid login'; } ?> It's a notice.
-
No worries. It's usually the simple things that you don't spot. Can you mark the topic as solved if you're all done?
-
Try: <?php class someClass { private $array = array(); function __construct() { $this->array[1] = "hello"; $this->array[2] = "goodbye"; } function getGreeting ($i) { return $this->array[$i]; } } $greeting = new someClass(); echo $greeting->getGreeting(1); ?> You always need the to use $this-> when using class attributes.
-
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Missing closing bracket: $sql = "INSERT INTO " . DBPREFIX . "users (`Username` , `Password`, `date_registered`, `Email`, `Random_key`,`image`) VALUES (" . $db->qstr ( $_POST['username'] ) . ", " . $db->qstr ( md5 ( $_POST['password'] ) ).", '" . time () . "', " . $db->qstr ( $_POST['email'] ) . ", '" . random_string ( 'alnum', 32 ) . "', '0.jpg')"; $result = mysql_db_query($database,$sql,$connection) or die(mysql_error().'<br />Query was:'.$sql) -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Any chance you can modify your query function so that it also echoes the query? Like i said previously, it's much easier to spot an error that way. -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Think i see it. Try: $query = $db->query ( "INSERT INTO " . DBPREFIX . "users (`Username` , `Password`, `date_registered`, `Email`, `Random_key`,`image`) VALUES (" . $db->qstr ( $_POST['username'] ) . ", " . $db->qstr ( md5 ( $_POST['password'] ) ).", '" . time () . "', " . $db->qstr ( $_POST['email'] ) . ", '" . random_string ( 'alnum', 32 ) . "', '0.jpg'" ); -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Instead of just executing the above query, can you put it in a variable first and echo it? It's just easier to spot an error when you can see exactly what's being executed. -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Looks like you closed off the query too soon. Try: <?php $query = $db->query ( "INSERT INTO " . DBPREFIX . "users (`Username` , `Password`, `date_registered`, `Email`, `Random_key`,`image`) VALUES (" . $db->qstr ( $_POST['username'] ) . ", " . $db->qstr ( md5 ( $_POST['password'] ) ).", '" . time () . "', " . $db->qstr ( $_POST['email'] ) . ", '" . random_string ( 'alnum', 32 ) . ", '0.jpg'" ); ?> -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Sorry, i never really read your SQL code properly. Must be tired. You need to use an UPDATE statement when changing data in a row that already exists. INSERT is used to create a new row. Try: $sql = "UPDATE `users` SET images = '".$id.".".$file_ext."' WHERE ID=$id"; Im assuming that $id is always an integer. Otherwise you'll need quotes around the second $id. Im also assuming that you're using the field name images rather than image. -
[SOLVED] How to change the value of a variable..???
GingerRobot replied to RRVARMA's topic in PHP Coding Help
Well, the problem is that the the changing of the $start2 variable occurs after the form has been echoed to the browser -- therefore this value always stays the same. You would probably be better off passing all of the variables needed in the URL, however. It would then work for those with javascript turned off too. -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Did you ever add the field users.image and put anything in it? The above code works fine for me with the structure you gave. As for the UPDATE: $sql = "INSERT INTO `users` (images) VALUES ('".$id.$file_ext."') WHERE ID = '$id'"; -
Refresh if the user hasn't clicked on an input field
GingerRobot replied to Vigilant Psyche's topic in PHP Coding Help
Yes, it could be done. My javsacript is a touch rusty, but i think it would require a bit of work. As far as i'm aware, there's no simple way of checking if an element is in focus. Instead, you would have to set a variable to true using the onFocus event and then set it to false with onBlur. You would then need to use the settimeout() function every 7 seconds to call another function which would check the above value and reload only when required. My recommendation: switch to loading the chat content with AJAX. It'd be less resource intensive, and much nicer for the user. If you google for AJAX chat rooms im sure you'll find some examples to follow. -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Yes, i understand what you mean about the account now. Are you now using the above suggestion for storing the image? If so, the following should work for you: <?php $sql = "SELECT t.* ,u.image FROM `tagboard` AS t, users AS u WHERE t.account = '$user2' AND t.nick = u.Username ORDER BY t.id DESC LIMIT 10"; $result = mysql_db_query($database,$sql,$connection) or die(mysql_error().'<br />Query was:'.$sql); while($row = mysql_fetch_assoc($result)){ $nick = $row["nick"]; $url = $row["url"]; $message = $row["message"]; $datetime = $row["datetime"]; $senton= date("M jS, Y \a\\t h:i A T", $datetime); $img = $row['image']; //echo out as required echo 'Username:'.$nick.'<br />'; echo 'URL:'.$urk.'<br />'; echo 'Message:'.$message.'<br />'; echo 'Time:'.$senton.'<br />'; echo 'Img: <img src="http://www.r.com/members/images/mini/'.$img.'" /><br />'; echo "<hr />\n"; } ?> As for when you're uploading your image, all you need to do is store the image name and extension in users.image. The same goes for when you're displaying it; just retrieve that value. Finally, i should probably add that there are certain oddities in your database structure -- for example, it would be better to store a user id, rather than a user's name, in the tagboard table to identify the user. It is also strange that you have a character limit on the tagboard.nick field of 16, whilst tagboard.account has a limit of 99 and users.Username has a limit of 255, yet all of these are storing the same piece of data! I think there are others, but perhaps that's for a different day. -
Get a users IP address ( for banning).
GingerRobot replied to Vigilant Psyche's topic in PHP Coding Help
I would suggest a certain amount of caution with this approach. See this thread for a bit of a discussion on the problems of retrieving a true IP. -
Indeed. But you can use the imagegrabwindow function to take a screenshot of what's in IE, for example. So it should be possible take a screenshot of the particular page the user is browsing.
-
You'll have to take them out -- files names shoudn't have slashes in them.
-
Very well said fenway, couldn't agree more.
-
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
I'm not even sure why you have a separate field for the extension - why not just store the image name and extension together? Run this query to add a new field to your users table: ALTER TABLE `users` ADD `image` VARCHAR( 255 ) NOT NULL Then run this query to make the image field equal to the user's id plus the relevant extension: UPDATE users SET image = CONCAT(ID,'.',(SELECT ext FROM user_images WHERE user_images.user_id=users.ID)) The above queries only need be run once. I should probably add that if you were wanting to let users add more than one image, you would be correct in thinking you need a second table. However, if you're just wanting to allow users to have an avatar (or similar) then you might as well stick with the one table. And lastly, im still unsure about this account field. Whats the difference between account and nick? -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Ok, and what is the point of the account fiend in the tagboard table? What does it store? And while we're on the subject, why are you storing the extension for an image in a separate table? Is a user only ever allowed to have one picture? If so, why dont you just store the path to the image in the users table? -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
Right, i dont think we're getting anywhere are we? Perhaps its time you posted up your database structure including relevant tables, field names and the links between field names across your tables. -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
This is always the trouble with trying to write queries for a database you didn't created/don't use. Try: <?php $sql = "SELECT t.*,user_images.ext as ext,users.ID as pid FROM $tablname as t,users,user_images WHERE t.nick=users.Username AND users.ID = user_images.user_id ORDER BY t.id DESC LIMIT 10"; $result = mysql_db_query($database,$sql,$connection) or die(mysql_error().'<br />Query was:'.$sql); while($row = mysql_fetch_assoc($result)){ $nick = $row["nick"]; $url = $row["url"]; $message = $row["message"]; $datetime = $row["datetime"]; $senton= date("M jS, Y \a\\t h:i A T", $datetime); $id = $row['pid']; $ext = $row['ext']; //echo out as required echo 'Username:'.$nick.'<br />'; echo 'URL:'.$urk.'<br />'; echo 'Message:'.$message.'<br />'; echo 'Time:'.$senton.'<br />'; echo 'Img: <img src="http://www.r.com/members/images/mini/'.$id.'.'.$ext.'" /><br />'; echo "<hr />\n"; } ?> -
[SOLVED] showin image of user who posted a message
GingerRobot replied to runnerjp's topic in PHP Coding Help
What is the purpose of t.account="$user2'? It was in your original query, so i left it there initially. However, i have to wonder why it was there in the first place. You also seem to have removed an alias somewhere along the line. Try: $sql = "SELECT t.*,user_images.ext as ext,users.ID as pid FROM $tablname as t,users,user_images WHERE t.nick=users.nick AND users.ID = user_images.user_id ORDER BY t.id DESC LIMIT 10"; -
Looking for a clean approach to my solution
GingerRobot replied to jessadactyl's topic in PHP Coding Help
Unless i've misunderstood, it sounds to me like you just need to re-organise your script a touch. The creating of a new id for a new template should occur before you check the URL for a given ID. You should then only check the URL if a new id has not been created. If it hasn't use the ID in the URL. If it has, use the created ID instead.