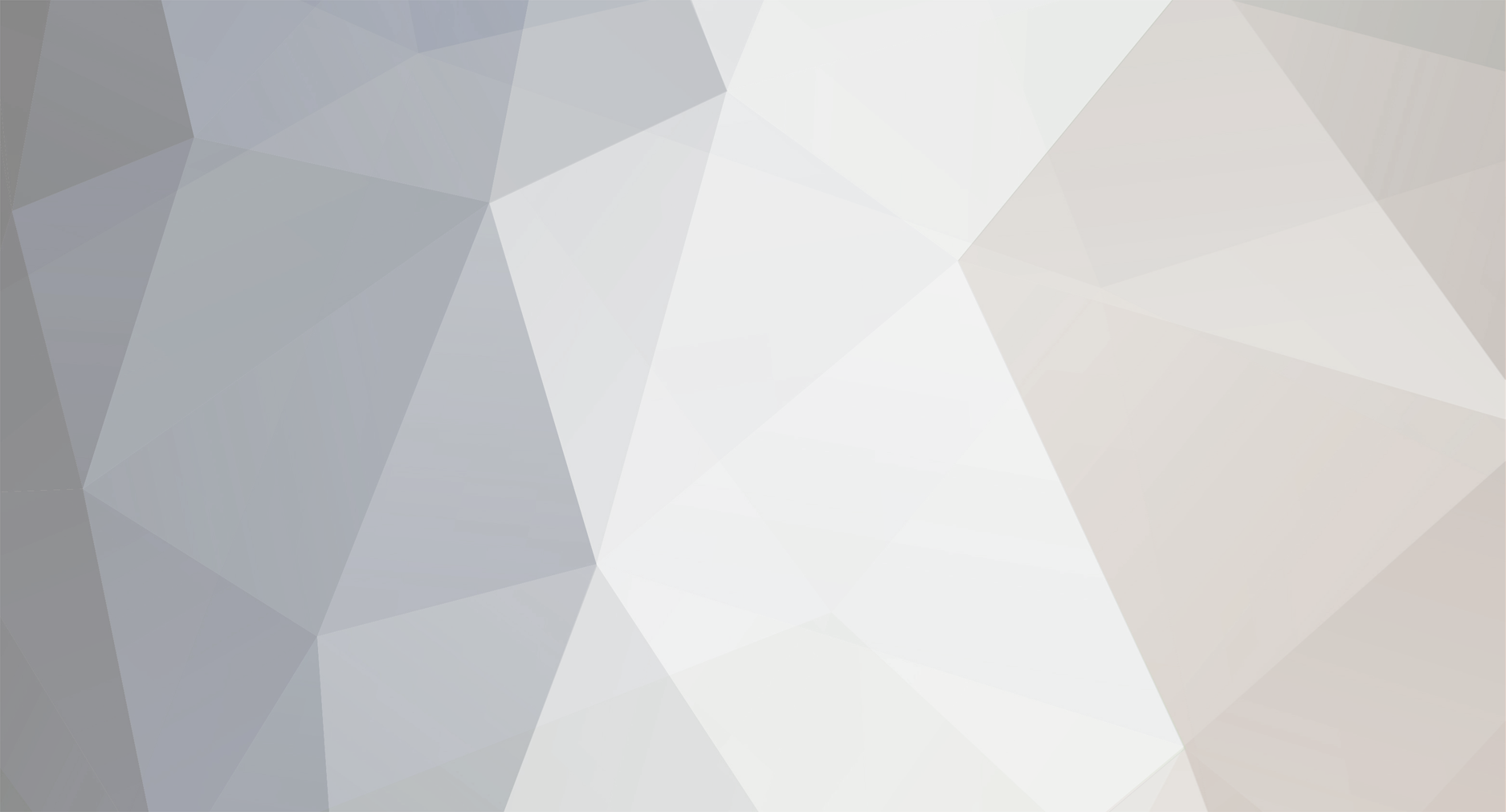
GingerRobot
Staff Alumni-
Posts
4,082 -
Joined
-
Last visited
Everything posted by GingerRobot
-
How about: <?php $url='http://youtube.com/watch?v=w8etbQnvZm0'; list($junk,$v) = explode('v=',$url); $im = @imagecreatefromjpeg('http://i.ytimg.com/vi/'.$v.'/default.jpg'); if($im){ imagejpeg($im,'images/thumbnails/'.$v.'.jpg'); echo 'Image saved to: images/thumbnails/'.$v.'.jpg'; }else{ echo 'Image could not be loaded'; } ?>
-
You ought to consider using a separate table in your database for your images, rather than having all these different fields in the same table. Try reading up on database normalization. Maybe this post will help you start: http://www.phpfreaks.com/forums/index.php/topic,126097.0.html
-
The problem is that you're trying to send post data, but as far as i can see you dont actually have a form. You need a form and you need to replace your button with the javascript onclick event with a submit button. To pass on the id, either use a hidden field or add it to the url of the form's action.
-
I suspect $_POST['pid'] is empty. Try this to check: $sql = "UPDATE reff SET notes = '" . $_POST['mytitle'] . "'WHERE pid='" . $_POST['pid'] . "'"; mysql_query($sql) or die(mysql_error()); echo '<br />Query was:'.$sql;
-
[SOLVED] Script crashes after move_uploaded_file
GingerRobot replied to Pickle's topic in PHP Coding Help
You'll need to check your PHP's settings for max_execution_time,max_input_time, memory_limit, post_max_size and upload_max_filesize as well as ensuring that the hidden form field containg the maximum file size is not exceeded. -
More information is required. How are you stroing these grades? Numerically? Or as in a school system (A,B,C etc). If it's the latter, how do you propose to average them? Are you giving each a numerical value? Is the data in a database?
-
Have a search for a pagination tutorial. You'll mostly come across these for mysql results, but the principles are the same. You'll need to read your includes into an array in the same order each time, then only use a selection of those for display depending on the page number.
-
[SOLVED] Count # of rows from MYSQL Query Result
GingerRobot replied to bsamson's topic in PHP Coding Help
You could use a subquery: SELECT score1+score2+score3 as totalscore,(SELECT COUNT(*)+1 FROM v2breakdown WHERE totalscore < score1+score2+score3) as position FROM v2breakdown WHERE DATE_FORMAT(FROM_UNIXTIME(`entrydate`), '%Y/%m/%d') >= '2008/05/01' ORDER BY totalscore ASC -
Can you show us a bit more of the code you're using? A still dont really follow. Is this the name of a form field?
-
You can't have a WHERE clause with an INSERT statement. Your inserting a new row, so there's nothing to compare pid with. Perhaps you wanted to UPDATE a row? UPDATE reff SET notes = '$mynotes' WHERE pid='$pid';
-
Perhaps it's just me, but i dont understand the question. What do you mean by that? You have a field in your form which sometimes contains double quotes? What do you mean you just like PHP to display the double quotes? My only guess is you wanted to get rid of the forward slash added by magic_quotes? If so, see stripslashes()
-
You can unset() the submit element from the array: <?php $file=fopen("response.txt","a+") or exit("Unable to open file!"); unset($_POST['submit']);//assuming your submit button has the name submit $data = implode('|', $_POST) . "\n"; fputs($file, $data); fclose($file); echo $data; ?>
-
Your function should not be making anything run slow - there's nothing in there which could cause any slow down. If your script is running slow then either a.)you have something else in your script or b.) your host is rubbish.
-
Use imagecopy() or, if you need any transparency effects, use imagecopymerge() Basically the idea would be to create an image of the desired size, then use the above functions to copy the smaller images onto the large image. I suspect this wouldn't be overly quick, however.
-
There's a very good FAQ/post here on just this very thing. You are correct that the best approach to use is to use the modulus operator to work out when to create your new rows. Your code is quite hard to read. Not sure you're opening and closing PHP with every line; it's certainly not necessary!
-
Why cant you get error reporting to work? Does your host not allow it? You could always install PHP on your computer and test locally. And yes, they are strings. However, if you place your string inside double quotes, then any variables will be evaluated. That is: <?php $foo = 'bar'; echo "$foo";//echos the text bar echo '$foo';//echos the text $foo ?> They do therefore need to be defined.
-
Im afraid there are an awful lot of errors in your script. If you place this line: error_reporting(E_ALL); At the top of your script, you'll see what i mean. The first lot are due to $a,$b and $c being undefined - you should move the creation of the $functions array to after they have been defined on these lines: $a = $_POST['A']; $b = $_POST['B']; $c = $_POST['C']; You also make use of the $functions variable inside your brute() function, but it is again undefined. If you're not quite sure why, you'll need to read up on variable scope. You should be passing that array to your function. $num also appears to be undefined on these lines: array_push ($num, brute($a, $c, $b));//i guess it should be $nums? There are other errors too, but im not entirely sure how this is supposed to work, so you'll need to fix those for yourself. On a side note, the caret(^) character cannot be used to raise two numbers to a power. You'll need PHP's pow() function for that.
-
Try: <?php $url = 'http://clickserve.cc-dt.com/link/click?lid=41000346346356262638103'; $parts = explode('/',$url); $newurl = $parts[0].'//'.$parts[2]; echo $newurl; ?>
-
Or even just: <select name="month"> <?php $monthno = date('n'); for($x=1;$x<=12;$x++){ $month = date('F',strtotime($x.'/01/08')); $selected = ($monthno == $x)? ' selected="selected" ':''; echo '<option value="'.$month.'"'.$selected.'>'.$month.'</option>'; } ?> </select>
-
What's the actual output from the above? Do you mean that nothing from this line: echo 'contactID = ' . $contactID; // nothing appeared, wonder why ? ! is displayed? In which case, the if statement is evaluating to false. You appear to be using get and post. Perhaps that is the problem?
-
Can anyone code my register page like this code?
GingerRobot replied to Robert Elsdon's topic in PHP Coding Help
So you're looking for someone to do this for you? You should head over to the freelance forum. This section is for people to help you with what you're trying to do yourself -
What are you trying to do? List all possible combinations? Of course this is going to take a long time. There are n^n possibilities. The number of possibilities is going to grow pretty rapidly as n increases. However sasa' code does not return all possible values. For example, proding two numbers, 1 and 2, it would not return 2,1 as a combination. The code provided appears to run relatively quickly for 12 numbers here.
-
The # is the mysql comment character - anything following is taken to be a comment. You should avoid giving your databases,tables or fields names with special characters or reserved words. You can get around the problem by placing the table name in backticks(`), but it would be better to change the table name. $query = "SELECT * FROM `#__events` WHERE state =1 ORDER BY publish_up RAND() LIMIT 1";
-
[SOLVED] How do I find the position of a regex match?
GingerRobot replied to jumpfroggy's topic in PHP Coding Help
Tried looking in the manual? The 4th parameter is what you need.