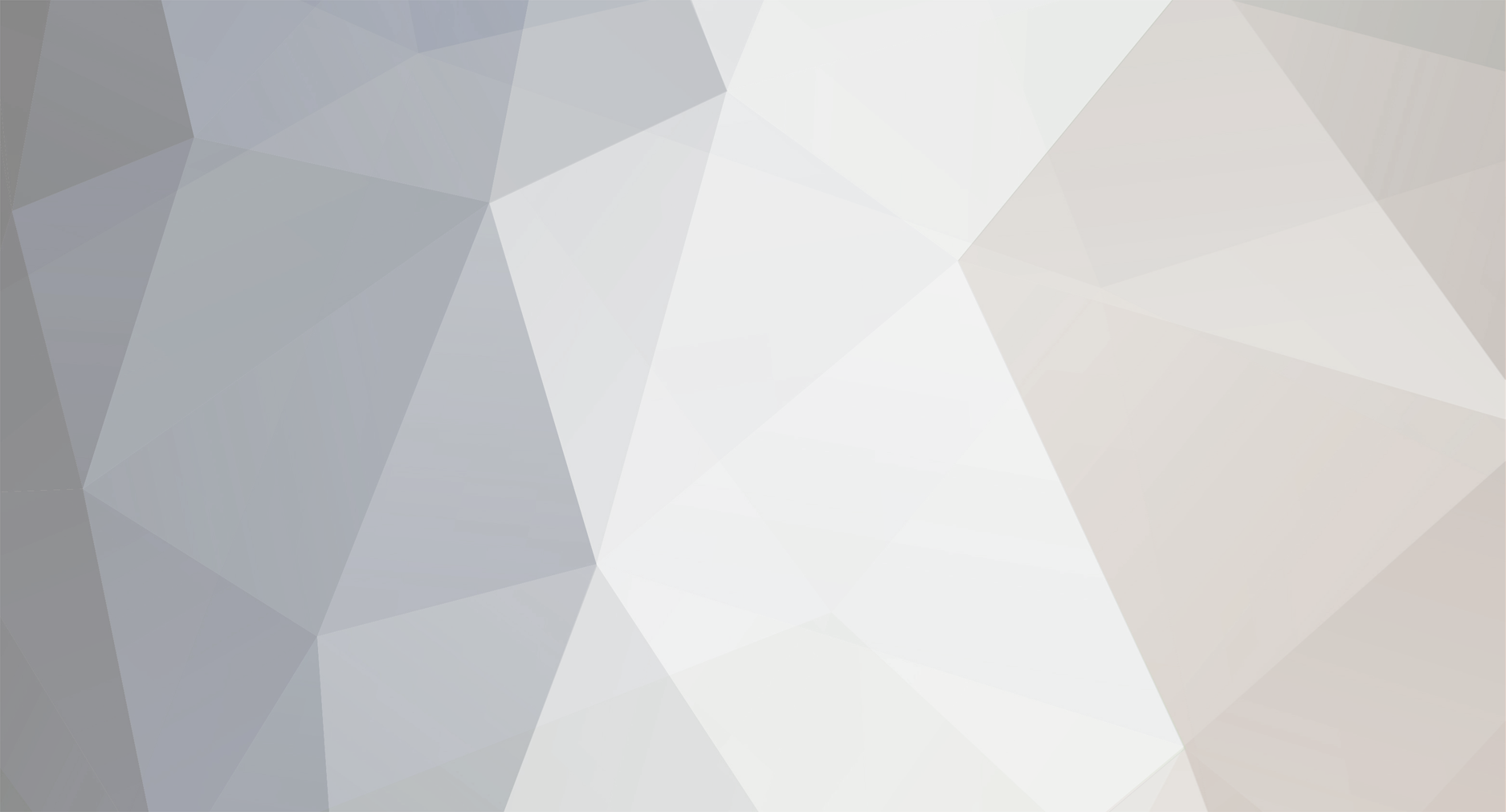
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
See the setlocale() function. You should be able to change the output with that.
-
1.) Whenever you have a problem with a query, you need to debug it. Do something like: <?php $query = mysql_query("select total from tblinquiries where course='$course' and inq_date='4/21/2008'"); //becomes $sql = "select total from tblinquiries where course='$course' and inq_date='4/21/2008'"; $query = mysql_query($sql) or die(mysql_error()); echo '<br />Query was: '.$sql; ?> 2.) Your approach will be slow, since you're performing a lot of queries. You'd be better of using a join/subquery to select data from two tables. If you post up what your trying to achieve (e.g. output) and your table structure, we might be able to help with that. 3.) When you post code, try and remember to put it inside tags
-
Well, you could quite easily get firefox to start when he logs in by adding it to the 'startup' folder. If you add in a shortcut to it in C:\Documents and Settings\his-user-name\Start Menu\Programs then it will launch when he logs in. Now, i'm not sure if there's an easy way of only allowing this program to be run - which sounds like the kind of thing your after. It might be possible to deliberately mess with the user permissions and take control of most of his documents and settings folder as the administrator, only allowing access to a few things like firefox. Not sure how well that would work though. As for the browsing, i think you'll need third party software. Perhaps there's a decent firefox extension. All you need is something with a whitelist. You dont need a maintained black list, which im guessing you'd probably have to pay for. You just want something where you can say you only want him to have access to these 3/4 websites.
-
Take a look at the date() function. It will show you how to achieve whatever output you're looking for. Basically, you need to change the 'l' for whatever you want.
-
I dont think it worked particularly well either. As has been mentioned, its pretty difficult to draw a line to define "easy" and "advanced" PHP questions. I think you also get a lot of people who would jump straight to the advanced section to post their basic question because they believe (and wrongly) that the level of help will be better there.
-
Whenever you're having problems with a query, you should add an or die statement and echo the query. That is, this: $check_user = mysql_query("SELECT username FROM accounts WHERE username IN($user)"); Becomes: $sql = "SELECT username FROM accounts WHERE username IN($user)"; $check_user = mysql_query($sql) or die(mysql_error()); echo '<br />Query was:'.$sql; Now, when you do that, i suspect you'll find that $user is undefined, since your form has this: Username:<input type=text size=16 maxlength=32 name=profile align=right> Im guessing that should be: Username:<input type=text size=16 maxlength=32 name=user align=right>
-
If you've installed wamp then you've installed mySQL - its what the 'm' stands for
-
That'd be slow too. =/ Much slower than a database. @_@ I dont think thats necessarily true. Have you seen the amount of use SQLite gets? While i have no experience with it, I understand that given the right situation, it works fantastically well. Also, i think working with text files can be a great learning tool. If you've only started with programming recently, then it can help your understanding of arrays a lot. That said, i'd still use mySQL.
-
It wouldn't THAT bad. If you made use of the file() function to read the textfile into an array, it would be straightforward to keep track of which lines you wanted deleting. But yes, a database would be easier.
-
If you were to use an array, you could do this: <?php $v[1] = 1; $v[2] = 1; $v[3] = 2; if(count($v) != count(array_unique($v))){ echo 'duplicates!'; }else{ echo 'fine'; } ?>
-
How secure are session variables for tracking logged in users?
GingerRobot replied to Ritchie-T's topic in PHP Coding Help
Its quite well documented : http://www.outsidethecode.com/faq/aol_ip.aspx http://www.usenet-forums.com/php-general/358507-php-re-about-session-cookies.html http://www.sitemeter.com/?a=help&area=compare Edit: Oh, and from AOL themselves: -
It's possible you could have the wrong privileges - though you would get an error message to this effect if that was the case (assuming you are debugging). So yes, we'll need to see your code.
-
How secure are session variables for tracking logged in users?
GingerRobot replied to Ritchie-T's topic in PHP Coding Help
Unfortunately not true. Some people will have a slightly different IP for each request (e.g. AOL users) -
Try something like this: <?php function count_types($area,$types){ $num_rows = array(); $sql = "SELECT COUNT(*),type FROM property_info WHERE location='$area' AND type IN(".implode($types).") GROUP BY type"; $result = mysql_query($sql) or die(mysql_error()); while(list($count,$type) = mysql_fetch_row($result)){ $num_rows[$type] = $count; } return $num_rows; } $area = 'Some area'; $types = array(1,2,3,4);//specify the types in an array. If you're wanting ALL types in your query, delete this, delete the parameter and remove the AND type IN(...) part of the WHERE clause from the query. echo '<pre>'.count_types($area,$types).'</pre>'; ?>
-
Tibberous was pretty much there - just you dont need the if keyword with the ternary operator, and that we should be checking if the hour is less than 18, not 14: echo ((date('G') < 18) ? date('l') : date('l', time()+60*60*24));
-
You're missing a closing bracket after your date function. A prime example why it's better to split this sort of thing up onto multiple lines. You would have seen it if you did - it was the only way i could find it: <?php echo '<td><font color="silver">'.$gd["lname"].'</font></td><td><font color="silver">'.date('m/d/y g:i A', strtotime($gd["dtime"])).'</td><td><a href="udt.php?user='.$uid.'&lid='.$gd['lname'].'&sid='.$gd['sid'].'">Update '.$gd['lname'].' Now></a></td>';}?>
-
Perhaps its just me, but i've still no idea what the problem is. I've tried to follow what you've said, but havn't been able to. What does the above code currently do? Are there any errors? What is it supposed to do? See if you can answer those questions clearly and concisely and perhaps we'll get somewhere.
-
1.) drop the . from the possible extensions: $allowedExt = array('mp3','wma','wav','mpeg'); 2.) Add in a direction to the $newname: $newname = 'songs/'.substr($filename, 0, $dotPos) . '-' . rand(10000, 99999) . $ext;
-
Indeed. Forcing the user to put their own date separators in isn't great in my opinion. You should use three different text boxes and place the slashes/dashes yourself. As for validation, most people would use javascript and PHP. Javascript is user friendly, since a user will be notified of mistakes without having to submit the page and wait for a reload. However, you need PHP to repeat the checks server side, since a user may have javascript turned off.
-
[SOLVED] Check if user enters characters
GingerRobot replied to allistera's topic in PHP Coding Help
I certainly wouldn't use that regular expression. 1.) It would block punctuation 2.) Regex is (relatively) slow -
[SOLVED] Check if user enters characters
GingerRobot replied to allistera's topic in PHP Coding Help
How about: if(empty(trim($comment)){ //comment empty }else{ //process comment } -
Try: SELECT * FROM tbl WHERE id > 500 ORDER BY id ASC LIMIT 1
-
If you just append a random 6 digits there is a small chance that a file will already exist with the same name. Either check the file doesn't exist before you try and move it, or (better) append something which will definitely not be repeated - such as a unix timestamp.
-
Either do something like: $row = mysql_fetch_assoc($sql_doublecheck); $first = $row['first']; Or: extract(mysql_fetch_assoc($sql_doublecheck)); echo $first;//assuming your field is called first See the manual for what happens when you use extract and there is already a variable with the field name.
-
Yeah, that's an example of numerical integration.